Day 14 : Python Data Types and Data Structures for DevOps

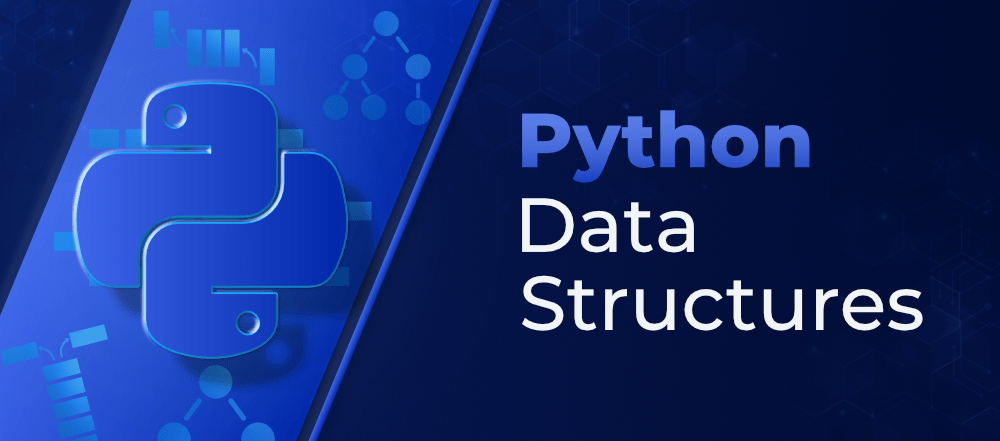
In the world of DevOps, efficiency and automation are paramount. Python, with its versatility and robustness, is a preferred choice for many DevOps professionals. Understanding Python data types and data structures is fundamental for effective scripting and automation in DevOps workflows. Let's delve into these concepts and explore how they can be applied in real-world scenarios.
Data Types
Python offers several built-in data types to represent different kinds of data. Some of the commonly used data types in DevOps include:
Numeric Types: Integers (
int
) and Floating-Point numbers (float
) are used to represent numeric data such as counts, measurements, and other numerical values.String Type: Strings (
str
) are sequences of characters enclosed within single, double, or triple quotes. Strings are used to represent textual data, such as file paths, configuration details, or logs.Boolean Type: Booleans (
bool
) represent truth values, eitherTrue
orFalse
. Booleans are used for conditional expressions and logical operations.List Type: Lists (
list
) are ordered collections of items, mutable and can contain elements of different data types. Lists are extensively used for storing and manipulating data in sequences.Tuple Type: Tuples (
tuple
) are ordered collections of items, immutable and similar to lists but cannot be modified once created. Tuples are often used to represent fixed collections of data.Set Type: Sets (
set
) are unordered collections of unique items. Sets are used for mathematical operations like union, intersection, and difference.Dictionary Type: Dictionaries (
dict
) are unordered collections of key-value pairs. Dictionaries are efficient for storing and retrieving data using keys.
Data Structures
Data structures are fundamental components of programming languages that allow you to organize, store, and manipulate data efficiently. They provide a way to represent and manage collections of data, each with its own properties and methods. Here are some common data structures:
Arrays: An array is a collection of elements stored at contiguous memory locations. It is the most basic and widely used data structure, often used to store a fixed-size sequential collection of elements of the same type.
Linked Lists: A linked list is a linear data structure where elements are stored in nodes. Each node consists of data and a reference (or pointer) to the next node in the sequence. Linked lists can be singly linked (each node points to the next node) or doubly linked (each node points to both the next and previous nodes).
Stacks: A stack is a data structure that follows the Last In, First Out (LIFO) principle. Elements can be inserted (pushed) and removed (popped) only from the top of the stack. It operates on the principle of "Last in, first out."
Queues: A queue is a data structure that follows the First In, First Out (FIFO) principle. Elements can be inserted at the rear (enqueue) and removed from the front (dequeue). It operates on the principle of "First in, first out."
Trees: A tree is a hierarchical data structure consisting of nodes connected by edges. Each node has a value and zero or more child nodes, forming a hierarchical structure. Common types of trees include binary trees, binary search trees, and balanced trees like AVL trees and Red-Black trees.
Graphs: A graph is a collection of nodes (vertices) and edges that connect pairs of nodes. Graphs can be directed (edges have a direction) or undirected. They are used to model relationships between entities.
Hash Tables: A hash table is a data structure that stores key-value pairs. It uses a hash function to compute an index into an array of buckets or slots, from which the desired value can be found. Hash tables provide fast retrieval of data and are often used for implementing associative arrays, sets, and caches.
Sets: A set is a collection of unique elements with no specific order. It is useful for tasks that require checking for the presence of elements or removing duplicates from a collection.
Tasks
Give the Difference between List, Tuple and set. Do Hands-on and put screenshots as per your understanding.
Lists:
Lists are ordered collections of items.
They are mutable, meaning the elements can be changed after the list is created.
Lists are defined using square brackets
[ ]
.Lists allow duplicate elements.
Example:
my_list = [1, 2, 3, 4, 5]
Tuples:
Tuples are ordered collections of items similar to lists.
However, tuples are immutable, meaning once they are created, their elements cannot be changed.
Tuples are defined using parentheses
( )
.Tuples allow duplicate elements.
Example:
my_tuple = (1, 2, 3, 4, 5)
Sets:
Sets are unordered collections of unique items.
They are mutable, meaning elements can be added or removed after the set is created.
Sets are defined using curly braces
{ }
.Sets do not allow duplicate elements. If you try to add a duplicate element, it will be ignored.
Example:
my_set = {1, 2, 3, 4, 5}
Now, let's see some sample code demonstrating these differences:
As you can see from the screenshots, lists and tuples maintain the order of elements and allow duplicates, while sets do not maintain the order and eliminate duplicates. Additionally, tuples are immutable, so you cannot change their elements once created.
Create below Dictionary and use Dictionary methods to print your favourite tool just by using the keys of the Dictionary.
Create a List of cloud service providers
Write a program to add
Digital Ocean
to the list of cloud_providers and sort the list in alphabetical order.
Conclusion:
Mastering Python data types and data structures is indispensable for effective DevOps automation and scripting. By leveraging these concepts, DevOps engineers can streamline workflows, manage infrastructure efficiently, and build robust automation solutions.
I'm confident that this article will prove to be valuable, helping you discover new insights and learn something enriching .
thank you : )
Subscribe to my newsletter
Read articles from Prathmesh Vibhute directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
