Java Best Practices: Improve Your Java Coding Standards
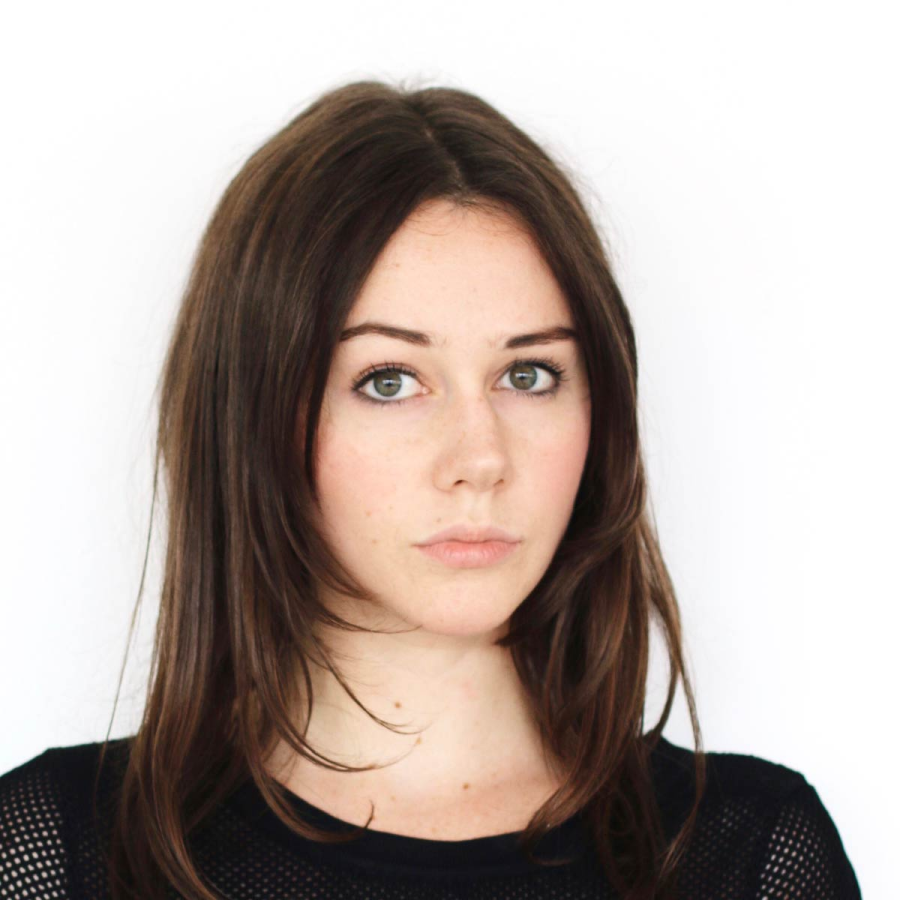
Developers follow a certain set of rules that improve software quality, maintainability, and system information readability. These rules are the best practices that help developers maintain different types of projects.
Best practices are beneficial when an app remains in use for a longer period, so the initial development team will make rules that future development teams can use as guidance on how to work on that project.
Similarly, there are a lot of common best practices to follow while working on Java development. Java is a language that has been executed successfully for a long time. It’s because of constant evolution and developers following Java best practices to make their Java projects efficient.
Let’s learn some powerful Java techniques to make Java development easier!
The popularity of Java
Java is the fifth most preferred programming language across the world. Almost 65% of respondents in modern times prefer to use Java programming for everyday software-related work.
Here are the factors that help in increasing Java's popularity:
Scalability
Garbage Collection
Cross-platform compatibilities
The active community of developers
Multi-threading functionality
And energy efficiency
Now, let’s see Java best practices that assist you in improving your Java development skills.
Java Best Practices to Improve Your Java Coding Standards
Here is the list of Java best practices that improve Java coding standards:
Use Proper Naming Conventions
A programmer writes clean code, where naming has to be appropriate so that other developers can easily find out the function of that code and make the LOC more readable.
