[Part-4] React Router and Navigation: Navigating Through Single Page Applications
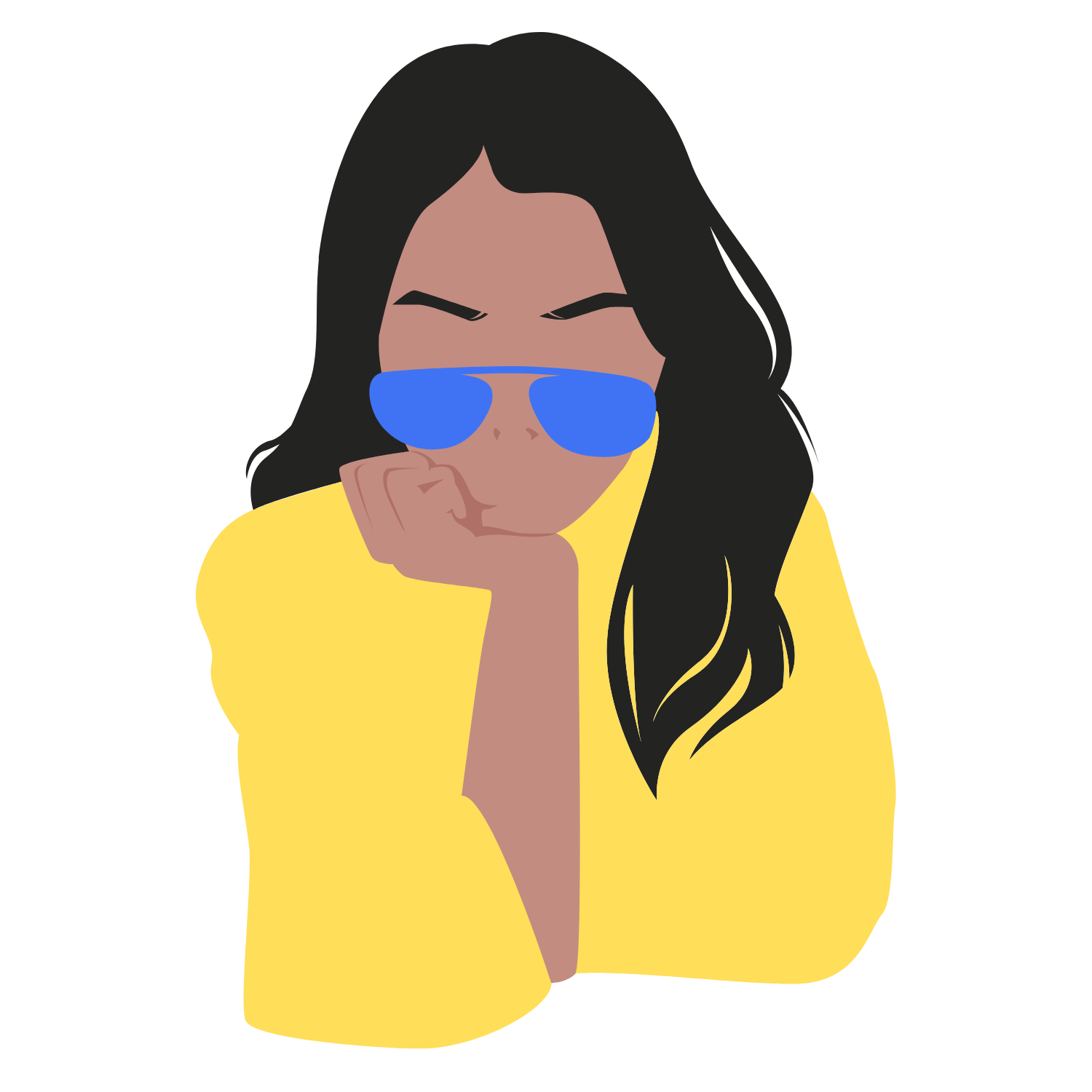
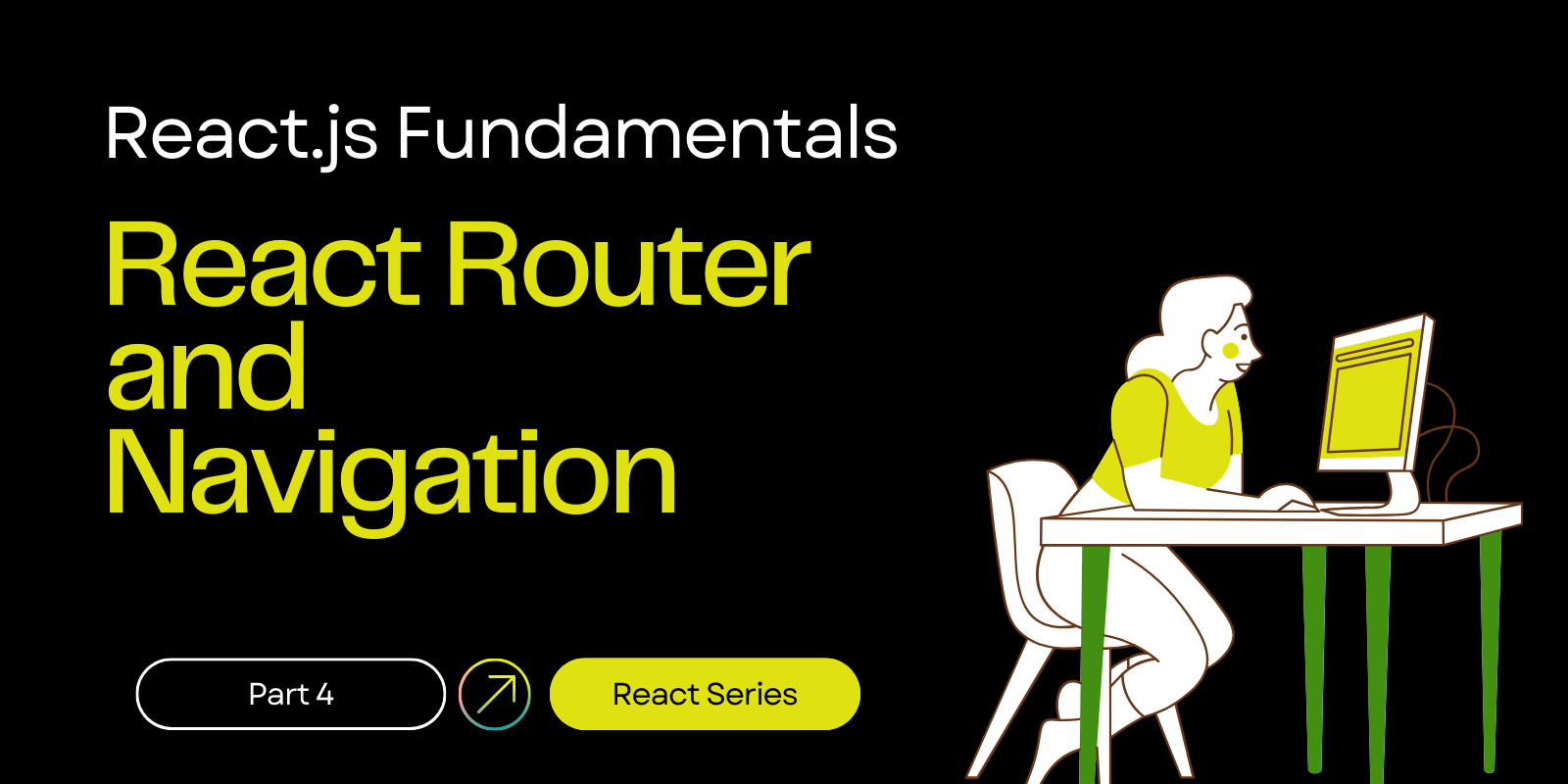
React, known for its component-based architecture, is commonly used to build single-page applications (SPAs). In SPAs, navigation plays a crucial role in providing a seamless and dynamic user experience. React Router is a popular library that simplifies navigation in React applications, allowing developers to create a smooth and responsive navigation system.
Understanding React Router
React Router is a declarative routing library for React applications. It enables the navigation of different components while maintaining a single-page application architecture. React Router provides a set of navigational components and a dynamic routing system, allowing developers to define how the application should navigate based on the URL.
