Elevated Button and Attributes

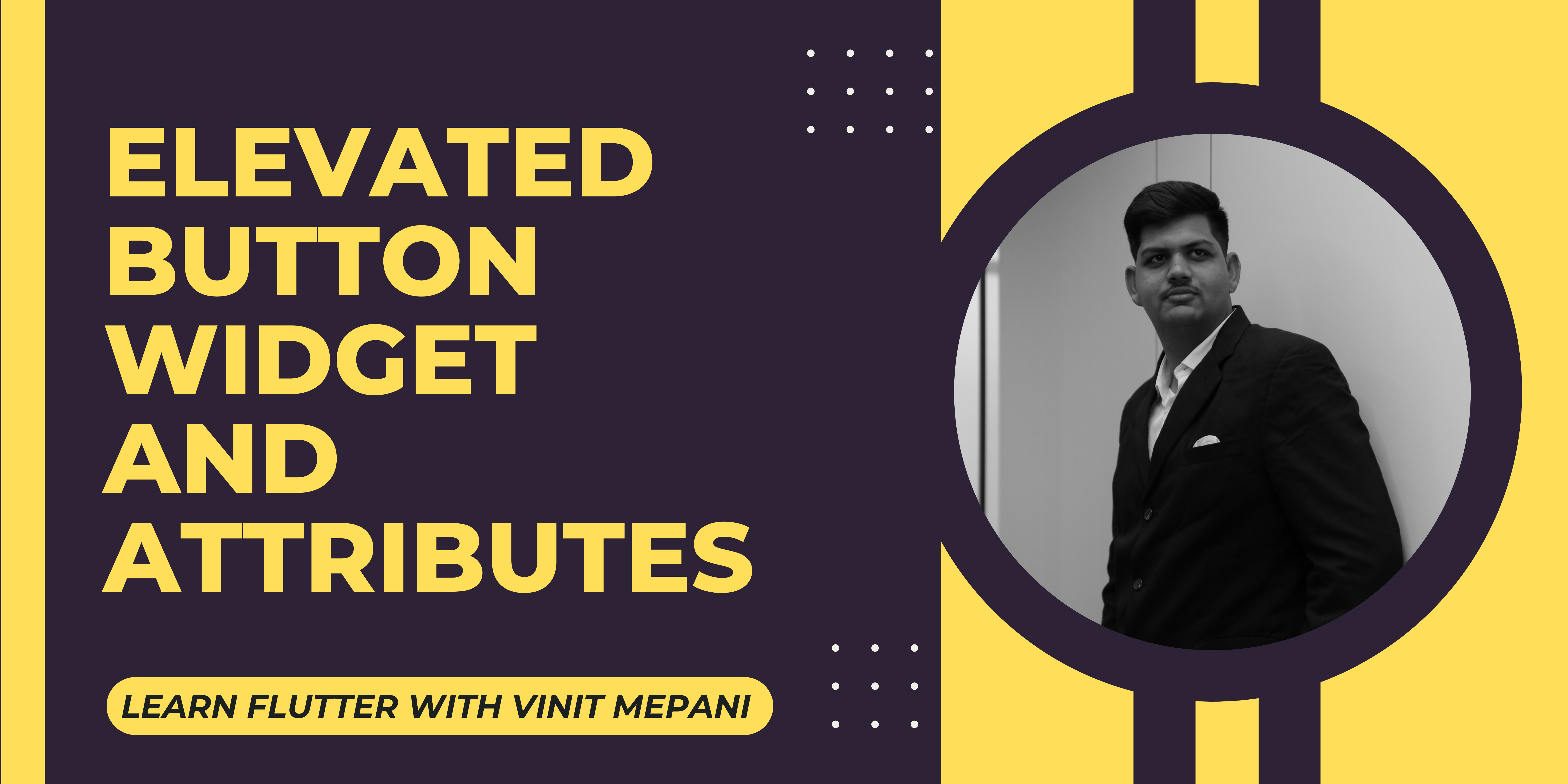
The ElevatedButton widget is part of the Flutter material library and is used to create a button with a raised appearance. It's a great choice for primary action buttons in your application.
Key Attributes and Properties:
onPressed:
- The onPressed attribute is essential for defining the action to be taken when the button is pressed. It typically takes a callback function.
Example:
dartCopy codeElevatedButton(
onPressed: () {
// Your action here
},
child: Text('Click me'),
)
child:
- The child attribute is where you specify the widget that represents the content of the button. It can be text, an icon, or any other widget.
Example:
ElevatedButton(
onPressed: () {
// Your action here
},
child: Text('Click me'),
)
style:
- The style attribute allows you to customize the appearance of the button, such as its background color, text style, elevation, and more.
Example:
ElevatedButton(
onPressed: () {
// Your action here
},
child: Text('Click me'),
style: ElevatedButton.styleFrom(
primary: Colors.blue, // Background color
onPrimary: Colors.white, // Text color
elevation: 5, // Elevation
),
)
Example:
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('ElevatedButton Example'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
'Flutter ElevatedButton Example',
style: TextStyle(fontSize: 18),
),
SizedBox(height: 20),
ElevatedButton(
onPressed: () {
// Handle button press
print('ElevatedButton pressed!');
},
child: Text('Click me'),
),
SizedBox(height: 20),
ElevatedButton.icon(
onPressed: () {
// Handle button press
print('Icon ElevatedButton pressed!');
},
icon: Icon(Icons.star),
label: Text('Icon Button'),
),
SizedBox(height: 20),
ElevatedButton(
onPressed: () {
// Handle button press
print('Custom Styled Button pressed!');
},
child: Text('Custom Styled Button'),
style: ElevatedButton.styleFrom(
primary: Colors.green,
onPrimary: Colors.white,
elevation: 8,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10),
),
),
),
],
),
),
),
);
}
}
Explanation:
The example demonstrates the usage of ElevatedButton and ElevatedButton.icon.
It includes a basic styled button and a button with an icon.
The style attribute is utilized to customize the appearance of the button with a custom background color, text color, elevation, and shape.
Subscribe to my newsletter
Read articles from Vinit Mepani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vinit Mepani
Vinit Mepani
"Hello World, I'm Vinit Mepani, a coding virtuoso driven by passion, fueled by curiosity, and always poised to conquer challenges. Picture me as a digital explorer, navigating through the vast realms of code, forever in pursuit of innovation. In the enchanting kingdom of algorithms and syntax, I wield my keyboard as a magical wand, casting spells of logic and crafting solutions to digital enigmas. With each line of code, I embark on an odyssey of learning, embracing the ever-evolving landscape of technology. Eager to decode the secrets of the programming universe, I see challenges not as obstacles but as thrilling quests, opportunities to push boundaries and uncover new dimensions in the realm of possibilities. In this symphony of zeros and ones, I am Vinit Mepani, a coder by passion, an adventurer in the digital wilderness, and a seeker of knowledge in the enchanting world of code. Join me on this quest, and let's create digital wonders together!"