Flutter : TextSpan Widget , RichText Widget ,Text.rich Widget

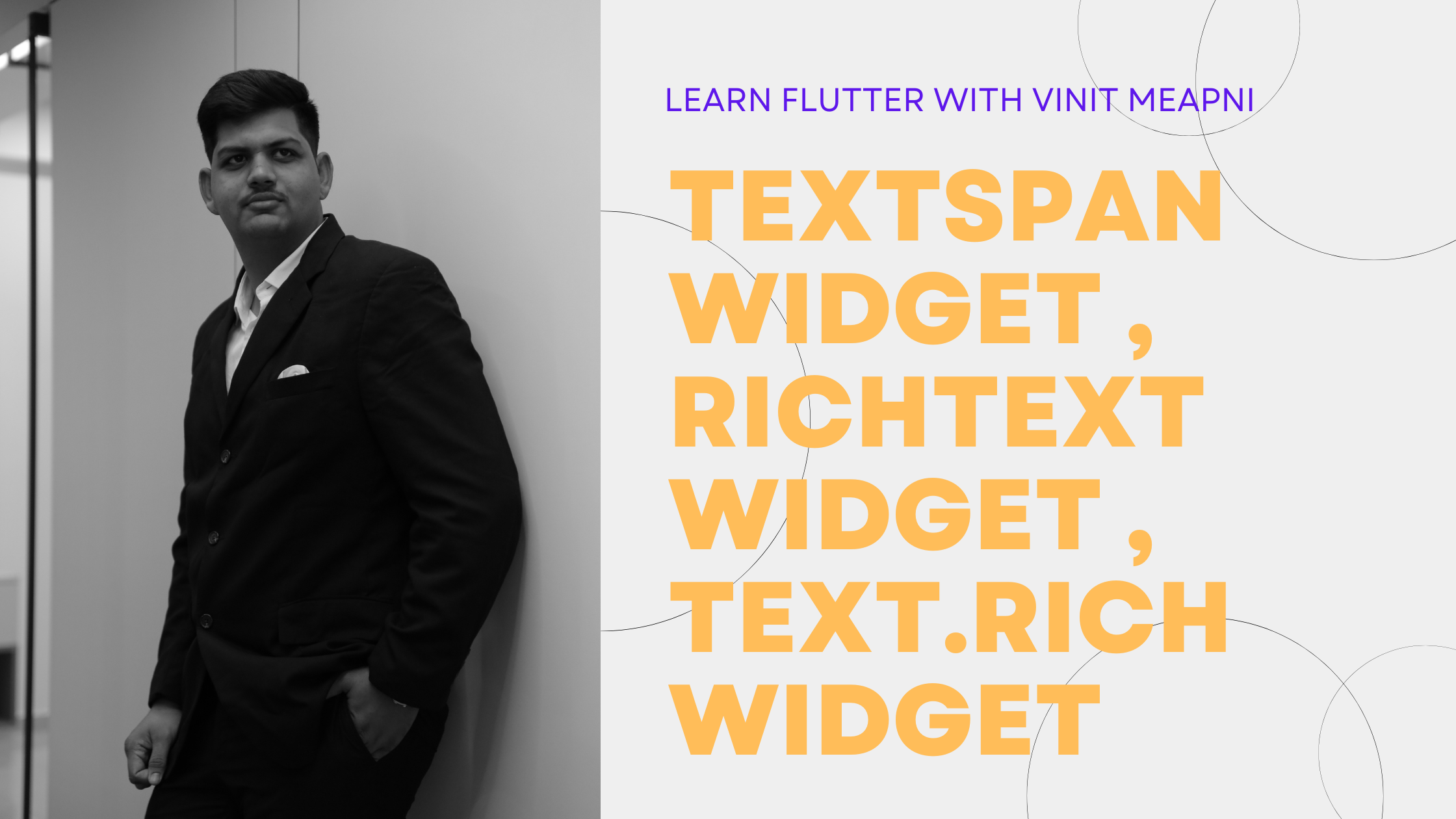
In Flutter, TextSpan, RichText, and Text.rich are components that allow you to create rich and stylized text with different styles, spans, and formatting within a single text widget. Let's explore each of them:
1. TextSpan:
TextSpan is a part of the RichText widget and represents a portion of styled text. It is commonly used to apply different styles to different parts of a text string.
