How to Catch, Handle and Re-throw EXCEPTIONS in Python ?
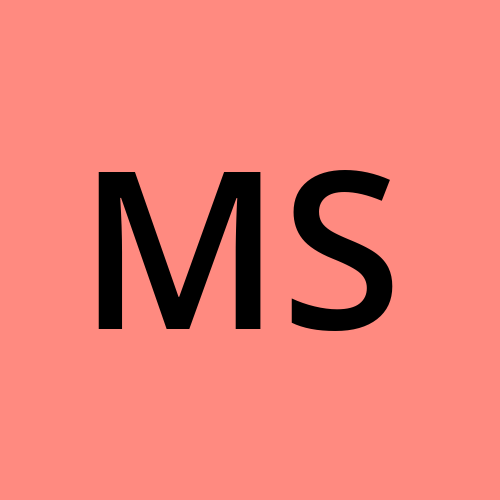
In Python, you can catch, handle, and re-throw exceptions using a try
, except
, and raise
block. Here's an example:
def example_function():
try:
# Code that may raise an exception
result = 10 / 0 # This will raise a ZeroDivisionError
except ZeroDivisionError as ex:
# Handle the exception
print(f"Caught an exception: {ex}")
# Log the exception or perform other actions
# Re-throw the exception
raise # Re-throw the caught exception
# Call the function
try:
example_function()
except ZeroDivisionError as ex:
# Handle the re-thrown exception
print(f"Caught re-thrown exception: {ex}")
In this example:
The
example_function
contains code that may raise aZeroDivisionError
.Inside the
try
block, the code is executed, and if an exception occurs, it is caught by the correspondingexcept
block.Within the
except
block, you can handle the exception, perform logging, or take other actions as needed.After handling the exception, you can choose to re-throw the same exception using the
raise
statement without any specific exception type. This will propagate the exception to the calling code.
When the function is called, the initial exception is caught, handled, and then re-thrown. The outer try-except
block in the calling code can catch the re-thrown exception.
It's important to note that re-throwing an exception should be done judiciously, and it's generally a good practice to provide additional information or context when re-throwing to aid in debugging. You can also choose to raise a different exception if needed.
def example_function():
try:
result = 10 / 0 # This will raise a ZeroDivisionError
except ZeroDivisionError as ex:
# Add context information
print("Error in example_function:", ex)
# Log the exception or perform other actions
# Re-throw with additional context
raise RuntimeError("An error occurred in example_function") from ex
# Call the function
try:
example_function()
except RuntimeError as ex:
# Handle the re-thrown exception
print(f"Caught re-thrown exception: {ex}")
# Access the original exception using __cause__
original_exception = ex.__cause__
print(f"Original exception: {original_exception}")
In this modified example, a RuntimeError
is raised with additional context, and the original exception (ZeroDivisionError
) is set as the cause using the from ex
syntax. This can provide more information about the context in which the error occurred.
Subscribe to my newsletter
Read articles from Muhammad Sohail directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
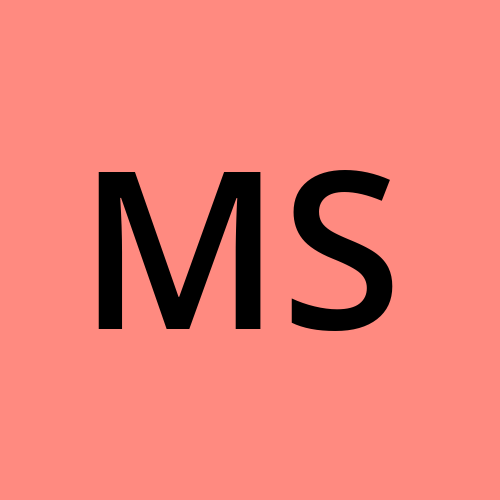