Lexical Environment
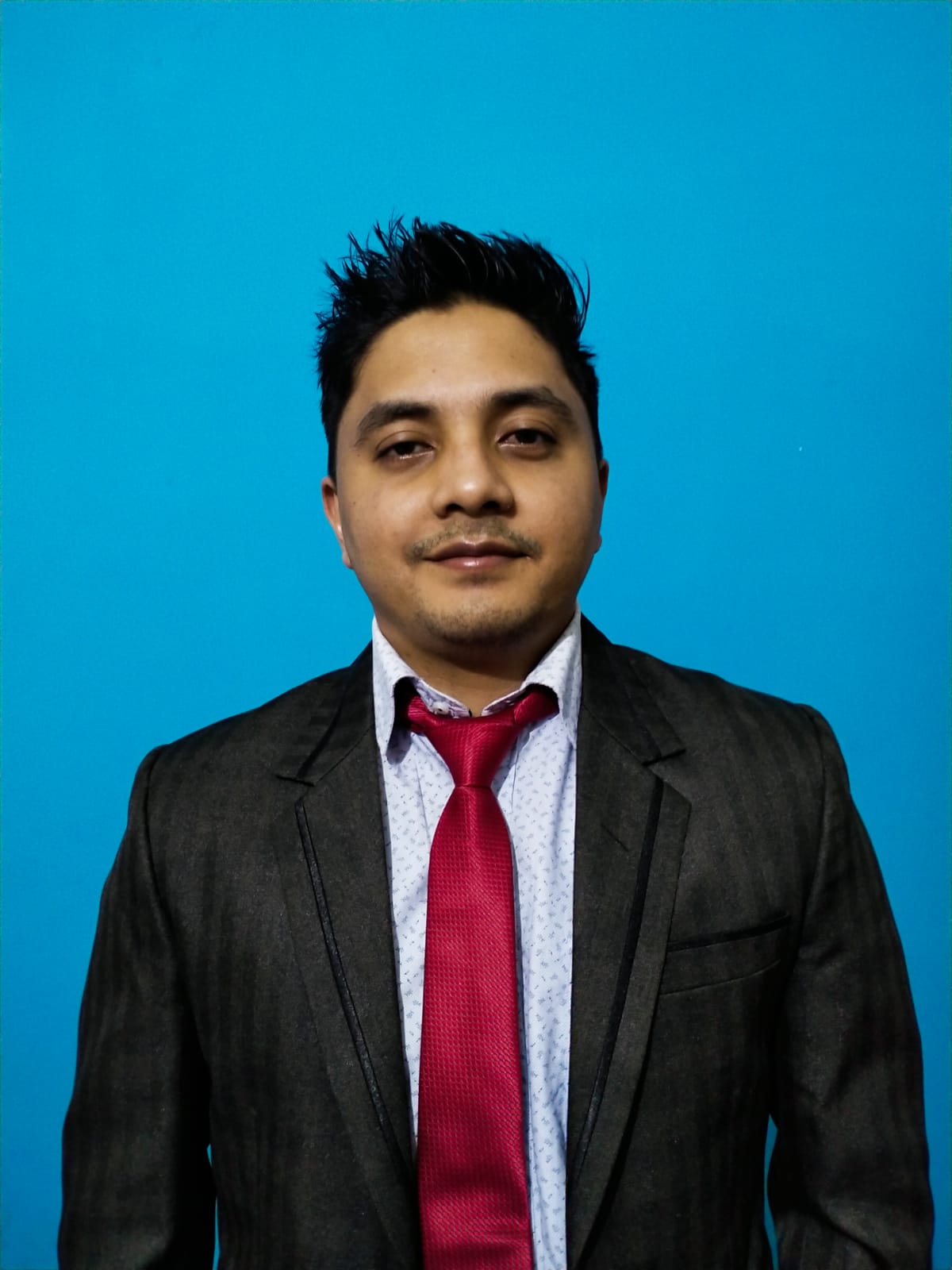
Delving into JavaScript programming often introduces the concept of "lexical environment." While it may initially appear daunting, fret not! In this blog post, we'll demystify what lexical environment means in simple terms and explore its practical implications through examples.
Understanding Lexical Environment
A lexical environment serves as a container for variables and functions within a specific scope in JavaScript. It dictates the accessibility and visibility of these identifiers during code execution.
Components of Lexical Environment
Environment Record: This component stores variables and functions declared within the scope.
Reference to Outer Environment: Each lexical environment maintains a reference to its outer lexical environment, forming a scope chain. This linkage enables inner functions to access variables from outer scopes.
Exploring with an Example
Let's illustrate lexical environment through a straightforward example:
function outer() {
let outerVar = 'Outer variable';
function inner() {
let innerVar = 'Inner variable';
console.log(innerVar); // Output: Inner variable
console.log(outerVar); // Output: Outer variable
}
inner();
}
outer();
Here, outerVar
is declared in the outer function outer()
, while innerVar
is declared within inner()
. The lexical scoping rules allow inner()
to access both outerVar
and innerVar
, showcasing the concept of lexical environment.
