Automating Gherkin Scenario Generation with OpenAI's API
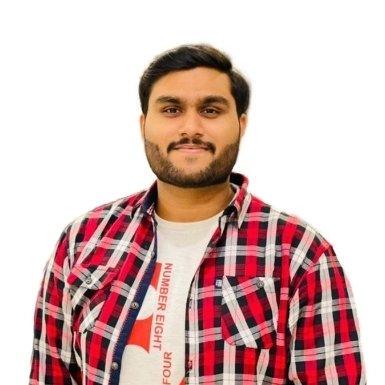
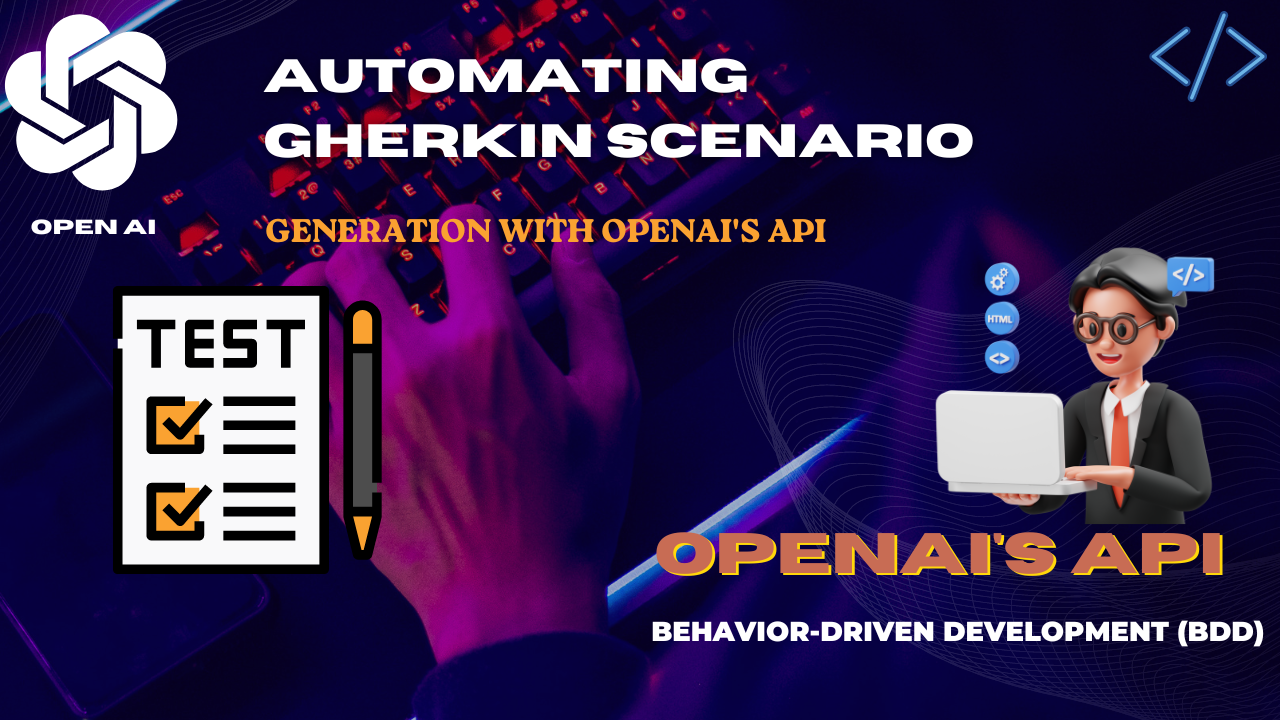
In today's fast-paced software development world, efficiency and speed are critical. Test case creation is one area ripe for optimisation, particularly for Behavior-Driven Development (BDD), where Gherkin scenarios play a crucial role. Thanks to AI and machine learning advancements, we can leverage OpenAI's powerful language models to automate this process. In this blog post, we'll create a simple Python script that transforms your test cases into Gherkin scenarios using OpenAI's API.
Setting Up the Environment: Before diving into the code, please ensure your environment is ready. You'll need Python and an API key from OpenAI installed on your system. We recommend using virtual environments to manage your Python packages.
python -m venv venv
source venv/bin/activate # On Windows, use `venv\Scripts\activate`
pip install openai python-dotenv
Storing the API Key securely: Security is paramount. Therefore, we'll use environment variables to hold the OpenAI API key. Create a.env
file in your project's root directory and add your API key like this:
OPENAI_API_KEY=your_api_key_here
Please don't commit this file to your version control system. Add .env
to your .gitignore
file.
Project Structure: Our project has a simple structure:
GherkinScenario/
│
├── code/
│ ├── main.py
│ └── ...
│
├── data.txt
├── output.txt
├── .env
└── README.md
data.txt
contains the test case andoutput.txt
will be the generated Gherkin scenario.
The Python Script: Our scriptmain.py
performs a few key tasks:
Reads the test case from
data.txt
.Sends the test case to OpenAI's API.
Writes the Gherkin scenario to
output.txt
.
Here's a snapshot of the core function:
def query_openai_chat_api(prompt):
response = openai.ChatCompletion.create(
model="gpt-3.5-turbo",
messages=[{"role": "user", "content": prompt}],
max_tokens=150
)
return response
Handling the API Response: We handle the response from OpenAI's API by parsing the JSON and extracting the content. We then write it downoutput.txt
, ensuring that our Gherkin scenarios are stored persistently.
Running the Script: Execute the script with the following command:
python code/main.py
Upon completion, output.txt
will contain the Gherkin scenario generated by OpenAI's model.
Conclusion: Automating Gherkin scenario generation can save precious time and reduce errors in test case creation. By leveraging OpenAI's API, we've shown that it's possible and straightforward. As AI models continue to improve, we anticipate even more advanced capabilities in automating various software development and testing facets.
Next Steps: You'll be able to experiment with different test cases and consider integrating this script into your CI/CD pipeline for even more significant efficiency gains. Stay tuned for future posts to explore fine-tuning AI models for domain-specific scenario generation.
Remember, AI is a tool to augment our capabilities, and with great power comes great responsibility. Use it wisely to enhance your development workflow!
If you want the source code, you can contact me through email. Thanks 👨💻
Subscribe to my newsletter
Read articles from Muhammad Fazeel Arif directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
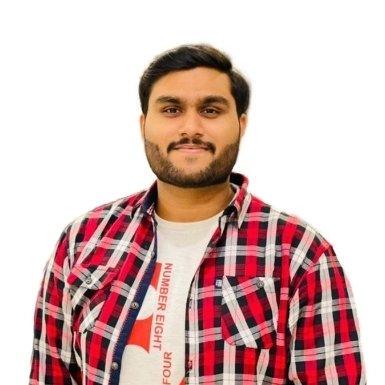
Muhammad Fazeel Arif
Muhammad Fazeel Arif
As a Master's degree candidate in artificial intelligence at FAU Erlangen-Nürnberg, I am passionate about using AI technology to solve complex problems.