Mastering Dart: A Deep Dive into 'static' Keyword
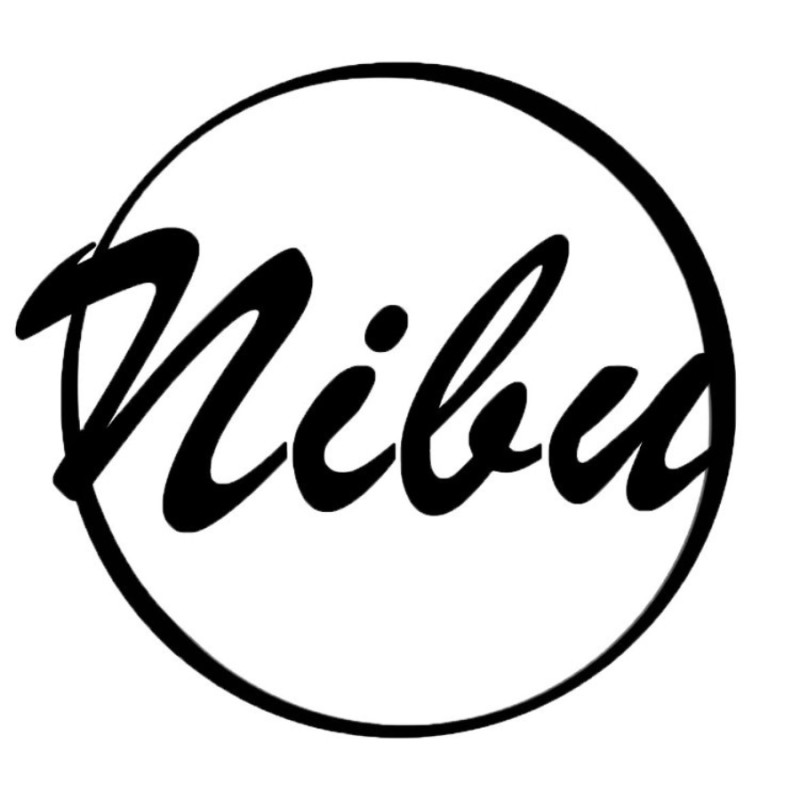
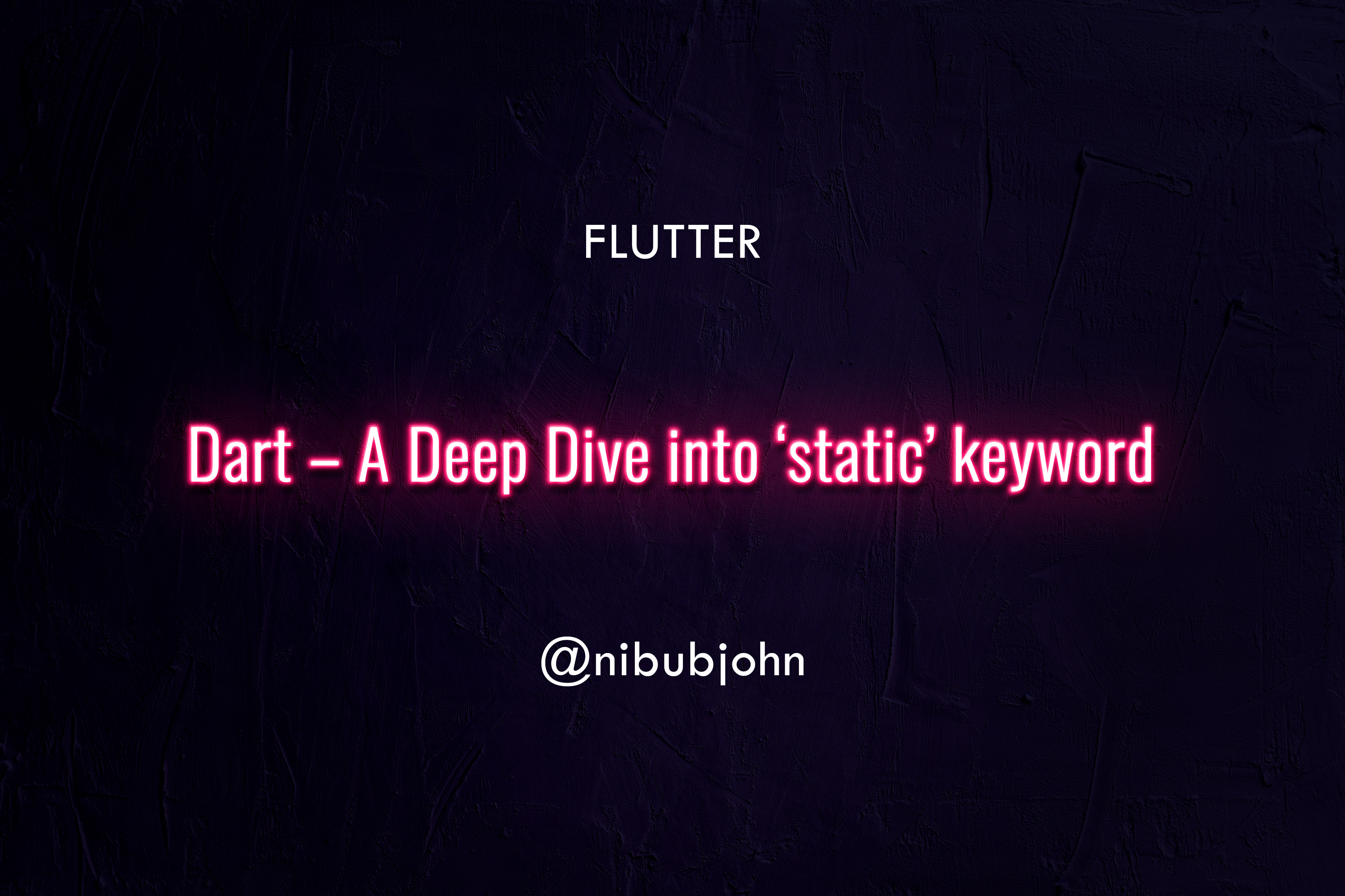
Introduction
Welcome to our comprehensive guide on mastering Dart programming! In this blog post, we're about to dive deeply into one of Dart's essential keywords: 'static'. Don't worry if you're new to programming or if you find these concepts a bit intimidating – we'll break them down into simple, easy-to-understand explanations with plenty of examples to help you grasp them quickly.
Harnessing the Power of 'static' keyword
The static keyword in Dart is essential for defining class-level variables and methods, accessible without class instantiation. It eliminates ambiguity when attributes or methods are shared among class instances and allows direct access from the class, enhancing code organization and execution efficiency.
Static variables
The static variables belong to the class instead of a specific instance. A static variable is common to all instances of a class: this means only a single copy of the static variable is shared among all the instances of a class. The memory allocation for static variables happens only once in the class area at the time of class loading.
Syntax: Static variables can be declared using the static keyword followed by data type then the variable name
class ClassName {
static [DataType] [variableName];
}
The static variable can be accessed directly from the class without needing an instance.
void main(){
[Classname].[staticVariable];
}
Static Methods
The static method belongs to a class instead of class instances. A static method is only allowed to access the static variables of class and can invoke only static methods of the class.
Syntax: A static method is defined by using the keyword static
, followed by the return type, and then the method name.
static returnType methodName()
{
// Statement(s)
}
The static methods can be accessed directly from the class without needing an instance.
void main(){
[ClassName].[staticMethod]();
}
'static' keyword is your shortcut to instant access, bypassing the need for class instantiation and bringing order to the chaos.
Why is this useful? Let's see!
Examples
Example 1
class Counter {
