Elevate Your Code: Exploring the Significance of Function Purity, Pure Function Design, and Composition
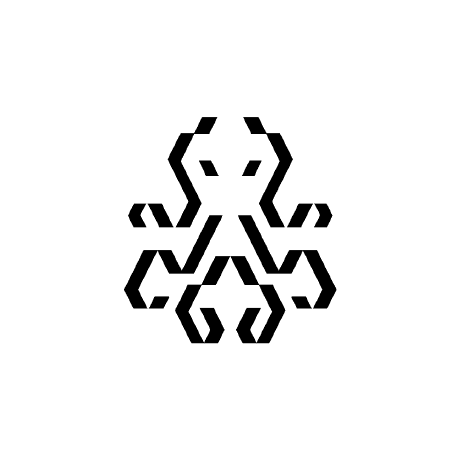
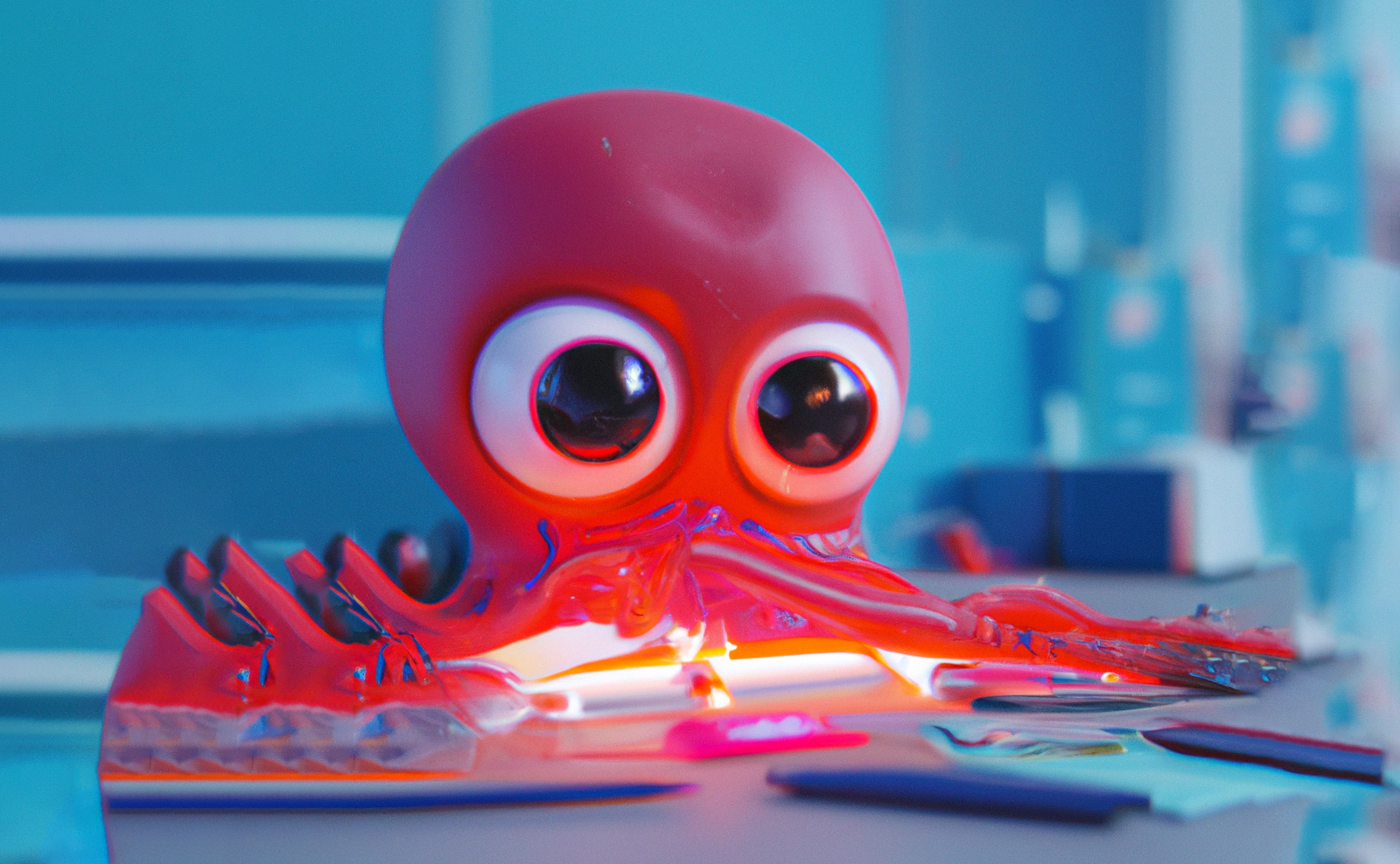
Introduction
Functional programming is a paradigm of computer programming that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. It emphasizes the application of functions, in contrast to the imperative programming paradigm, which emphasizes changes in state and the execution of sequential commands.
