Mastering Real-Time Communication with Socket.IO and Next.js: A Comprehensive Guide
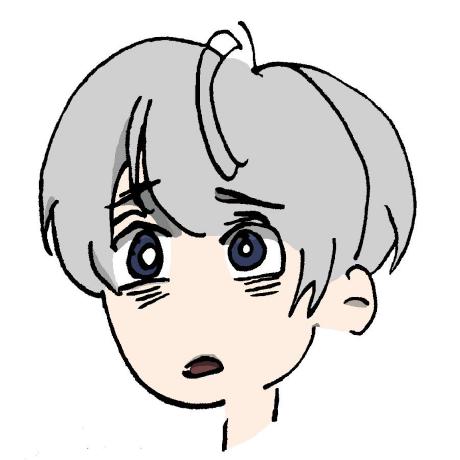
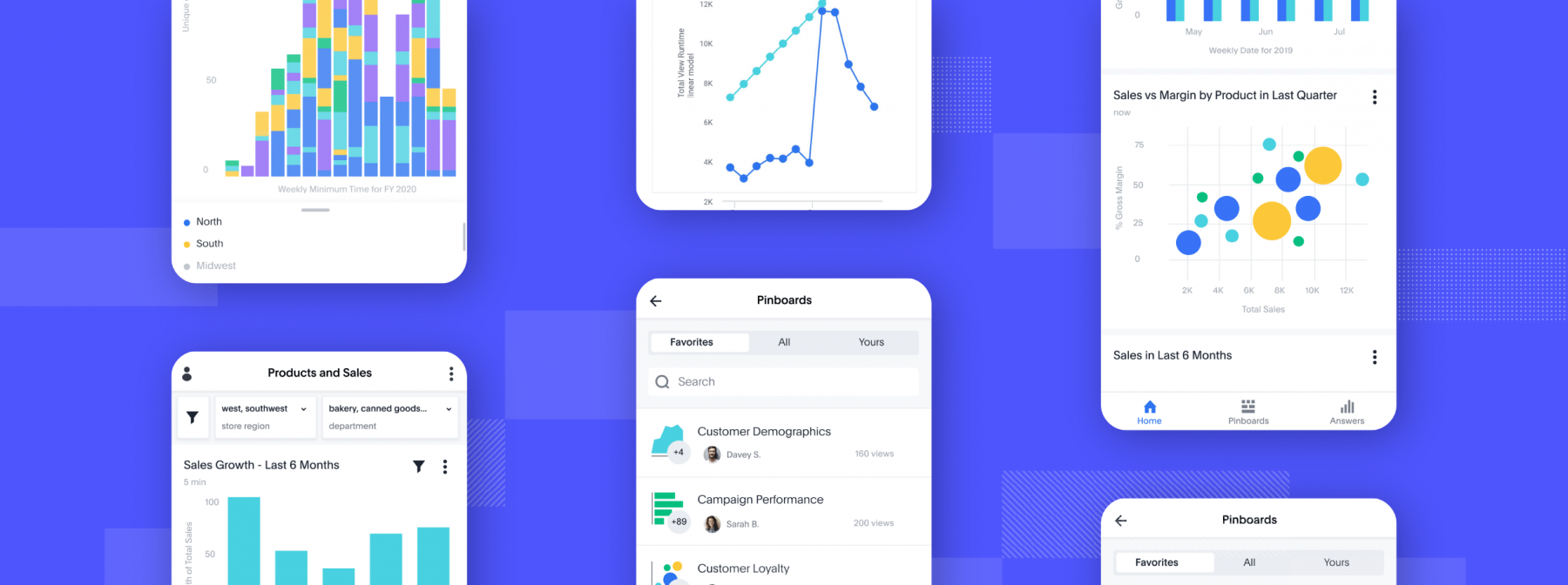
Title: Mastering Real-Time Communication with Socket.IO and Next.js: A Comprehensive Guide
Introduction
In the dynamic landscape of web development, real-time communication plays a pivotal role in creating engaging and interactive applications. One powerful tool that stands out in this domain is Socket.IO. In this blog post, we will embark on a journey to understand Socket.IO, its features, and how to leverage it in conjunction with Next.js to build robust real-time applications.
Table of Contents
UnderstandingSocket.IO
What is Socket.IO?
