Day 10 - Advance Git & GitHub for DevOps Engineers: Part 1
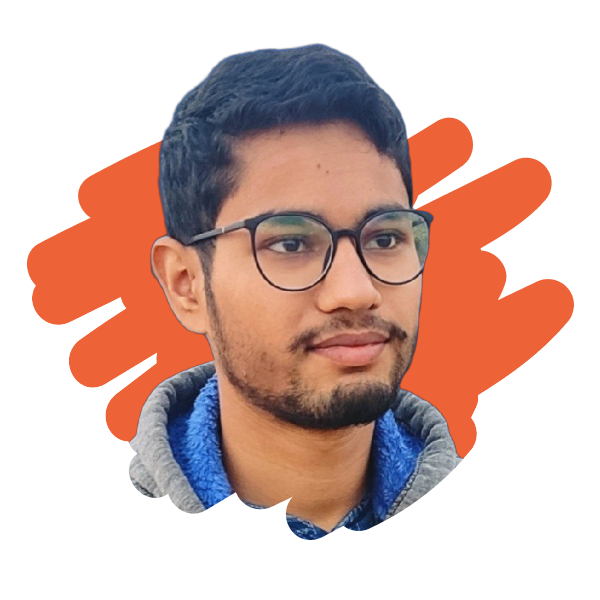

Git Branching
Git branching is a feature in the version control system Git that allows developers to create separate lines of development called branches. Each branch represents an independent line of work that can include new features, bug fixes, or experiments without affecting the main codebase. Branching enables developers to work on different tasks simultaneously, collaborate with others, and manage code changes effectively.
Here are some key concepts related to Git branching:
Main Branch (often called
master
ormain
): This is the default branch in a Git repository. It usually represents the stable version of the project.Branch: A separate line of development that diverges from the main branch. Developers can create branches to work on new features or bug fixes without altering the main codebase.
Branching Off: Creating a new branch from an existing one. This allows developers to start working on a new feature or bug fix independently.
Merge: Combining changes from one branch into another. When a feature or bug fix is complete and tested, it can be merged back into the main branch to incorporate the changes into the main codebase.
Pull Request (PR): In Git-based collaboration workflows, a pull request is a way to propose changes to a repository. It allows other developers to review the code before merging it into the main branch.
Branch Management: Managing branches effectively, including creating, renaming, deleting, and merging branches as needed. Git provides commands and tools for managing branches efficiently.
Using branches in Git facilitates collaboration among developers, allows for experimentation without affecting the main codebase, and helps in organizing and managing code changes effectively throughout the development process.
Git Revert and Reset
Git revert and git reset are both commands used in Git to manage changes in a repository's commit history, but they serve different purposes and have different effects.
Git Revert:
The
git revert
command is used to create a new commit that undoes the changes introduced by a specified commit or commits.When you revert a commit using
git revert
, Git creates a new commit with the inverse changes of the specified commit(s). This means that the commit history remains intact, and the changes are undone in a new commit.Reverting is useful when you want to undo specific changes while preserving the commit history. It's a safe way to undo changes because it doesn't alter the existing commit history.
Example:
git revert <commit>
Git Reset:
The
git reset
command is used to reset the current state of the repository to a specified state. It can be used to move the HEAD (the currently checked out branch) and optionally the index (staging area) and the working directory to a specified state.git reset
can be used with different options like--soft
,--mixed
, or--hard
, which determine how much of the state is reset.When using
git reset --soft
, the HEAD is moved to the specified commit, but the index and working directory are not changed. This means that the changes are staged for commit.With
git reset --mixed
(which is the default if no option is provided), the HEAD and index are moved to the specified commit, but the working directory is not changed. This means that the changes are unstaged.Using
git reset --hard
moves the HEAD, index, and working directory to the specified commit, discarding all changes made after that commit.
Example:
git reset --soft <commit>
git reset --mixed <commit>
git reset --hard <commit>
In summary, git revert
is used to create a new commit that undoes the changes of a specific commit while preserving the commit history, whereas git reset
is used to move the HEAD and optionally the index and working directory to a specified state, potentially discarding changes.
Git Rebase and Merge
Git rebase
and git merge
are both used in Git to integrate changes from one branch into another, but they do so in different ways and have different implications for the repository's history.
Git Rebase:
The
git rebase
command is used to incorporate changes from one branch onto another branch by reapplying each commit on the branch being rebased onto the tip of the branch it's being rebased onto.When you rebase a branch onto another branch, Git essentially takes the changes introduced by each commit on the rebased branch and "replays" them onto the target branch. This results in a linear history, as if the changes were made directly on top of the target branch.
Rebase is useful for maintaining a clean and linear history, as it avoids unnecessary merge commits and can make it easier to understand the chronological order of changes.
Example:
git checkout feature-branch
git rebase main
Git Merge:
The
git merge
command is used to integrate changes from one branch into another by combining the commit histories of both branches.When you merge one branch into another, Git creates a new commit that has two parent commits: one from the current branch and one from the branch being merged. This creates a merge commit, which represents the combination of the changes from both branches.
