Laravel Magic
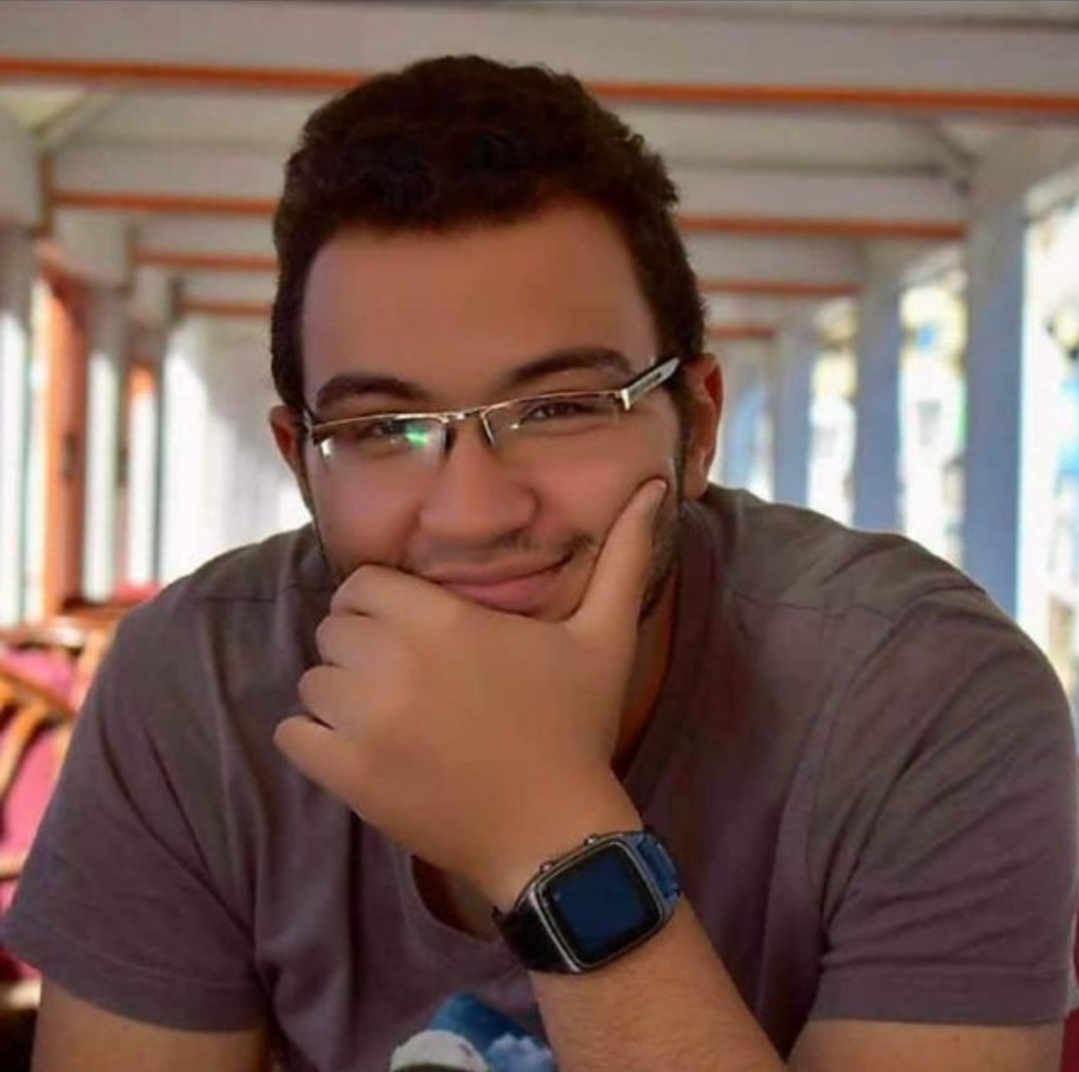
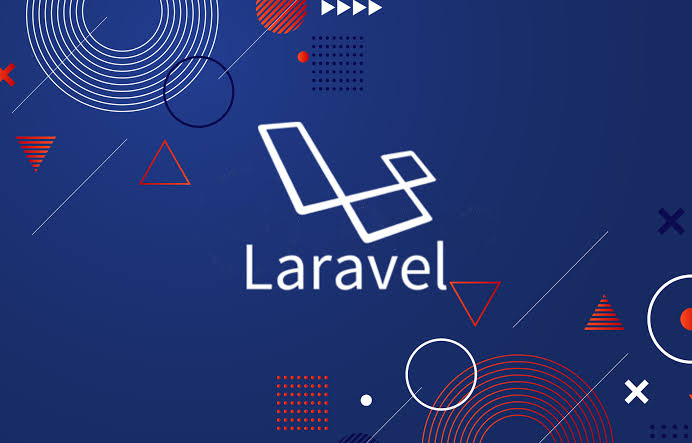
If you are new to Laravel or any framework, You may be confused and sometimes when you start learning you may find some built-in classes or functions that you don't know where it comes from and sometimes you are saying It's a magic trick.
So, I will give you one Example of Laravel Magic.
In the following Image I created a simple command that count the user in Database and you can find that we used DB Class from Illuminate\Support\Facades
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
use Illuminate\Support\Facades\DB;
class MagicTrick extends Command
{
protected $signature = 'magic:run';
protected $description = 'Show a magic trick';
public function handle()
{
$usersCount = DB::table('users')->count();
$this->info("Users count: $usersCount");
}
}
And when we run it.
php artisan magic:run
We will find that.