In smaller projects, this seems a little meaningless, but it’s important in enterprise-level projects. It is necessary to give proper naming to the code to save time and make the code more understandable even by other developers.
In Java programming, naming a variable, class, and method properly is a good practice rather than naming them randomly. Here are some popular ways to name the Java components:
Packages- Package names are in lowercase
Classes- Class names should include nouns, and start with uppercase
Interfaces- Interface names follow CamelCase
Methods- Method names should include verbs with each word in capital
Variables- Variable names have mixed case convention
Follow a proper Project Structure
Project outline/structure displays the things on how to arrange different components in your project like Java source files, documentation, test files, config files, etc.
A clean Java project structure helps you to navigate and update the codebase. However, a poor Java project structure can confuse when developers are working on multiple project files together. So, keeping all relevant files as per the project structure is important.
src
├── main
│ ├── java App/Library sources
│ ├── resources Application/Library resources
│ ├── filters Resource filter files
│ └── web app Web app sources
│
└── test
├── java Test resources
├── resources Test resources
└── filters Test resource filter files
Do not write Repeating Initializations
Although languages like TypeScript encourage you to perform variable initialization at the time of declaration, it’s not always necessary in Java language. In Java, when a variable is declared, it assigns default values like false, null, and 0.
Hence, as per the Java best practice, you should be aware of the default initialization values of variables.
For instance, let’s calculate the addition of natural numbers from 1 to 1000.
Note: See only some variables are initialized:
class VariableInitializationExample {
public static void main(String[] args) {
// sum variable value is automatically set to 0
int sum;
final numberOfIterations = 1000;
// Set the loop counter to 1
for (int i = 1; i &= numberOfIterations; ++i) {
sum += i;
}
System.out.println("Sum = " + sum);
}
}
No leaving any Empty Catch Block
Using Java’s try catch block, you can handle exceptions well. The code that can throw exceptions is kept in the try block, and to handle the exception, a corresponding catch block is necessary to write.
So, if an unplanned error happens inside the try block such as file not found, by zero, etc., it will show an exception that the catch block has to deal with.
Though, in theory having an empty catch block is possible, it’s not possible in reality. It’s a bad practice and should be skipped. It’s because an empty catch block can prolong the error-solving process, and also doesn’t help provide any relevant information to the user.
Use Java libraries Intuitively
A primary reason why Java is still a popular programming language across the globe is that it supports a huge variety of coding libraries. These libraries are called Java Class Libraries. These libraries are a set of pre-written code that is an important tool for software developers.
Using these libraries, Java programmers can gather parts of Java code faster without any need to write every function manually.
It’s worth noting that using multiple libraries isn’t that good for your article. In the software programming domain, it can be harmful in the long term. The reason behind this is each library needs dedicated project resources. Other than that, using more libraries can use more system memory. So, they can interrupt the app's performance as well.
If the quality of libraries isn’t accurate, it can include buggy code as well. So, it’s good practice for developers to use libraries from trusted resources only.
Double or Float- Which to use?
Some insufficiently experienced developers use double and float data types inappropriately in the apps. They pick only a single type and do not check the system’s guidelines.
If you use precise calculations, you can choose a double rather than float.
Most of the CPUs take the same amount of time to process the operations either on double or float, but double offers higher accuracy than float type.
When precision isn’t important, a Java best practice says to use Float type instead of Double as it takes up half of the memory space.
An experienced Java developer should always remember that using accurate data type essentially improves your code’s performance.
DRY and KISS
Let’s keep it simple and know the full form of these two words:
KISS- Keep It Simple, Stupid!
DRY- Don’t Repeat Yourself!
KISS is a principle that mentions- ‘projects should be as simple as possible’. If possible, developers should avoid unnecessary complexities and should prioritize keeping the system simple and easy to understand and use.
Try to break complex methods into smaller methods as it will make the code easy to read, and find errors quickly. Smaller parts of code are easy to maintain.
Moreover, the DRY principle aims at reducing or avoiding the repetition of data or code. This principle states that each piece of logic should have one unambiguous representation in the system.
