Organize your files using python, shell and bat scripts ✨
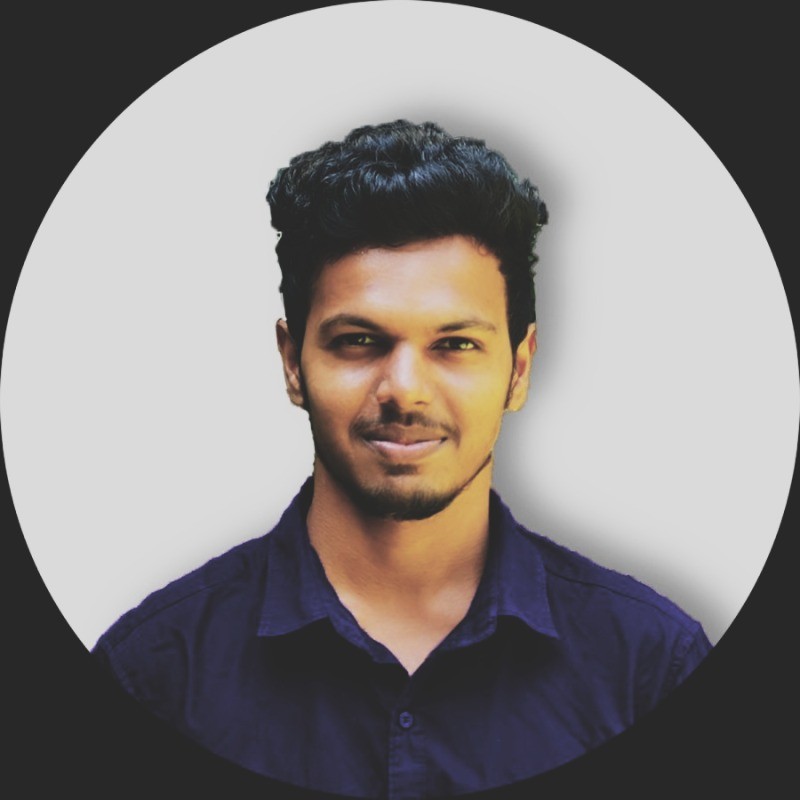
2 min read
Table of contents
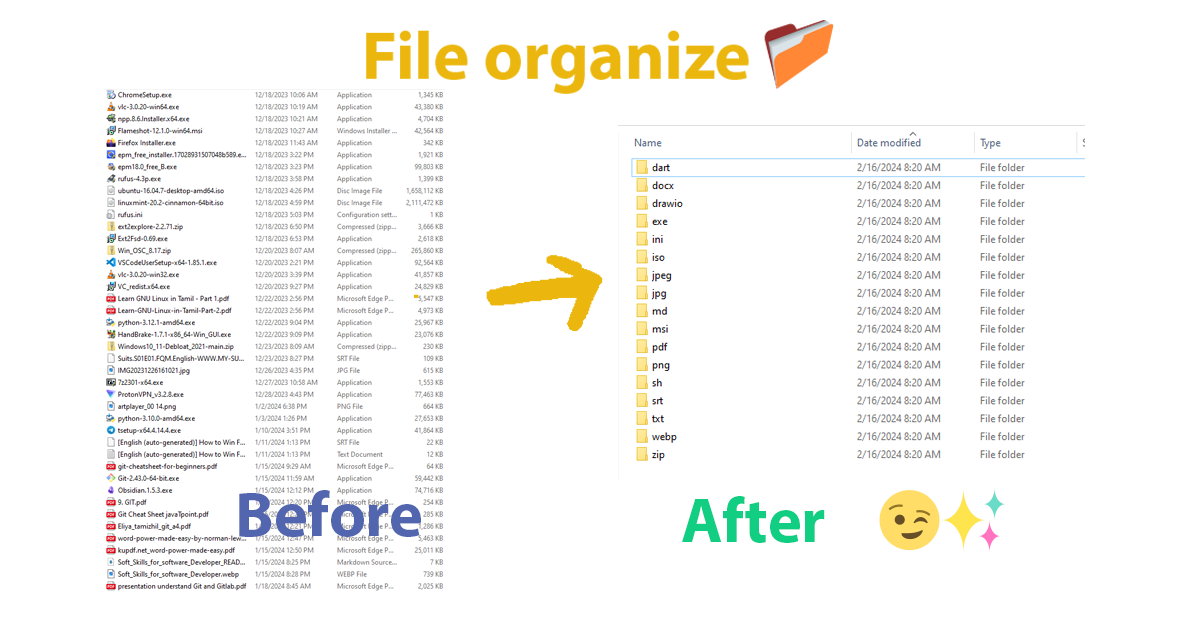
These scripts will automates file organization by categorizing files based on their extensions into individual directories within a parent directory.