Users count: 1
Till now its fine but what about if we removed this line use Illuminate\Support\Facades\DB;
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
class MagicTrick extends Command
{
protected $signature = 'magic:run';
protected $description = 'Show a magic trick';
public function handle()
{
$usersCount = \DB::table('users')->count();
$this->info("Users count: $usersCount");
}
}
We will get the same result.
So How it works?
Laravel behind the scene uses class alias.
Let Me explain it in a simple way. For Example create a simple Enum Class
<?php
namespace App\Enums;
enum Gender: string
{
case MALE = 'M';
case FEMALE = 'F';
}
Then get back to the command
<?php
namespace App\Console\Commands;
use App\Enums\Gender;
use Illuminate\Console\Command;
class MagicTrick extends Command
{
protected $signature = 'magic:run';
protected $description = 'Show a magic trick';
public function handle()
{
$this->info('Male Enum value: ' . Gender::MALE->value);
}
}
Then when we run we will get Male Enum value: M.
What will happen if we remove use App\Enums\Gender;
We will get an error Class "App\Console\Commands\Gender" not found
So if we want to use a magic here simply we can use a simple PHP function class_alias()
class_alias('App\Enums\Gender', 'Gender');
You can simply put the above snippet into your service provider to use the alias anywhere in your project
Your final code in command will be:
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
class MagicTrick extends Command
{
protected $signature = 'magic:run';
protected $description = 'Show a magic trick';
public function handle()
{
$this->info('Male Enum value: ' . \Gender::MALE->value);
}
}
Finally you can check the following link of Mohamed Said to learn more about Laravel magic tricks
Laravel Magic Tricks
Subscribe to my newsletter
Read articles from Abdelrhman Mgahed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
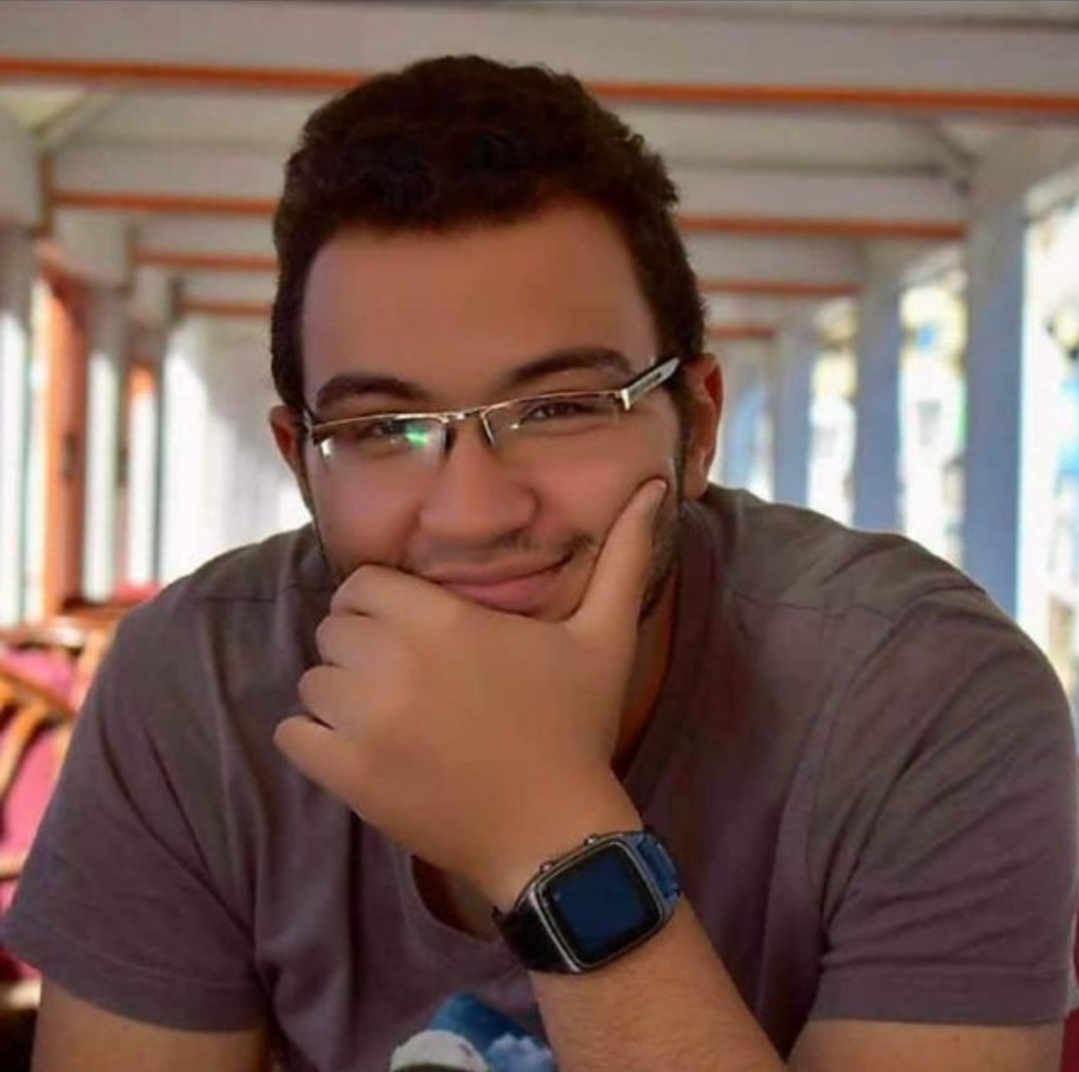
Abdelrhman Mgahed
Abdelrhman Mgahed
I'm a full-stack developer