Introduction to UI Automation with Nightwatch.js

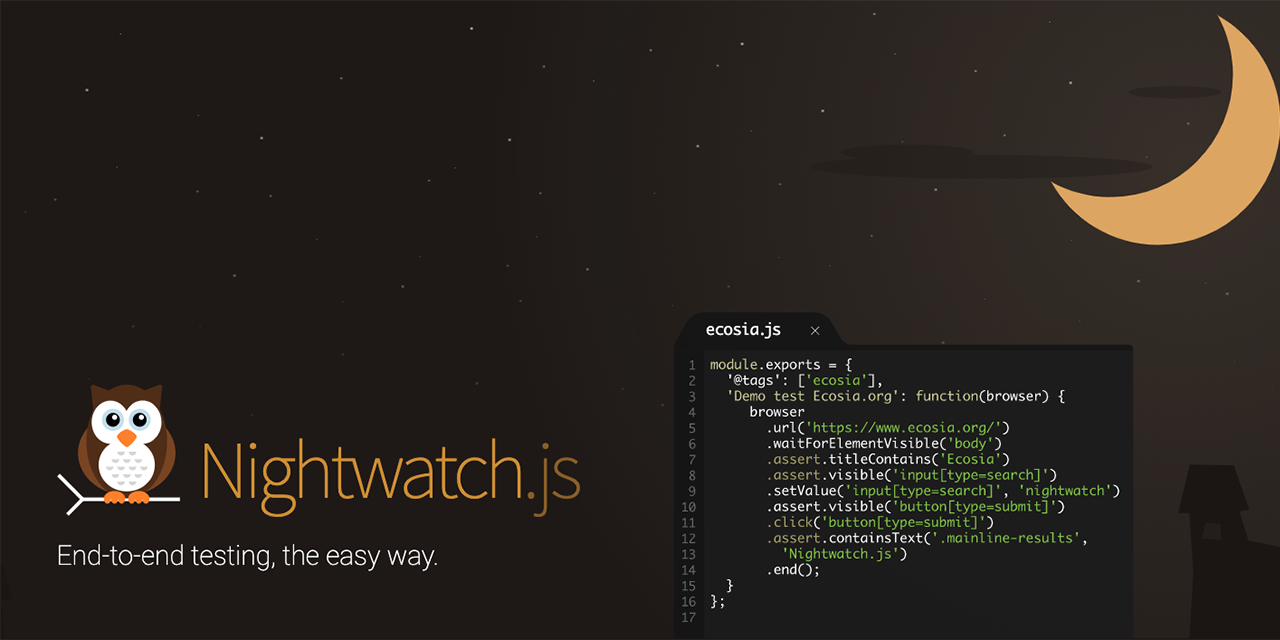
Hello everyone! ๐
In the dynamic realm of software development, UI automation stands as a crucial pillar in ensuring the seamless functionality and user experience of web applications. As technology continues to advance, so does the demand for efficient and reliable UI testing solutions.
Recently, I've been working on UI automation using Nightwatch.js, striving to enhance test coverage and refine automation frameworks. The objective? To streamline future automation efforts within this domain, making them more effective and less time-consuming than the initial implementation.
Through this blog, I endeavor to provide insights into Nightwatch.js, illuminating its capabilities, sharing my hands-on experiences, and discussing the advantages and challenges it presents. This isn't merely a blog; it's a platform for knowledge exchange. I welcome you to embark on this journey into the realm of UI automation with Nightwatch.js. Along the way, your feedback and contributions are not only encouraged but valued immensely! ๐
NightwatchJs
Nightwatch.js is an open-source automated testing framework for web applications and websites. It utilizes the Node.js runtime environment and the WebDriver API to automate interactions with web browsers. Nightwatch.js is primarily focused on end-to-end (E2E) testing, allowing developers and QA engineers to write and run automated tests to ensure the functionality, performance, and behavior of web applications across different browsers and platforms.
1. Getting Started with Nightwatch.js:
Introduction to Nightwatch.js and its features.
Installation guide for setting up Nightwatch.js.
Configuring your project structure for efficient automation.
// nightwatch.conf.js
module.exports = {
src_folders: ['tests'],
webdriver: {
start_process: true,
server_path: 'node_modules/.bin/chromedriver',
port: 9515,
},
test_settings: {
default: {
desiredCapabilities: {
browserName: 'chrome',
},
},
},
};
2. Writing Your First Test:
Understanding the structure of a Nightwatch.js test.
