Day 63 - Terraform Variables
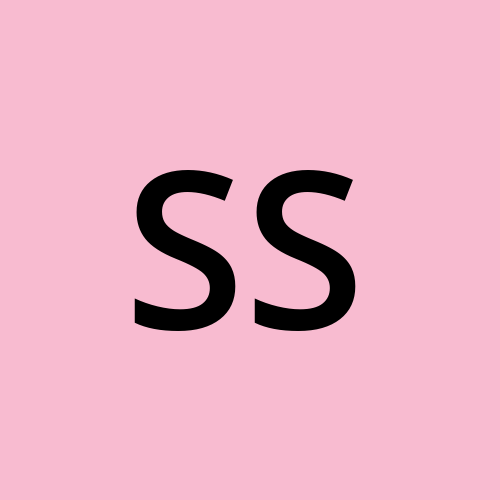
Table of contents
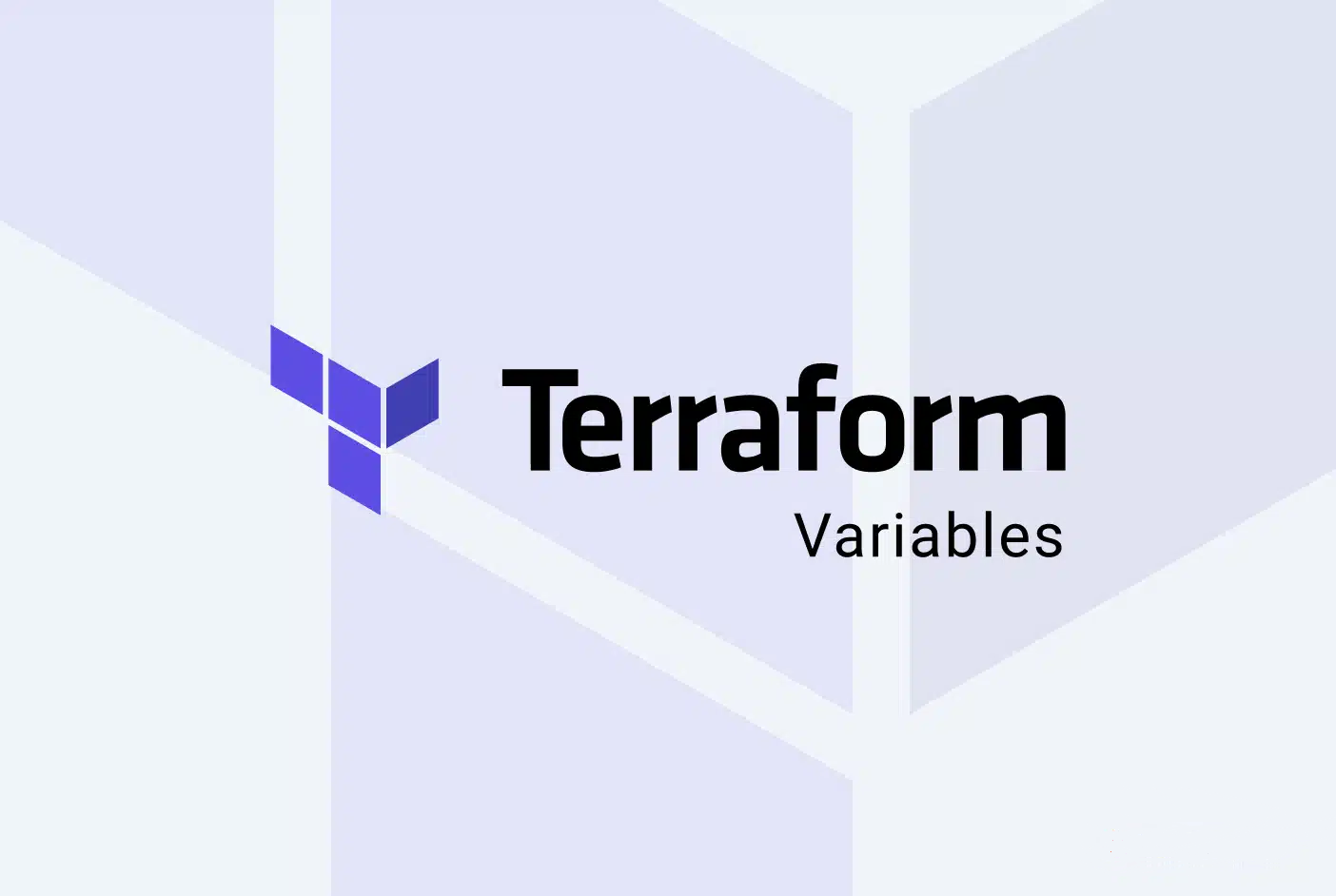
What is Terraform Variables?
