Dictionary Data Structure In Python
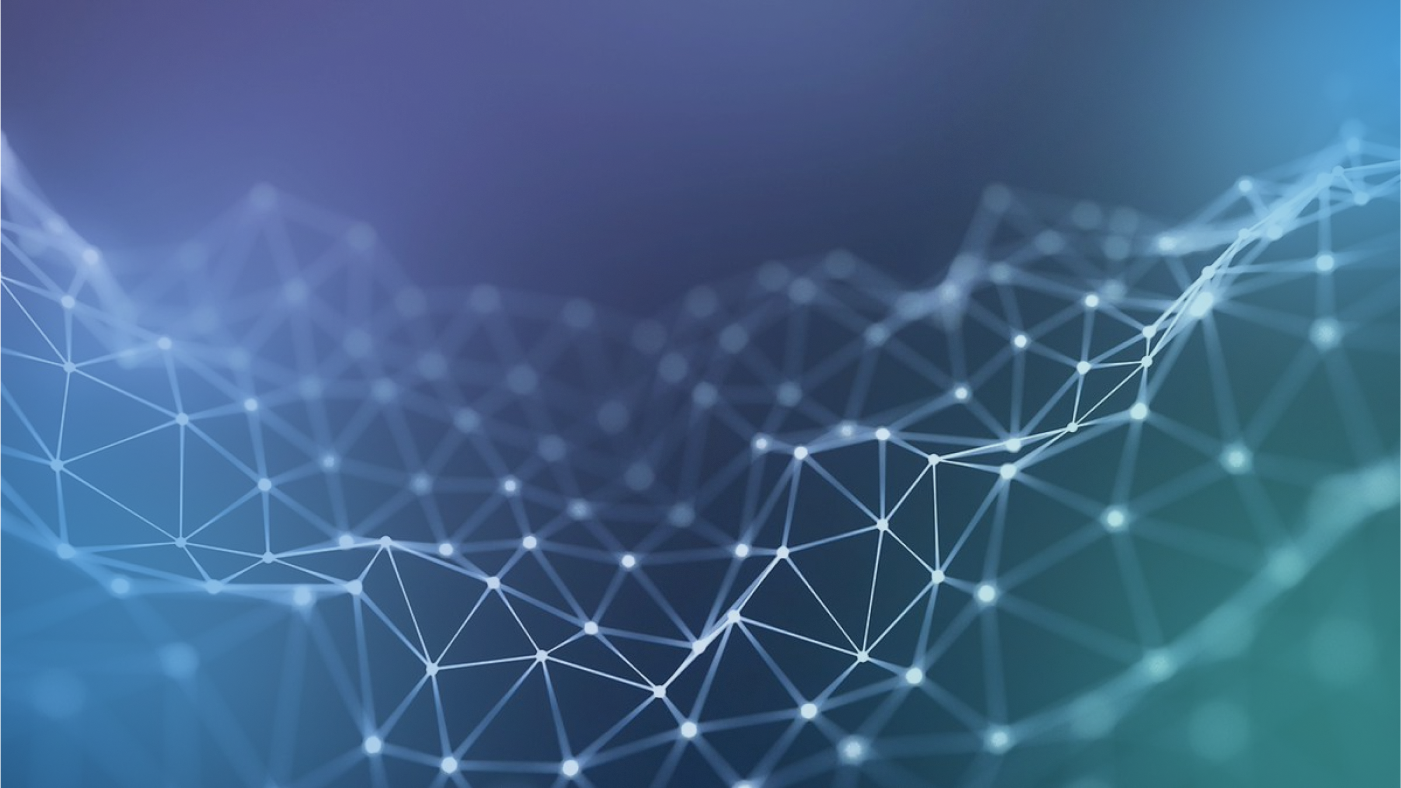
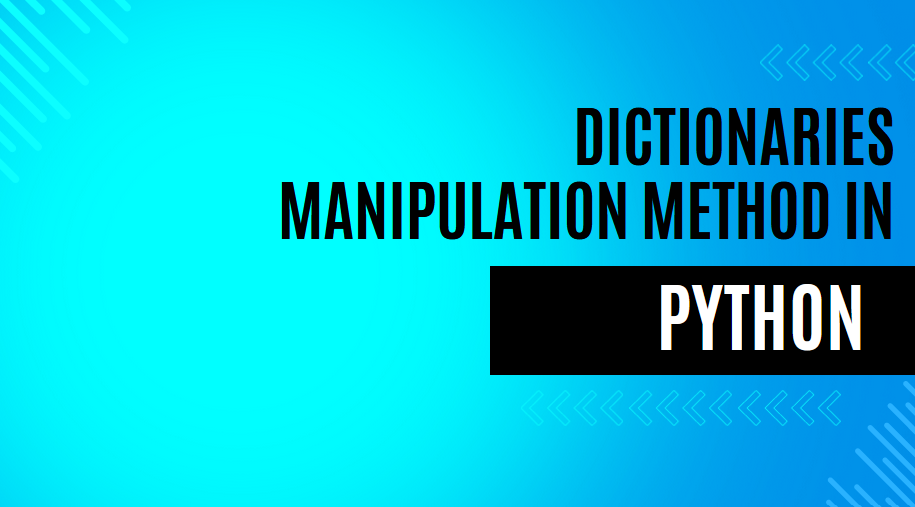
Python, a versatile and powerful programming language, boasts a rich set of data structures that contribute to its popularity among developers. One such fundamental data structure is the dictionary. In this blog post, we'll explore the ins and outs of dictionaries in Python, understanding their methods.
