Simple JS website (no frame works / libraries)
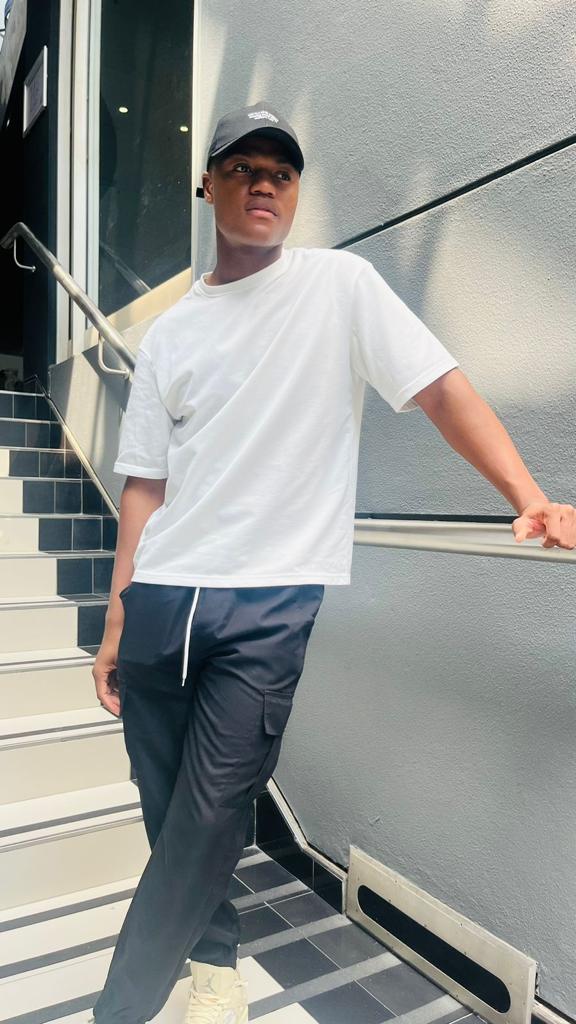
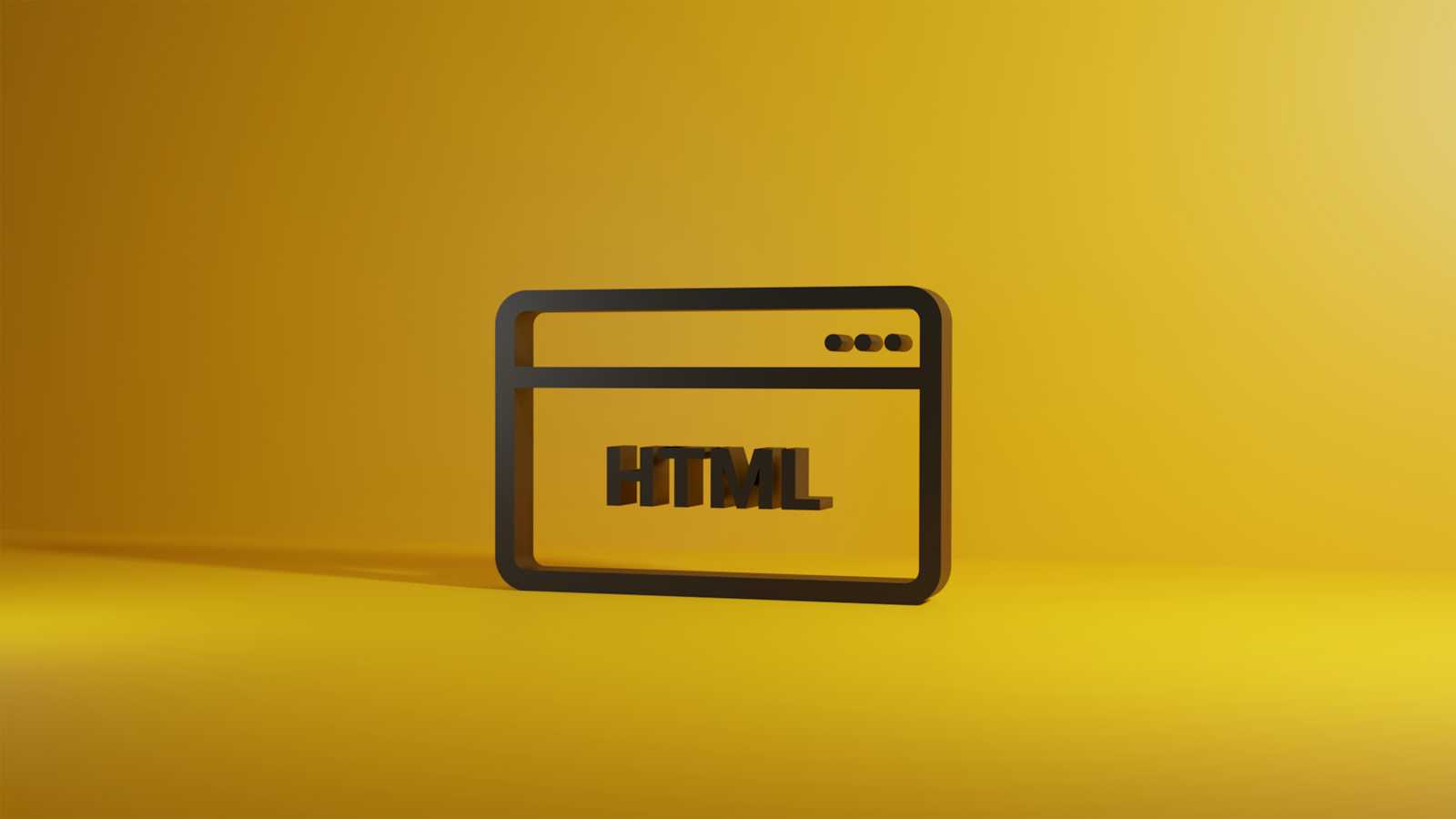
<!DOCTYPE html>
<html>
<head>
<title>Form</title>
<style>
body {
margin: 0;
padding: 0;
font-family: sans-serif;
background-image: url(background.jpg);
background-position: center;
}
.Invalid_Messages {
color: yellow;
visibility: hidden;
}
.form {
width: 300px;
box-shadow: 0 3px rgba(0, 0, 0, 0.3);
background: #fff;
padding: 20px;
margin: 8% auto 0;
text-align: center;
padding-bottom: -20px;
}
</style>
</head>
<body>
<div class="Invalid_Messages">
<label id="EmailError">Please enter a valid email address (should contain "@")</label><br>
<label id="NameError">First name must start with a capital letter</label><br>
<label id="CellNoError">Please enter a valid cell phone number</label><br>
<label id="GenderError">Please select your gender</label><br>
<label id="LocationError">Location was not selected</label><br>
<label id="AddressError">Address should contain numbers and text</label><br>
<label id="LastNameError">Last name must start with a capital letter</label>
</div>
<div class="form">
<h1>Sign Up Now!</h1>
<img src="logo (2).jpg" height="300px" width="300px">
<form action="message.html" onsubmit="return main()">
<label>First Name</label>
<input id="First_Name" type="text" placeholder="First Name" required><br><br>
<label>Last Name</label>
<input id="Last_Name" type="text" placeholder="Last Name" required><br><br>
<label>Address</label>
<input type="text" placeholder="Address" id="Address" required><br><br>
<div class="Gender">
<label>Gender</label>
<input type="radio" name="Gender" id="Male" required>Male
<input type="radio" name="Gender" id="Female" required>Female
</div><br><br>
<label>Email:</label>
