Dictionaries in Python
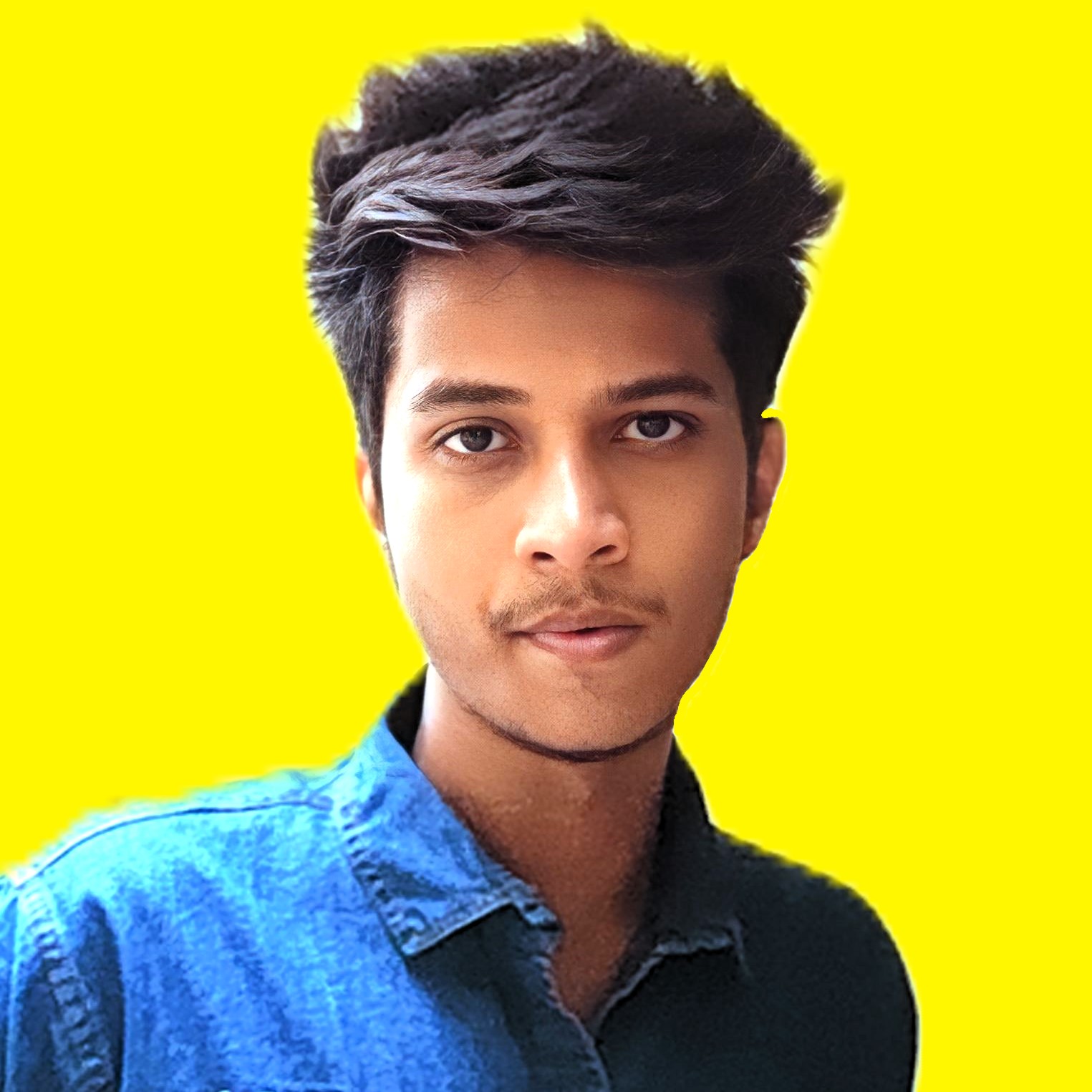
Dictionaries are an essential data structure in Python, offering a flexible way to store and manipulate data. They consist of key-value pairs, allowing for efficient data retrieval and manipulation. In this guide, we'll delve into the fundamentals of dictionaries through practical examples and explore various methods to work with them effectively.
Understanding Dictionaries
Let's start with a basic understanding of dictionaries using a common example: types of chai.
chai_types = { "Masala": "Spicy", "Ginger": "Zesty", "Green": "Mild" }
In this dictionary:
Keys are the types of chai (e.g., "Masala", "Ginger", "Green").
Values represent the corresponding flavor profiles (e.g., "Spicy", "Zesty", "Mild").
Accessing Values
Accessing values in a dictionary can be done using keys:
print(chai_types["Masala"]) # Output: Spicy
print(chai_types.get("Ginger")) # Output: Zesty
Handling Missing Keys
When trying to access a key that doesn't exist, KeyError
is raised. To avoid this, get()
method can be used:
print(chai_types.get("Gingery")) # Output: None
Modifying and Adding Elements
Dictionaries are mutable, allowing for easy modification:
chai_types["Green"] = "Fresh"
This line updates the value of the "Green" key to "Fresh".