FP has its roots in mathematics, particularly lambda calculus, and has been prevalent in academia for decades. In functional programming, functions are first-class citizens and can be passed around and used like any other data. It aims for functions with no side effects, which means that they don't alter the state or have an observable interaction with other functions or the outside world except through their return value.
This leads to several benefits, such as clearer code due to the absence of side effects, easier-to-reason-about programs, often making FP a good fit for concurrent and parallel programming. Functional concepts have also been integrated into many mainstream programming languages, including C# and Java, with features like lambda expressions, higher-order functions, and immutability .
Why Function Purity Matters?
Function purity matters for several reasons, which enhance the reliability, maintainability, and clarity of code:
Predictability: Pure functions always produce the same output given the same input, without relying on or altering the state of the program. This makes them predictable and easier to reason about.
No Side Effects: Since pure functions don’t have side effects, they don’t change any state or cause unexpected behavior elsewhere in the system. This reduces the chance of bugs related to shared mutable state.
Concurrency and Parallelism: Pure functions can be parallelized or run concurrently without the risk of race conditions because they do not share state with other functions. This is increasingly important for performance as systems utilize multicore processors more intensively.
Testability: Pure functions are easier to test because they do not require context or the setting up of a particular state. You can test them in isolation, which makes writing unit tests simpler.
Caching and Memoization: Pure functions lend themselves to techniques like memoization, where the result of a function call is cached and reused for identical calls without re-computation, optimizing performance.
Refactoring: Code refactoring is less hazardous because functions can be modified or replaced without worrying about unintended consequences in unrelated parts of the program.
These advantages contribute to the robustness and simplicity of software, and they align with best practices in software development. These principles are covered extensively in the functional programming paradigm, including in the book "Functional Programming in C#: How to write better C# code" by Enrico Buonanno .
Designing Pure Functions and signature types (in C#)
Designing function signatures and types in the context of functional programming involves creating clear and expressive declarations for functions that convey their intent, the nature of the data they operate on, and the kind of computation they perform. Here’s a brief overview of how function signatures and types can be designed effectively:
Expressive Signatures: Function signatures should ideally be self-descriptive. They should give as much indication as possible about what the function does. For example, a signature like
(IEnumerable<T>, (T → bool)) → IEnumerable<T>
suggests that the function operates on a sequence of items of generic type T and filters based on a predicate, resulting in another sequence of the same type. This is informative because it hints at the function being a filtering operation, such as theEnumerable.Where
in C# .Arrow Notation: In functional programming, the arrow notation is often used to describe function types concisely. The notation
(T1, T2) → TResult
would denote a function taking two parameters of types T1 and T2 and returning a value of type TResult. This is considered more readable, especially for higher-order functions .Higher-Order Functions: Functions that take other functions as parameters or return functions as their result are called higher-order functions. For example, a function signature like
(string, (IDbConnection → R)) → R
represents a function that takes a string and a function that operates on anIDbConnection
and returns a typeR
. The HOF itself returnsR
and is typical in scenarios where you want to abstract over behavior, such as database connections or transactional operations .Type Safety: Choosing appropriate types for function inputs helps prevent invalid inputs from ever being considered by the function. For example, instead of using generic types such as
int
for specific domains, you can create custom types that encapsulate validation rules and can only represent valid data. In this way, incorrect values are caught at compile time rather than at runtime .Custom Types for Domain Representation:
Patterns in Functional Programming
Patterns in functional programming are common solutions to recurring problems that adhere to the principles of functional programming. These solutions often involve the use of pure functions, immutable data, and higher-level abstractions to write code that is more predictable and easier to reason about.