<input id="Email" type="email" placeholder="example@gmail.com" required><br><br>
<label>Mobile</label>
<input id="Mobile_Number" type="text" required><br><br>
<label>Location</label>
<select id="Locations">
<option value="">Select location</option>
<option value="boardwalk">Boardwalk</option>
<option value="brooklyn">Brooklyn</option>
<option value="lynwood">Lynwood</option>
<option value="sunnyside">Sunnyside</option>
</select><br><br>
<input type="submit" id="submit">
<button type="button" id="Reset">Reset</button>
</form>
</div>
<script type="text/javascript">
function Sign() {
// Validation logic
}
function cookie(name, value, daysToLive) {
const date = new Date();
date.setTime(date.getTime() + (daysToLive * 24 * 60 * 60 * 1000));
let expires = "expires=" + date.toUTCString();
document.cookie = `${name}=${value};${expires};path=/`;
}
function main() {
if (Sign()) {
const firstName = document.querySelector("#First_Name").value;
const email = document.querySelector("#Email").value;
const phone = document.querySelector("#Mobile_Number").value;
cookie("FirstName", firstName, 365);
cookie("email", email, 365);
cookie("mobile number", phone, 365);
console.log("Form was submitted");
return true;
} else {
return false;
}
}
// Reset button functionality
document.getElementById('Reset').addEventListener('click', function () {
document.querySelector('form').reset();
});
</script>
</body>
</html>
This code simply does this:
HTML Structure:
The HTML code represents a sign-up form for users. It includes input fields for first name, last name, address, gender, email, mobile number, and location. There's a separate div element with the class Invalid_Messages to display custom error messages. Each error message is labeled with an ID to target them individually.
