Attributes of Text Widget

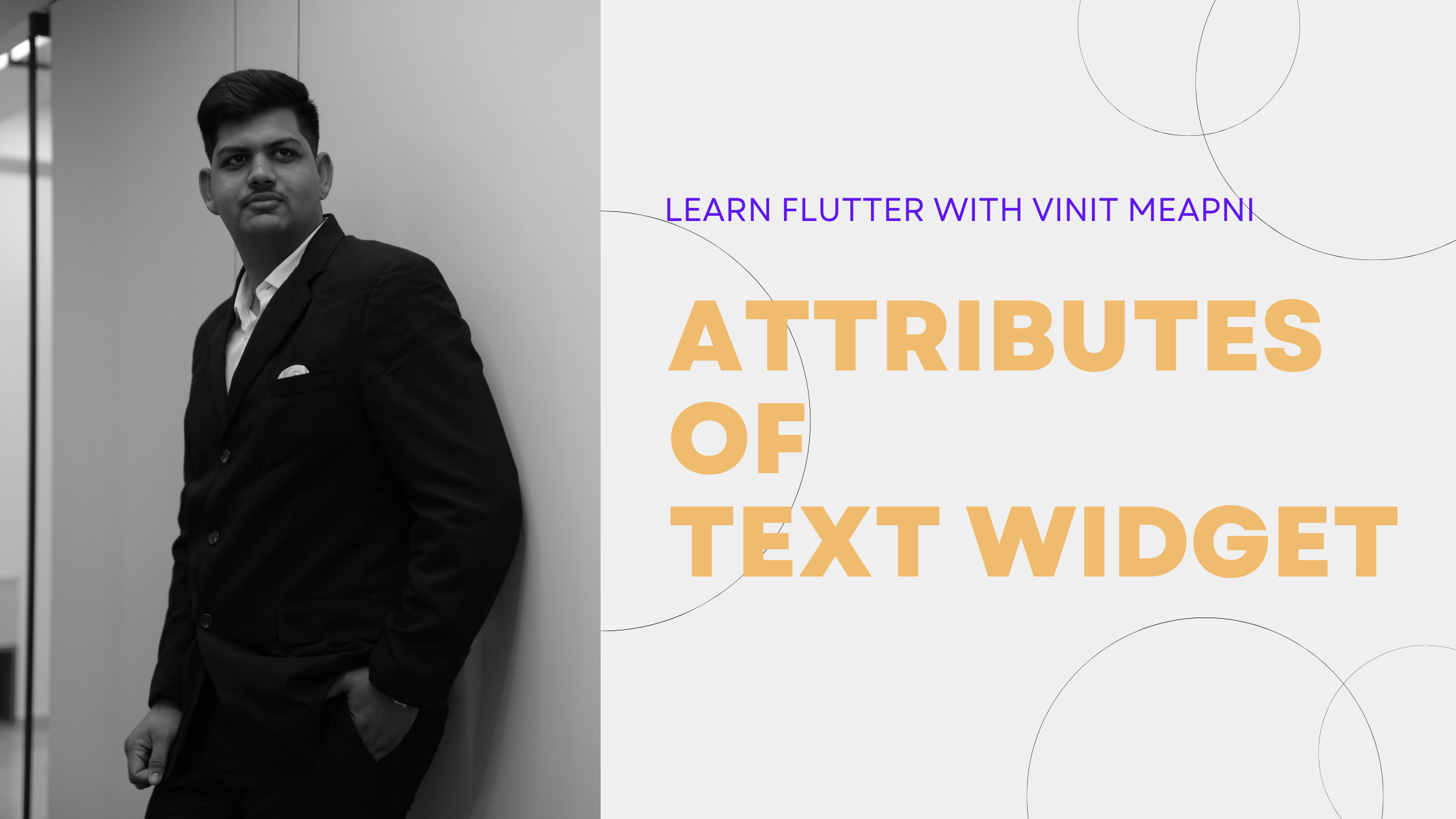
Flutter's Text widget is a fundamental component for displaying text in your app. It allows you to customize the appearance of the text, such as the font, size, color, and more. Here are some key attributes of the Text widget in Flutter:
data (String):
- This attribute represents the actual text content that will be displayed.
Text(
'Hello, Flutter!',
)
style (TextStyle):
- The style attribute is used to define the text's appearance, including font size, color, weight, and more.
Text(
'Stylish Text',
style: TextStyle(
fontSize: 18.0,
color: Colors.blue,
fontWeight: FontWeight.bold,
),
)
textAlign (TextAlign):
- textAlign is used to specify the horizontal alignment of the text within its container.
Text(
'Aligned Center',
textAlign: TextAlign.center,
)
textDirection (TextDirection):
- Defines the reading direction of the text. It can be either TextDirection.ltr (left-to-right) or TextDirection.rtl (right-to-left).
Text(
'Right-to-Left Text',
textDirection: TextDirection.rtl,
)
overflow (TextOverflow):
- Determines how the text should behave if it overflows its container. Options include ellipsis, clip, or fade.
Text(
'This is a long text that might overflow the container.',
overflow: TextOverflow.ellipsis,
)
maxLines (int):
- Specifies the maximum number of lines to display. Useful when dealing with multiline text.
Text(
'Multiline Text Example',
maxLines: 2,
)
softWrap (bool):
- Indicates whether the text should wrap to the next line if it exceeds the width of its container.
Text(
'This is a very long sentence that may wrap to the next line.',
softWrap: true,
)
textScaleFactor (double):
- Allows you to scale the text size. A value greater than 1.0 increases the text size, and a value less than 1.0 decreases it.
Text(
'Scaled Text',
textScaleFactor: 1.5,
)
These are some of the essential attributes of the Text widget in Flutter. Combining these attributes allows you to create a wide variety of text styles and layouts in your Flutter applications.
Here is a comprehensive list of attributes for the Text widget in Flutter, along with a sample example showcasing each attribute:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Text Widget Attributes Example'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
'1. Data Attribute',
style: TextStyle(fontSize: 20.0),
),
Text(
'2. Style Attribute',
style: TextStyle(
fontSize: 18.0,
color: Colors.blue,
fontWeight: FontWeight.bold,
),
),
Text(
'3. TextAlign Attribute',
textAlign: TextAlign.center,
),
Text(
'4. TextDirection Attribute',
textDirection: TextDirection.rtl,
),
Text(
'5. Overflow Attribute',
overflow: TextOverflow.ellipsis,
),
Text(
'6. MaxLines Attribute',
maxLines: 2,
),
Text(
'7. SoftWrap Attribute',
softWrap: true,
),
Text(
'8. TextScaleFactor Attribute',
textScaleFactor: 1.5,
),
],
),
),
),
);
}
}
Subscribe to my newsletter
Read articles from Vinit Mepani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vinit Mepani
Vinit Mepani
"Hello World, I'm Vinit Mepani, a coding virtuoso driven by passion, fueled by curiosity, and always poised to conquer challenges. Picture me as a digital explorer, navigating through the vast realms of code, forever in pursuit of innovation. In the enchanting kingdom of algorithms and syntax, I wield my keyboard as a magical wand, casting spells of logic and crafting solutions to digital enigmas. With each line of code, I embark on an odyssey of learning, embracing the ever-evolving landscape of technology. Eager to decode the secrets of the programming universe, I see challenges not as obstacles but as thrilling quests, opportunities to push boundaries and uncover new dimensions in the realm of possibilities. In this symphony of zeros and ones, I am Vinit Mepani, a coder by passion, an adventurer in the digital wilderness, and a seeker of knowledge in the enchanting world of code. Join me on this quest, and let's create digital wonders together!"