Terraform variables
refer to input variables that define parameters without hardcoding them into the configuration. For example, you could create variables that let users specify the number and type of Amazon Web Services EC2 instances they want to provision with a Terraform module.
In other words, Terraform, variables
play a crucial role in customizing your infrastructure configurations. They allow you to tailor aspects of your setup without directly modifying the main configuration files.
We can create a variables.tf
file which will hold all the variables. We declare a Terraform variable using the variable
block. The label after the variable
keyword is the variable’s name, which should be unique across all the variables in the same module:
variable "image_id" {
type = string
}
A Terraform variable block supports several optional arguments. For instance, the default
argument sets a variable’s default value. And the type
indicates what value types are accepted for the variable. In addition, the description
specifies the variable documentation.
As an example, the following code shows a region
variable of the type string
, a default
value of us-west-2
, and a description
:
variable "region" {
description = "The AWS region where resources will be created"
type = string
default = "us-west-2"
}
For more information about optional arguments, check out the Official Terraform Documentation.
Benefits of using Terraform variables
There are two main benefits of using Terraform variables: -
Reusability: By utilizing variables in your IaC configuration, you can avoid hardcoding values such as instance type or region names directly. This flexible approach allows you to utilize the same configuration files across multiple environments (i.e., development, testing, and production) or with different settings simply by changing the assigned values to the variable.
Security: To minimize the risk of exposing sensitive data, it’s recommended that you do not hardcode specific values, such as API keys and passwords, directly into your configurations. Instead, you should treat them as variables that can be dynamically provided when needed.
Accessing the Variables
After we define the Terraform variables
in our Terraform module, there are several ways to assign values to these variables, including the following:
Default values: As you’ve already seen,
default
values can be used to assign default values to the variable in itsvariable
block declaration.Terraform modules: Terraform variables can be referenced using this format:
var.<name>
.Variable interpolation: This method consists of using the variable name as part of another string.
Variable substitution in the CLI: This involves setting the value of the Terraform variable as part of the command when running Terraform commands.
Terraform configuration files: With this method, you set the variable values in Terraform configuration files. These files are typically called
terraform.tfvars
or*.auto.tfvars
.
Variable Interpolation
In Terraform, we can perform variable interpolation (also referred to as string interpolation, variable substitution, or variable expansion). Variable interpolation allows you to include a variable value within another string, ultimately, helping you build dynamic configuration values.
As an example, the following code shows a variable called filename
and its usage as a string interpolation in a resource called index
of type local_file
, which creates a file in the operating system:
variable "filename" {
description = "The name of the file to be created"
type = string
default = "index"
}
resource "local_file" "index" {
filename = "${var.filename}.txt"
content = "foo!"
}
This code creates a local file with the name index.txt
. The filename
property in the local_file
resource is interpolated with the value of the defined variable filename
. The filename
variable is accessed in the string using the interpolation format: ${var.<variableName>}
.
Variable Substitution in the CLI
In addition to using variable interpolation to include a variable value within another string, you can also override the values of the variables directly from the command line using the -var
flag when running Terraform commands. This is commonly used in scripting and automation tasks.
