isdigit() function in C
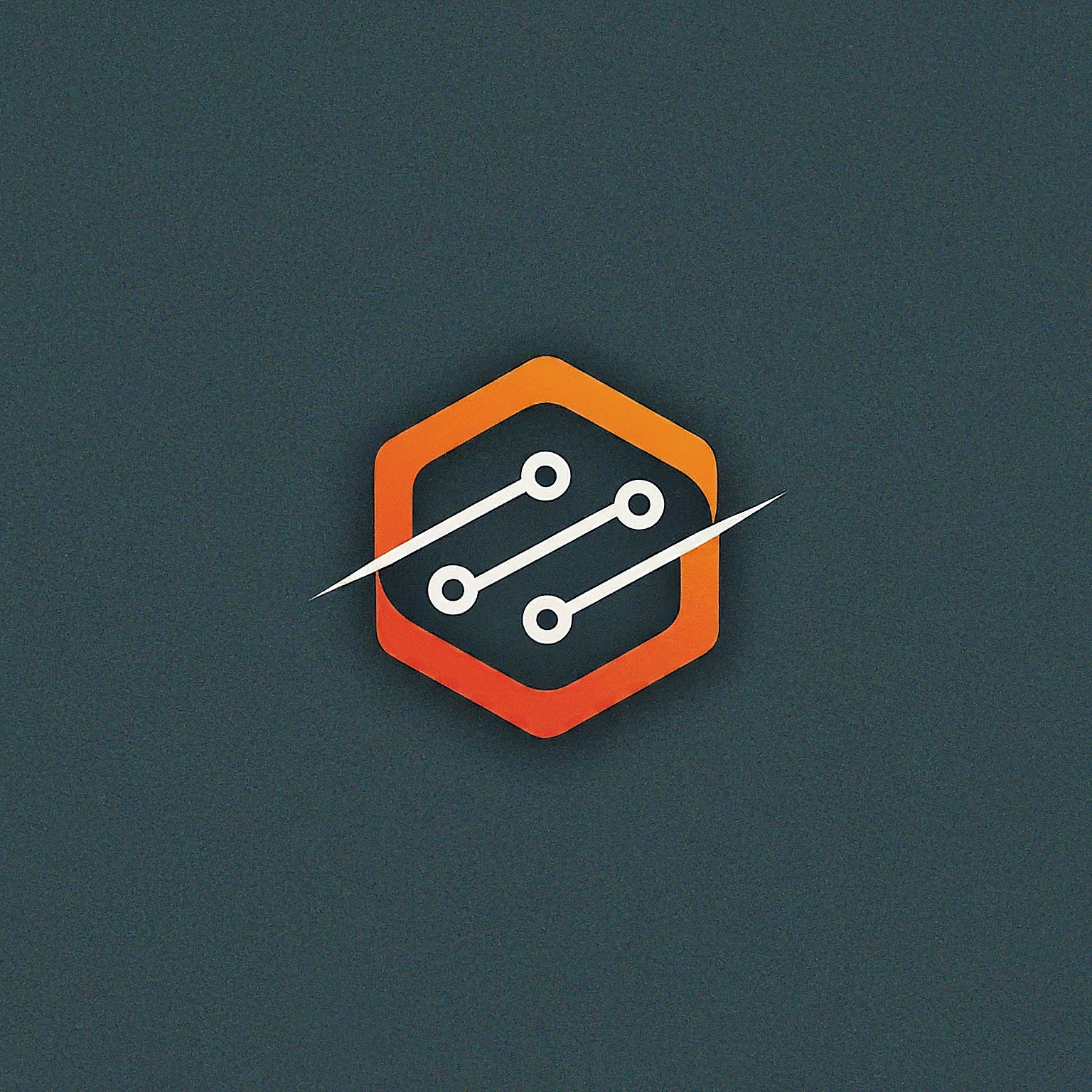
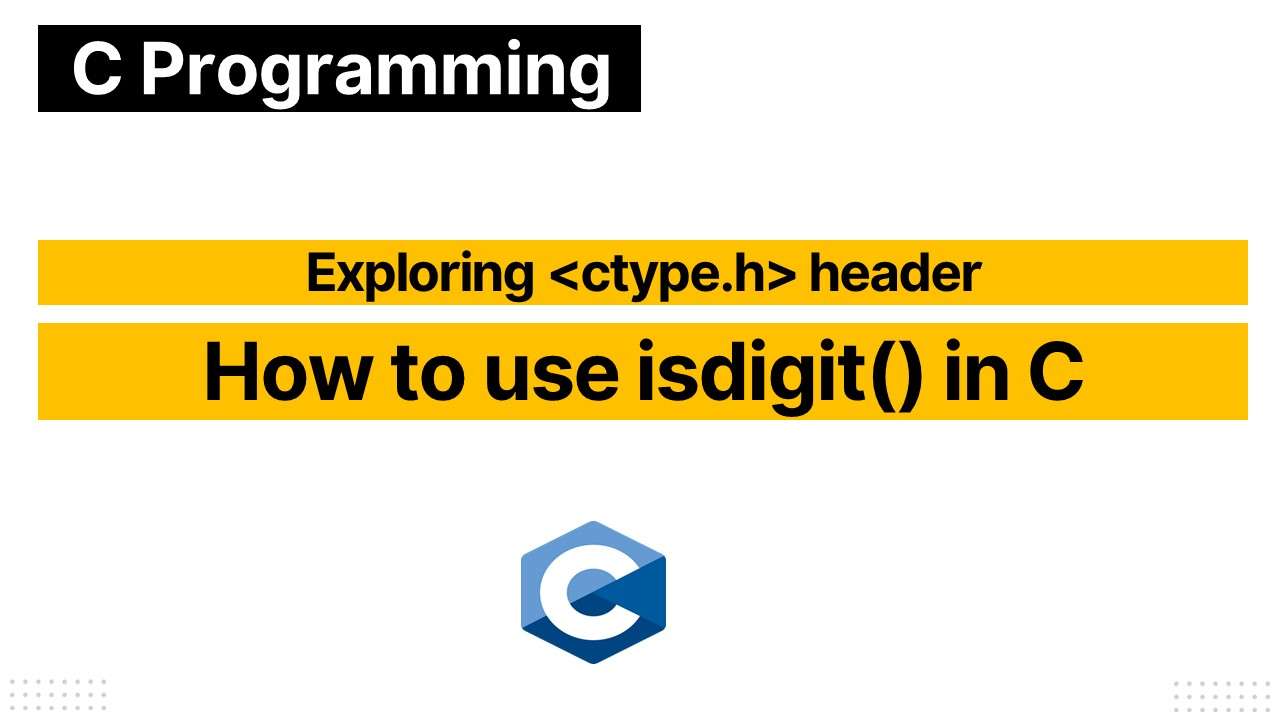
Using the isdigit()
function in C
The isdigit()
function in C is used to check whether a character is a digit (0 to 9). The function takes a character as input and returns a non-zero value if the character is a digit, or zero if the character is not a digit.
Here is an example of how to use the isdigit()
function:
#include <stdio.h>
#include <stdint.h>
#include <ctype.h>
int32_t main(int32_t argc, char const *argv[])
{
char c = '0';
int32_t result = isdigit(c);
if (result > 0x00)
{
printf("The character '%c' is a digit.\n", c);
}
else
{
printf("The character '%c' is not a digit.\n", c);
}
return 0;
}
The isdigit()
function takes a character as input and returns a non-zero value if the character is a digit, or zero if the character is not a digit. In this example, the character '0'
is a digit, so the function will return a non-zero value and the printf()
statement will print the output.
Explore the complete list of functions available in ctype header in C standard library.
