toupper() function in C
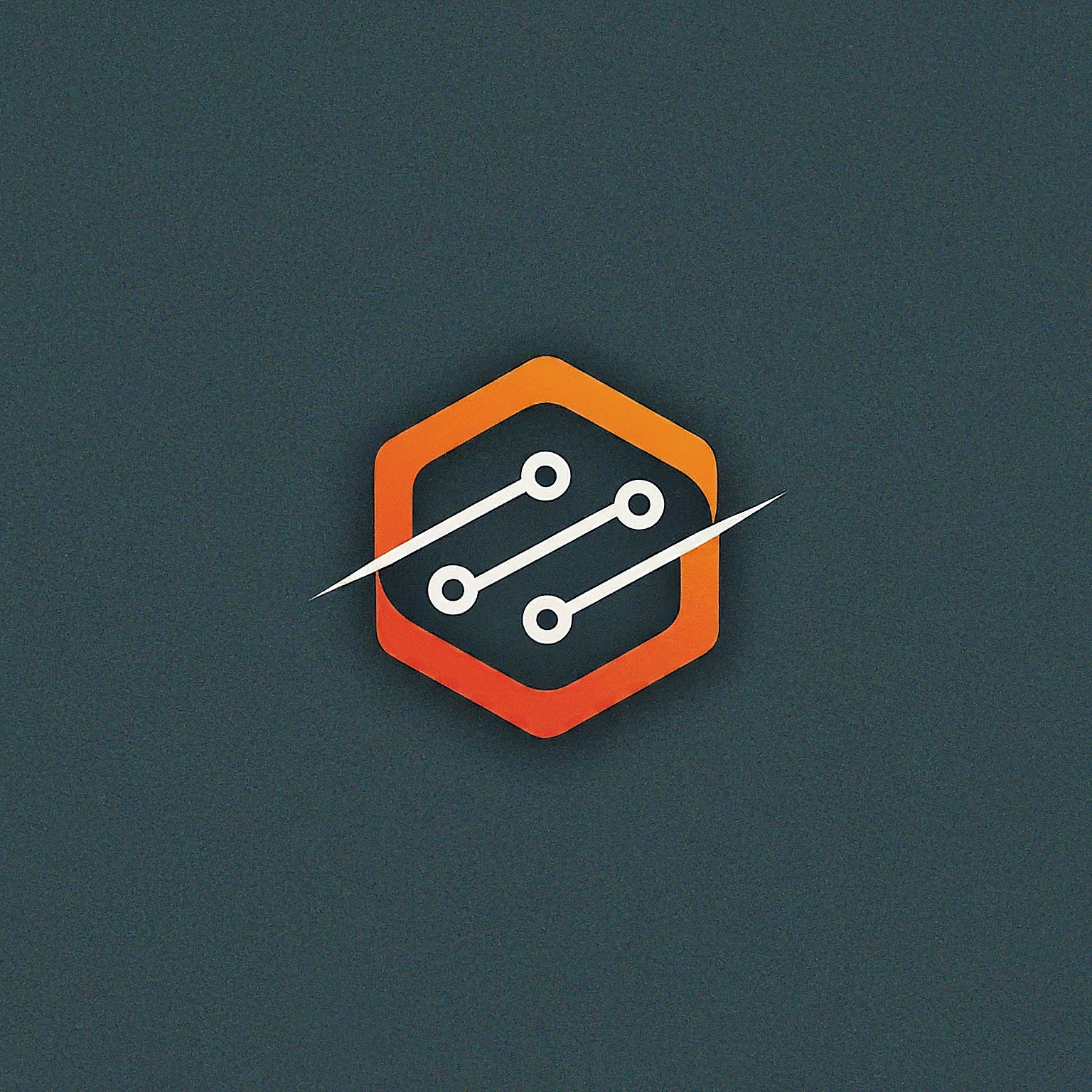
Table of contents
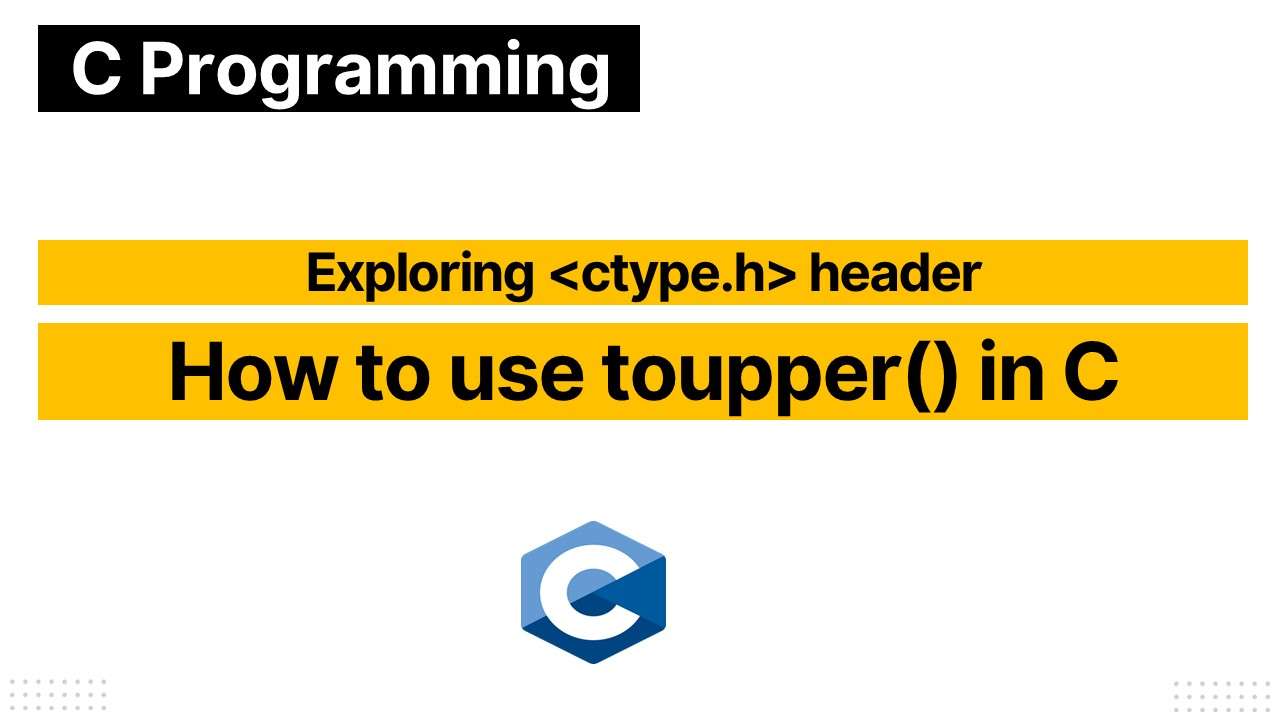
Using the toupper()
function in C
The toupper()
function in C is used to convert a lowercase character to its uppercase counterpart. For example, the toupper()
function would convert the character 'a'
to 'A'
.
The toupper()
function takes a character as input and returns the converted character.
#include <stdio.h>
#include <stdint.h>
#include <ctype.h>
int32_t main(int32_t argc, char const *argv[])
{
char c = 'a';
char upper_c = toupper(c);
printf("The uppercase version of '%c' is '%c'.\n", c, upper_c);
return 0;
}
Converts lowercase letters to uppercase. Returns the uppercase equivalent to letters, if such value exists, else letters remains unchanged. The character’s value must be representable as an unsigned char or the value of EOF.
Explore the complete list of functions available in ctype header in C standard library.
