Harnessing the Power of the Intersection Observer API for Enhanced Web Performance
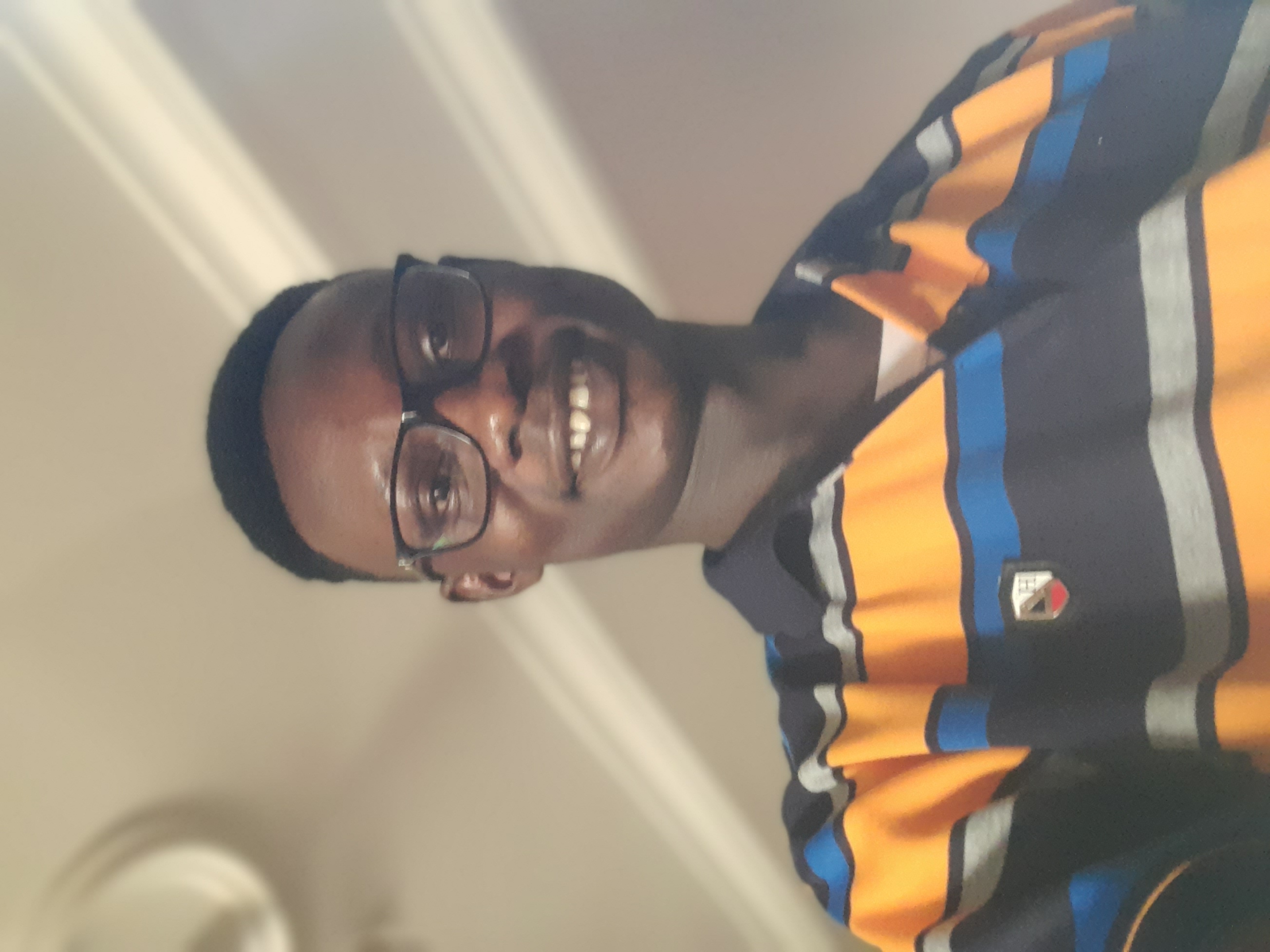
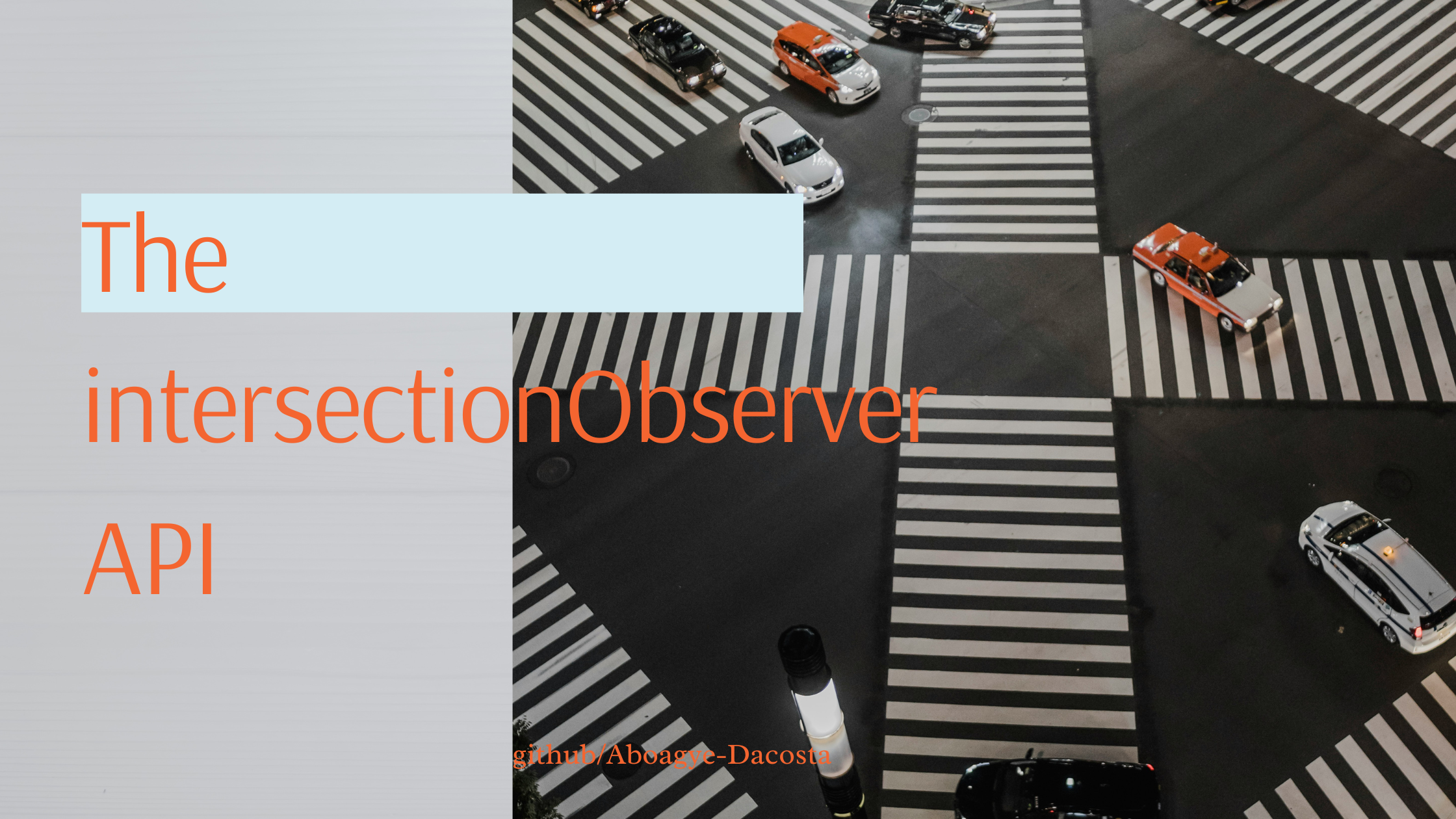
Let's dive right in and discover the amazing power of IntersectionObserver, but before everything, I think a small introduction will not hurt ๐๐.
In today's fast-paced digital landscape, optimizing website performance is crucial for providing a seamless user experience. One effective technique for achieving this goal is lazy loading, which defers the loading of non-essential resources until they are needed. Enter the Intersection Observer API, a modern web API that makes it easy to handle viewport-related interactions in web development without using any third-party packages.
What is the Intersection Observer API?
