Let's FizzBuzz together (Part 1)

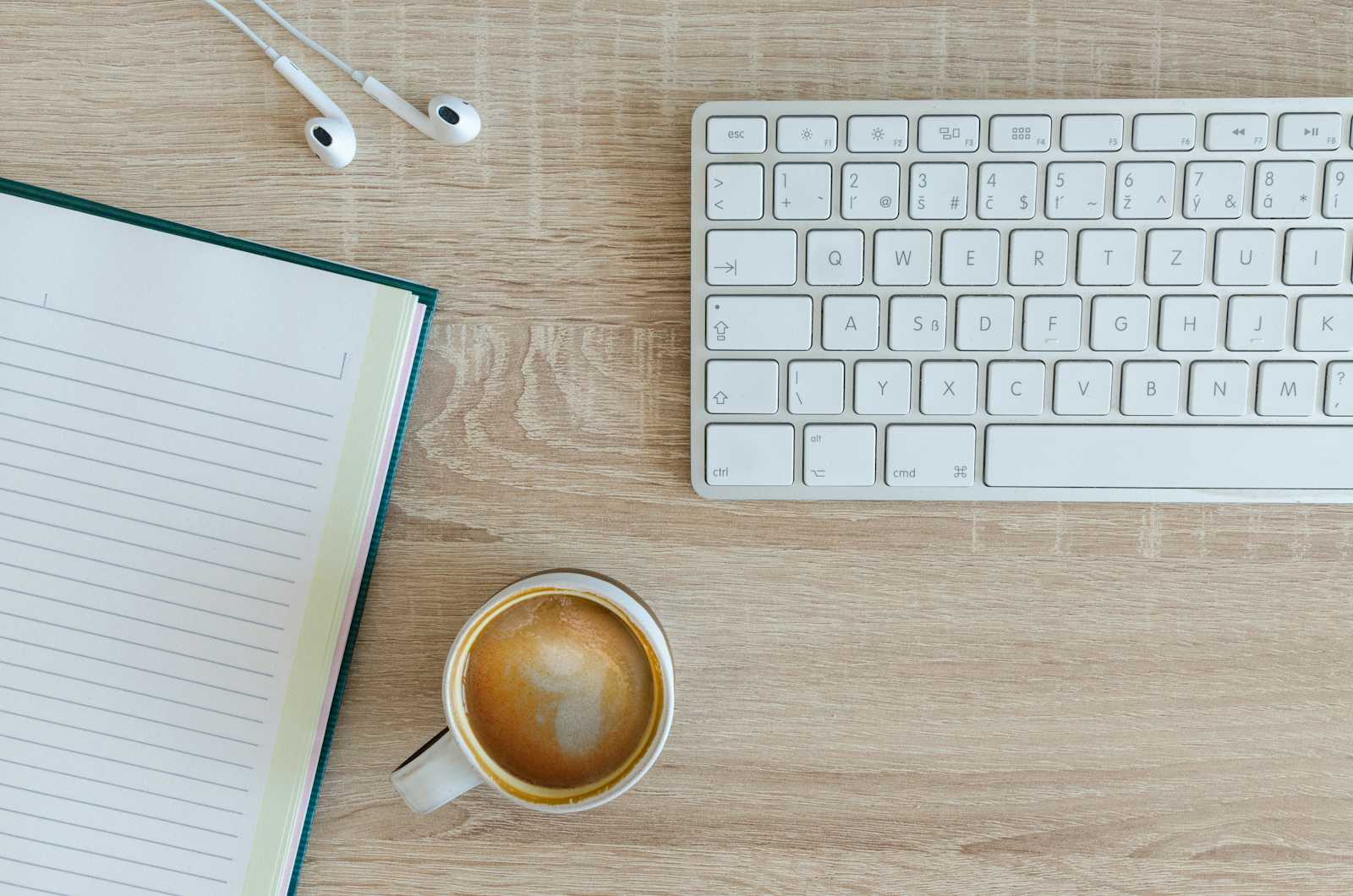
Introduction
Getting into the habit of writing code tests is something I've been wanting to become better at to strengthen my skills as a developer. Until now, my focus has been on front-end development so it didn't necessarily make sense to write tests ensuring a particular element displayed correctly or if colors followed brand guidelines. All of which is to say: Most of the labs in my coursework have been structured to teach software development from a Test-Driven Development methodology and I'm excited to practice working this way. While I won't be writing my own unit tests, I will be getting solid experience developing using this structured approach.
