Cache Data and HTML on the Web Server's Disks

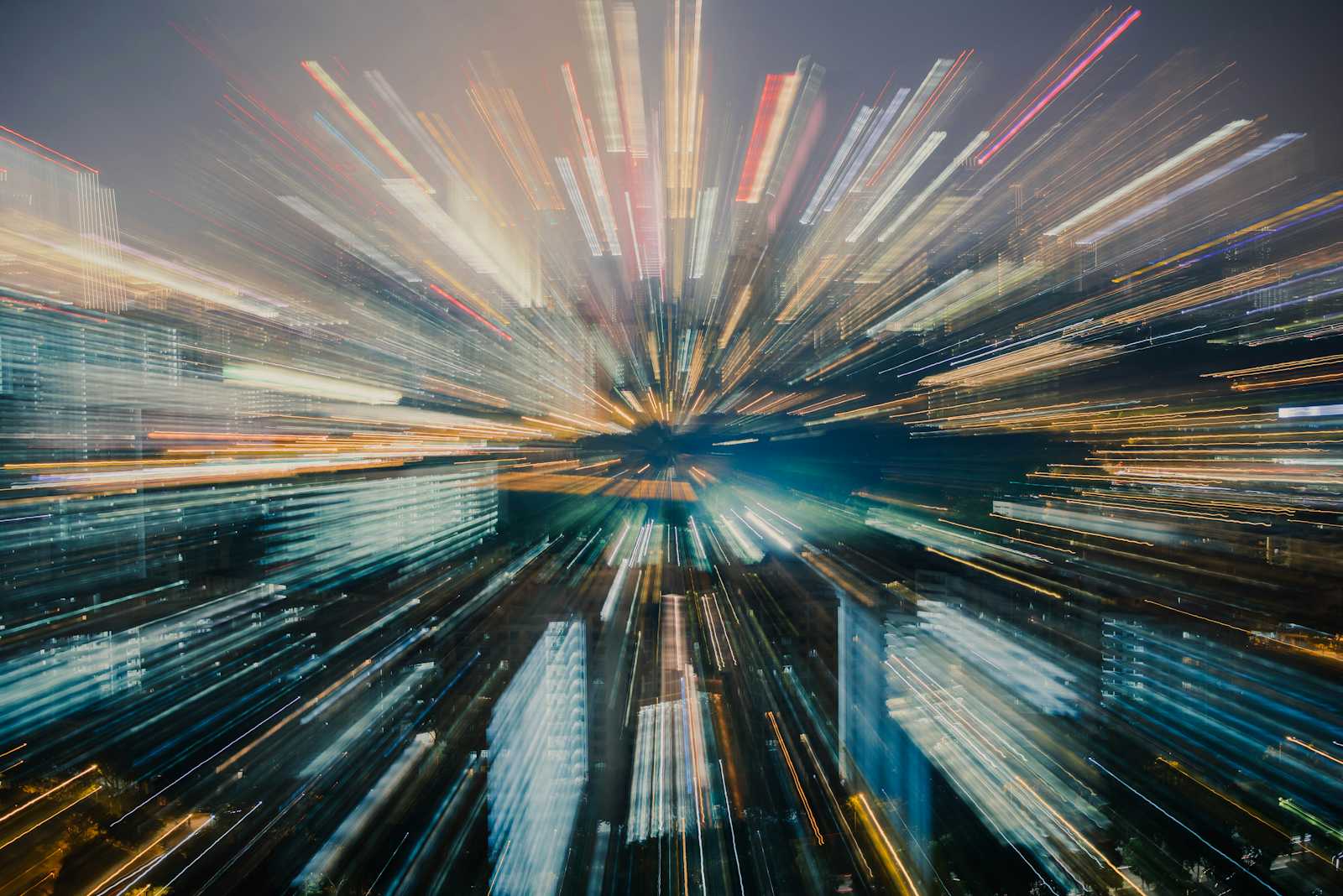
In the dynamic realm of web development, optimizing performance often involves judicious caching strategies. In this article, we delve into the world of disk-based caching in Classic ASP, exploring two powerful techniques: storing data as JSON and creating HTML cache files. These methods not only reduce database load but also pave the way for efficient retrieval and utilization of cached content.
