Embarking on the Python Journey: Day 13 of 90 Days of DevOps
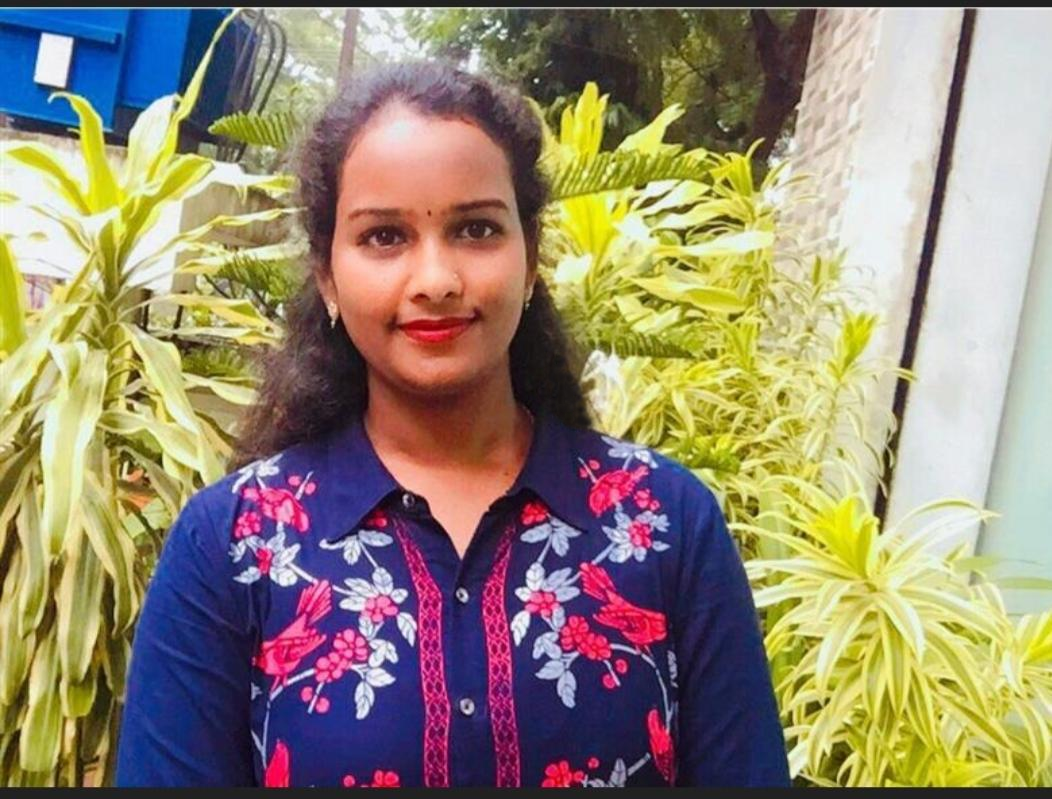
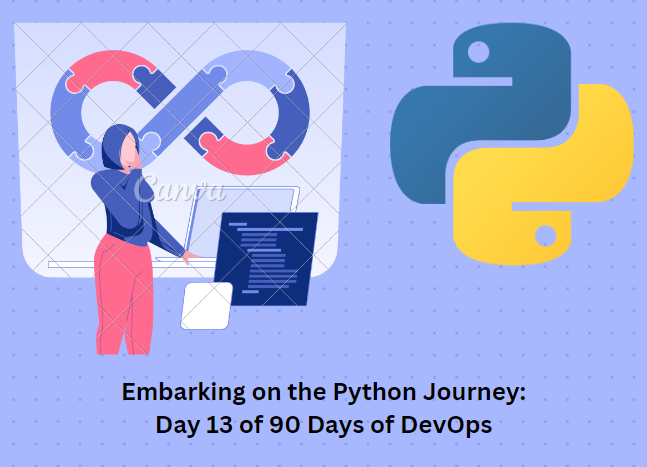
Welcome to Day 13 of the 90 Days of DevOps challenge! Today, we're diving into the fundamentals of Python, an essential programming language for DevOps engineers. Python's simplicity, versatility, and extensive libraries make it a powerful tool for automating tasks, scripting, and building applications in the DevOps ecosystem.
Introduction to Python:
Python is a high-level, interpreted programming language known for its readability, simplicity, and versatility. It was created by Guido van Rossum and first released in 1991. Python's design philosophy emphasizes code readability and a clean, expressive syntax, making it an excellent language for beginners and experienced programmers alike.
Setting Up Python Environment:
Before we dive into Python programming, let's make sure you have Python installed on your system. Here's how you can set up Python on different operating systems:
Windows Installation:
Visit the official Python website at python.org.
Download the latest version of Python for Windows.
Run the installer and follow the installation instructions.
Once installed, open Command Prompt and type
python --version
to check the installed Python version.
macOS Installation:
macOS typically comes with Python pre-installed. You can check the installed version by opening Terminal and typing
python --version
.If you need to install a specific version or manage Python versions, consider using a package manager like Homebrew.
