Image widget and Attributes

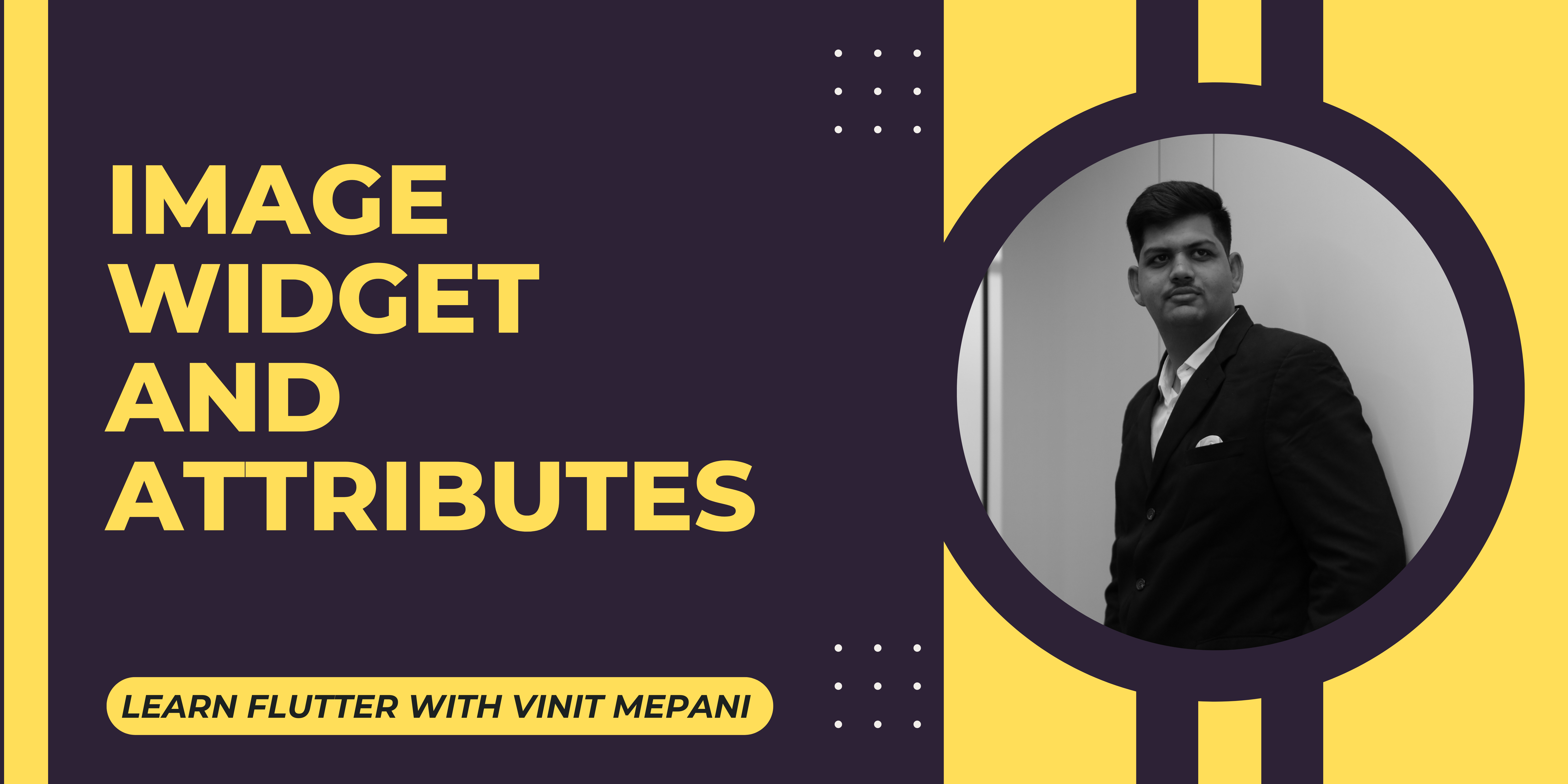
In Flutter, creating visually appealing and dynamic user interfaces is a breeze, thanks to its extensive widget library. One such crucial component is the Image widget, which enables the display of images in your application. In this blog post, we'll explore the Image widget and its attributes, allowing you to harness its capabilities to enhance the visual aspects of your Flutter app.
Image Widget Overview: The Image widget in Flutter provides a flexible and efficient way to display images in various formats, including JPEG, PNG, GIF, and more. Let's delve into the key attributes and properties that make the Image widget a versatile tool for handling images.
Attributes and Properties:
image:
- The image attribute is where you specify the image to be displayed. It can be an asset, a network image, or any other valid ImageProvider in Flutter.
Example:
Image(
image: AssetImage('assets/my_image.png'),
)
width and height:
- These attributes define the dimensions of the image. You can set them to specific values or use constraints like BoxFit to determine how the image should be sized within the available space.
Example:
Image(
image: AssetImage('assets/my_image.png'),
width: 200,
height: 150,
fit: BoxFit.cover,
)
fit:
- The fit property determines how the image should be fitted into the given space. Options include BoxFit.contain, BoxFit.cover, BoxFit.fill, and more.
Example:
Image(
image: AssetImage('assets/my_image.png'),
fit: BoxFit.cover,
)
alignment:
- Use the alignment property to specify the alignment of the image within its container. It takes a AlignmentGeometry value.
Example:
Image(
image: AssetImage('assets/my_image.png'),
alignment: Alignment.center,
)
repeat:
- For ImageRepeat property, you can specify how the image should repeat in case it doesn't cover the entire space.
Example:
Image(
image: AssetImage('assets/my_pattern.png'),
repeat: ImageRepeat.repeat,
)
here's a simple example:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Image Widget Example'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
'Flutter Image Widget Example',
style: TextStyle(fontSize: 18),
),
SizedBox(height: 20),
Image.asset(
'assets/flutter_logo.png',
width: 150,
height: 150,
fit: BoxFit.contain,
),
SizedBox(height: 20),
Image.network(
'https://example.com/my_image.jpg',
width: 200,
height: 100,
fit: BoxFit.cover,
),
],
),
),
),
);
}
}
Subscribe to my newsletter
Read articles from Vinit Mepani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vinit Mepani
Vinit Mepani
"Hello World, I'm Vinit Mepani, a coding virtuoso driven by passion, fueled by curiosity, and always poised to conquer challenges. Picture me as a digital explorer, navigating through the vast realms of code, forever in pursuit of innovation. In the enchanting kingdom of algorithms and syntax, I wield my keyboard as a magical wand, casting spells of logic and crafting solutions to digital enigmas. With each line of code, I embark on an odyssey of learning, embracing the ever-evolving landscape of technology. Eager to decode the secrets of the programming universe, I see challenges not as obstacles but as thrilling quests, opportunities to push boundaries and uncover new dimensions in the realm of possibilities. In this symphony of zeros and ones, I am Vinit Mepani, a coder by passion, an adventurer in the digital wilderness, and a seeker of knowledge in the enchanting world of code. Join me on this quest, and let's create digital wonders together!"