Iterating Through a Dictionary
Iterating over a dictionary provides access to keys:
for type in chai_types:
print(type)
# Output:
# Masala
# Ginger
# Green
Accessing Keys and Values Simultaneously
To access both keys and values in a loop, use items()
:
for key, value in chai_types.items():
print(key, value)
# Output:
# Masala Spicy
# Ginger Zesty
# Green Fresh
Common Operations
Checking for Key Existence
To check if a key exists in a dictionary:
if "Masala" in chai_types:
print("I have Masala chai") # Output: I have Masala chai
Length of a Dictionary
To get the number of key-value pairs:
print(len(chai_types)) # Output: 3
Removing Elements
You can remove elements using pop()
or popitem()
:
chai_types.pop("Earl Grey") # Removes and returns the value of "Earl Grey"
chai_types.popitem() # Removes and returns the last inserted item
Deleting Entire Dictionary or Specific Items
del chai_types['Ginger'] # Removes the key "Ginger" and its value
Copying Dictionaries
To create a copy of a dictionary:
chai_types_copy = chai_types.copy()
Advanced Techniques
Dictionary Comprehension
Using dictionary comprehension to create dictionaries:
squared_num = {x: x**2 for x in range(5)}
print(squared_num) # Output: {0: 0, 1: 1, 2: 4, 3: 9, 4: 16}
Creating Dictionaries with Default Values
keys = ["Masala", "Ginger", "Lemon"]
default_value = "Nice"
new_dict = dict.fromkeys(keys, default_value)
print(new_dict) # Output: {'Masala': 'Nice', 'Ginger': 'Nice', 'Lemon': 'Nice'}
Will meet you in the next article on Tuples.
Subscribe to my newsletter
Read articles from Hruthik ks directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
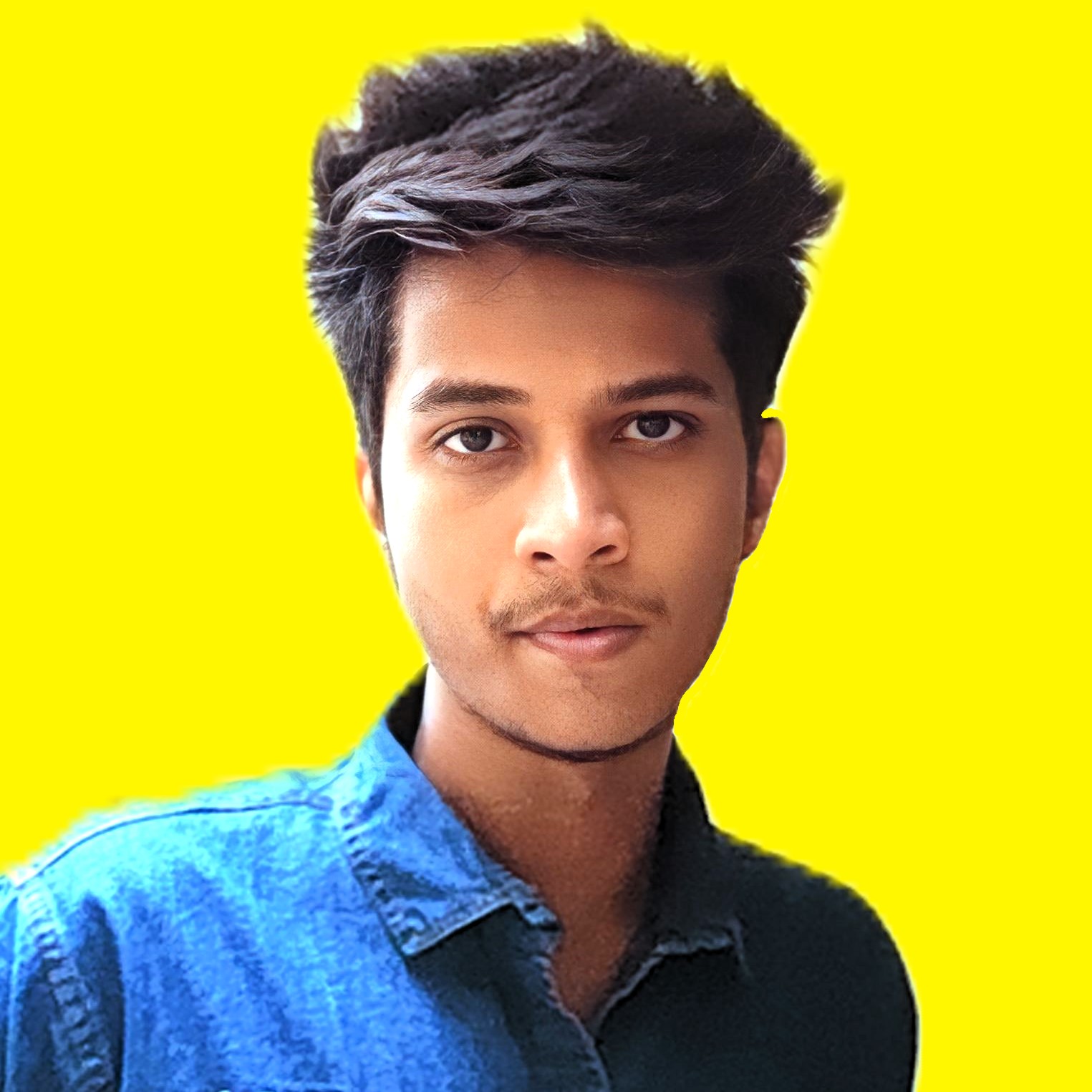