Linux Installation (Ubuntu):
Open Terminal and run
sudo apt-get update
to update the package list.Install Python 3 by running
sudo apt-get install python3
.After installation, verify the installed version by typing
python3 --version
.
Python Basics:
Now that you have Python installed, let's explore some basic Python concepts:
Variables and Data Types:
In Python, variables are used to store data values. Python supports various data types, including integers, floats, strings, lists, tuples, dictionaries, and more. Here's a quick overview:
Integers: Whole numbers without a fractional part (e.g.,
5
,-10
).Floats: Numbers with a decimal point (e.g.,
3.14
,2.5
).Strings: Sequences of characters enclosed in single or double quotes (e.g.,
"Hello"
,'Python'
).Lists: Ordered collections of items enclosed in square brackets (e.g.,
[1, 2, 3]
).Tuples: Ordered collections of items enclosed in parentheses (e.g.,
(1, 2, 3)
).Dictionaries: Collections of key-value pairs enclosed in curly braces (e.g.,
{'name': 'John', 'age': 30}
).
Operators and Control Structures:
Python supports various operators for performing arithmetic, comparison, logical, and assignment operations. Additionally, Python provides control structures such as if statements, loops (for and while), and functions for controlling the flow of execution in a program.
Working with Python Libraries:
Python's extensive standard library and third-party libraries provide pre-built modules and functions for a wide range of tasks, including file operations, networking, web development, data analysis, and more. Throughout your Python journey, you'll learn how to leverage these libraries to perform various tasks efficiently.
Action Plan for Day 13:
Today marks the beginning of our journey into the world of Python, a versatile and powerful programming language essential for any DevOps engineer. Here's your action plan for Day 13 of the 90 Days of DevOps challenge:
Install Python: If you haven't already, take the first step by installing Python on your system. Follow the instructions provided above based on your operating system. Once installed, verify the installation by checking the Python version.
Explore Python Basics: Dive into the fundamentals of Python by familiarizing yourself with variables, data types, operators, and control structures.
Variables: Variables are used to store data in a program. In Python, you can create a variable and assign a value to it using the equals sign
=
. Variable names can contain letters, numbers, and underscores, but they cannot start with a number.Example:
# Variable assignment name = "John" age = 30
Data Types: Python supports various data types, including integers, floats, strings, booleans, lists, tuples, dictionaries, and more. Each data type has its own characteristics and operations.
Example:
# Integer data type num1 = 10 # Float data type num2 = 3.14 # String data type message = "Hello, Python!" # Boolean data type is_student = True
Tuple
A tuple in Python is a collection of ordered and immutable elements. This means that once a tuple is created, its elements cannot be changed, added, or removed. Tuples are often used to group related data together. Here's an example of a tuple:
# Tuple containing information about a person person = ("John", 30, "New York") # Accessing elements of the tuple name = person[0] age = person[1] city = person[2] # Printing the tuple elements print("Name:", name) print("Age:", age) print("City:", city)
In this example:
person
is a tuple containing three elements: the person's name, age, and city.We can access individual elements of the tuple using indexing. Indexing in Python starts from 0, so
person[0]
refers to the first element (name),person[1]
refers to the second element (age), andperson[2]
refers to the third element (city).Tuples are immutable, meaning we cannot modify their elements once they are created. Attempting to reassign or modify elements of a tuple will result in an error.
Sure, here are examples of lists and dictionaries in Python:
Lists:
A list in Python is a collection of ordered and mutable elements. Lists can contain elements of different data types, and you can modify the elements of a list after it has been created. Here's an example:
# List of numbers
numbers = [1, 2, 3, 4, 5]
# List of strings
fruits = ["apple", "banana", "orange", "grape"]
# List of mixed data types
mixed_list = [1, "hello", True, 3.14]
# Accessing elements of a list
print(numbers[0]) # Output: 1
print(fruits[2]) # Output: orange
# Modifying elements of a list
numbers[0] = 10
print(numbers) # Output: [10, 2, 3, 4, 5]
# Adding elements to a list
fruits.append("watermelon")
print(fruits) # Output: ['apple', 'banana', 'orange', 'grape', 'watermelon']
Dictionaries:
A dictionary in Python is a collection of key-value pairs. Each key in a dictionary must be unique, and it maps to a corresponding value. Dictionaries are unordered and mutable. Here's an example:
# Dictionary of student information
student = {
"name": "John",
"age": 25,
"city": "New York"
}
# Accessing values in a dictionary
print(student["name"]) # Output: John
print(student["age"]) # Output: 25
# Modifying values in a dictionary
student["age"] = 30
print(student) # Output: {'name': 'John', 'age': 30, 'city': 'New York'}
# Adding new key-value pairs to a dictionary
student["grade"] = "A"
print(student) # Output: {'name': 'John', 'age': 30, 'city': 'New York', 'grade': 'A'}
In these examples:
numbers
,fruits
, andmixed_list
are examples of lists containing elements of various data types.student
is an example of a dictionary containing information about a student, where keys represent attributes (e.g., name, age, city) and values represent corresponding data.
