*args and **kwargs in Python

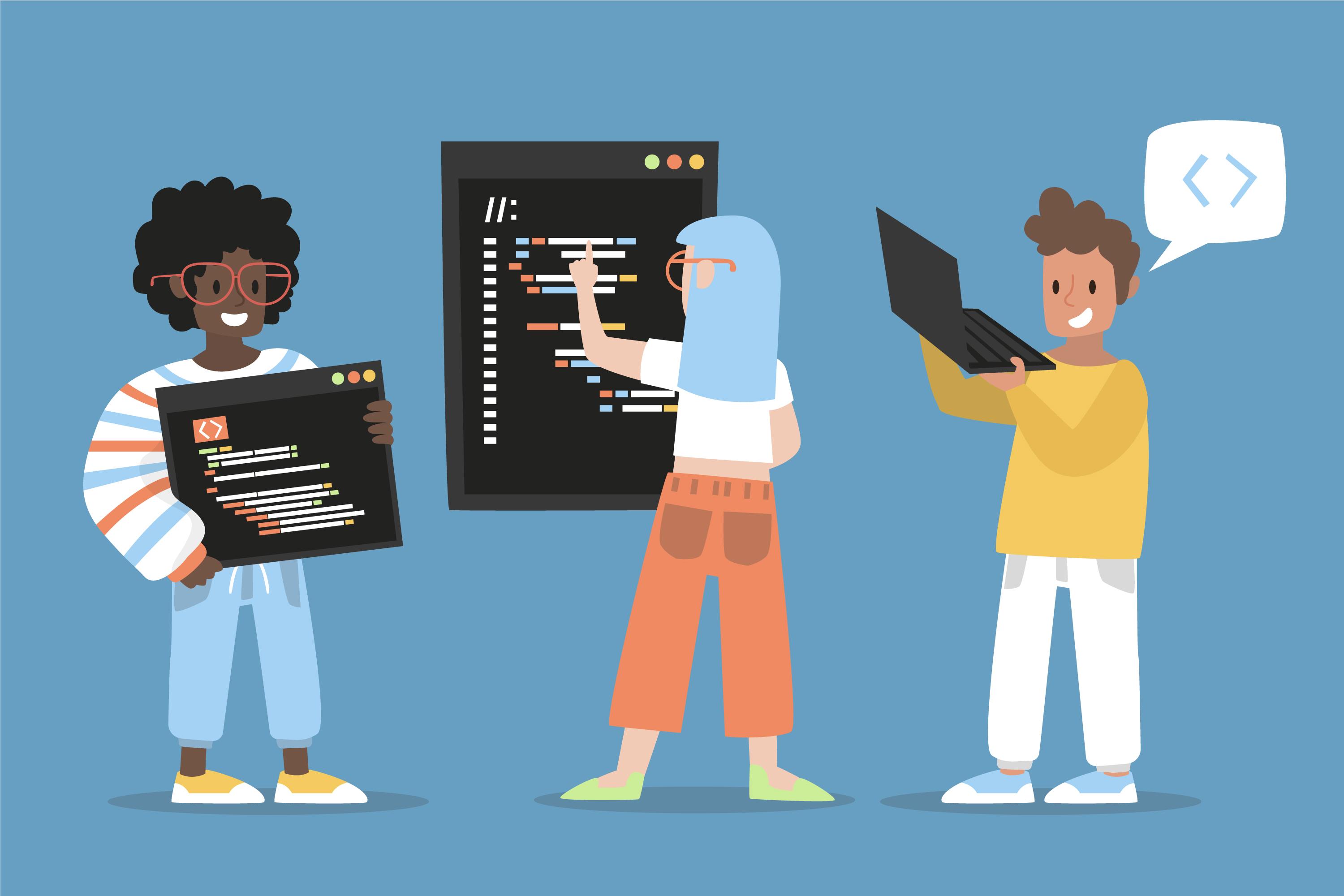
Python is an awesome and easily readable programming language that a lot of beginner programmers take to quickly for a plethora of reasons. In this article we would be taking a look at one of its many convenient features; the non-keyword (*args) and keyword (**kwargs) arguments.
In a typical python function, there are features we expect to see. There is the 'def' keyword used to initiate a function, followed by a parenthesis that may or may not house a parameter (or number of parameters), the body of the function and a return statement (optional). These can be noted in the code snippet below.
def typicalFunction(someParameter):
#Some body of code that should do something such as:
print(someParameter)
"""
You are free to use 'return' instead, but be sure to print
the function when calling it!
"""
If you are quick to observe, you would see that 'someParameter' is a single entity, a single parameter. What if we required two parameters? That would be easy to implement.
def typicalFunction(someParameter, someOtherParameter):
#Some body of code that should do something such as:
print(someParameter, someOtherParameter)
Here, we have two parameters. Just that, two. We are only permitted to have two parameters because that is what is required by the function that we wrote and so the computer would go berserk if we did anything else. Don't believe me? Try adding a third when running the function as in below.
def typicalFunction(someParameter, someOtherParameter):
#Some body of code that should do something such as:
print(someParameter, someOtherParameter)
typicalFunction("Kirk", "Spock", "McCoy")
Above we supplied three arguments even though we indicated two parameters. Try copying and running the code above and tell me how angry the warnings look. So what do we do if we need to build a program that accepts an indefinite or variable number of parameters? That's when *args and **kwargs come in. It should be noted that the key stars in this play are the asterisk symbols and using any other terms in place of 'args' and 'kwargs' is acceptable (such as *num). However, for the purpose of consistency and because it is generally accepted, it is good to stick to 'args' and 'kwargs'.
def typicalFunction(*args):
#Some body of code that should do something such as:
print(args)
typicalFunction("Kirk", "Spock", "McCoy", "Scotty", "Uhura")
# We could keep on going! but we won't because I have a life so...
By doing the above we are able to supply an endless (well, not literally) number of arguments and we would still be able to run our code successfully. The same applies to **kwargs with the exception of the fact that this second kind of argument applies to keyword type arguments, the sort that concerns keys and values (as in a dictionary). This can be seen below.
def typicalFunction(**kwargs):
#Some body of code that should do something such as:
for key, value in kwargs.items():
print(f"{key}, I think is {value}")
typicalFunction(Kirk="a good leader", Spock="smart and has pointy ears", McCoy="a doctor, not a ballerina")
If the crew of the USS Enterprise have taught us anything, it is that with **kwargs we can have variable length keyword arguments in our code and they are better than Star Wars. Try altering the argument list by adding to or reducing the number of arguments and watch your code run like Kirk in that one episode of Star Trek.
Subscribe to my newsletter
Read articles from Amanda Ene Adoyi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Amanda Ene Adoyi
Amanda Ene Adoyi
I am a Software Developer from Nigeria. I love learning about new technologies and talking about coding. I talk about coding so much that I genuinely think I could explode if I don't . I have been freelance writing for years and now I do the two things I love the most: writing and talking about tech.