Pure Functions: Functions that do not change or depend on any state outside their scope and do not produce any side effects. For any given input, they always return the same output. Example:
int Add(int x, int y) => x + y;
Recursion: Functions that call themselves in order to solve a problem by breaking it down into smaller sub-problems. Example: Efficiently calculating Fibonacci numbers using a recursive function.
Immutable Data Types: Data structures that, once created, cannot be changed. All operations that appear to modify data return a new instance instead. Example: String manipulation in many languages always returns a new string rather than changing the original.
Monads: Types that encapsulate a value and a computation pattern, providing a way to chain computations together. Monads include
Option
,Result
, andList
types, and they provide operations likebind
(also known asflatMap
) andreturn
(for wrapping values into the monad). Example:IEnumerable<T>
in C# is a monad that allows chaining with LINQ expressions.Pattern Matching: A way to de-structure and inspect data, especially useful with algebraic data types like unions or sum types. It replaces complex conditionals and is more declarative. Example: Using switch expressions in C# 8.0 and above to match against multiple potential shapes of data.
Function Composition: Creating new functions by combining two or more functions where the output of one is the input to another. Example:
.Select(...).Where(...)
in LINQ in C# composes functions to first transform, then filter a collection.Higher-order Functions: Functions that take other functions as parameters and/or return them as results. Example: The
Select
andWhere
methods in LINQ are HoFs; they take lambda expressions as arguments.Currying: Transforming a function that takes multiple arguments into a series of functions that each take a single argument. Example: Breaking down a function that calculates the area of a rectangle into a sequence of functions each requiring one parameter. ```Javascript const add = (x, y) => x + y; add(10, 2); // 12
const curriedAdd = (x) => { return (y) => { return x + y; }; };
currriedAdd(10)(2); // 12
9. **Lazy Evaluation**: An evaluation strategy that delays the computation of a value until it is actually needed. Example: The `IEnumerable<T>` execution in LINQ queries is lazy, executing the query only when you iterate over them.
10. **Memoization**: Caching the results of a function to avoid repeated computations for the same inputs, often implemented through higher-order functions that take a function and return a memoized version of it. Example: In C#, a memoization function might store results of expensive computations in a `Dictionary` and return the cached result on subsequent calls with the same arguments.
```Javascript
const memoize = (fn) => {
const cache = {};
return (...args) => {
const stringifiedArgs = JSON.stringify(args);
const result = cache[stringifiedArgs] = cache[stringifiedArgs] || fn(...args);
return result;
};
};
- Option Type/Maybe: A pattern to handle the absence of a value without resorting to null references, which often lead to
NullPointerException
s. Example: C# does not have a built-in option type, but you can create a customOption<T>
type that either holds a value of typeT
or represents the absence of a value. ```C# // Using functional C# libraries Option Divide(int x, int y) => y == 0 ? None : Some(x / y);
12. **Algebraic Data Types**: Types that are composed of other types, often used to create complex type hierarchies that are pattern matched. Example: In other functional programming languages like F#, you have discriminated unions that act as ADTs, allowing for expressive domain modeling that C# can mimic with inheritance and pattern matching.
These patterns are foundational in functional programming and guide the development of robust, concise, and maintainable code. They can drastically improve the way developers approach problems and systems architecture, leading to benefits like easier concurrency, better testability, and clearer code. (Buonanno, E. (n.d.). Functional Programming in C#: How to write better C# code.)