Writing a basic test scenario to interact with a web page.
Executing the test and analyzing the results.
// sampleTest.js
module.exports = {
'Open Google on browser' : function (browser) { // step 1
browser
.url('https://www.google.com')
.waitForElementVisible('body', 1000)
.assert.title('Google')
.assert.visible('input[type=text]')
.setValue('input[type=text]', 'nightwatch')
.waitForElementVisible('input[name=btnK]', 1000)
},
'Input value assertion' : function (browser) { // step 2
browser
.click('input[name=btnK]')
.pause(1000)
.assert.containsText('#search', 'Nightwatch.js')
.end();
},
};
3. Leveraging Page Objects for Maintainable Tests:
Introduction to the Page Object Model (POM).
Creating and organizing page objects to represent web pages and components.
Integrating page objects into Nightwatch.js tests for improved maintainability and reusability.
// googlePage.js (Page Object)
module.exports = {
elements: {
searchBar: 'input[type=text]',
searchButton: 'input[name=btnK]',
searchResults: '#search',
},
commands: [{
performSearch(query) {
return this.setValue('@searchBar', query)
.click('@searchButton')
.waitForElementVisible('@searchResults');
}
}]
};
4. Enhancing Tests with Assertions and Verifications:
Exploring the assertion and verification capabilities of Nightwatch.js.
Writing assertions to validate expected outcomes and behaviors.
Handling asynchronous operations and dynamic content in tests.
// sampleTest.js (Enhanced)
module.exports = {
'Open Google on browser and perform Input value assertion' : function (browser) {
const googlePage = browser.page.googlePage();
googlePage
.navigate()
.performSearch('nightwatch')
.assert.containsText('@searchResults', 'Nightwatch.js');
}
};
5. Working with Selectors and Interactions:
Understanding CSS and XPath selectors for locating elements.
Performing various interactions such as clicks, input, and navigation.
Handling different types of input fields and controls.
// sampleTest.js (Selectors and Interactions)
module.exports = {
'Open Google on browser and perform Input value assertion' : function (browser) {
const googlePage = browser.page.googlePage();
googlePage
.navigate()
.setValue('@searchBar', 'nightwatch')
.click('@searchButton')
.pause(1000)
.assert.containsText('@searchResults', 'Nightwatch.js');
}
};
6. Configuring Test Environments and Parallel Execution:
Setting up test environments for different browsers and devices.
Configuring Nightwatch.js to run tests in parallel for faster execution.
Leveraging cloud testing platforms for cross-browser testing.
// nightwatch.conf.js module.exports = { src_folders: ['tests'], webdriver: { start_process: true, server_path: 'node_modules/.bin/chromedriver', port: 9515, }, test_settings: { default: { desiredCapabilities: { browserName: 'chrome', }, }, firefox: { desiredCapabilities: { browserName: 'firefox', }, }, safari: { desiredCapabilities: { browserName: 'safari', }, }, parallel: true, // Enable parallel execution }, };
7. Integrating Reporting and Continuous Integration:
Generating detailed test reports for actionable insights.
Integrating Nightwatch.js with popular CI/CD tools like Jenkins or Travis CI.
Automating test execution as part of the build pipeline.
// nightwatch.conf.js const HtmlReporter = require('nightwatch-html-reporter'); const path = require('path'); module.exports = { src_folders: ['tests'], webdriver: { start_process: true, server_path: 'node_modules/.bin/chromedriver', port: 9515, }, test_settings: { default: { desiredCapabilities: { browserName: 'chrome', }, }, parallel: true, }, reporter: (output, _module, _browser) => { const htmlReporter = new HtmlReporter({ openBrowser: true, reportsDirectory: path.join(__dirname, 'reports'), uniqueFilename: true, reportFilename: 'index.html', }); htmlReporter.fn(output, (error) => { if (error) { console.error('Failed to generate HTML report:', error); } else { console.log('HTML report generated successfully.'); } }); }, };
8. Best Practices and Tips for Successful Automation:
Following best practices for writing maintainable and scalable test suites.
Handling common challenges in UI automation with Nightwatch.js.
Tips for debugging and troubleshooting test failures effectively.
9. Conclusion:
In conclusion, Nightwatch.js empowers developers and QA engineers to build robust UI automation frameworks that ensure the quality and reliability of web applications. By mastering the principles and techniques outlined in this guide, teams can accelerate their testing efforts and deliver high-quality software with confidence.
10. References:
Subscribe to my newsletter
Read articles from Pratik Zinjurde directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
