Day 15: Mastering DevOps with Python Libraries
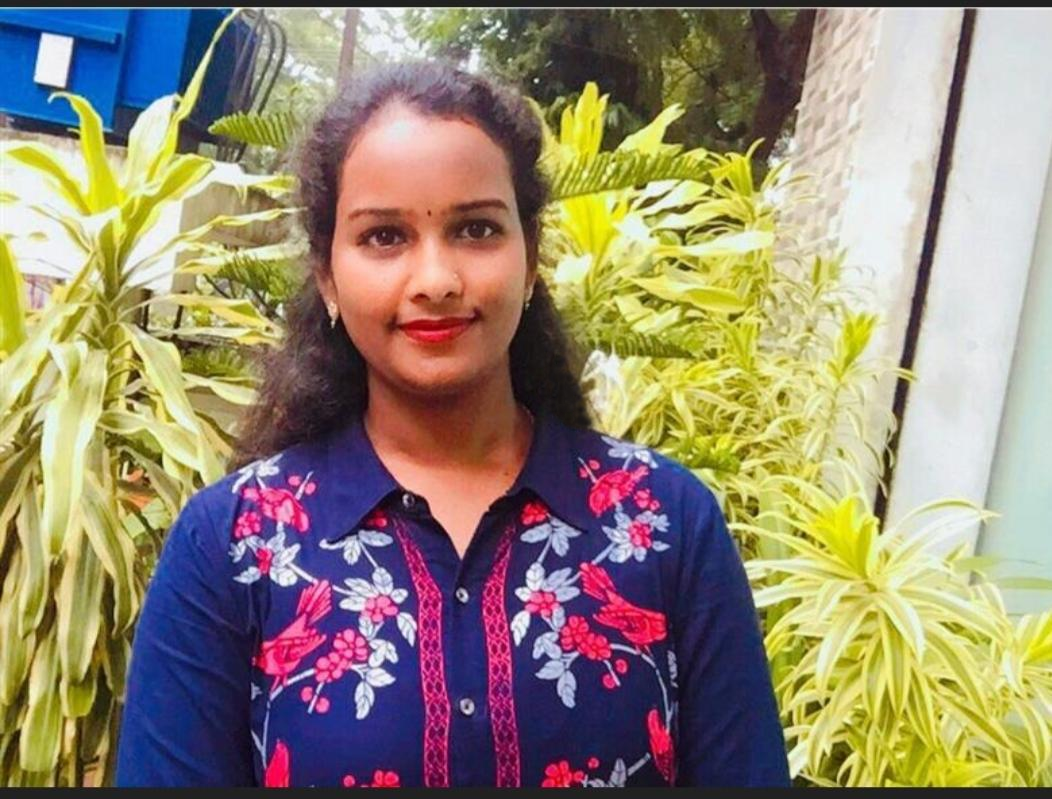
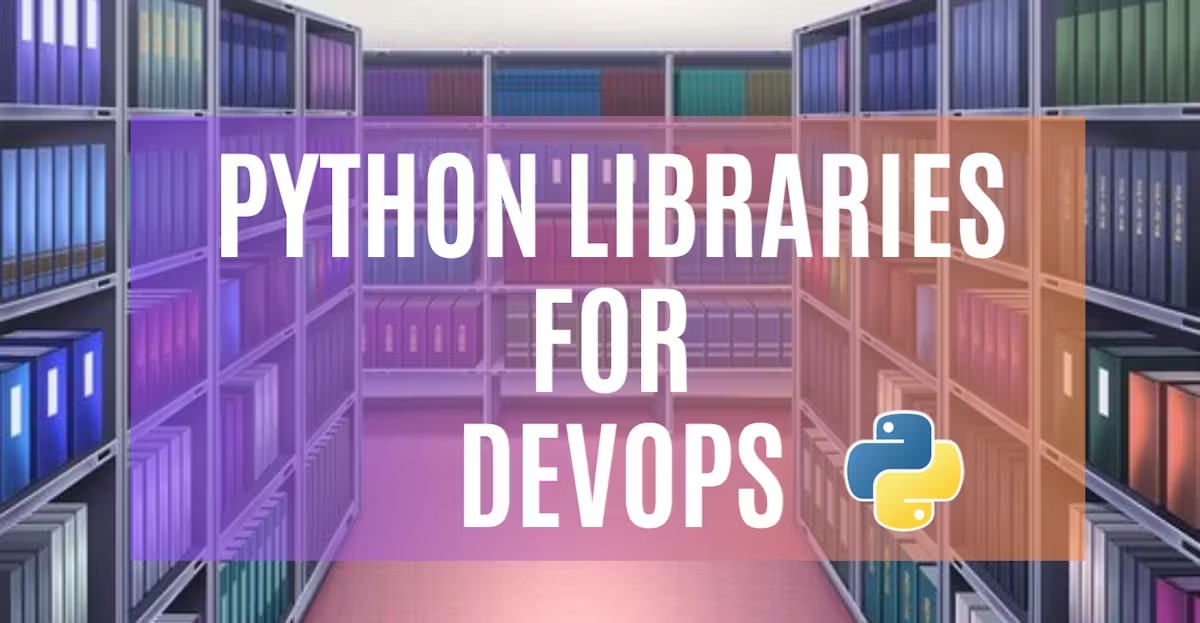
Python Libraries: Empowering DevOps Excellence
Python's strength lies not only in its simplicity and readability but also in its vast array of libraries catering to diverse needs across various domains. In the realm of DevOps, where automation, configuration management, and infrastructure orchestration are paramount, Python libraries serve as indispensable tools for engineers. Let's explore some key Python libraries that empower DevOps excellence:
os and sys: The
os
andsys
libraries provide essential functions for interacting with the operating system, managing files and directories, and accessing system-level information. DevOps engineers leverage these libraries to automate system administration tasks, execute shell commands, and handle file operations efficiently.json: The
json
library facilitates working with JSON (JavaScript Object Notation) data, a ubiquitous format for exchanging information. DevOps practitioners use this library to parse JSON configurations, manipulate data structures, and exchange data with APIs seamlessly.yaml: While Python natively supports JSON, YAML (YAML Ain't Markup Language) is another popular format for configuration files due to its human-readable syntax. The
pyyaml
library allows DevOps engineers to parse YAML files, convert YAML to JSON, and vice versa, enabling smoother integration with infrastructure-as-code tools and configuration management systems.paramiko and fabric: For managing remote servers and automating SSH operations, the
paramiko
andfabric
libraries come to the rescue. DevOps teams utilize these libraries to deploy code, execute commands on remote hosts, and automate routine tasks across distributed environments.requests: The
requests
library simplifies HTTP requests, making it effortless to interact with web services and APIs. DevOps engineers leverage this library to automate interactions with cloud platforms, fetch configuration data from external sources, and orchestrate deployments seamlessly.docker and kubernetes: As containerization and container orchestration gain prominence in modern DevOps practices, libraries like
docker
andkubernetes