Installation
To get started with React Router, you need to install the package.
npm install react-router-dom
Basic Usage
React Router provides several components to define routes and navigation. The BrowserRouter
is commonly used to wrap the entire application, providing the routing context.
// index.js
import React from 'react';
import ReactDOM from 'react-dom';
import { BrowserRouter } from 'react-router-dom';
import App from './App';
ReactDOM.render(
<BrowserRouter>
<App />
</BrowserRouter>,
document.getElementById('root')
);
In the example above, the BrowserRouter
is used to wrap the App
component, making the routing functionality available throughout the application.
Route Component
The Route
component is a fundamental building block of React Router. It renders some UI if the current location matches the route’s path.
// App.js
import React from 'react';
import { Route, Switch } from 'react-router-dom';
import Home from './Home';
import About from './About';
const App = () => {
return (
<div>
<Switch>
<Route path="/about" component={About} />
<Route path="/" component={Home} />
</Switch>
</div>
);
};
export default App;
In this example, the Switch
component is used to render the first Route
that matches the current location. If no Route
matches, a "404 Not Found" page can be rendered.
Link Component
The Link
component is used to navigate between different routes, ensuring a smooth transition without a full page reload.
// Home.js
import React from 'react';
import { Link } from 'react-router-dom';
const Home = () => {
return (
<div>
<h2>Home</h2>
<Link to="/about">Go to About</Link>
</div>
);
};
export default Home;
In the example above, clicking the "Go to About" link will navigate to the /about
route without reloading the entire page.
Route Parameters
React Router allows the definition of dynamic route parameters, enabling the creation of flexible and reusable components.
// App.js
import React from 'react';
import { Route, Switch } from 'react-router-dom';
import Home from './Home';
import UserProfile from './UserProfile';
const App = () => {
return (
<div>
<Switch>
<Route path="/user/:id" component={UserProfile} />
<Route path="/" component={Home} />
</Switch>
</div>
);
};
export default App;
In this example, the :id
in the route path is a parameter that can be accessed in the UserProfile
component.
// UserProfile.js
import React from 'react';
const UserProfile = ({ match }) => {
const userId = match.params.id;
return (
<div>
<h2>User Profile</h2>
<p>User ID: {userId}</p>
</div>
);
};
export default UserProfile;
Nested Routes
React Router supports nested routes, allowing the creation of complex navigational structures.
// App.js
import React from 'react';
import { Route, Switch } from 'react-router-dom';
import Home from './Home';
import UserProfile from './UserProfile';
const App = () => {
return (
<div>
<Switch>
<Route path="/user/:id" component={UserProfile} />
<Route path="/" component={Home} />
</Switch>
</div>
);
};
export default App;
In this example, the UserProfile
component can be a parent component for additional nested routes.
// UserProfile.js
import React from 'react';
import { Route, Link } from 'react-router-dom';
import UserPosts from './UserPosts';
const UserProfile = ({ match }) => {
const userId = match.params.id;
return (
<div>
<h2>User Profile</h2>
<p>User ID: {userId}</p>
<nav>
<ul>
<li>
<Link to={`/user/${userId}/posts`}>User Posts</Link>
</li>
</ul>
</nav>
<Route path={`/user/${userId}/posts`} component={UserPosts} />
</div>
);
};
export default UserProfile;
In this example, clicking the "User Posts" link will render the UserPosts
component as a nested route under UserProfile
.
Programmatic Navigation
React Router provides a history
object, allowing programmatic navigation through the application.
// NavigationButton.js
import React from 'react';
import { withRouter } from 'react-router-dom';
const NavigationButton = ({ history }) => {
const handleClick = () => {
// Programmatic navigation to the /about route
history.push('/about');
};
return (
<button onClick={handleClick}>Go to About</button>
);
};
export default withRouter(NavigationButton);
In this example, the history.push('/about')
line programmatically navigates to the /about
route.