Subscribe to my newsletter
Read articles from Muhammad Hassaan Shah directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
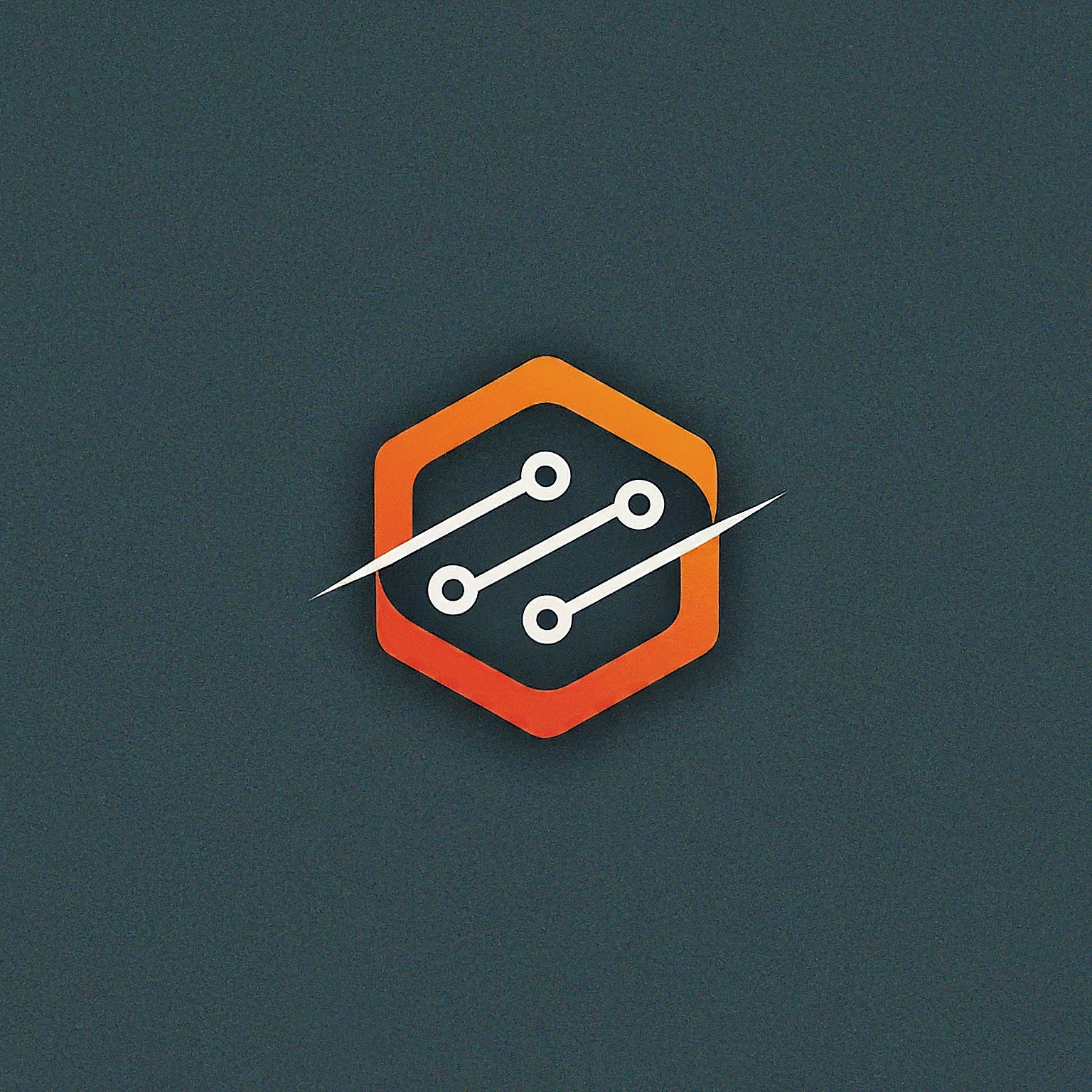
Muhammad Hassaan Shah
Muhammad Hassaan Shah
Passionate about new technological trends and like to share practical tips and tutorials about C, C++, Python, MATLAB, STM32, Arduino, ESP32, and more …