How to create ERC20 Tokens code breakdown
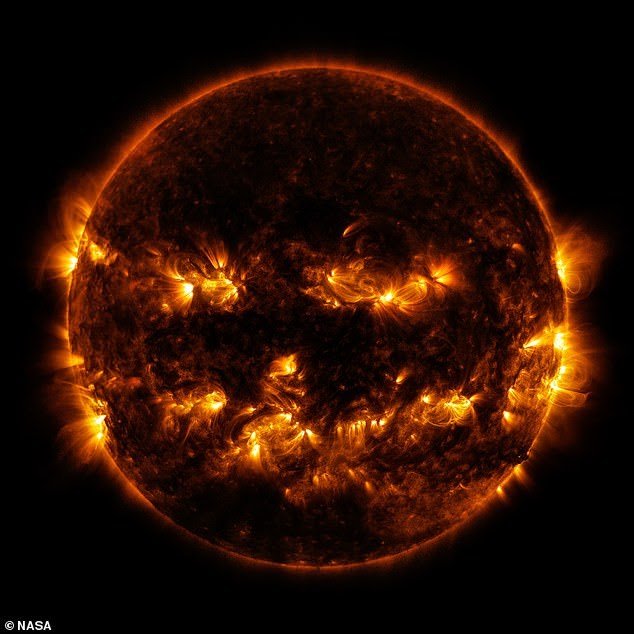
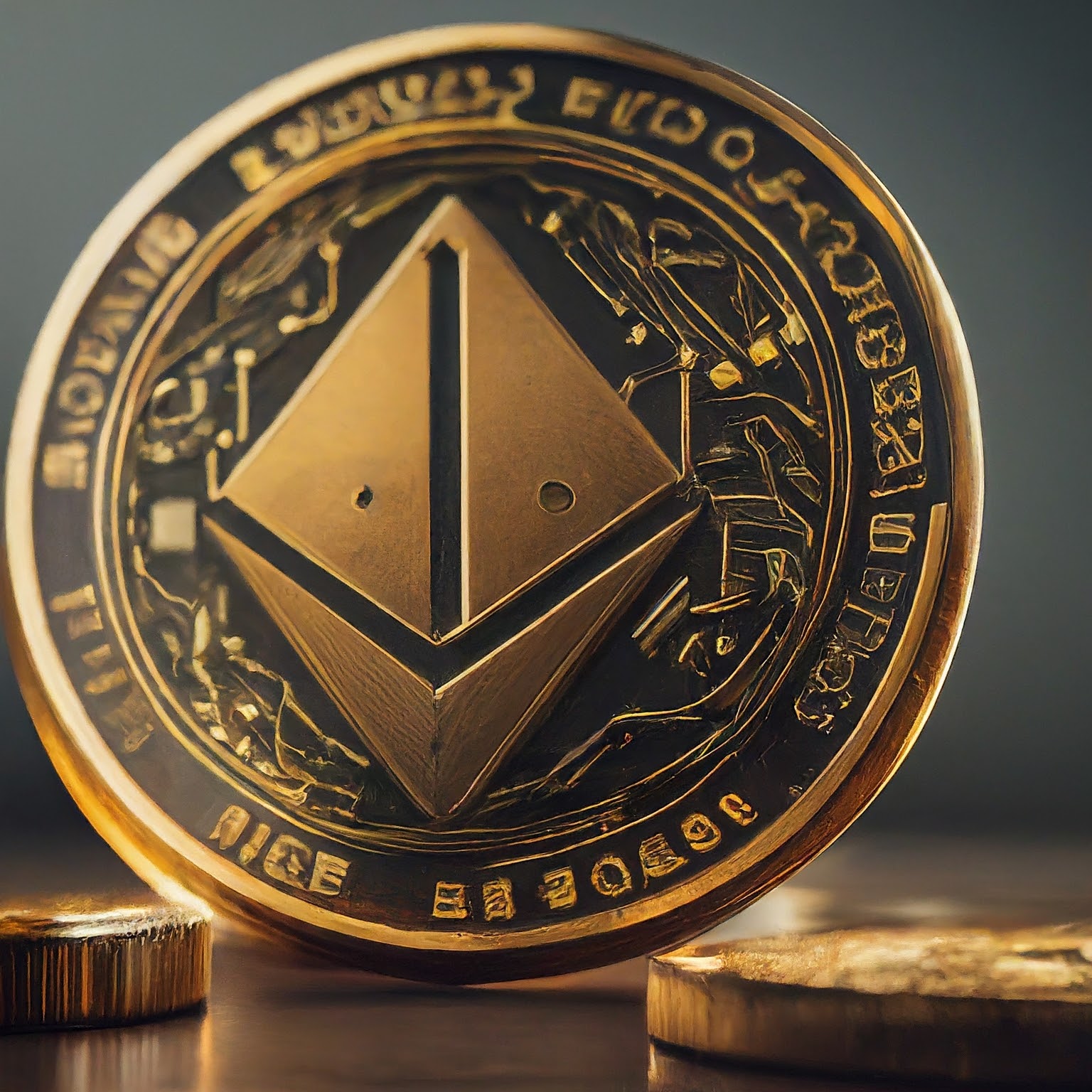
Sure, here is a more organized article breakdown of the BFAToken smart contract:
BFAToken: A Decentralized Token with a Built-in Burn Mechanism
The BFAToken smart contract is an ERC-20 token implemented on the Ethereum blockchain that incorporates a unique burn mechanism to reduce the total supply of tokens over time. This article delves into the key features and functionalities of the contract, providing a comprehensive understanding of its inner workings.
Key Features:
Token Creation and Management: The contract facilitates the creation and management of the BFA Token, including defining its name, symbol, and decimal precision.
Transfer and Allowance: Users can seamlessly transfer tokens between addresses and leverage the allowance mechanism to authorize other parties to spend their tokens on their behalf.
Built-in Burn Mechanism: A distinctive feature of the contract is its automatic token burn, where 10% of each transferred amount is permanently removed from circulation, effectively reducing the total supply and potentially increasing the token's value over time.
Code Breakdown:
Contract Definition: The smart contract is named BFAToken and adheres to the ERC-20 token standard, ensuring compatibility with ETH wallets and applications.
Token Properties: Essential token attributes like name, symbol, and decimal places are clearly defined within the contract's initial code.
string public name = "BFA Token"; // Token name string public symbol = "BFA"; // Token symbol uint8 public decimals = 18; // Decimal precision
Total Supply: The variable
totalSupply
acts as a ledger, keeping track of the total number of BFA Tokens in circulation.// Total supply of tokens (initialize in constructor) uint256 public totalSupply;
Balance Tracking: An address-based mapping named
balances
stores the individual token balances for each user.// Mapping to store individual token balances mapping(address => uint256) public balances;
Allowance Mechanism: The
allowances
mapping records how much a specific spender is authorized to transfer tokens on behalf of another user (the owner).// Mapping to track allowed transfer amounts (spender => owner => amount) mapping(address => mapping(address => uint256)) private allowances;
Event Recording: Two events,
Transfer
andApproval
, are triggered during token transfers and allowance modifications, respectively, providing a transparent record of these actions on the blockchain.// Event emitted when tokens are transferred event Transfer(address indexed from, address indexed to, uint256 value); // Event emitted when allowance is changed event Approval(address indexed owner, address indexed spender, uint256 value);
Constructor: Upon contract deployment, the constructor initializes the total token supply and assigns the entire supply to the deployer's address.
