Angular Signals
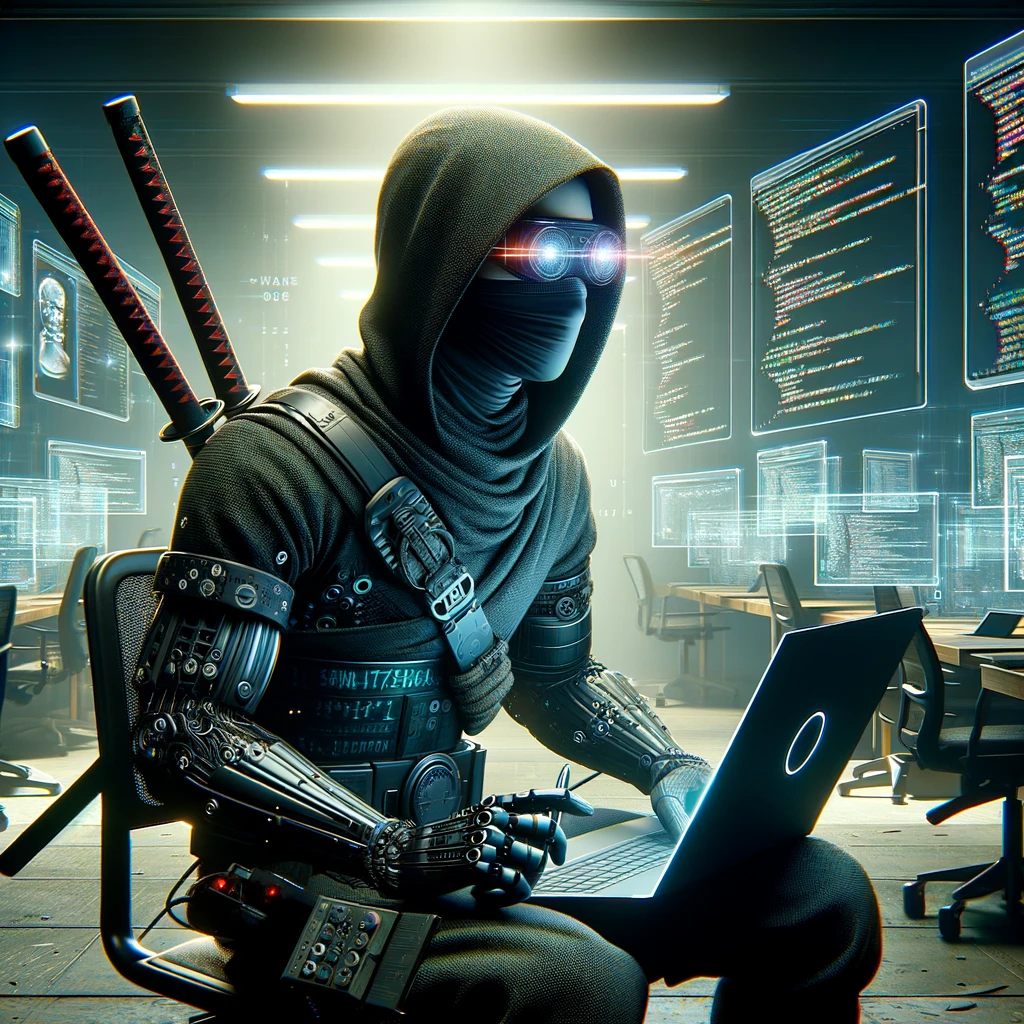
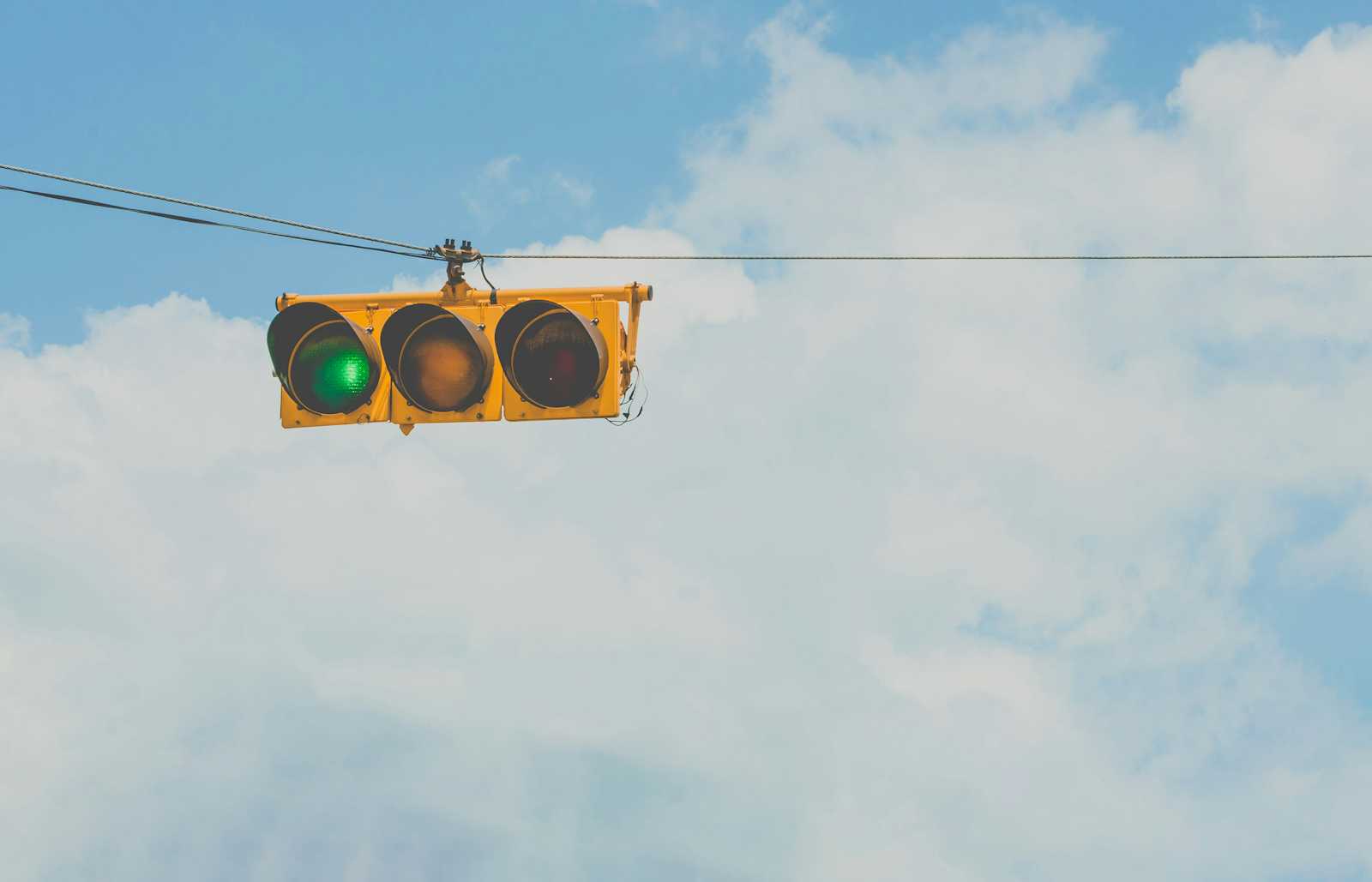
Angular Signals, introduced in Angular 17, are a part of Angular's efforts to enhance reactive programming capabilities within the framework. Signals provide a new way to manage and propagate changes in your application's state, making it easier to build dynamic and responsive user interfaces. Below are some basic examples to illustrate how you can use Angular Signals in your projects. These examples assume familiarity with Angular basics, including components, services, and TypeScript syntax.
Example 1: Defining and Using a Signal
First, let's see how to define a Signal and use it within a component to reactively update the UI.
import { Component } from '@angular/core';
import { bootstrapApplication } from '@angular/platform-browser';
import 'zone.js';
import { signal } from '@angular/core';
@Component({
selector: 'app-root',
standalone: true,
template: `
<h1>{{ name }}!</h1>
<a target="_blank" href="https://angular.io/guide/signals">
Learn more about Angular signals
</a>
<div>Count: {{ count() }}</div>
<button (click)="increment()">Increment</button>
`,
})
export class App {
name = 'Angular Signals';
count = signal(0); // Define a signal with an initial value of 0
increment() {
this.count.update( value => value+1); // Update the signal's value
}
}
bootstrapApplication(App);
In this example, count
is a Signal that holds a numeric value 0
. The increment()
method updates the value of count
by incrementing it.