Operators:
Operators are symbols used to perform operations on variables and values. Python supports various operators, including arithmetic, comparison, logical, assignment, and more.
Example:
# Arithmetic operators result = 10 + 5 print("Addition:", result) # Comparison operators is_greater = 10 > 5 print("Greater than:", is_greater) # Logical operators is_both_true = True and False print("Both true:", is_both_true)
Control Structures: Control structures, such as if statements, loops, and function definitions, control the flow of execution in a Python program.
Example:
# If statement num = 10 if num > 0: print("Positive number") elif num < 0: print("Negative number") else: print("Zero")
for
Loop: Thefor
loop is used to iterate over a sequence (such as a list, tuple, or string) and execute a block of code for each item in the sequence.Example:
python pythonCopy code# Iterate over a list of numbers and print each number numbers = [1, 2, 3, 4, 5] for num in numbers: print(num)
*
while
Loop: Thewhile
loop is used to repeatedly execute a block of code as long as a specified condition is true.Example:
python pythonCopy code# Print numbers from 1 to 5 using a while loop num = 1 while num <= 5: print(num) num += 1
Hands-On Practice: Put your newly acquired knowledge into practice by completing exercises provided in tutorials or creating your own small projects. One excellent exercise is to write a Python script to calculate the area of a circle or convert temperature units. Don't hesitate to challenge yourself and explore different Python concepts.
Here's a simple example of calculating the area of a circle using Python:
pythonCopy codeimport math def calculate_circle_area(radius): return math.pi * radius ** 2 radius = float(input("Enter the radius of the circle: ")) area = calculate_circle_area(radius) print("The area of the circle is:", area)
Learning Resources: Expand your understanding of Python basics by exploring online tutorials, documentation, and courses. Platforms like Real Python, Codecademy, and Automate the Boring Stuff with Python offer comprehensive resources tailored for beginners and experienced programmers alike. Take advantage of these resources to deepen your knowledge and skills.
Here are some recommended resources to explore:
Codecademy
AutomatetheBoring Stuff with Python
By following this action plan, you'll lay a solid foundation in Python programming, setting the stage for further exploration and mastery in the daysahead. Remember, practice is key, so don't hesitate to experiment and challenge yourself. Happy coding! ๐โจ
Subscribe to my newsletter
Read articles from SWATHI PUNREDDY directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
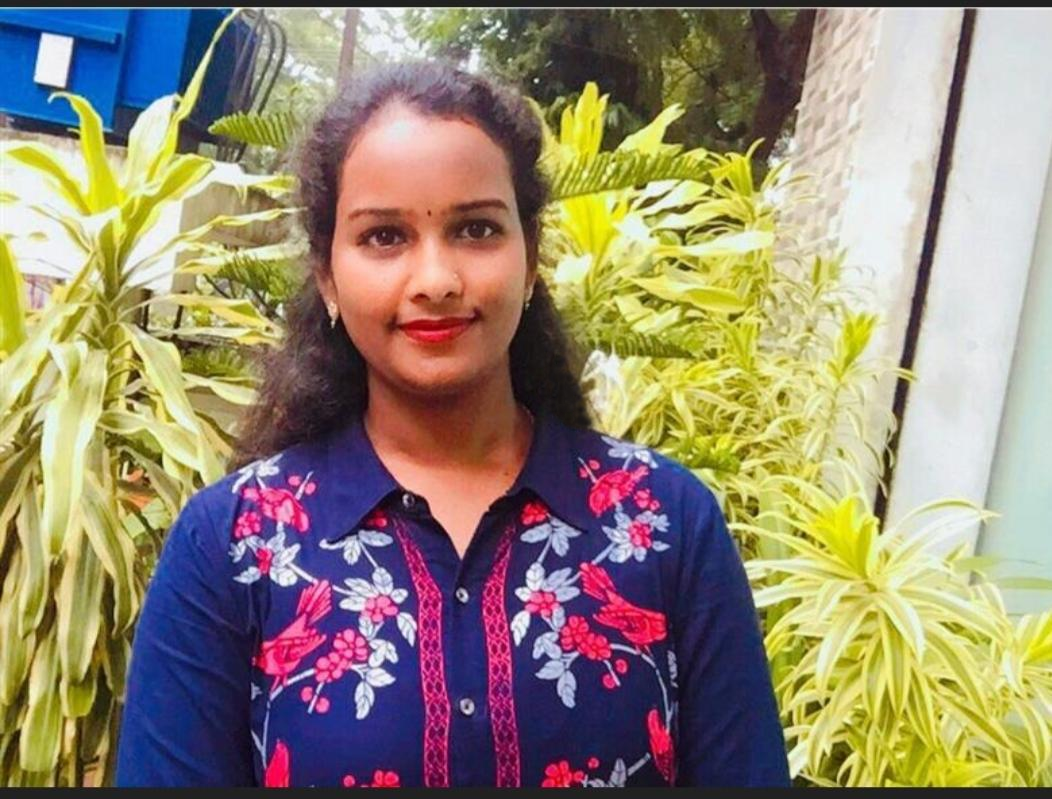