Storing Data as JSON:
Classic ASP, with its rich set of tools, allows us to store data from a recordset as JSON, offering a structured and easily consumable format. Let's explore a practical example:
<%
Dim adoConn, adoRs, jsonData
Set adoConn = Server.CreateObject("ADODB.Connection")
adoConn.Open "your_connection_string"
Set adoRs = Server.CreateObject("ADODB.Recordset")
adoRs.Open "SELECT * FROM YourTable", adoConn
' Convert recordset to JSON
jsonData = JsonFromRecordset(adoRs)
' Save JSON to disk
SaveJsonToDisk jsonData, "cachedData.json"
' Close connections
adoRs.Close
adoConn.Close
' Custom functions for JSON handling
Function JsonFromRecordset(rs)
Dim json, field
json = "["
Do While Not rs.EOF
json = json & "{"
For Each field In rs.Fields
json = json & """" & field.Name & """:""" & Replace(field.Value, """", "\\""") & ""","
Next
json = Left(json, Len(json) - 1) & "},"
rs.MoveNext
Loop
If Len(json) > 1 Then json = Left(json, Len(json) - 1)
json = json & "]"
JsonFromRecordset = json
End Function
Sub SaveJsonToDisk(jsonData, fileName)
Dim fs, file
Set fs = Server.CreateObject("Scripting.FileSystemObject")
Set file = fs.CreateTextFile(Server.MapPath(fileName), True)
file.Write jsonData
file.Close
End Sub
%>
In this example, the JsonFromRecordset
function converts a recordset to JSON, and the SaveJsonToDisk
function saves the JSON data to a file named "cachedData.json" on the server's disk.
HTML Cache File for Efficient Content Delivery:
Another powerful caching strategy involves storing data from a table in an HTML cache file and using it to fill a specific section within a larger HTML file. This approach minimizes database interactions and optimizes content delivery. Let's illustrate this with a practical example:
<%
Dim adoConn, adoRs, htmlData, mainHtmlContent
Set adoConn = Server.CreateObject("ADODB.Connection")
adoConn.Open "your_connection_string"
Set adoRs = Server.CreateObject("ADODB.Recordset")
adoRs.Open "SELECT * FROM YourTable", adoConn
' Generate HTML content dynamically
htmlData = GenerateHtmlFromRecordset(adoRs)
' Save HTML data to a cache file
SaveHtmlToDisk htmlData, "cachedTableData.htm"
' Close connections
adoRs.Close
adoConn.Close
' Load the main HTML file
mainHtmlContent = ReadHtmlFromDisk("mainContent.htm")
' Insert the cached table data into the main HTML
mainHtmlContent = Replace(mainHtmlContent, "<!-- INSERT_TABLE_DATA -->", htmlData)
' Output the final HTML content
Response.Write mainHtmlContent
' Custom functions for HTML handling
Function GenerateHtmlFromRecordset(rs)
' Logic to generate HTML from recordset data
End Function
Sub SaveHtmlToDisk(htmlData, fileName)
Dim fs, file
Set fs = Server.CreateObject("Scripting.FileSystemObject")
Set file = fs.CreateTextFile(Server.MapPath(fileName), True)
file.Write htmlData
file.Close
End Sub
Function ReadHtmlFromDisk(fileName)
Dim fs, file, content
Set fs = Server.CreateObject("Scripting.FileSystemObject")
Set file = fs.OpenTextFile(Server.MapPath(fileName), 1)
content = file.ReadAll
file.Close
ReadHtmlFromDisk = content
End Function
%>
In this example, the GenerateHtmlFromRecordset
function creates HTML content from the table data, which is then saved to a cache file named "cachedTableData.htm." The main HTML file is read from disk, and the cached table data is inserted into the designated section, enhancing the overall efficiency of content rendering.
Conclusion:
Mastering disk-based caching in Classic ASP involves leveraging versatile techniques such as storing data as JSON and creating HTML cache files. These approaches not only reduce the burden on the database server but also enhance the overall performance of web applications. Whether it's structured data in JSON format or pre-rendered HTML cached on disk, Classic ASP empowers developers to implement caching strategies that significantly optimize content delivery.
More resources: https://axcs.blogspot.com/2006/07/asp-tips-tip-3-cache-data-and-html-on.html
Subscribe to my newsletter
Read articles from Edward Anil Joseph directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
