Sorting a List of Objects in Java: A Configurable Approach with Comparator and Enums
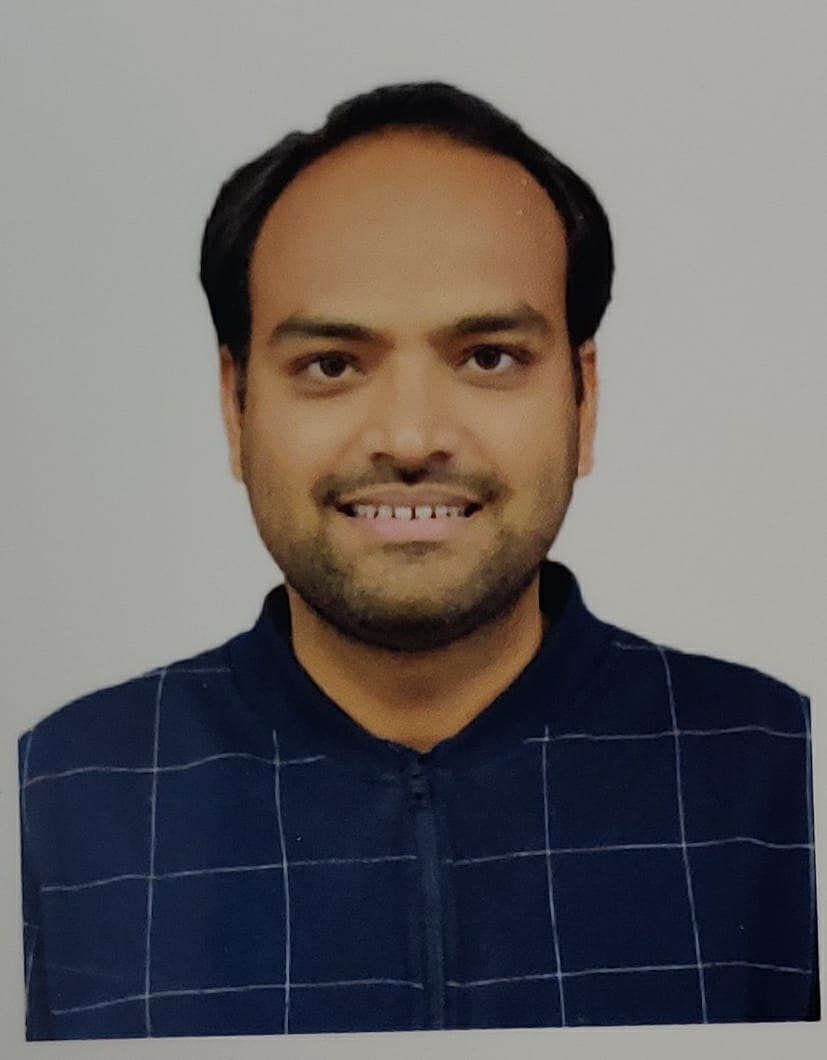
In this article, I have shown a way to sort a list of Employee object based on a specific field and the sorting order using Comparator. The code snippet shows that the sorting criteria are configurable, eliminating the need to add an if/else block when a new criterion is introduced.
class Employee {
private int ID;
private String name;
private String age;
public int getID() {
return ID;
}
public void setID(int ID) {
this.ID = ID;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAge() {
return age;
}
public void setAge(String age) {
this.age = age;
}
}
Create two enums SortingCriteria and SortingOrder.
enum SortingCriteria {
NAME, AGE
}
enum SortingOrder {
ASC, DESC
}
Now create a Map to store SortingCriteria mapping with the ascending order (default) Comparators e.g., Name comparator, Age comparator, etc.
static Map<SortingCriteria, Comparator<Employee>> comparatorMap = Map.of(NAME, Comparator.comparing(Employee::getName), AGE, Comparator.comparing(Employee::getAge));
Create a method to accept the SortingCriteria and the SortingOrder as user input and return the sorted employees. Here we are assuming that a List of employees is already available.
static List<Employee> sort(SortingCriteria criteria, SortingOrder order) {
List<Employee> employees = new ArrayList<>(); // consider populated
//If order is DESC then we take reversed() comparator
Comparator<Employee> employeeComparator = order == ASC ? comparatorMap.get(criteria) : comparatorMap.get(criteria).reversed();
employees.sort(employeeComparator);
return employees;
}
Conclusion: The benefit of this approach is that to enable sorting for a new criterion, let's say ID, we just need to add ID in SortingCriteria and add a new mapping in the comparatorMap. This way, the core sorting logic remains unchanged.
Subscribe to my newsletter
Read articles from Alok Dubey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
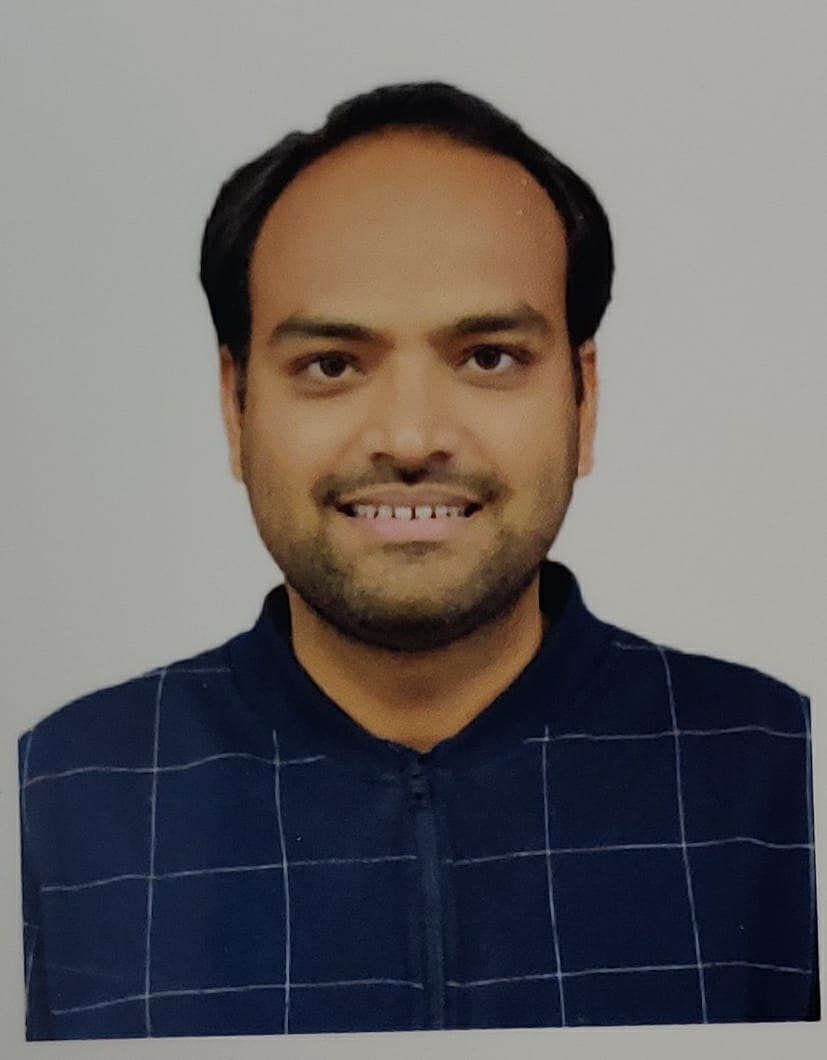