NB : The images and the html file are in the same folder.
CSS Styling:
The CSS styles define the appearance of the form elements and error messages. The background image is set for the body.
JavaScript Functionality:
Form Validation (Sign() Function):
The Sign() function is supposed to contain validation logic for the form fields. However, it's left empty in the provided code. Typically, this function would check each input field for validity based on certain criteria, such as whether the first name starts with a capital letter, if the email format is correct, etc. Cookie Handling (cookie() Function):
The cookie() function is responsible for setting cookies with the provided name, value, and expiration duration. It creates a Date object to calculate the expiration date of the cookie. The cookies are set for the first name, email, and mobile number upon form submission (main() function). Main Function (main()):
The main() function is called when the form is submitted. It should call the Sign() function to perform form validation. If validation passes, it sets cookies with the form data. If validation fails, the form submission is prevented. Reset Button Functionality:
The reset button with the ID Reset has an event listener attached to it. When clicked, it resets the form fields using the reset() method on the form element.
Purpose of Invalid_Messages div:
The Invalid_Messages div serves to display custom error messages. Each error message is hidden by default (visibility: hidden) and can be made visible based on the validation logic within the Sign() function (which is not implemented in the provided code). In summary, while the HTML structure and JavaScript setup are in place, the Sign() function needs to be completed with appropriate validation logic to enable form validation and error message display.
The output of the project:
Conclusion:
This was a very cool JS project for me as a beginner , because on this project I managed to learn the DOM, and get deep understanding also about cookies and error handling ,so if you are a beginner you can try it out also and see for yourself if it works NB this project does not save the info into a database it is just javascript only without any additional backend proccesses.
Subscribe to my newsletter
Read articles from Yinhla Eddy NCenya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
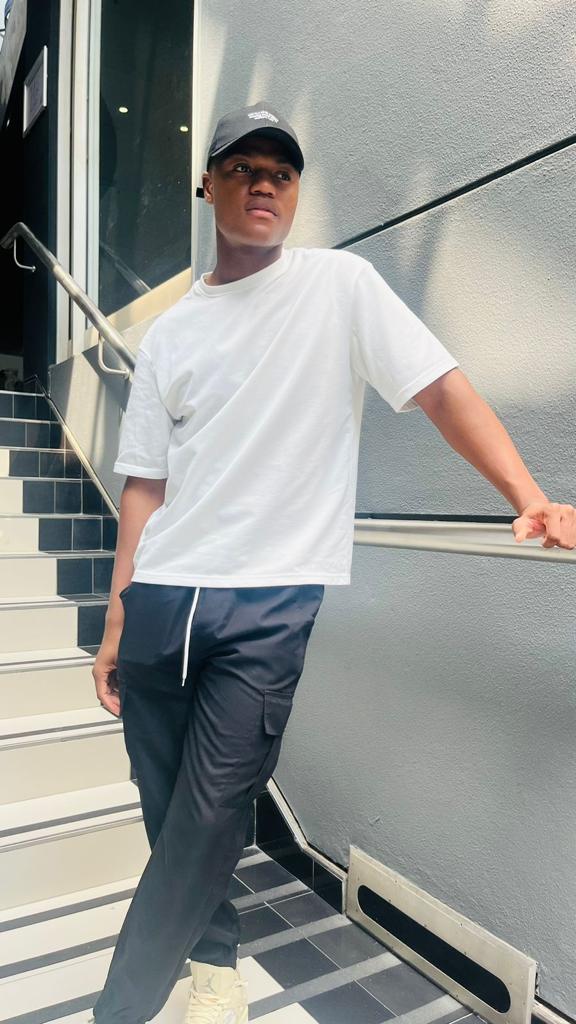
Yinhla Eddy NCenya
Yinhla Eddy NCenya
Candidly, I'm a second-year student at Richfield College with a heart that beats for programming. Admittedly, I'm still on the journey to mastering it, but next year, in my final year, I'm determined to hustle hard and build a profile that's, well, impressive enough to help others endure the delightful torment of programming (yes, I said it, 'delightful torment'). Let's embrace the quirks, the bugs, and the 'why-did-I-even-start-this' moments together, and maybe, just maybe, we'll find ourselves laughing at the sheer audacity of it all!