Ok, but what is TDD?
A lot hasbeen writtenon the history of TDD so I encourage you to read more about it on your own. Rather than trying to present an exhaustive article on the topic, I'll focus on defining what it is and how it is useful to me.
Test-driven development is a software development process where defined requirements are converted to tests before writing code, writing code until it passes the test, refactoring the code, and then continuing this cycle until all of the requirements have been met. This means that rather than writing code to meet a list of requirements and then testing, the steps of testing and development are done in parallel, iteratively.
Test Driven Development cycle via Wikimedia Commons
Ok, but why use TDD?
There are many benefits of practicing TDD. Personally, it feels useful as a method to stay focused on solving for functionality. It forces you to cut down to "What are the bare bones to meet the requirement?" As someone who can (and will) spend hours nudging pixels to make everything look justright, It's helpful to have constraints and a defined outcome.
What does this look like in practice?
Let's walk through the FizzBuzz problem using TDD to learn how it works. This walkthrough is in vanilla javascript using jest for unit testing.
Define the Requirements
Here's the problem statement directly from HackerRank*.
Write a short program that prints each number from 1 to 15 on a new line.
For each multiple of 3, print "Fizz" instead of the number.
For each multiple of 5, print "Buzz" instead of the number.
For numbers which are multiples of both 3 and 5, print "FizzBuzz" instead of the number.*
*Shortened range to 1-15 for the sake of brevity
Think about the approach and possible tests for requirements
The solution needs to print out a set of numbers. A looping method could be useful since we know we'll have to iterate through the set. We know that the numbers increment by 1 as they progress so it may not be necessary to define an array, it may be sufficient to define when the loop should start and stop. The first test could check for 15 lines of responses. The test could be vague (test for 15 responses) or specific (expect 1 2 Fizz
etc).
Let's take into account the other requirements before writing the first unit test. We might use an if...else statement to check for the remaining conditions. We can expect the output for 3, 6, 9, and 12 to be "Fizz". Similarly, for the next requirement, we can check that 5 or 10 show as "Buzz".
As for the last requirement, we'll expect 15 to be our only "FizzBuzz" since it's the only number in our set divisible by both 3 and 5. If we think about 15 as a value that could return "true" for either of the "fizz" or "buzz" clauses of the if statement, we might want to set that clause higher up in the if statement to catch the most complex requirement before checking for simpler conditions.
We expect the final output to be:
1
2
fizz
4
buzz
fizz
7
8
Fizz
Buzz
11
Fizz
13
14
FizzBuzz
Write the first test (expect it to fail)
Let's have the first test check for a function called fizzBuzz.
Run your test
We haven't started writing code for fizzBuzz yet so we expect the first test to fail.
You can try it yourself by running npm test
.
Write just enough code so that it will pass the test
Let's go ahead and declare fizzBuzz in our fizzBuzz.js file.
Run the test again
Now, let's try running the test again. Here's what we get back:
This post is the first of a multi-part series, so stay tuned for the next round of TDD where we write a unit test to check that numbers divisible by 3 return "Fizz".
Do you have a good idea of what that test will look like? Feel free to comment below.
Subscribe to my newsletter
Read articles from Peggy Wang directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Peggy Wang
Peggy Wang
I'm currently a student enrolled in Flatiron School's Software Engineering bootcamp. With a background in frontend web development, I'm taking steps towards becoming a Full-stack Developer and writing about it along the way. When I'm not thinking about code, you'll find me listening to an audiobook, working on a jigsaw puzzle, or looking for the best snacks.