The Intersection Observer API is a JavaScript API that allows developers to asynchronously observe changes in the intersection of a target element with an ancestor or the viewport. Unlike traditional scroll event listeners, which can be resource-intensive and less precise, the Intersection Observer API provides a more efficient and performant solution for handling visibility-related interactions.
Use Cases:
Lazy Loading Images: Lazy loading images with the Intersection Observer API can significantly improve page load times by only loading images when they enter the viewport. This approach reduces initial page weight and enhances user experience, especially on content-heavy websites.
Infinite Scrolling: Implementing infinite scrolling becomes seamless with the Intersection Observer API. By detecting when the user scrolls near the end of a page or container, developers can dynamically fetch and load additional content, providing a smooth browsing experience without the need for manual pagination.
Dynamic Content Loading: The Intersection Observer API enables developers to dynamically load content as it becomes visible to the user. Whether it's loading more articles in a news feed or revealing interactive elements on scroll, this approach enhances the perceived speed of page navigation and keeps users engaged.
Adaptive content layouts: Developers can make more responsive and user-friendly interfaces that adjust to different screen sizes and device orientations by keeping an eye on how elements within a layout intersect. This allows for layouts that change depending on which elements are visible.
I think it's time to see some code in action instead of all these readmes.
In this post, we will be building together a static webpage using IntersectionObserver to add sticky navigation and lazy loading of images. But before we jump into it, let's look at the ins and outs of this API.
Steps to using the API.
- Create a new observer and assign it to a variable.
const observer = new IntersectionObserver(callback,options);
- Assign an object to be observed from the view port.
//let's say we have a couple of elements with the class .lazy-load
const entities = document.querySelectorAll(".lazy-load");
entities.forEach(entity=> observer.observe(entity));
// the observer is the object we created from above.
and that's all you need to get going. But you might be asking, What is this callback, and what are the options? Don't worry, we will get into that right away.
OK, let's talk about the options first. The options object takes a couple of values
root (default=null): This option specifies the element that is used as the viewport for checking visibility changes. If not specified or null, the browser viewport is used.
rootMargin (default: "0px"): This option allows you to specify a margin around the root element's bounding box. This margin is used to grow or shrink the root element's bounding box for the purpose of calculating intersections.
threshold (default: 0): This option specifies the threshold(s) at which to trigger the callback function. It can be a single number or an array of numbers between 0 and 1. A value of 0 means the callback will be triggered as soon as even a single pixel is visible within the target element, while a value of 1 means the entire target element must be visible.
trackVisibility (default: false): This option specifies whether to automatically track the visibility of the target element even if it is not currently intersecting with the root element. When set to true, the observer will continue to monitor the target element's visibility even when it's outside the viewport.
delay (default: null): This option allows you to specify a delay in milliseconds before the observer's callback is invoked after a change in intersection status occurs. This can be useful for optimizing performance by reducing the frequency of callback invocations.
intersectionCallback (default: null): This option allows you to specify a callback function that will be invoked whenever the intersection status of the target element changes. This callback function receives an array of IntersectionObserverEntry objects as its argument, each representing an intersection change.
Now, that's all for the options object. I hope we are cool now. If so, let's dive into the callback and how to wire it up.
//for the options
const options = {
root: null,
delay: 200,
rootMargin: "0px",
trackVisibility: false,
...
}
const callback = entries => {
entries.forEach(entry => {
if(entry.isIntersecting) {
//your code goes here
observer.unobserve(entry.target);
//stop observing the target once the operations are done.
}
}
}
const observer = new IntersectionObserver(callback,options);
This is mostly all you need to perform your lazy loading, but you can add more complex operations.
Advantages of the Intersection Observer API:
Improved Performance: The Intersection Observer API offers a more efficient alternative to traditional scroll event listeners, resulting in better overall performance and reduced browser overhead.
Precise Visibility Detection: Unlike scroll event listeners, which may not accurately detect visibility changes, the Intersection Observer API provides precise visibility detection based on element intersections.
Support for Complex Layouts: The Intersection Observer API seamlessly handles complex layout scenarios, making it ideal for modern web development projects with dynamic content and adaptive designs.
BAMMER alert
Now I think this post is getting way too long, or don't you think so? Let's continue in my next post, where we build an amazing webpage using this API.
I would love to hear what you think of this API but from my perspective i think its one of the best APIs to leverage when building modern web application.