For instance, in the following code block, you override the value of the variable instance_type
to be equal to t2.medium
when running terraform apply
to apply the IaC changes:
terraform apply -var 'instance_type=t2.medium'
Terraform Configuration Files
In Terraform, you have the option to utilize configuration files to define variable values to your IaC. Say you have a main.tf
file that defines an AWS EC2
configuration like this:
provider "aws" {
region = var.region
}
resource "aws_instance" "example" {
ami = var.ami
instance_type = var.instance_type
}
Here, the configuration uses var.region
, var.ami
, and var.instance_type
variables to set the region, AMI, and instance type.
In addition, the configuration values can be provided in another configuration file named terraform.tfvars
or any file ending with .auto.tfvars
. Here’s an example of a terraform.tfvars
file:
region = "us-west-2"
ami = "ami-0c94855ba95c574c8"
instance_type = "t2.micro"
When you run the terraform apply
command to apply the changes to your infrastructure, Terraform automatically loads any files with the names terraform.tfvars
or *.auto.tfvars
and populates the corresponding variables.
Moreover, you can manually load variable files with different names using the -var-file
flag when running terraform apply
or terraform plan
:
terraform apply -var-file="variables.tfvars"
Creating local file using variables.tf
Step 1: Create a variable.tf, and add details regarding file creation by providing the new file complete path.
sudo vim variables.tf
variable "filename" {
default = "/home/ubuntu/terraform/terraform-variables/demo-var.txt"
}
variable "content" {
default = "This is coming from a variable which was updated"
}
Step 2: Create a main.tf file where we will access the variables that have defined before.
resource "local_file" "devops" {
filename = var.filename
content = var.content
}
Step 3: Initializes a new terraform working directory.
terraform init
Step 4: Generates an execution plan by using terraform plan
command that shows what actions Terraform will take to reach the desired state specified in the configuration file.
Step 5: Once terraform apply
command runs, Terraform will create a file called demo-var.txt in the local directory with the content specified in the content variable that we had mentioned before.
Step 6: Check whether the file is created in a specified folder using ls
command.
terraform refresh
The terraform refresh
command in Terraform compares the current state of the infrastructure with the state defined in the Terraform configuration files. It updates the Terraform state file to reflect any changes made outside of Terraform, ensuring accurate tracking of resource status and attributes.
In short, we can say this command is used to refresh the state of your configuration file and reloads the variables.
Data Types in Terraform
Terraform supports various data types, such as maps, lists, sets, and objects, allowing us to handle complex data structures within our configurations. Let’s explore some of these data types and demonstrate their usage.
1. Map
In Terraform, a map
is a data structure that allows you to store and manage a collection of key-value pairs. Maps
are often used to define input variables, output values, and resource configurations.
Step 1: Inside variable.tf, Create a variable type map
and put content inside it.
variable "filename" {
default = "/home/ubuntu/terraform/terraform-variables/terra-dt/demo-map1.txt"
}
variable "content" {
default = "This is coming from a variable which was updated"
}
variable "file_contents" {
type = map
default = {
"statement1" = "this is cool stmt1"
"statement2" = "this is cooler stmt2"
}
}
Step 2: Now from the main.tf access the variables that have been created before inside variable.tf file.
resource "local_file" "devops" {
filename = var.filename
content = var.file_contents["statement1"]
}
resource "local_file" "devops_var" {
filename = "/home/ubuntu/terraform/terraform-variables/terra-dt/demo-map2.txt"
content = var.file_contents["statement2"]
}
In this example, we set the content parameter of the local_file
resource using the file_contents
variable. We use dot notation and the syntax var.file_contents[“content1”]
to refer to the value associated with the key “content1.”
Step 3: Run the commands terraform init, terraform plan and terraform apply.
Step 4: After successfully commands run check now the demo-map1.txt and demo-map2.txt files content.
2. List
In Terraform, a list
is a data structure that allows you to store and manage a collection of values. Lists
are often used to define input variables, output values, and resource configurations.