Recognizing Lexical Scopes
The lexical scoping mechanism permits inner functions to access variables from outer scopes. However, variables declared within inner scopes remain inaccessible to outer functions due to unidirectional lexical scope.
Conclusion
In essence, lexical environments contextualize variables and functions within JavaScript scopes. Mastering lexical contexts is fundamental for comprehending how variables and functions behave across code segments, facilitating the development of robust and error-resistant JavaScript code.
To deepen your understanding of JavaScript programming, continue exploring and experimenting with lexical environments! Here's to your ongoing coding journey!
Subscribe to my newsletter
Read articles from Rahul Das Sarma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
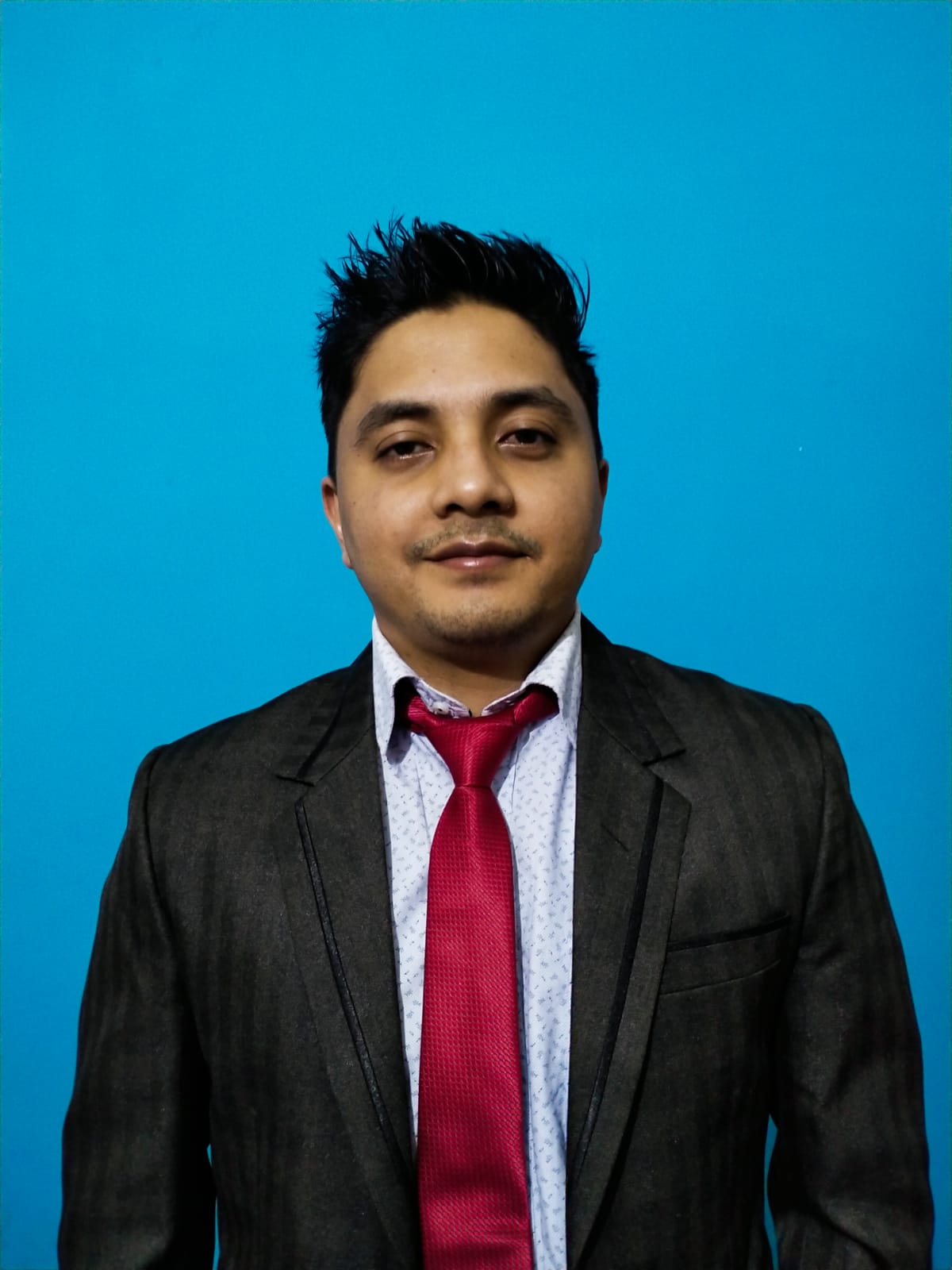
Rahul Das Sarma
Rahul Das Sarma
A react developer with dreams!