static int count = 0; // Static variable to track count
Counter() {
count++; // Increment count each time a Counter instance is created
}
static void resetCount() {
count = 0; // Reset count to 0
}
}
void main() {
Counter(); // Creating first instance
Counter(); // Creating second instance
Counter(); // Creating third instance
print(Counter.count); // Output: 3
Counter.resetCount(); // Resetting count
print(Counter.count); // Output: 0
}
Output:
3
0
Notice the change? Instead of each Counter instance maintaining its own count, a single count variable is shared among all instances. It's akin to having a global variable. Interestingly, these variables are not bound to the class; rather, they are inherited by their subclasses.
This means that any modifications made to the static variable count
are reflected across all instances of the Counter class, providing a centralized way to manage shared data. It's a powerful feature that enhances code clarity and efficiency by avoiding unnecessary duplication and ensuring consistent behavior across the application.
Example 2
class Player {
static int totalCoins = 0; // Static variable to track total coins across all players
int coinsCollected = 0; // Instance variable to track coins collected by each player
void collectCoin() {
coinsCollected++;
// Increment totalCoins whenever a coin is collected by any player
totalCoins++;
}
}
void main() {
var player1 = Player();
player1.collectCoin(); // Player 1 collects a coin
player1.collectCoin(); // Player 1 collects another coin
var player2 = Player();
player2.collectCoin(); // Player 2 collects a coin
print('Total coins collected by all players: ${Player.totalCoins}');
print('Total coins collected by player1: ${player1.coinsCollected}');
print('Total coins collected by player2: ${player2.coinsCollected}');
}
Output:
Total coins collected by all players: 3
Total coins collected by player1: 2
Total coins collected by player2: 1
This example vividly demonstrates the utility of the static keyword. In this scenario, while each class instance maintains its own instance variable to track its values, we require a mechanism that keeps a collective account of the total coins collected across all instances, independent of any specific instance.
