Find the maximum and minimum element in an array - 450DSA Cracker Solution
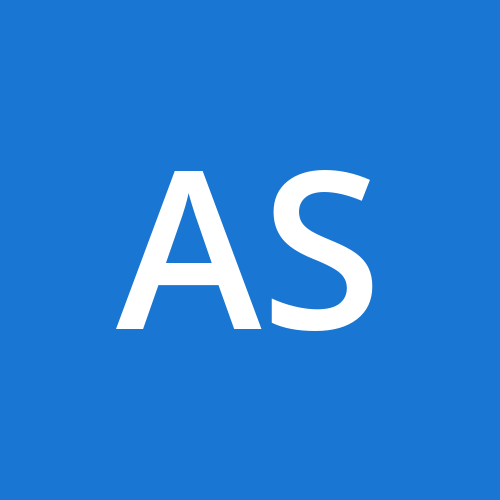
1 min read
Table of contents
Find minimum and maximum element in an array
Given an array A of size N of integers. Your task is to find the minimum and maximum elements in the array.
Example 1:
Input:
N = 6
A[] = {3, 2, 1, 56, 10000, 167}
Output: 1 10000
Explanation: minimum and maximum elements of array are 1 and 10000.
Example 2:
Input:
N = 5
A[] = {1, 345, 234, 21, 56789}
Output: 1 56789
Explanation: minimum and maximum element of array are 1 and 56789.
Your Task:
You don't need to read input or print anything. Your task is to complete the function getMinMax() which takes the array A[] and its size N as inputs and returns the minimum and maximum element of the array.
Expected Time Complexity: O(N)
Expected Auxiliary Space: O(1)
Code
Solution 1 :
pair<long long , long long > obj;
obj.first = INT_MAX;
obj.second = INT_MIN;
for(int i=0;i<n;i++)
{
if(a[i] < obj.first)
obj.first = a[i];
if(a[i] > obj.second)
obj.second = a[i];
}
return obj;
Solution 2:
long long min = INT_MAX;
long long max = INT_MIN;
for(int i=0;i<n;i++)
{
if(a[i] > max)
max = a[i];
if(a[i] < min)
min = a[i];
}
return {min,max};
Solution 3:
sort(a, a+n);
return {a[0],a[n-1]};
0
Subscribe to my newsletter
Read articles from Akshima Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
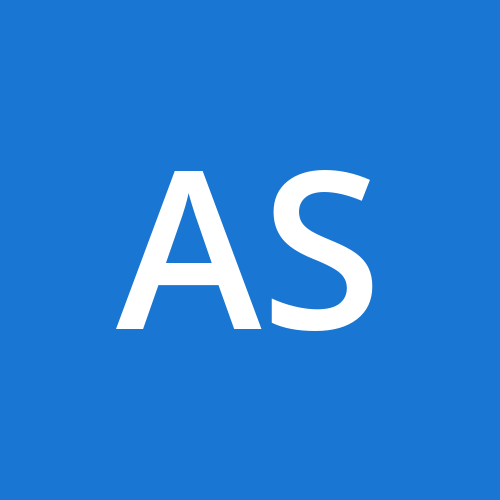