Day 14 Task: Understanding Python Data Types and Data Structures for DevOps
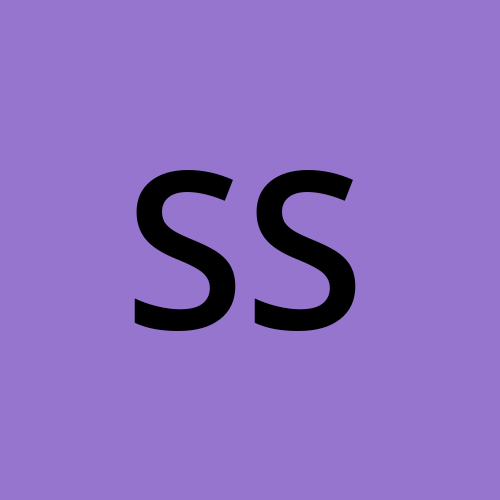
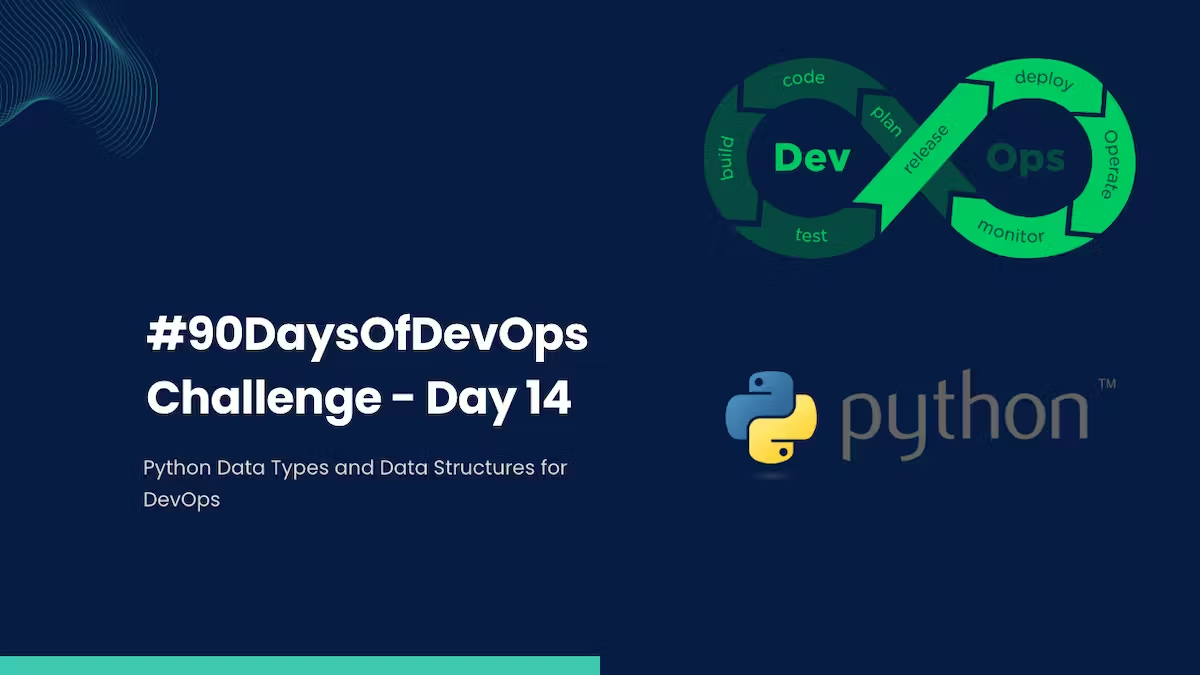
This is #90DaysofDevops challenge under the guidance of Shubham Londhe sir.
Introduction
Welcome to Day 14 of the 90DaysOfDevOps challenge! Today, we embark on a journey to explore Python's data types and data structures, fundamental concepts crucial for every DevOps engineer. Let's dive in and unravel the mysteries of Python's versatile tools.
Data Types in Python
In Python, data types categorize and define operations for data items. They're like the building blocks of our programs, enabling us to work with different kinds of data efficiently.
Common Python Data Types:
Numeric: Integers, complex numbers, and floats.