Conclusion
React Router is a powerful tool for handling navigation in React applications. Whether you're building a simple portfolio site or a complex web application, React Router provides the flexibility and tools needed to create a seamless and dynamic user experience. Understanding the basics of routing, handling parameters, nested routes, and programmatic
Subscribe to my newsletter
Read articles from Anisha Swain | The UI Girl directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
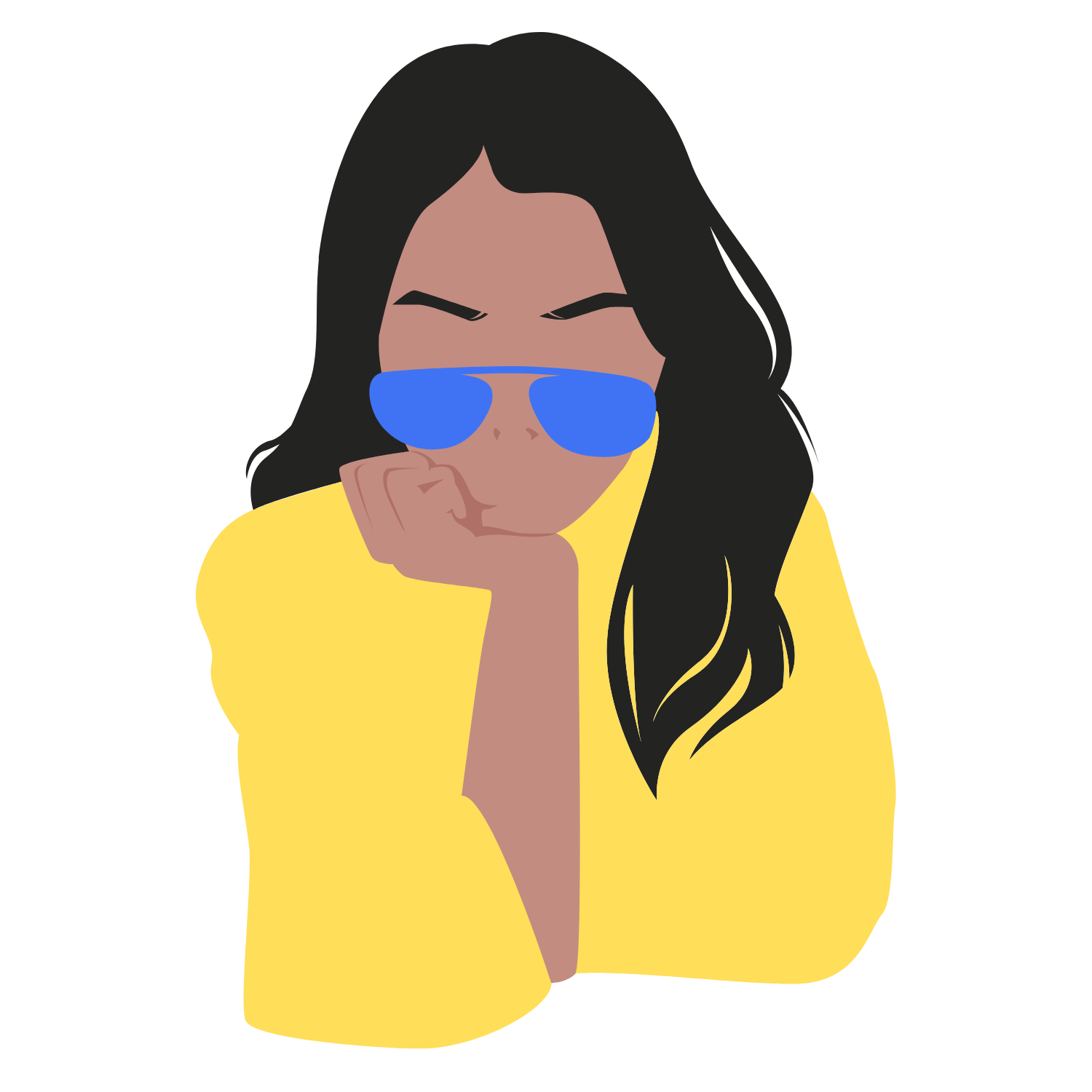
Anisha Swain | The UI Girl
Anisha Swain | The UI Girl
Anisha Swain | The UI Girl Hello world! Anisha this side👋 💪Making @theuigirl 💻 speaker http://t.ly/9D22 🍀 1:1 https://topmate.io/anishaswain 🎙️ podcast host http://t.ly/_MUml ✏️ blog https://medium.com/the-ui-girl 🧳 Travel story http://t.ly/Xa-5v https://bento.me/anishaswain Let's connect 🤝