Creating a Dictionary
A dictionary is a built-in data type that represents a collection of key-value pairs. Dictionaries are enclosed in curly braces {}
.
dict_name = {} #Creates an empty dictionary
person = { "name": "John", "age": 30, "city": "New York"}
Accessing Values
You can access the values in a dictionary using their corresponding keys
.
name = person["name"]
age = person["age"]
Add or Modify
Inserts a new key-value pair into the dictionary. If the key already exists, the value will be updated; otherwise, a new entry is created.
person["Country"] = "USA" # A new entry will be created.
person["city"] = "Chicago" # Update the existing value for the same key
Clear()
The clear()
method empties the dictionary, removing all key-value pairs within it. After this operation, the dictionary is still accessible and can be used further.
person.clear()
Copy()
Creates a shallow copy of the dictionary. The new dictionary contains the same key-value pairs as the original, but they remain distinct objects in memory.
new_person = person.copy()
new_person = dict(person) # another way to create a copy of dictionary
del
Removes the specified key-value pair from the dictionary. Raises a KeyError
if the key does not exist.
del person["Country"]
items
Retrieves all key-value pairs as tuples and converts them into a list of tuples. Each tuple consists of a key and its corresponding value.
info = list(person.items())
In keyword
You can check for the existence of a key in a dictionary using the in
keyword
if "name" in person:
print("Name exists in the dictionary.")
keys()
Retrieves all keys from the dictionary and converts them into a list. Useful for iterating or processing keys using list methods.
person_keys = list(person.keys())
Update()
The update()
method merges the provided dictionary into the existing dictionary, adding or updating key-value pairs.
person.update({"Profession": "Doctor"})
values()
Extracts all values from the dictionary and converts them into a list. This list can be used for further processing or analysis.
person_values = list(person.values())
Subscribe to my newsletter
Read articles from Srushti directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
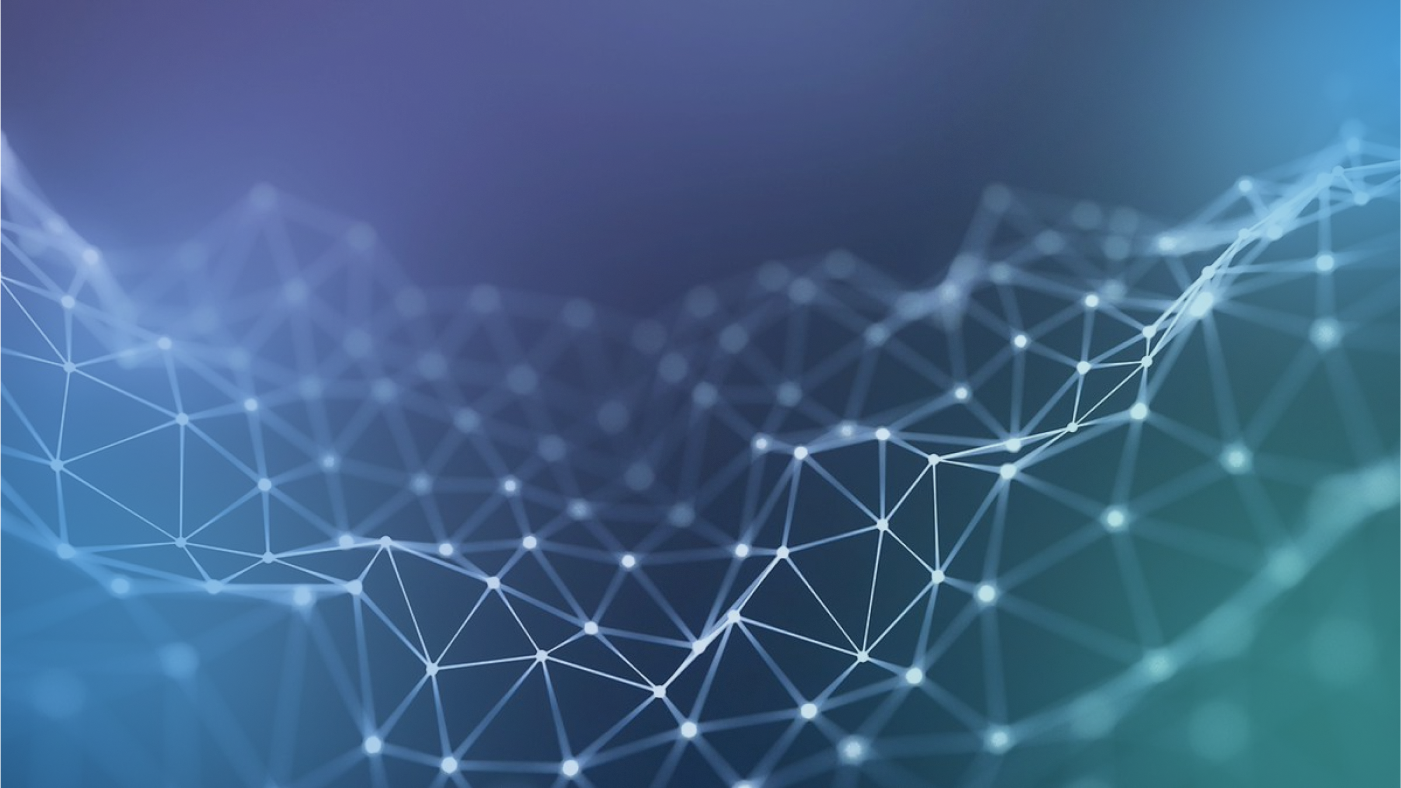