provide Python bindings for managing Docker containers and Kubernetes clusters programmatically. DevOps teams utilize these libraries to automate container deployment, scaling, and management tasks, streamlining the container lifecycle.pytest: For automated testing and continuous integration (CI) pipelines, the
pytest
library offers a robust testing framework with extensive capabilities for writing and executing test cases. DevOps engineers leverage pytest to ensure code quality, validate infrastructure changes, and maintain reliability across deployments.
In essence, Python libraries serve as indispensable allies for DevOps engineers, empowering them to automate tasks, manage configurations, and orchestrate infrastructure with ease. By harnessing the power of Python libraries, DevOps teams can enhance efficiency, accelerate workflows, and drive innovation in their organizations.
1. Creating and Writing to JSON:
import json
# Create a dictionary to represent our data
data = {
"aws": "ec2",
"azure": "VM",
"gcp": "compute engine"
}
# Write data to a JSON file
with open('services.json', 'w') as json_file:
json.dump(data, json_file)
Explanation:
We import the
json
module, which allows us to work with JSON data in Python.We create a Python dictionary called
data
, which contains information about different cloud service providers and the services they offer.We open a file named
services.json
in write mode ('w'
) using a context manager (with
statement).We use the
json.dump()
function to write the contents of thedata
dictionary into the JSON file.
2. Reading JSON and Printing Cloud Service Providers:
import json
# Read JSON file and print service names of every cloud service provider
with open('services.json', 'r') as json_file:
services = json.load(json_file)
for provider, service in services.items():
print(f"{provider} : {service}")
Explanation:
We import the
json
module again.We open the
services.json
file in read mode ('r'
) using a context manager.We use the
json.load()
function to load the JSON data from the file into a Python dictionary calledservices
.We iterate over the items in the
services
dictionary, which represents each cloud service provider and the corresponding service they offer, and print them out.
