Send Tweets from Google Sheets using a Google Apps Script
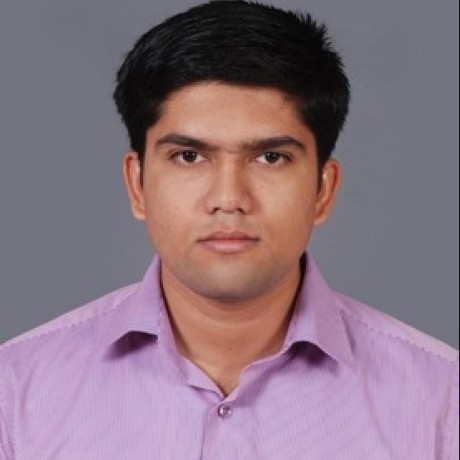
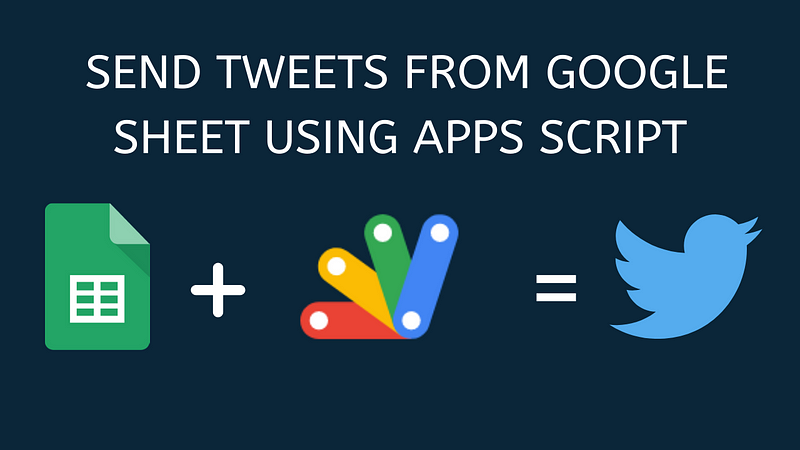
Source: Nidhinkumar
Overview
In this post, you will learn how to send automated tweets from Google Sheets to Twitter using Google Apps Script.
Objectives
What is Apps Script
Create a Twitter developer account
Create a Google Sheet and enable Apps Script
Integrate Twitterlib in Apps Script
Write the logic for the tweets
Tweet it
Prerequisites
- G Suite account
1. What is Apps Script
Google Apps Script is a rapid application development platform that makes it fast and easy to create business applications that integrate with G Suite. You can write code in modern JavaScript and have access to built-in libraries such as Gmail, Calendar, Drive, etc.,
2. Create a Twitter developer account
To send tweets we some API keys and tokens which we can get only if we have a Twitter developer account (if you have a Twitter developer account you can move to Step 3 :)
Create a Twitter developer account from this link. Click the Create button like below
Create an App
Fill the form with the app title, description, website link, and the purpose and click save (typically it would take some time).
Make sure you give the Read and Write permission for the app
once the app is approved you could see a page like below
Keys and Tokens
In the keys and tokens section, you will have the API, API secret, Access token, and Access token secret keys (copy those keys in your local machine).
Permissions
3. Create a Google Sheet and enable Apps Script
We will create some motivational quotes and try to send those quotes as daily tweets.
Open Google Sheets and add some quotes like below with a date field.
Quotes
Once the quotes are added click Tools -> Script Editor in Google Sheets.
Script Editor
Once you click the Script Editor you will see a window like below.
Script Editor
Here we will write the logic to take the data from Google Sheet and compare the current date and the date in the sheet. If it matches we will send the tweet.
4. Integrate Twitterlib in Apps Script
To send Tweets from Apps Script we are in need of the Twitterlib to do that click Resources -> Libraries and then add the below the number in the input box
MKvHYYdYA4G5JJHj7hxIcoh8V4oX7X1M_
and then click Add
Adding Twitter Lib
Select the latest version and click Save
Twitter Lib latest version
Now the Twitterlib has been added
5. Write the logic for the tweets
Now we will write the logic to send tweets by taking the data from Google Sheet and compare the current date with the date from Google sheet and if both the dates match, we will send the tweet.
First, we will get the active sheet and the rows
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
var startRowNumber = 1;
var endRowNumber = sheet.getLastRow();
Now we will copy the keys which we have done in Step 2 like below
var twitterKeys = {
TWITTER_CONSUMER_KEY: "//add your API key",
TWITTER_CONSUMER_SECRET: "//add your API secret key",
TWITTER_ACCESS_TOKEN: "//add your access token key",
TWITTER_ACCESS_SECRET: "//add your access token secret key",
}
Now we will initialize Twitterlib for authentication
var props = PropertiesService.getScriptProperties();
props.setProperties(twitterKeys);
var params = new Array(0);
var service = new Twitterlib.OAuth(props);
var quote;
var identifier;
Now we will have the final logic to get the data from the Google sheet and compare the dates, as well as whether the service has access to send tweets to Twitter
for (var currentRowNumber = startRowNumber; currentRowNumber <= endRowNumber; currentRowNumber++) {
var row = sheet.getRange(currentRowNumber + ":" + currentRowNumber).getValues();
// check that the second column (Date) is equal to today
if (isToday(row[0][1])) {
quote = row[0][0];
identifier = currentRowNumber - 1;
if (!service.hasAccess()) {
console.log("Authentication Failed");
} else {
console.log("Authentication Successful");
var status = quote + "\n\n" + "#Quotes #Motivation";
try {
var response = service.sendTweet(status, params);
console.log(response);
} catch (e) { console.log(e) }
}
break;
}
}
Compare Dates logic
function isToday(date) {
var today = new Date();
var dateFromRow = new Date(date);
return dateFromRow.getDate() == today.getDate() &&
dateFromRow.getMonth() == today.getMonth() &&
dateFromRow.getFullYear() == today.getFullYear()
}
Now the final code would be like below
6. Tweet it
Now if the run the function sendTweets() you will be asked for authorization like below
App script Login
App script permission
Once the function is executed you could see the output like below
Source: Nidhinkumar
Instead of manually triggering the function you can trigger it automatically like below
Click Edit -> Current Project’s Trigger which will open a new tab click Add Trigger which will open a pop-up modal like below
Trigger
Trigger
Click Save now it will automatically send tweets daily
Reference Links
TwitterLib — Click here to view
App Script Code — Click here to view
Congratulations!
You have learned how to send automated tweets from Google Sheet using Apps Script
Happy Learning :)
Subscribe to my newsletter
Read articles from nidhinkumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
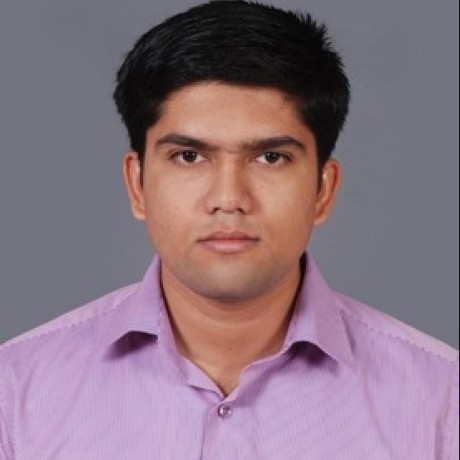