## Designing programs with function composition
Let's kick things off by revisiting function composition and its link to method chaining. For any programmer, function composition is like second nature – it's something you pick up along the way, maybe in school, and then just start using without giving it much thought. So, let's quickly run through the definition and get a solid grasp on it.
### Function Composition
Function composition involves combining multiple functions to create a new function. Given two functions, `f` and `g`, the composition of these functions, denoted as `h = f · g`, allows you to apply `g` to a value and then apply `f` to the result. This can be useful for creating complex workflows by breaking them down into smaller, reusable functions.
For example, in the context of a money transfer workflow, you can define functions for validating the transfer and booking the transfer. These functions can be composed to create a higher-level function that represents the entire workflow.
### Method Chaining
Method chaining is a syntax that allows you to chain multiple method invocations together using the dot operator. This provides a more readable way of achieving function composition in languages like C#. By chaining methods, you can create a sequence of operations that represent a workflow.
For example, say you want to get an email address for someone working at Manning. You can have a function calculate the local part (identifying the person) and another append the domain:
```C#
Func<Person, string> emailFor = p => AppendDomain(AbbreviateName(p));
var opt = Some(new Person("John", "Doe"));
var a = opt.Map(emailFor);
varb = opt.Map(AbreveateName)
.Map(AppendDomain);
For instance, in the example of determining a person's email address, you can chain methods like AbbreviateName()
and AppendDomain()
to obtain the desired result. This improves readability, especially as the complexity of the workflow increases.
Benefits of Workflows with Function Composition and Method Chaining Defining workflows with function composition and method chaining offers several benefits. It allows for modular and reusable code, as individual functions can be composed and reused in different workflows. It also improves readability and maintainability, as the sequence of operations in a workflow is expressed in a clear and concise manner.
By leveraging these techniques, you can design programs that are expressive, efficient, and easy to understand. Whether you're working with LINQ in C# or other functional programming paradigms, function composition and method chaining are valuable tools for designing workflows.
Thinking in terms of data flow
The concept of function composition allows you to construct entire programs by chaining functions together. In this approach, each function processes its input, and the resulting output serves as the input for the subsequent function in the chain. This way of structuring your program encourages viewing it through the lens of data flow. Essentially, your program becomes a sequence of functions, and data smoothly traverses through the program, moving from one function to the next.
In the context of Figure 5.1, it illustrates a linear flow, which is the most straightforward and practical type of data flow. This linear progression showcases the simplicity and utility of organizing functions in a sequential manner, where the output of one function seamlessly feeds into the input of the next.
Conclusion
Functional Programming presents us a different way of thinking about programming, that brings us different solutions to the most common problems in the Object-Oriented Paradigm. It is a declarative paradigm that focuses on what to solve rather than how to solve it. Having this knowledge of different paradigms will help you to become a better programmer, and even imitate different patterns from other paradigms in your code to solve specific problems. Programming paradigms are not mutually exclusive, and often evolve with language capabilities, which lead the software industry to a more flexible and powerful way of solving problems.
References
- Functional Programming in C#. (n.d.). Manning Publications. https://www.manning.com/books/functional-programming-in-c-sharp\
- Widman, J. (2022). Learning Functional Programming. (n.p.): O'Reilly Media.
Subscribe to my newsletter
Read articles from Lambda directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
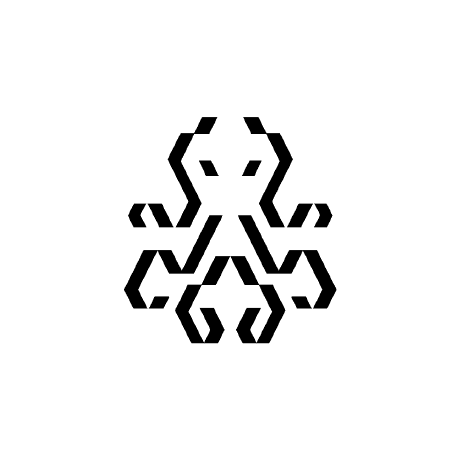
Lambda
Lambda
I am Computer Engineer with experience in web development, microservices and embedded programming as well.