The template binds directly to {{ count() }}
, and Angular automatically updates the UI when the Signal's value changes.
Demo : Stack Blitz
Programming is not just about writing code; it's about breathing life into ideas, solving the unsolvable, and creating a future that only exists in our imaginations.
Subscribe to my newsletter
Read articles from VJ directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
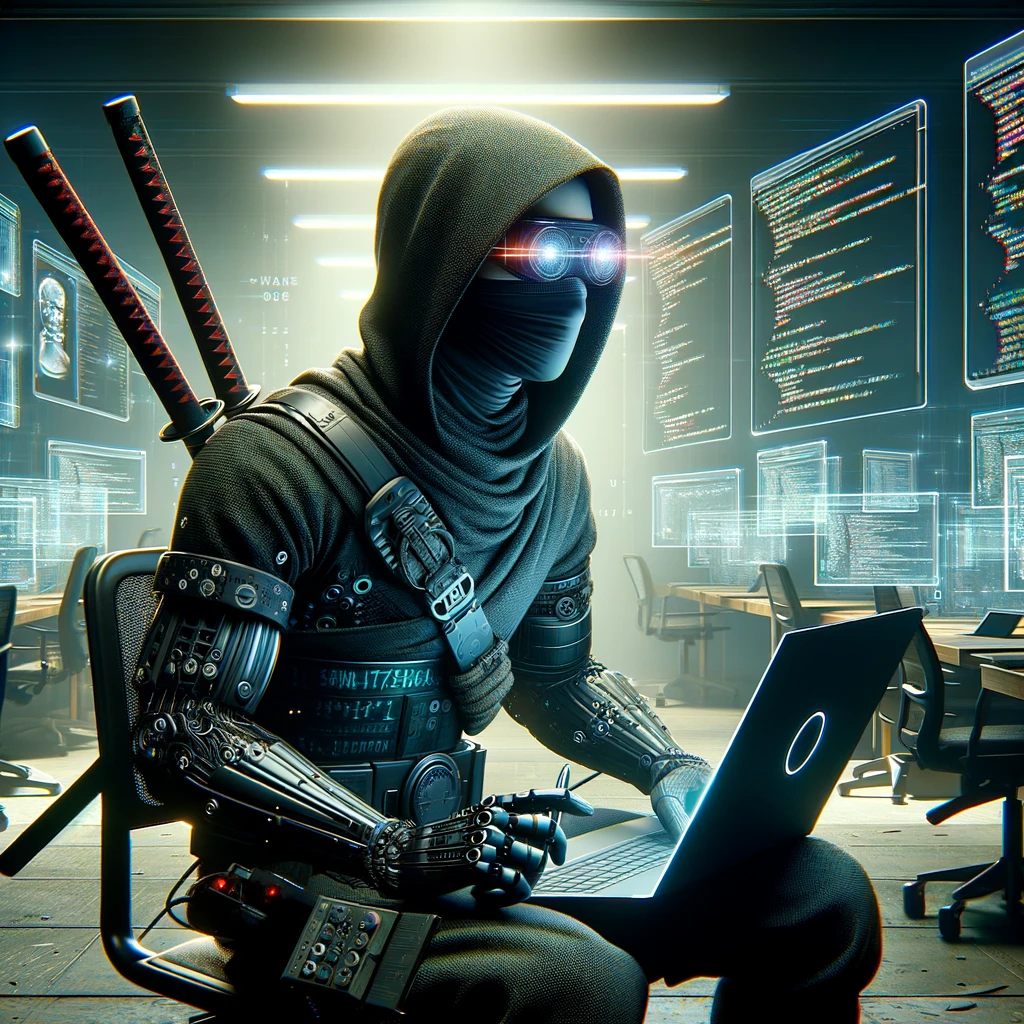
VJ
VJ
#Coding #Tech #FullStack #WebDev