Divide the code into smaller and reusable logical units and use the code by calling it whenever needed. Do not write long methods, but divide the logic and try using the existing code with a few updates when required.
Restrict the Number of Parameters in a Method
Parameters in a method are essential. However, too many parameters in a method can create a violation of the single responsibility principle.
Here’s an example for the same:
public void processOrder(String customerName, String shippingAddress, String billingAddress,
String productName, int quantity, double price, boolean isExpressShipping) {
// Method implementation
}
Here’s how you can refactor the above situation and group the related parameters in an object to enhance the readability of the code:
public class Order {
private String customerName;
private String shippingAddress;
private String billingAddress;
private String productName;
private int quantity;
private double price;
private boolean isExpressShipping;
// Constructors, getters, and setters
// Other methods related to an order
}
public void processOrder(Order order) {
// Method implementation
}
These are some top Java best practices that Java developers should use to make high-quality Java software.
Concluding Verdict
Writing Java clean code is an essential factor for producing high-quality Java software.
Here, you have learned some popular best practices that will help you improve your Java coding standards. However, it’s essential to know that there are many other Java best practices other than the ones mentioned in this article. You can find them in the future on our site. Till then, happy reading!
Subscribe to my newsletter
Read articles from Jenifer directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
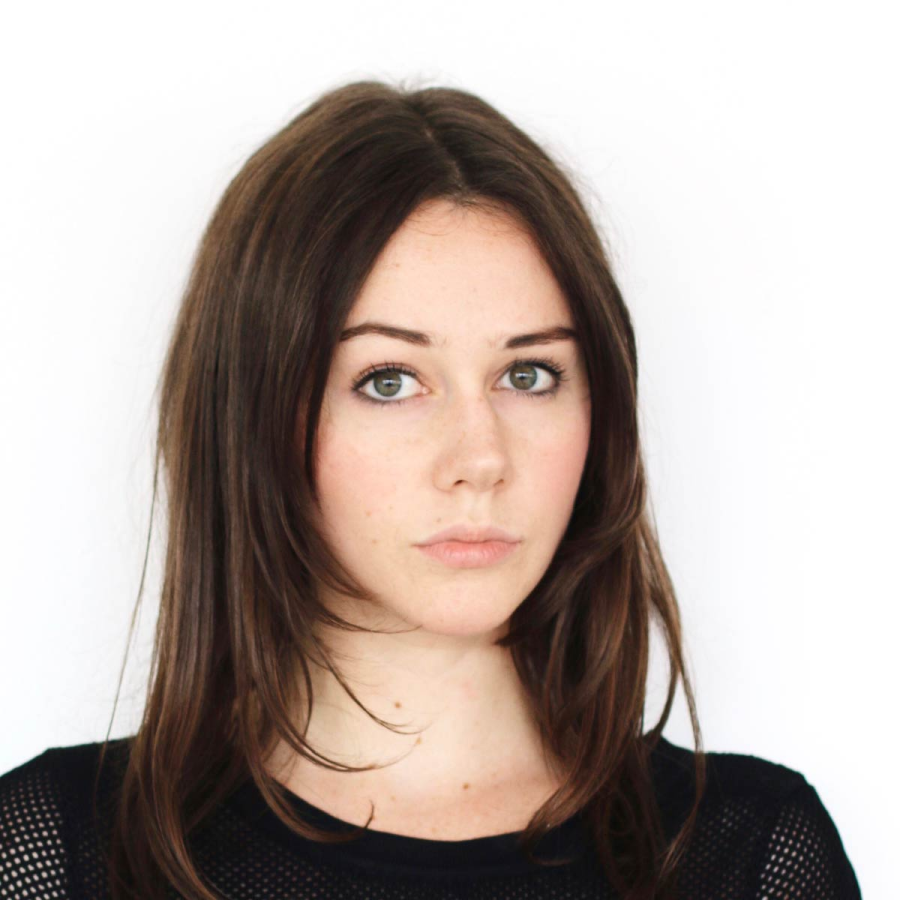