3. Reading YAML and Converting to JSON:
import yaml
import json
# Read YAML file and convert to JSON
with open('services.yaml', 'r') as yaml_file:
yaml_data = yaml.safe_load(yaml_file)
json_data = json.dumps(yaml_data, indent=2)
print(json_data)
Explanation:
We import the
yaml
andjson
modules.We open the
services.yaml
file in read mode using a context manager.We use the
yaml.safe
_load()
function to load the YAML data from the file into a Python dictionary calledyaml_data
.We use the
json.dumps()
function to convert theyaml_data
dictionary into a JSON-formatted string with indentation (indent=2
).Finally, we print the JSON-formatted data.
These showcase how to create, read, and manipulate JSON and YAML files in Python using respective libraries, making it easier to work with configuration data and automate tasks in DevOps workflows.
Conclusion:
As DevOps engineers, proficiency in handling various file formats like JSON and YAML is crucial. Python, with its rich assortment of libraries such as json
and pyyaml
, empowers DevOps practitioners to efficiently parse, manipulate, and manage configuration files within their workflows. By leveraging Python's simplicity and versatility, DevOps teams can streamline processes, automate tasks, and maintain robust infrastructure setups effectively. So, whether you're orchestrating deployments, configuring cloud environments, or fine-tuning system settings, Python libraries have got your back in simplifying your DevOps journey.
Subscribe to my newsletter
Read articles from SWATHI PUNREDDY directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
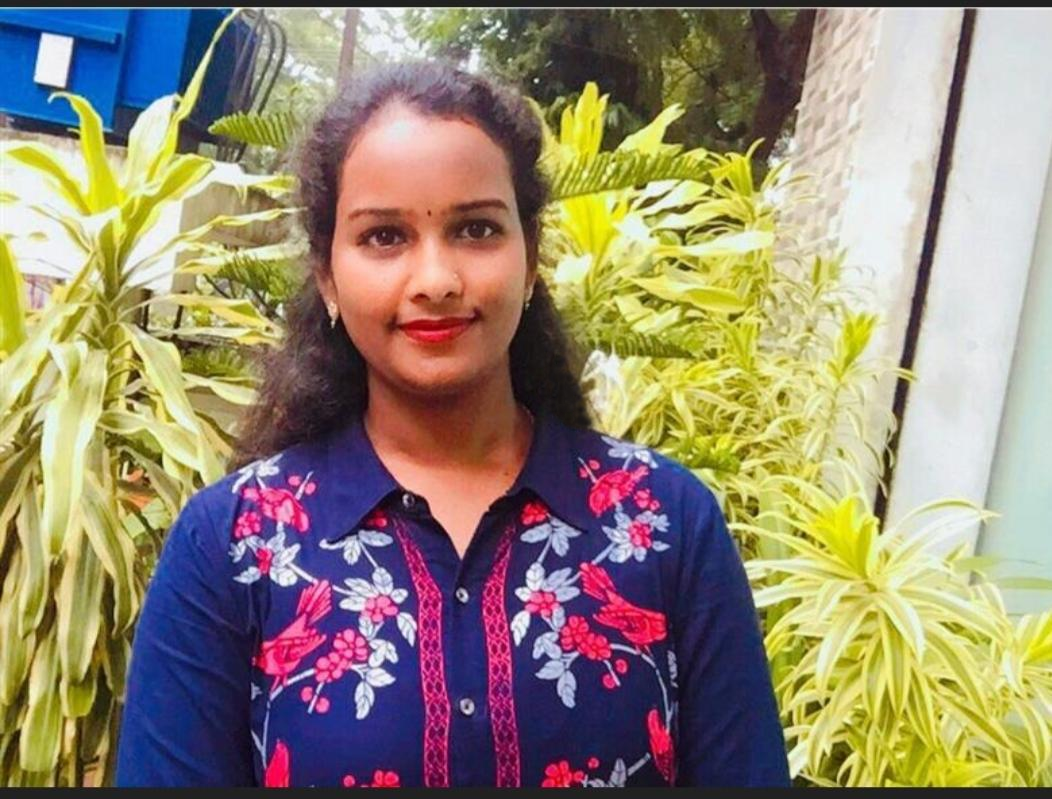