What is the difference between while, do-while and for loops in JavaScript ?

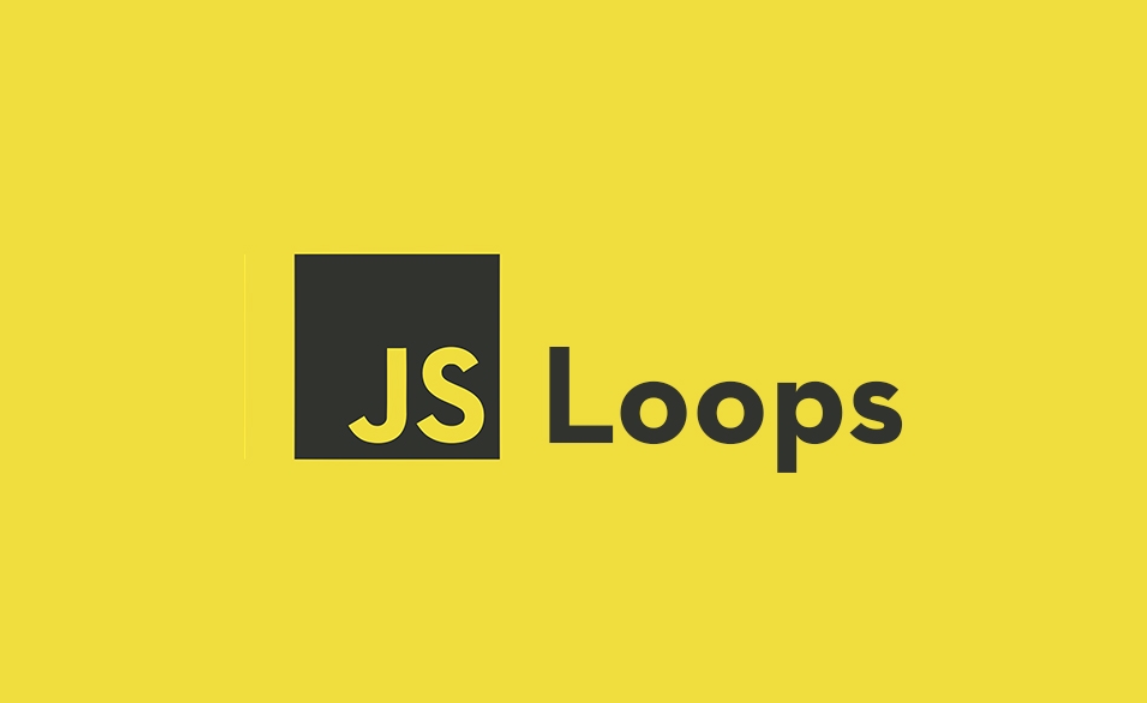
Loops are powerful control flow statements in JavaScript that allow you to execute a block of code repeatedly until a certain condition is met.
1. While Loop:
The while loop repeatedly executes a block of code as long as the specified condition is true.
The condition is checked before entering the loop.
Syntax:
while (condition) {
// code to be executed
}
Execution:
The
condition
is evaluated.If
condition
is true, the loop body is executed.Repeat steps 1 and 2 until
condition
becomes false.
Use cases:
When you don't know the exact number of iterations beforehand.
When you need to keep iterating as long as a certain condition is met.
Example: Reading user input until they enter "stop":
let input = ""; while (input !== "stop") { input = prompt("Enter something (or 'stop' to quit): "); console.log("You entered:", input); }
2. Do-While Loop:
The do-while loop is similar to the while loop, but the condition is checked after the execution of the block of code.
This ensures that the code inside the loop is executed at least once, even if the condition is initially false.
Syntax:
do {
// code to be executed
} while (condition);
Execution:
The loop body is executed at least once.
Then, the
condition
is evaluated.If
condition
is true, repeat steps 1 and 2.Stop when
condition
becomes false.
Use cases:
When you want to ensure the loop body is executed at least once, even if
condition
is initially false.When you need to perform an action before checking a condition.
Example: Asking the user for a number between 1 and 10 until they enter a valid one:
let number; do { number = parseInt(prompt("Enter a number between 1 and 10: ")); } while (number < 1 || number > 10); console.log("You entered:", number);
3. For Loop:
The for loop is used for iterating over a sequence or for a known number of iterations.
It consists of three parts: initialization (executed before the loop starts), condition (checked before each iteration), and iteration (executed after each iteration).
The initialization, condition, and iteration can all include multiple expressions separated by commas.
Syntax:
for (initialization; condition; iteration) {
// code to be executed
}
Execution:
initialization
is executed (usually used to set a loop counter).condition
is evaluated.If
condition
is true, the loop body is executed.increment
is executed (usually used to update the loop counter).Repeat steps 2-4 until
condition
becomes false.
Use cases:
When you know the exact number of iterations in advance.
When you need to combine initialization, condition check, and increment in a single construct.
Example: Printing numbers from 1 to 5:
for (let i = 1; i <= 5; i++) { console.log(i); }
Example of using each loop in JavaScript:
// While Loop
let count = 0;
while (count < 5) {
console.log(count);
count++;
}
// Do-While Loop
let count2 = 0;
do {
console.log(count2);
count2++;
} while (count2 < 5);
// For Loop
for (let i = 0; i < 5; i++) {
console.log(i);
}
These loop constructs in JavaScript serve similar purposes as in other programming languages, providing flexibility for different looping scenarios. It's essential to understand the specific syntax and behavior of each loop type to use them effectively in your code.
Key Differences:
Feature | While Loop | Do-While Loop | For Loop |
Condition | Checked before | Checked after | Checked before |
Minimum Run | None | At least once | Defined by init |
Use Cases | Unknown iterations, long-running conditions | At least once execution, pre-checking actions | Exact iterations, structured iteration |
Choosing the Right Loop:
If you know the number of iterations and want a structured approach, use
for
.If you want to execute the loop body at least once, use
do-while
.If you have an unknown number of iterations or a long-running condition, use
while
.
MDN References
Subscribe to my newsletter
Read articles from Bharat Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Bharat Kumar
Bharat Kumar
I am a frontend developer.