Data Handling in Node.js
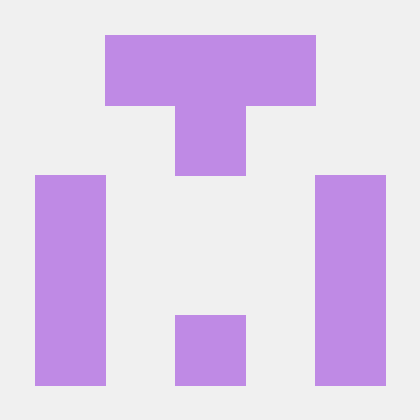
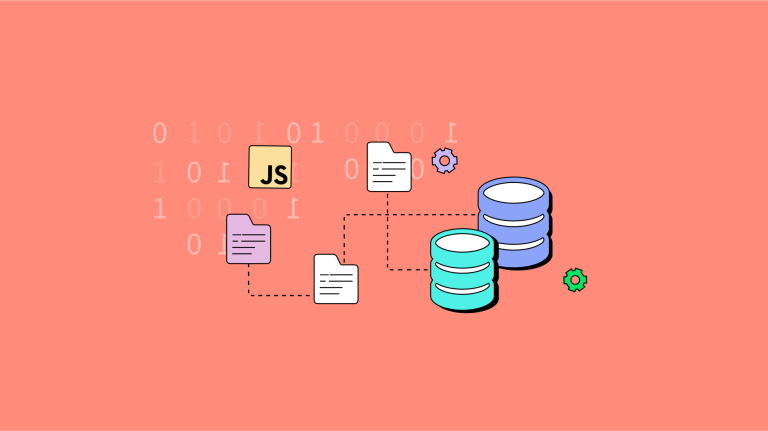
Introduction: In the digital age, data is at the heart of every application. As developers, mastering data handling is essential for building efficient, and reliable systems. Node.js, with its asynchronous nature provides powerful libraries for managing data effectively. In this blog post, we'll delve into the world of data handling in Node.js, exploring various techniques and tools to empower you in your journey as a data-driven developer.
Understanding Data Handling in Node.js: Data handling in Node.js includes a wide range of tasks, including reading and writing files, interacting with databases, processing streams, and managing asynchronous operations. Node.js's event-driven architecture makes it particularly well-suited for handling large volumes of data efficiently.
Reading and Writing Files: Node.js provides built-in modules like fs
(File System) for reading from and writing to files. Whether it's reading configuration files, parsing CSV data, or generating reports, Node.js offers straightforward APIs for file manipulation.
const fs = require('fs');
// Reading from a file
fs.readFile('data.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
// Writing to a file
fs.writeFile('output.txt', 'Hello, World!', (err) => {
if (err) throw err;
console.log('File written successfully');
});
Interacting with Databases: Node.js supports a variety of databases, including SQL (e.g., MySQL, PostgreSQL) and NoSQL (e.g., MongoDB). Libraries like mysql
, pg
, mongodb
, and redis
provide convenient APIs for connecting to and databases
const mysql = require('mysql');
// Creating a connection to MySQL database
const connection = mysql.createConnection({
host: 'localhost',
user: 'root',
password: 'password',
database: 'mydb'
});
// Querying data from MySQL database
connection.query('SELECT * FROM users', (error, results) => {
if (error) throw error;
console.log(results);
});
// Closing the connection
connection.end();
Processing Streams:
Node.js's Stream API is a powerful feature that enables developers to handle data efficiently, especially when dealing with large volumes of data or real-time processing scenarios. Streams in Node.js represent a continuous flow of data that can be read from or written to asynchronously, making them ideal for tasks such as file compression, data transformation, and real-time data processing.
Types of Streams:
Readable Streams: Readable streams allow you to read data from a source, such as a file, HTTP request, or network socket. You can consume data from readable streams using event listeners or by piping them to writable streams or transform streams for processing.
Writable Streams: Writable streams allow you to write data to a destination, such as a file, HTTP response, or network socket. You can write data to writable streams using methods like
write()
or by piping data from readable streams or transform streams.Transform Streams: Transform streams are a special type of duplex stream that allow you to modify or transform data as it passes through the stream. They are both readable and writable, making them useful for tasks like data encryption, compression, or parsing.
Example Use Cases:
File Compression: Streams can be used to compress or decompress files on-the-fly, reducing memory usage and improving performance. For example, you can use the
zlib
module to create a gzip-compressed file from a readable stream and then pipe the compressed data to a writable stream.Data Transformation: Streams can be used to transform data from one format to another, such as converting CSV data to JSON or XML. You can use transform streams to parse and serialize data as it passes through the stream, allowing for efficient processing of large datasets.
Real-Time Data Processing: Streams are ideal for processing real-time data streams, such as sensor data, log files, or network traffic. You can use readable streams to consume data as it becomes available and process it in real-time using transform streams or writable streams.
const fs = require('fs');
const zlib = require('zlib');
// Creating a readable stream
const readableStream = fs.createReadStream('input.txt');
// Creating a writable stream
const writableStream = fs.createWriteStream('output.txt');
// Piping data through a transform stream (e.g., gzip compression)
readableStream.pipe(zlib.createGzip()).pipe(writableStream);
Conclusion:
Node.js's Stream API is a versatile and powerful feature that enables efficient data handling in a wide range of scenarios. Whether you're compressing files, transforming data, or processing real-time streams, streams provide a flexible and scalable solution for handling data in Node.js applications. By understanding the different types of streams, their use cases, and best practices for working with streams, you can harness the full potential of Node.js's Stream API and build high-performance & data-driven applications.
Let me know your thoughts in the comments below.
#Explore. Code. Evolve.
Happy coding! ๐
Subscribe to my newsletter
Read articles from Amod directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
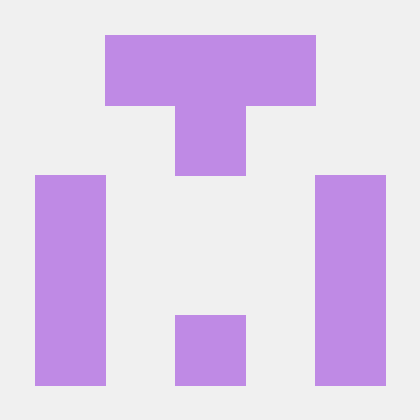