Exercises
Multiple Choice Questions
What does the 'static' keyword signify in Dart?
a) It refers to the current instance of a class.
b) It is used to invoke superclass methods.
c) It defines abstract methods.
d) It indicates class-level variables and methods.
When should you use the 'static' keyword in Dart?
a) To create instance-specific variables.
b) To access instance variables from outside the class.
c) To define methods that can be invoked without class instantiation.
d) To resolve ambiguity between class constructors.
Code Completion
- Complete the following code snippet by using the 'static' keyword appropriately:
class Person {
String? city;
Person(String city){
Person.city = city;
}
}
Debugging Exercise
- Identify and correct the error in the following code snippet:
class Counter {
int count = 0;
Counter() {
count++;
}
static void printCount() {
print('The count is: $count');
}
}
void main() {
Counter c1 = Counter();
Counter c2 = Counter();
Counter c3 = Counter();
c1.printCount();
}
Answers
Multiple Choice Questions
What does the 'static' keyword signify in Dart?
Answer: d) It indicates class-level variables and methods.
When should you use the 'static' keyword in Dart?
Answer: c) To define methods that can be invoked without class instantiation.
Code Completion
class Person {
static String? city; // correction
Person(String city){
Person.city = city;
}
}
Debugging Exercise
class Counter {
static int count = 0; // Debug this line
Counter() {
count++;
}
static void printCount() {
print('The count is: $count');
}
}
void main() {
Counter c1 = Counter();
Counter c2 = Counter();
Counter c3 = Counter();
Counter.printCount(); // Debug this line to ensure the count is correctly printed.
}
Takeaway
Static in Dart denotes class-level variables/methods, shared among all instances.
Facilitates defining methods without class instantiation for efficient code execution.
Enables direct access to variables/methods from the class.
Resolves ambiguity in class constructors, aids in defining utility methods.
Enhances code organization, promotes reusability at the class level.
Further Reading
Thank You!
If you have any queries please comment down below.
Happy learning, everyone!
Subscribe to my newsletter
Read articles from Nibu B John directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
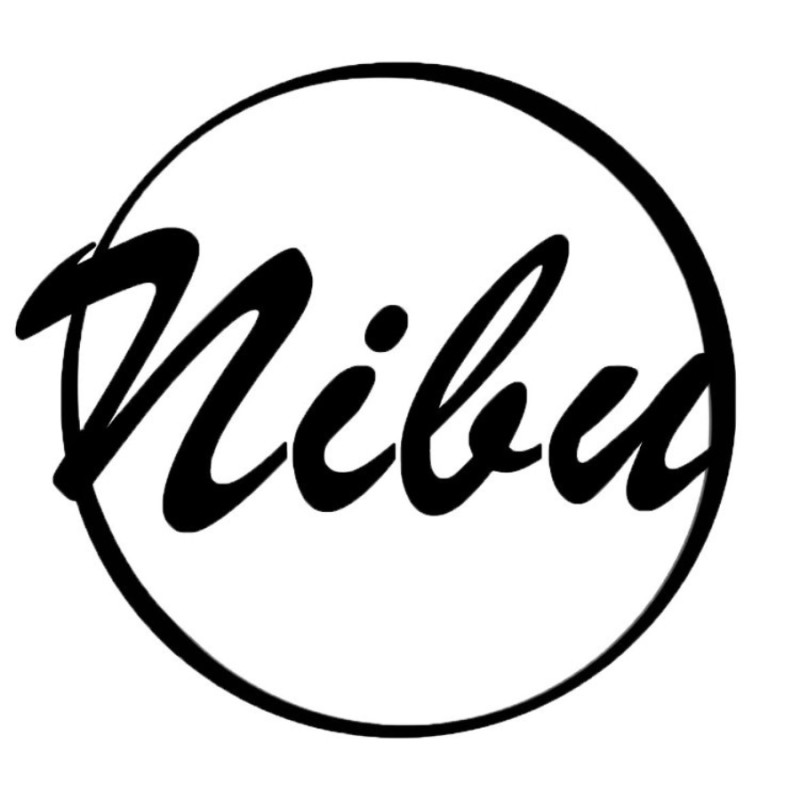
Nibu B John
Nibu B John
Full Stack Web and App Developer proficient in Selenium automation and Telegram bots.