Key Features
Getting Started: Setting UpSocket.IOwith Next.js
Installing Socket.IO
Setting Up the Server
Connecting from the Next.js Client
Real-Time Bidirectional Communication withSocket.IOand Next.js
Emitting and Handling Events
Server-to-Client Messages
Client-to-Server Messages
Broadcasting Events
Broadcasting from the Server
Broadcasting within a Room
Acknowledgments
- Confirming Event Reception
Namespace and Rooms
Namespace: Segregating Communication
Rooms: Grouping Clients
Handling Disconnects
Graceful Disconnects
Handling Client Disconnects
IntegratingSocket.IOwith Express and Next.js
Combining Socket.IO with Express
Building a Real-Time Chat Application
Scalability and Redis Integration
Scaling Socket.IO Applications
Using Redis for Horizontal Scaling
Caching and Performance Optimization
SecuringSocket.IOApplications
Implementing Secure Connections (HTTPS)
Authentication and Authorization
2. Getting Started: Setting UpSocket.IOwith Next.js
InstallingSocket.IO
To get started with Socket.IO in a Next.js project, install it using npm:
npm install socket.io-client
Setting Up the Server
Create a simple Socket.IO server using Node.js and Express:
// server.js
const express = require('express');
const http = require('http');
const { Server } = require('socket.io');
const app = express();
const server = http.createServer(app);
const io = new Server(server);
io.on('connection', (socket) => {
console.log(`A user connected: ${socket.id}`);
});
const PORT = process.env.PORT || 3000;
server.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
Connecting from the Next.js Client
Include the Socket.IO client library in your Next.js project:
// pages/index.js
import { useEffect } from 'react';
import io from 'socket.io-client';
const Home = () => {
useEffect(() => {
const socket = io('http://localhost:3000');
}, []);
return <div>Next.js with Socket.IO</div>;
};
export default Home;
Now, your server and Next.js client are set up to establish a real-time connection.
3. Real-Time Bidirectional Communication withSocket.IOand Next.js
Emitting and Handling Events
Socket.IO allows you to emit and handle custom events:
Server-to-Client Messages
// Server
io.on('connection', (socket) => {
socket.emit('message', 'Welcome to the real-time world!');
});
// Next.js Client
import { useEffect } from 'react';
import io from 'socket.io-client';
const Home = () => {
useEffect(() => {
const socket = io('http://localhost:3000');
// Handling server-to-client message
socket.on('message', (data) => {
console.log('Received message:', data);
});
}, []);
return <div>Next.js with Socket.IO</div>;
};
export default Home;
Client-to-Server Messages
// Next.js Client
import { useState, useEffect } from 'react';
import io from 'socket.io-client';
const Home = () => {
const [inputMessage, setInputMessage] = useState('');
const [serverResponse, setServerResponse] = useState('');
useEffect(() => {
const socket = io('http://localhost:3000');
// Handling server-to-client message
socket.on('message', (data) => {
setServerResponse(data);
});
}, []);
const sendMessage = () => {
const socket = io('http://localhost:3000');
// Emitting client-to-server message
socket.emit('message', inputMessage, (ack) => {
console.log('Server acknowledgment:', ack);
});
};
return (
<div>
<input
type="text"
value={inputMessage}
onChange={(e) => setInputMessage(e.target.value)}
/>
<button onClick={sendMessage}>Send Message</button>
<p>Server Response: {serverResponse}</p>
</div>
);
};
export default Home;
Broadcasting Events
Broadcasting from the Server
// Server
io.on('connection', (socket) => {
// Broadcasting a message to all connected clients
io.emit('message', 'A new user has joined!');
});
Broadcasting within a Room
// Server
io.on('connection', (socket) => {
// Joining a room
Subscribe to my newsletter
Read articles from Saksham Tomer directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
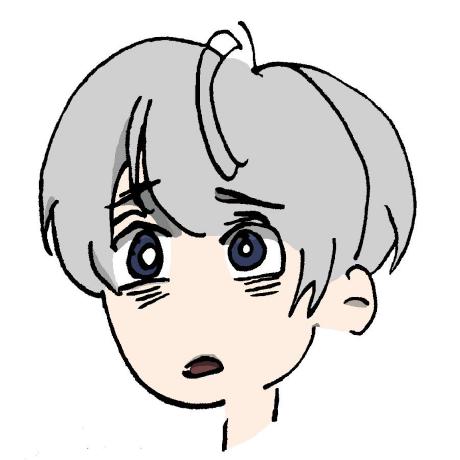