Merge is useful for integrating changes from one branch into another while preserving the commit history of both branches. It's especially suitable for feature branches that are intended to be merged back into a main branch.
Example:
git checkout main
git merge feature-branch
In summary, git rebase
is used to integrate changes from one branch into another by reapplying commits on top of the target branch, resulting in a linear history, while git merge
is used to integrate changes by combining the commit histories of both branches, resulting in a merge commit that preserves the history of both branches. Each approach has its advantages and use cases, and the choice between them depends on the specific requirements of the project and the desired repository history.
Task 1:
- Create a new branch from master and switch to it:
git checkout -b dev
- Add a text file version01.txt with the given content:
echo "This is first feature of our application" > Devops/Git/version01.txt
- Commit the changes:
git add Devops/Git/version01.txt
git commit -m "Added new feature"
- Push the changes to remote repository for review:
git push origin dev
- Add new content to version01.txt and commit:
echo "This is the bug fix in development branch" >> Devops/Git/version01.txt
git add Devops/Git/version01.txt
git commit -m "Added feature2 in development branch"
echo "This is gadbad code" >> Devops/Git/version01.txt
git add Devops/Git/version01.txt
git commit -m "Added feature3 in development branch"
echo "This feature will gadbad everything from now." >> Devops/Git/version01.txt
git add Devops/Git/version01.txt
git commit -m "Added feature4 in development branch"
- Restore the file to a previous version: If you want to completely remove the changes made in the last commits, you can use:
git reset --hard HEAD~3
This will remove the last 3 commits and restore the file to the state before those commits.
Task 2:
Demonstrate Branches:
Create Branches:
- Create two branches, let's call them
feature1
andfeature2
.
- Create two branches, let's call them
git checkout -b feature1
git checkout -b feature2
Make Changes:
- Make some changes in each branch.
# Make changes in feature1 branch
# Make changes in feature2 branch
Commit Changes:
- Commit changes in each branch.
git add .
git commit -m "Added feature1"
Merge Branches:
- Merge the branches into the main branch (master/dev).
git checkout master
git merge feature1
git merge feature2
Git Rebase:
Create a new branch and make changes:
- Create a new branch from master and make some changes.
git checkout -b feature3
# Make changes in feature3 branch
Commit Changes:
- Commit changes in the feature3 branch.
git add .
git commit -m "Added feature3"
Rebase onto master:
- Rebase the feature3 branch onto master.
git checkout master
git pull origin master
git checkout feature3
git rebase master
Resolve conflicts (if any):
- If there are any conflicts, resolve them.
# Resolve conflicts in feature3 branch
git add .
git rebase --continue
Finish rebase:
- Finish the rebase process.
git checkout master
git merge feature3
This process demonstrates branching, making changes, merging, and using git rebase. Remember to replace master
with dev
if you're working with a dev
branch.
Thank you for taking the time to read this blog. I hope you found valuable insights! If you enjoyed the content, please consider giving it a like, sharing it, and following for more insightful posts in the future. Your support means a lot! Looking forward to sharing more knowledge with you! ๐
๐ Let's bridge the distance and connect ๐๐คPriyam Kundu๐
Subscribe to my newsletter
Read articles from Priyam Kundu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
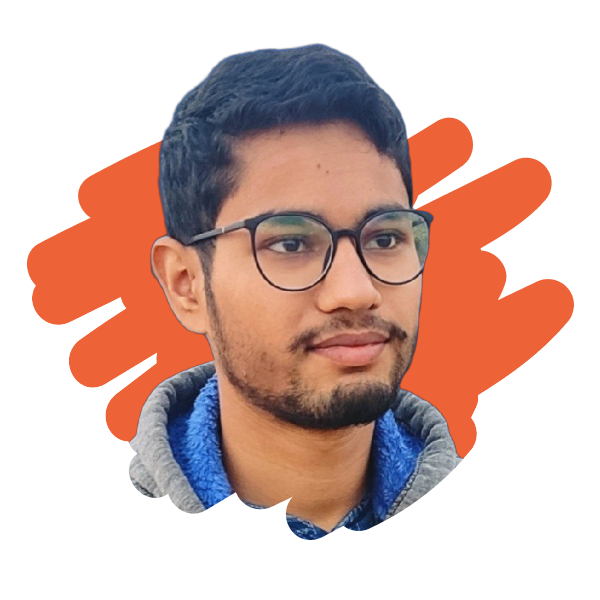
Priyam Kundu
Priyam Kundu
Hi there ๐ I'm Priyam Kundu, a final year undergrad from Kolkata and an aspiring DevOps & Cloud โ๏ธ professional. As an aspiring DevOps engineer with a keen interest in Cloud โ๏ธ and DevOps, I'm here to grow and expand my knowledge in this field๐. Looking forward to sharing my experiences and learning from all of you passionate professionals.