Batching of multiple render methods
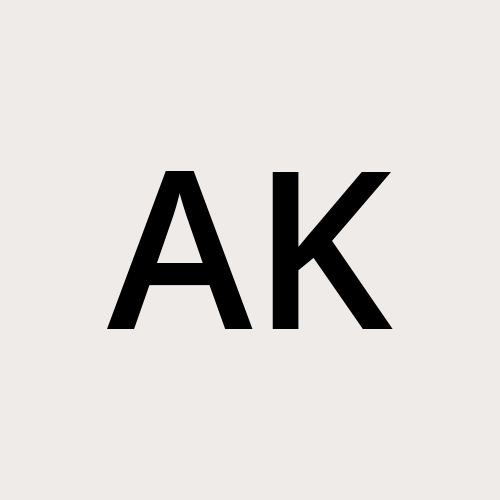
import React from 'react';
import UserClass from "./UserClass";
import UserClass2 from "./UserClass2";
const userData = [
{
name: "Ankur Kashyap",
location: "Bengaluru",
contact: "abc"
},
{
name: "Manjulika Mondal",
location: "Bengaluru",
contact: "def"
}
];
class About extends React.Component{
constructor(props) {
super(props);
console.log("parent constructor");
}
componentDidMount() {
console.log("parent componentDidMount");
}
render() {
console.log("parent render");
return (
<div>
<h1>About</h1>
<h2>This is Namaste React Web Series</h2>
<UserClass userData={userData} name="child 1" />
<UserClass userData={userData} name="child 2" />
<UserClass2 userData={userData} name="child 3" />
</div>
)
}
}
export default About;
import React from 'react';
class UserClass extends React.Component{
constructor(props) {
super(props);
this.state = {
count: 0
};
console.log(this.props.name + " constructor");
}
componentDidMount() {
console.log(this.props.name + " componentDidMount");
}
render() {
console.log(this.props.name + " render");
const { userData } = this.props;
const { count } = this.state;
return (
<>
{userData.map((data) => (
<div className="user-card">
<h2>{count}</h2>
<button
onClick={() => {
this.setState({
count: this.state.count + 1
})
}}
>Count Increase</button>
<h2>Name: {data.name}</h2>
<h3>Location: {data.location}</h3>
<h3>Contact: {data.contact}</h3>
</div>
))}
</>
)
}
};
export default UserClass;
import React from 'react';
class UserClass2 extends React.Component{
constructor(props) {
super(props);
this.state = {
count: 0
};
console.log(this.props.name + " constructor");
}
componentDidMount() {
console.log(this.props.name + " componentDidMount");
}
render() {
console.log(this.props.name + " render");
const { userData } = this.props;
const { count } = this.state;
return (
<>
{userData.map((data) => (
<div className="user-card">
<h2>{count}</h2>
<button
onClick={() => {
this.setState({
count: this.state.count + 1
})
}}
>Count Increase</button>
<h2>Name: {data.name}</h2>
<h3>Location: {data.location}</h3>
<h3>Contact: {data.contact}</h3>
</div>
))}
</>
)
}
};
export default UserClass2;
In the example above, we have one parent component which is having three child components. In parent component, we have console.log in constructor, render method and in componentDidMount(). Same console.log is present inside the three children components.
So, here how does life cycle method works?
First, console.log() is executed inside constructor() of the parent component, then render method and when it encounters the first child component, it executes console.log() of first child component's constructor() and then render() method and after that it executes console.log() of second child's component's constructor() and then render() method and after that third child component's constructor() and then render() method. At last it executes all the componentDidMount() methods of first, second and third children. After all the Child components are executed, finally the parent component's componentDidMount() method is called.
Did you notice that inside first child component, instead of executing the componentDidMount() exactly after the render() method and before the execution of second child component's constructor() method, it executed all the children render() methods first and then at last it executed all the componentDidMount()?
This is because, when you have a parent component with multiple child components, the rendering and lifecycle methods are not guaranteed to be executed in a specific order for each child component. React optimizes rendering by batching updates and making them asynchronous. This means that React may choose to update and render components in batches for performance reasons. To understand more, please refer to the Render method and Reconciliation article.
Subscribe to my newsletter
Read articles from Ankur Kashyap directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
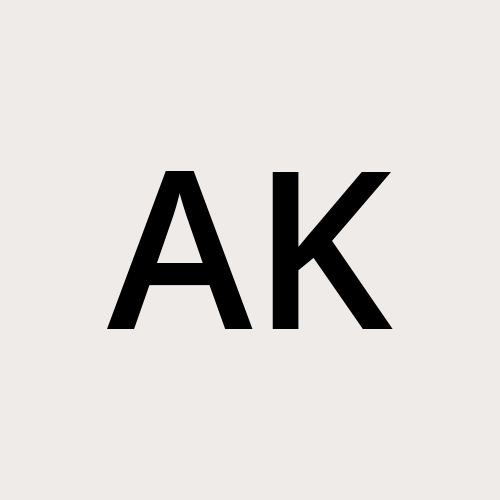
Ankur Kashyap
Ankur Kashyap
I am a Software Engineer