How to make a stopwatch in JavaScript?
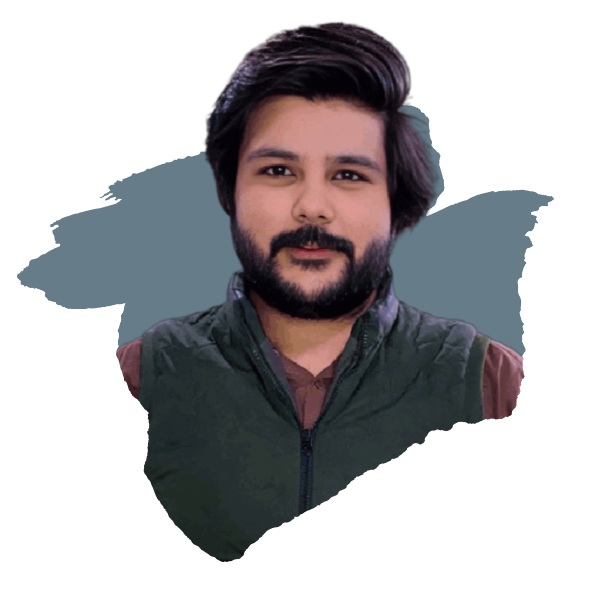
Table of contents
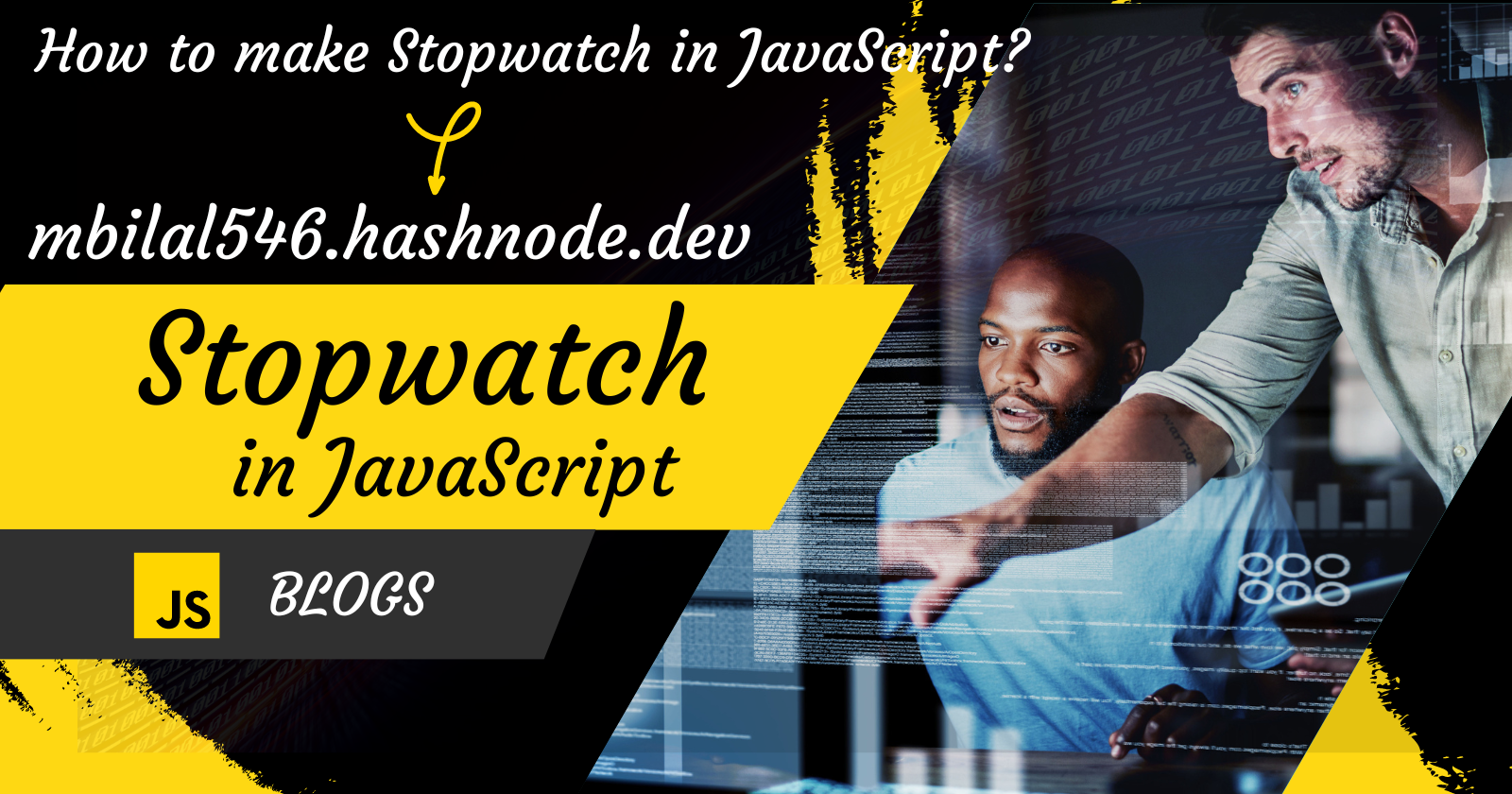
Output of the given code
Use the following code:
HTML code.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Stopwatch Project</title>
// Use this if you use seprate css file
<link rel="stylesheet" href="./stopwatch.css">
</head>
<body>
<h1>STOPWATCH</h1>
<div class="stopwatch">
<div class="timeDisplay">
00 : 00 : 00
</div>
</div>
<div class="buttons">
<button class="btn" style="--color:red;" id="stop">Stop</button>
<button class="btn" style="--color:green;" id="start">Start</button>
<button class="btn" style="--color:blue;" id="reset">Reset</button>
</div>
// Use this if you use seprate JS file
<script src="./stopwatch.js"></script>
</body>
</html>
CSS code.