Sequential: Strings, lists, and tuples.
Boolean: Represents True or False values.
Set: Represents a collection of unique elements.
Dictionary: Key-value pairs for efficient data storage.
To determine the data type of a variable, use the type()
function, passing the variable as an argument.
your_variable = 100
print(type(your_variable)) # Output: <class 'int'>
Output:
Python Data Structures
Data structures help organize and manage our data effectively. Python offers various built-in structures to suit different needs:
Lists
Lists are ordered collections of data, akin to arrays in other languages. They offer flexibility, allowing elements of different types within the same list.
Tuple
Tuples are similar to lists but immutable, meaning their elements cannot be changed once created. They can contain elements of various types, just like lists.
Dictionary
Dictionaries store data as key-value pairs, resembling hash tables in other languages. They offer fast access to values based on unique keys, optimizing data retrieval.
Hands-On Tasks
1. Difference between List, Tuple, and Set
List:
Ordered collection of items.
Mutable (can be modified after creation).
Allows duplicate elements.
Enclosed in square brackets
[]
.Commonly used for sequences of elements where order matters and flexibility is required.
List Example:
# Creating a list my_list = [1, 2, 3, 4, 5, 5] # Accessing elements print("List:", my_list) # Output: List: [1, 2, 3, 4, 5, 5] # Modifying elements my_list[0] = 10 print("Modified List:", my_list) # Output: Modified List: [10, 2, 3, 4, 5, 5]
Output:
Tuple:
Ordered collection of items.
Immutable (cannot be modified after creation).
Allows duplicate elements.
Enclosed in parentheses
()
.Used when the sequence of elements should not be changed.
Tuple Example:
# Creating a tuple my_tuple = (1, 2, 3, 4, 5, 5) # Accessing elements print("Tuple:", my_tuple) # Output: Tuple: (1, 2, 3, 4, 5, 5) # Immutable - Cannot modify elements (will raise an error) # my_tuple[0] = 10
Output:
Set:
Unordered collection of unique items.
Mutable (can be modified after creation).
Does not allow duplicate elements.
Enclosed in curly braces
{}
.Ideal for mathematical operations like unions, intersections, and differences.
Set Example:
# Creating a set my_set = {1, 2, 3, 4, 5, 5} # Accessing elements (order may vary) print("Set:", my_set) # Output: Set: {1, 2, 3, 4, 5} # Adding elements my_set.add(6) print("Modified Set:", my_set) # Output: Modified Set: {1, 2, 3, 4, 5, 6}
Output:
2. Use of Dictionary Methods
fav_tools = {
1: "Linux",
2: "Git",
3: "Docker",
4: "Kubernetes",
5: "Terraform",
6: "Ansible",
7: "Chef"
}
# Access favorite tool using key
print(fav_tools[3]) # Output: Docker
Output:
3. Manipulating Lists
cloud_providers = ["AWS", "GCP", "Azure"]
# Add Digital Ocean to the list
cloud_providers.append("Digital Ocean")
# Sort the list alphabetically
cloud_providers.sort()
print(cloud_providers) # Output: ['AWS', 'Azure', 'Digital Ocean', 'GCP']
Output:
Incorporate these Python concepts into your DevOps toolkit for enhanced automation and management. Stay tuned for more exciting challenges ahead!
Thanks for taking the time to read! ๐
Subscribe to my newsletter
Read articles from Supriya Surkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
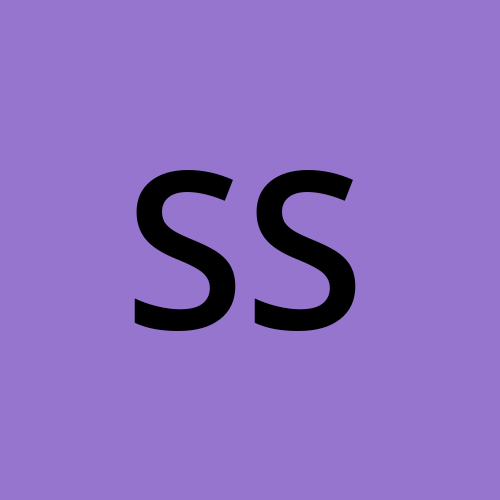