Subscribe to my newsletter
Read articles from Dacosta Solomon Aboagye directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
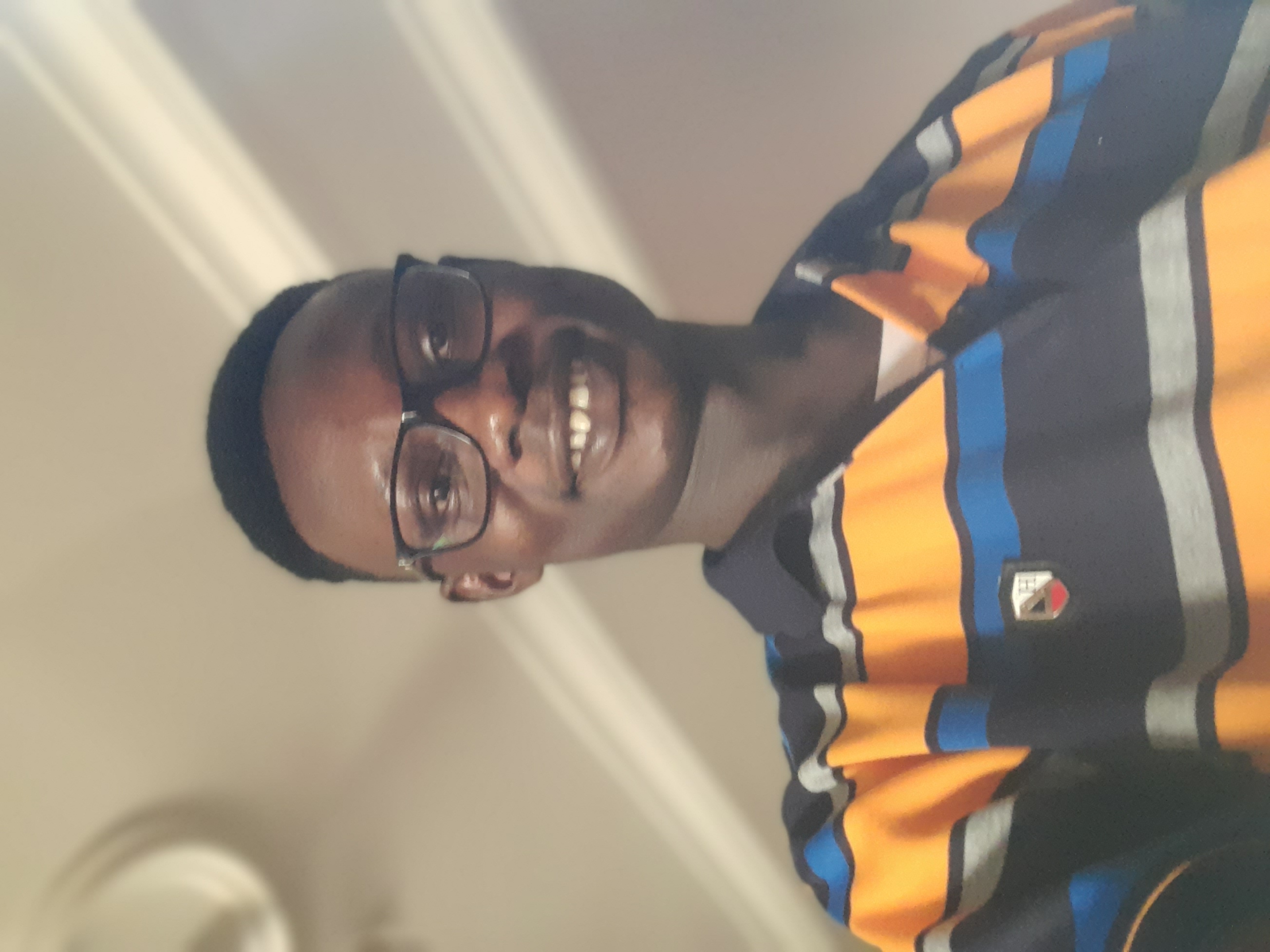
Dacosta Solomon Aboagye
Dacosta Solomon Aboagye
I am a self-motivated full-stack developer. I am currently schooling at Kwame Nkrumah University of Science and Technology. I enjoy programming. I also enjoy music.