Python (.py)
import os
import shutil
def fileOrganise(directory):
# Iterate over files in the directory
for element in os.listdir(directory):
# Get the full path of the element
full_path = os.path.join(directory, element)
# Check if it's a file
if os.path.isfile(full_path):
# Split the file name based on the '.' delimiter and extract the last part
parts = element.split(".")
file_extension = parts[-1]
# Create directory if not exists
destDir = os.path.join(directory, file_extension)
os.makedirs(destDir, exist_ok=True)
# Move the file to the corresponding directory
shutil.move(full_path, destDir)
if __name__ == '__main__':
directory = os.getcwd()
# directory = r"Enter your path to run this script"
# example,
# directory = r"C:\Users\navin\Downloads\New folder"
fileOrganise(directory)
Shell (.sh)
#!/bin/bash
# Function to organize files in the directory based on their extensions
fileOrganise() {
local directory="$1"
# Iterate over files in the directory
for element in "$directory"/*; do
# Check if it's a file
if [ -f "$element" ]; then
# Get the file extension
file_extension="${element##*.}"
# Create directory if not exists
destDir="$directory/$file_extension"
mkdir -p "$destDir"
# Move the file to the corresponding directory
mv "$element" "$destDir"
fi
done
}
# Main script
main() {
local parent_dir="$(pwd)" # Use current directory as the parent directory
# parent_dir="Enter your path to run this script"
# example,
# parent_dir="/c/Users/navin/Downloads/New folder"
# Call the function to organize files
fileOrganise "$parent_dir"
echo "Files organized successfully in $parent_dir"
}
# Call the main function
main
Batch (.bat)
@echo off
setlocal enabledelayedexpansion
set "directory=%~1"
REM set "directory=(Enter your path to run this script)"
REM example,
REM set "directory=C:\Users\navin\Downloads\New folder"
REM Iterate over files in the directory
for %%i in ("%directory%\*") do (
REM Check if it's a file
if exist "%%i" (
REM Get the file extension
for /f "tokens=*" %%j in ("%%~xi") do (
set "file_extension=%%j"
REM Remove leading dots from file extension
set "file_extension=!file_extension:~1!"
echo "Processing file: %%i, Extension: !file_extension!"
REM Create directory if not exists
mkdir "%directory%\!file_extension!" 2>nul
REM Move the file to the corresponding directory
move "%%i" "%directory%\!file_extension!" >nul
)
)
)
echo Files organized successfully in %directory%
:end
Conclusion
These scripts will organize your files with just one click. You can find additional code in my GitHub repository.
If you have any suggestions, improvements, feel free to comment below
Cheers! 🎉
0
Subscribe to my newsletter
Read articles from navinkumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
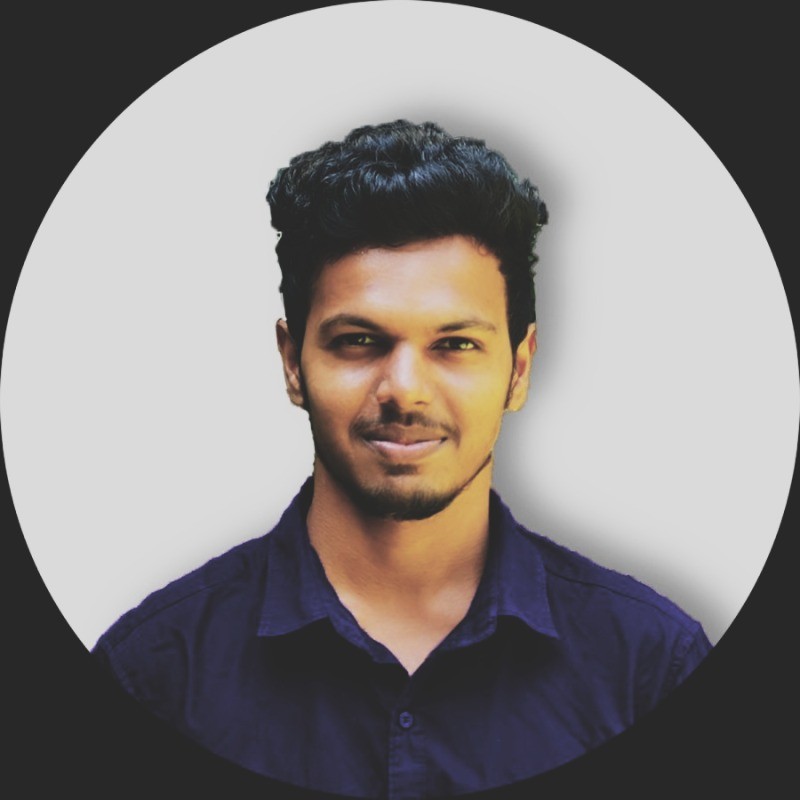
navinkumar
navinkumar
A passionate data engineer skilled in ETL, Data warehousing, SQL, Python, Docker & Kubernetes and more. Follow my blog for tech insights!