Example:
Text.rich(
TextSpan(
text: 'This is a ',
style: TextStyle(color: Colors.black),
children: <TextSpan>[
TextSpan(
text: 'rich',
style: TextStyle(fontWeight: FontWeight.bold, color: Colors.blue),
),
TextSpan(
text: ' text example.',
style: TextStyle(fontStyle: FontStyle.italic, color: Colors.red),
),
],
),
);
2. RichText:
RichText is a widget that displays text that uses multiple different styles. It is built with a list of TextSpan widgets, each of which can have its own style.
Example:
RichText(
text: TextSpan(
text: 'This is a ',
style: DefaultTextStyle.of(context).style,
children: <TextSpan>[
TextSpan(
text: 'rich',
style: TextStyle(fontWeight: FontWeight.bold, color: Colors.blue),
),
TextSpan(
text: ' text example.',
style: TextStyle(fontStyle: FontStyle.italic, color: Colors.red),
),
],
),
);
3. Text.rich:
Text.rich is a shorthand for creating a RichText widget with a single TextSpan. It's convenient when you only need one TextSpan in your rich text.
Example:
Text.rich(
TextSpan(
text: 'This is a ',
style: DefaultTextStyle.of(context).style,
children: <TextSpan>[
TextSpan(
text: 'rich',
style: TextStyle(fontWeight: FontWeight.bold, color: Colors.blue),
),
TextSpan(
text: ' text example.',
style: TextStyle(fontStyle: FontStyle.italic, color: Colors.red),
),
],
),
);
In these examples, we create rich text with different styles using TextSpan, RichText, and Text.rich. The children property of TextSpan allows you to add multiple spans with different styles, creating a visually rich and formatted text.
Here's a merged example that demonstrates the use of TextSpan, RichText, and Text.rich in a Flutter application:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Rich Text Example'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
// Example using TextSpan
Text.rich(
TextSpan(
text: '1. TextSpan Example: ',
style: TextStyle(fontSize: 16.0, color: Colors.black),
children: <TextSpan>[
TextSpan(
text: 'Rich',
style: TextStyle(
fontWeight: FontWeight.bold,
color: Colors.blue,
),
),
TextSpan(
text: ' Text with different styles.',
style: TextStyle(
fontStyle: FontStyle.italic,
color: Colors.red,
),
),
],
),
),
SizedBox(height: 20.0),
// Example using RichText
RichText(
text: TextSpan(
text: '2. RichText Example: ',
style: DefaultTextStyle.of(context).style,
children: <TextSpan>[
TextSpan(
text: 'Rich',
style: TextStyle(
fontWeight: FontWeight.bold,
color: Colors.blue,
),
),
TextSpan(
text: ' Text with different styles.',
style: TextStyle(
fontStyle: FontStyle.italic,
color: Colors.red,
),
),
],
),
),
SizedBox(height: 20.0),
// Example using Text.rich
Text.rich(
TextSpan(
text: '3. Text.rich Example: ',
style: DefaultTextStyle.of(context).style,
children: <TextSpan>[
TextSpan(
text: 'Rich',
style: TextStyle(
fontWeight: FontWeight.bold,
color: Colors.blue,
),
),
TextSpan(
text: ' Text with different styles.',
style: TextStyle(
fontStyle: FontStyle.italic,
color: Colors.red,
),
),
],
),
),
],
),
),
),
);
}
}
This example combines the use of TextSpan, RichText, and Text.rich. Each section demonstrates how to create rich text with different styles, using a combination of bold, italic, and colored text. Feel free to copy and paste this code into a new Flutter project to see the rich text in action.
Subscribe to my newsletter
Read articles from Vinit Mepani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vinit Mepani
Vinit Mepani
"Hello World, I'm Vinit Mepani, a coding virtuoso driven by passion, fueled by curiosity, and always poised to conquer challenges. Picture me as a digital explorer, navigating through the vast realms of code, forever in pursuit of innovation. In the enchanting kingdom of algorithms and syntax, I wield my keyboard as a magical wand, casting spells of logic and crafting solutions to digital enigmas. With each line of code, I embark on an odyssey of learning, embracing the ever-evolving landscape of technology. Eager to decode the secrets of the programming universe, I see challenges not as obstacles but as thrilling quests, opportunities to push boundaries and uncover new dimensions in the realm of possibilities. In this symphony of zeros and ones, I am Vinit Mepani, a coder by passion, an adventurer in the digital wilderness, and a seeker of knowledge in the enchanting world of code. Join me on this quest, and let's create digital wonders together!"