*{
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: Verdana, Geneva, Tahoma, sans-serif;
}
body{
background-color: #212121;
color: #ffffff;
display: flex;
justify-content: center;
align-items: center;
min-height: 100vh;
flex-direction: column;
}
h1{
font-size: 82px;
margin-bottom: 100px;
color:crimson;
}
.stopwatch{
background-color: #000;
padding: 30px 35px;
border-radius: 10px;
}
.timeDisplay{
font-size: 72px;
font-size: 700;
}
.buttons{
margin-top: 30px;
}
.btn{
font-size: 20px;
background: none;
border: none;
color: #ffffff;
background-color: var(--color);
padding: 12px 24px;
margin: 0px 12px;
cursor: pointer;
border-radius: 6px;
font-weight: 600;
}
JavaScript code.
let timeDisplay = document.querySelector('.timeDisplay');
let startBtn = document.getElementById('start');
let stopBtn = document.getElementById('stop');
let resetBtn = document.getElementById('reset');
let milliSeconds = 0;
let seconds = 0;
let minutes = 0;
let timer = null;
startBtn.addEventListener('click', ()=>{
if (timer != null) {
clearInterval(timer);
}
timer = setInterval(() => {
milliSeconds++;
if (milliSeconds == 100) {
milliSeconds = 0;
seconds++;
if (seconds == 60) {
seconds = 0;
minutes++;
};
};
let ms = milliSeconds < 10 ? '0' + milliSeconds : milliSeconds;
let s = seconds < 10 ? '0' + seconds : seconds;
let m = minutes < 10 ? '0'+ minutes : minutes;
timeDisplay.innerHTML = `${m} : ${s} : ${ms}`;
}, 10);
});
stopBtn.addEventListener('click', ()=>{
clearInterval(timer);
});
resetBtn.addEventListener('click', ()=>{
clearInterval(timer);
milliSeconds = 0;
seconds = 0;
minutes = 0;
timeDisplay.innerHTML = '00 : 00 : 00';
});
Subscribe to my newsletter
Read articles from Muhammad Bilal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
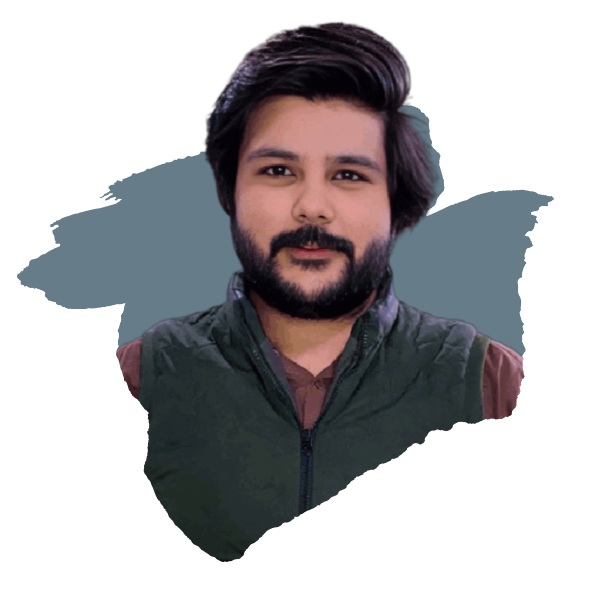
Muhammad Bilal
Muhammad Bilal
With a passion for creating seamless and visually appealing web experiences, I am Muhammad Bilal, a dedicated front-end developer committed to transforming innovative ideas into user-friendly digital solutions. With a solid foundation in HTML, CSS, and JavaScript, I specialize in crafting responsive and intuitive websites that captivate users and elevate brands. ๐ Professional Experience: In my two years of experience in front-end development, I have had the privilege of collaborating with diverse teams and clients, translating their visions into engaging online platforms. ๐ Skills: Technologies: HTML5, CSS3, JavaScript, WordPress Responsive Design: Bootstrap, Tailwind CSS, Flexbox, and CSS Grid Version Control: Git, GitHub ๐ฅ Projects: I have successfully contributed to projects where my focus on performance optimization and attention to detail played a crucial role in delivering high-quality user interfaces. โจ Continuous Learning: In the fast-paced world of technology, I am committed to staying ahead of the curve. Regularly exploring new tools and methodologies, I am dedicated to enhancing my skills and embracing emerging trends to provide the best possible user experience. ๐ Education: I hold a BSc degree in Software Engineering from Virtual University, where I gained both technical skills and knowledge. ๐ฅ Let's Connect: I am always open to new opportunities, collaborations, and discussions about the exciting possibilities in front-end development. Feel free to reach out via LinkedIn, Instagram, Facebook, Twitter, or professional email.