Subscribe to my newsletter
Read articles from Embedded DeepDive directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
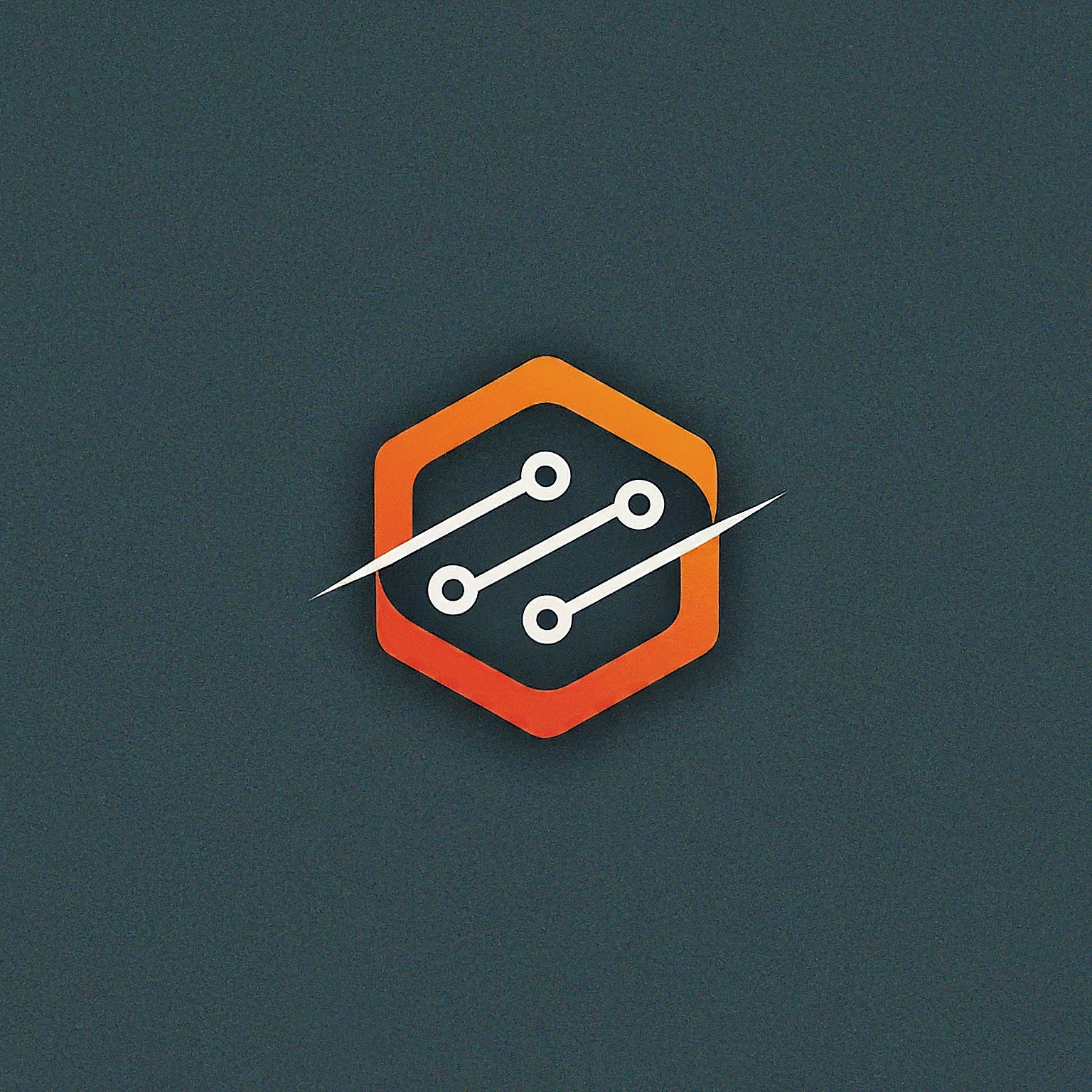
Embedded DeepDive
Embedded DeepDive
Driving innovation in embedded systems, IoT, and FPGA/SOC development. Delivering cutting-edge solutions, expert technical services, and empowering the tech community with tutorials and practical guides.