The Ultimate Guide to Mastering Python for AI/ML: Key Topics You Can't Afford to Miss
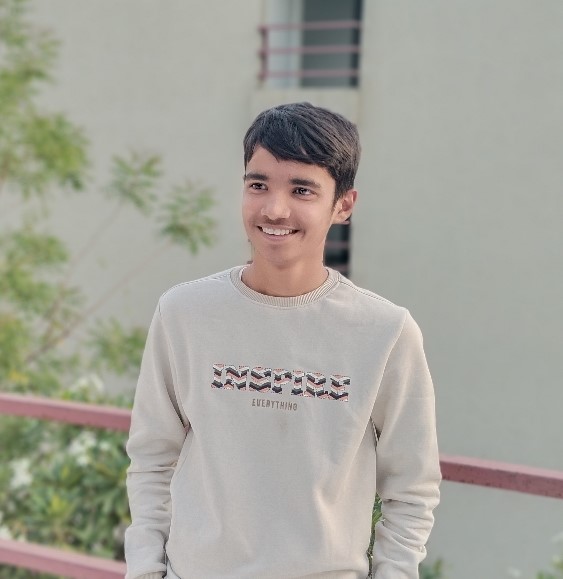
Introduction:
Welcome to the world of Python Programming! Python is a versatile and powerful programming language that has gained immense popularity in the field of AI/ML due to simplicity, readability, and vast array of libraries and frameworks. In this article, we will dive into the key topics that you need to master in Python to unlock your potential as an AI/ML expert.
Understanding Python Syntax and Semantics:
Understanding how to declare variables and work with different data types like integers, floats, strings, lists, and dictionaries is crucial. You must familiarize yourself with variable assignment, type conversion, and basic operations.
# Example of list manipulation
numbers = [1, 2, 3, 4, 5]
squares = [num ** 2 for num in numbers]
print(squares) # Output: [1, 4, 9, 16, 25]
Learn about conditional statements like if, else, and elif, along with loops such as for and while. Python's modular structure empowers developers to break down complex tasks into manageable functions and reuse code effectively. Gain proficiency in defining and utilizing functions, as well as importing modules/libraries to leverage pre-built AI/ML functionalities.
# Example of function definition
def greet(name):
print("Hello, " + name + "!")
greet("Alice") # Output: Hello, Alice!
Advanced Python Concepts:
Object Oriented Programming (OOP) principles are crucial in AI/ML for organizing code into modular components, encapsulating data and methods, enabling inheritance for code reuse, facilitating polymorphism for flexibility, and promoting abstraction for high-level design. Iterators and generators are indispensable in AI/ML for efficiently processing large datasets and performing complex computations. Iterators enable sequential access to data, facilitating tasks like iterating through training examples or evaluating models. Generators offer a memory-efficient approach to lazily generate data on-the-fly, beneficial for dealing with massive datasets or infinite streams of data. For instance, iterators can iterate over batches of training data, while generators can dynamically generate augmented data or handle asynchronous processing tasks.
Libraries and Frameworks:
Python's strength in the AI and Data Science domain lies in its rich ecosystem of libraries and frameworks. Familiarizing yourself with these essential libraries will empower you to build powerful Al/ML solutions. Let's explore them:
Data Manipulation using NumPy
NumPy is a fundamental library for scientific computing with Python. It provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on them. NumPy's efficiency and convenience make it an indispensable tool for Al/ML practitioners.
import numpy as np
# Creating a NumPy array
arr = np.array([1, 2, 3, 4, 5])
print(arr) # Output: [1 2 3 4 5]
# Performing arithmetic operations
result = arr * 2
print(result) # Output: [ 2 4 6 8 10]
Data Handling with Pandas
Pandas is a versatile data manipulation and analysis library. It offers data structures like DataFrames, which enable efficient handling of structured data. Pandas simplifies common data pre-processing tasks such as cleaning, filtering, and transforming data, making it an essential library for data science.
import pandas as pd
# Creating a DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35]}
df = pd.DataFrame(data)
print(df)
Data Visualization with Matplotlib
Data visualization plays a vital role in understanding patterns and trends in AI/ML. Matplotlib is a powerful library for creating static, animated, and interactive visualizations. With its intuitive interface, you can effortlessly generate plots, histograms, scatter plots, and more to gain insights from your data.
import matplotlib.pyplot as plt
# Plotting a simple line graph
x = [1, 2, 3, 4, 5]
y = [2, 4, 6, 8, 10]
plt.plot(x, y)
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.title('Simple Line Graph')
plt.show()
To sum up, mastering Python is a critical step toward becoming proficient in AI/ML development. By mastering the essential Python topics discussed in this guide and applying them to real-world projects, you'll be well-equipped to tackle complex AI/ML challenges. Keep learning, experimenting, and refining your skills to unlock new possibilities in the field of artificial intelligence and machine learning.
Subscribe to my newsletter
Read articles from Jay Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
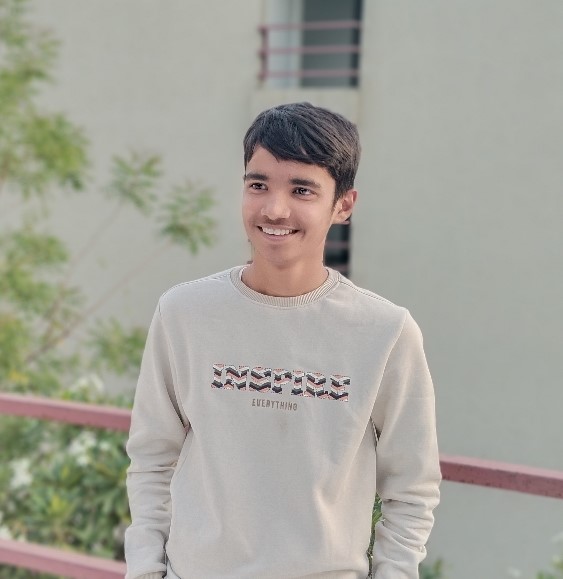