Step 1: Inside variables.tf, Create a variable of type list
to pass the list of files.
variable "file_list"{
type = list
default = ["/home/ubuntu/terraform/terraform-variables/terra-dt/file1.txt","/home/ubuntu/terraform/terraform-variables/terra-dt/file2.txt"]
}
Step 2: Now from the main.tf access the variables that have been created before inside variable.tf file.
resource "local_file" "devops" {
filename = var.file_list[0]
content = var.file_contents["statement1"]
}
resource "local_file" "devops_var" {
filename = var.file_list[1]
content = var.file_contents["statement2"]
}
Step 3: Run the commands terraform init, terraform plan and terraform apply.
Step 4: After successfully commands run check now the file1 and file2 content.
3. Object
Unlike a map and list, an object
is a structural type that is used to store structured data with named attributes and each has its type.
Objects
are typically used to group related attributes together and provide a structured way to organize and manage data within a configuration. Object data types in Terraform are represented using maps, which are key-value pairs.
Step 1: Inside variables.tf, Create a variable of type object
to pass the list of files.
variable "aws_ec2_object"{
type = object({
name = string
instances = number
keys = list(string)
ami = string
})
default = {
name = "test_ec2-instance"
instances = 4
keys = ["key1.pem", "key2.pem"]
ami = "ami-0c7217cdde317cfec"
}
}
Step 2: Now, create an output file to view the output of a specific value as per our wish. And add it to main.tf file.
resource "local_file" "devops" {
filename = var.file_list[0]
content = var.file_contents["statement1"]
}
resource "local_file" "devops_var" {
filename = var.file_list[1]
content = var.file_contents["statement2"]
}
output "aws-ec2-instance" {
value = var.aws_ec2_object.ami
}
Step 3: Run the commands terraform init, terraform plan and terraform apply.
Step 4: You can see the output of aws-ec2-instance value.
4. Set
In Terraform, a set
is a data structure that allows you to store and manage a collection of unique values. Sets
are often used to define input variables and to manage resource configurations that require unique sets of values.
Step 1: Inside variables.tf, Create a variable for the type set
to pass the string of security groups.
variable "security_group"{
type = set(string)
default = ["sg-131284", "sg-123184"]
}
Step 2: Create a output file to check the security group, add it in main.tf file.
output "security_group"{
value = var.security_group
}
Step 3: Run the commands terraform init, terraform plan and terraform apply.
Step 4: You can see the output of security_group value.
Conclusion
In Conclusion, Terraform variables
provide a powerful mechanism for parameterizing infrastructure configurations, enabling flexibility, reusability, and maintainability in infrastructure as code (IaC) projects. By defining variables
, users can dynamically customize deployments, manage environment-specific settings, and streamline collaboration.
In Terraform, data types
are fundamental building blocks used to define and manipulate values within infrastructure configurations. Some common data types in Terraform include: Map, Object, Set, List, String, Number, Boolean.
In this blog, we have seen how to create variables and access them. Also we have seen some of the terraform data types in detailed.
Hope you find it helpful🤞 So I encourage you to try this on your own and let me know in the comment section👇 about your learning experience.✨
👆The information presented above is based on my interpretation. Suggestions are always welcome.😊
~Smriti Sharma✌
Subscribe to my newsletter
Read articles from Smriti Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
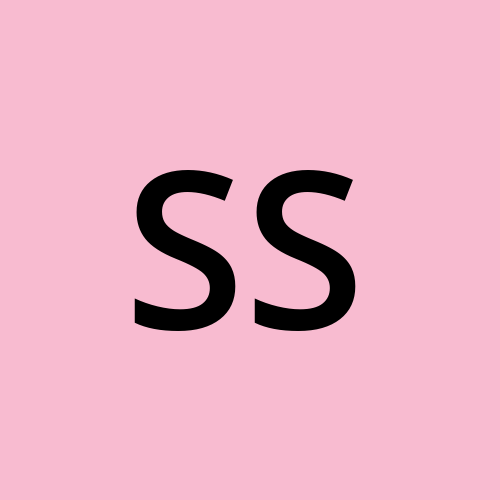