// Initial constructor to set the total supply (modify here) constructor(uint256 initialSupply) { totalSupply = initialSupply * 10**decimals; // Adjust for decimal precision balances[msg.sender] = totalSupply; // Grant total supply to deployer }
Balance Inquiry: The
balanceOf
function enables users to query the token balance of any address on the BSC network.// Function to get the balance of a specific account function balanceOf(address account) external view returns (uint256) { return balances[account]; }
Token Transfer with Burn: The
transfer
function facilitates user-initiated token transfers while incorporating the burn mechanism. Notably, 10% of the transferred amount is automatically burned, reducing both the sender's balance and the overall supply.function transfer(address recipient, uint256 amount) external returns (bool) { uint256 _burn_amount = amount / 10; require(balances[msg.sender] >= (amount + _burn_amount), "Insufficient balance"); totalSupply -= _burn_amount; balances[msg.sender] -= (amount + _burn_amount); balances[recipient] += amount; emit Transfer(msg.sender, recipient, amount); return true; }
Allowance Check: The
allowance
function allows users to verify the spending limit granted to a specific spender for their tokens.// Function to check the allowance of a spender for a specific owner function allowance(address owner, address spender) external view returns (uint256) { return allowances[owner][spender]; }
Allowance Granting: The
approve
function empowers token holders to authorize other addresses to transfer their tokens up to a specified amount.// Function to approve a spender to transfer tokens on the owner's behalf function approve(address spender, uint256 amount) external returns (bool) { require(balances[msg.sender] >= amount, "Cannot give allowance greater than balance"); allowances[msg.sender][spender] = amount; emit Approval(msg.sender, spender, amount); return true; }
Transfer Using Allowance: The
transferFrom
function enables authorized spenders to transfer tokens on behalf of owners, also applying the 10% burn mechanism on the transferred amount.// Function to transfer tokens from one address to another using an allowance function transferFrom( address sender, address recipient, uint256 amount ) external returns (bool) { // Check if sender has enough balance or approved allowance uint256 _burn_amount = amount / 10; require(balances[sender] >= (amount + _burn_amount) && allowances[sender][msg.sender] >= amount, "Insufficient funds or allowance"); // Update balances and allowance accordingly balances[sender] -= (amount + _burn_amount); allowances[sender][msg.sender] -= (amount + _burn_amount); // Decrement allowance if used totalSupply -= _burn_amount; balances[recipient] += amount; emit Transfer(sender, recipient, amount); return true; }
Additional Considerations:
Security: The contract incorporates
require
statements to enforce essential conditions and prevent potential errors or vulnerabilities during token transfers and allowance management. More checks can be added to ensure the contract is safe from attacksExtensibility: The core structure of the BFAToken contract can be extended to incorporate additional features or functionalities as needed, catering to evolving requirements or use cases.
Conclusion:
The BFAToken smart contract offers a well-designed and secure foundation for launching a decentralized token with a unique burn mechanism on the Binance Smart Chain. Its core functionalities cater to essential token creation, management, transfer, and allowance operations.
Subscribe to my newsletter
Read articles from Benjamin Faruna directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
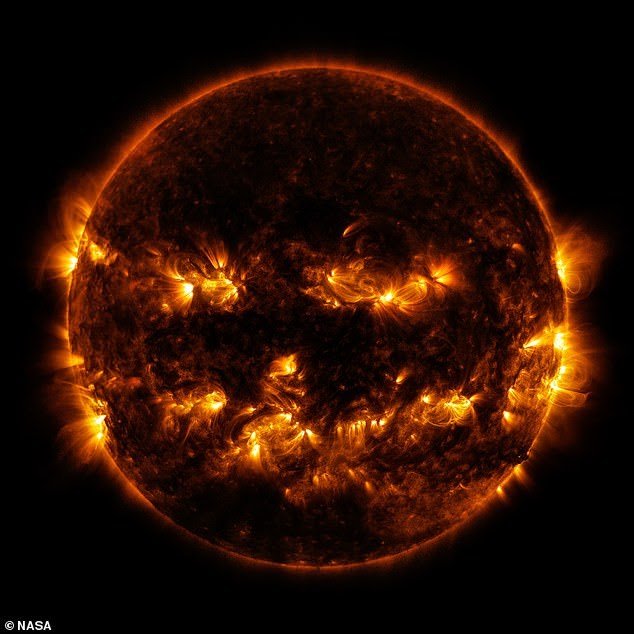
Benjamin Faruna
Benjamin Faruna
I am a developer from Nigeria, still trying to find what clicks and resonates with me. Coding is a hobby and I intend to keep it that way even when I am making profits off it.