Introduction to Algorithms
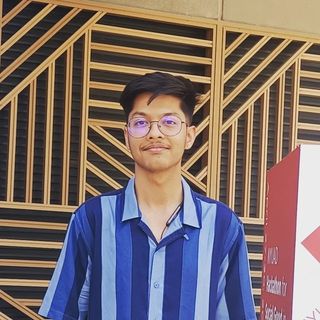
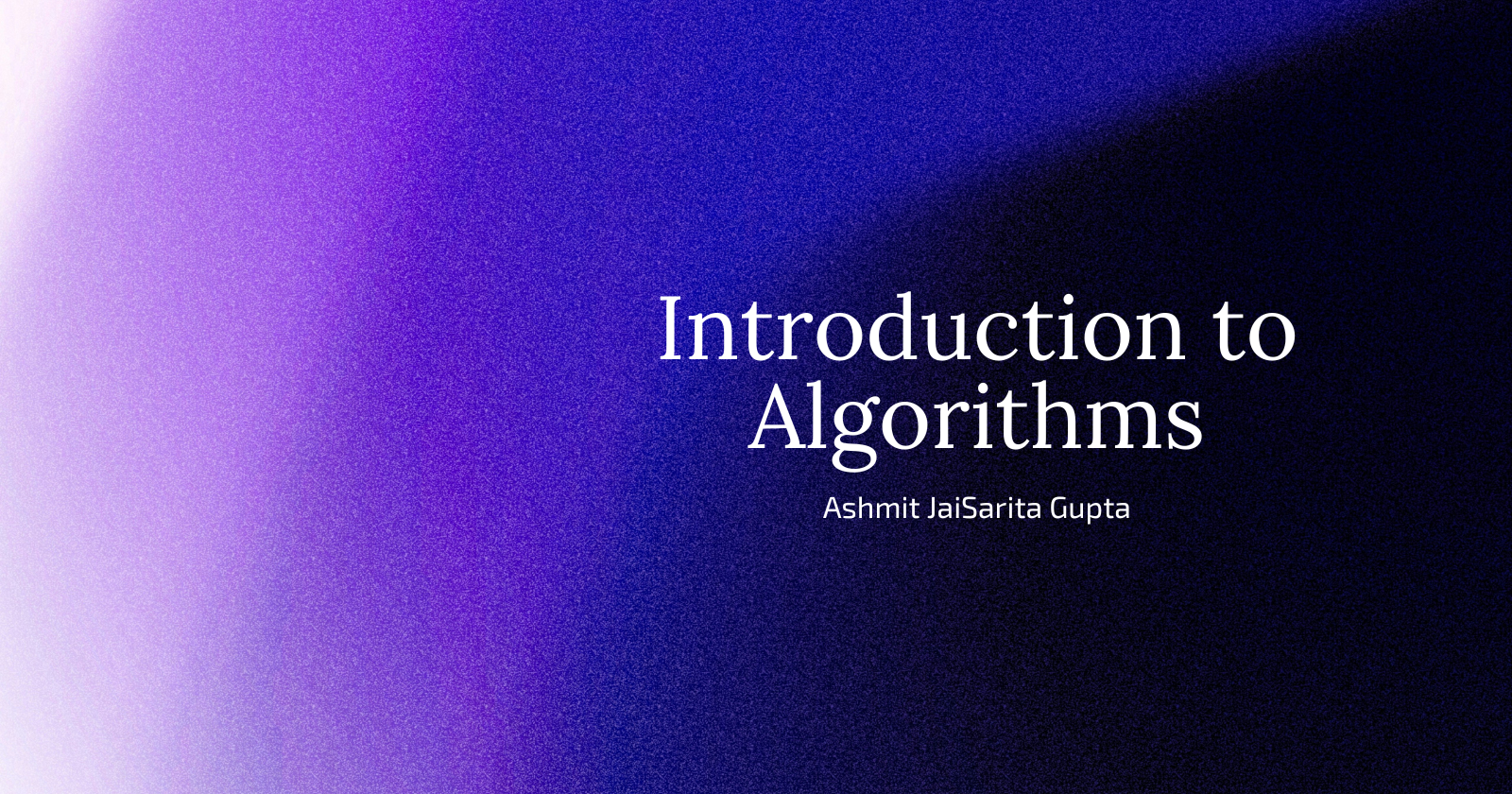
An algorithm can be defined as a step-by-step process of solving a problem. The problem can be from any area, including math, physics, computer science, and daily life. In this blog, I'll talk about how to analyze any algorithm in computer science.
Note: This blog is part of my journey to understand data structures and algorithms, and it is the culmination of my learning notes. I am not an expert, but I will try to be as accurate as possible.
In this blog, we will cover the following topics:
Difference between algorithm and program
Characteristics of algorithm
How to write an algorithm
An example of writing an algorithm
Different types of analysis
Key Terms Explained
Here's a quick explanation of some key terms you'll encounter in this blog post:
Algorithm: A step-by-step process for solving a problem or completing a task. It can be written in plain English, pseudocode, or flowcharts.
Program: The actual implementation of an algorithm in a specific programming language, designed to run on a computer.
Pseudocode: A way of writing algorithms using keywords that resemble programming languages, but are not specific to any particular language. It's easier to understand than formal code but more precise than natural language.
Input: The data or information provided to an algorithm to work with.
Output: The result or answer generated by an algorithm after processing the input.
Time Complexity: Describes how the execution time of an algorithm changes as the size of its input increases. Common notations include Big O notation (O).
Space Complexity: Describes how the memory usage of an algorithm changes as the size of its input increases. Common notations include Big O notation (O).
Difference between algorithm and program
While we've established what an algorithm is, you might wonder how it connects to the software we use daily. Here's where the concept of a program comes in:
Algorithm | Program |
It is the design of the solution. | It is the implementation of the solution. |
It can be written in human language. | It is only written in a programming language. |
It is independent of hardware and operating system. | It is hardware-dependent and may include an operating system. |
Characteristics of Algorithm
Every well-defined algorithm possesses certain essential characteristics. Let's delve into these key features to understand what makes an algorithm effective:
Feature | Description |
Input | An algorithm can have zero or more inputs. |
Output | An algorithm must generate at least one result. |
Definiteness | Every statement in the algorithm should be non-ambiguous. |
Finiteness | Algorithms should have a limited number of steps. |
Effectiveness | Every statement in an algorithm must perform some task. |
Feasible | An algorithm should be feasible to implement and give results using as low resources as it can. |
How to write an algorithm
Writing an algorithm involves specifying a set of steps or instructions to solve a particular problem or perform a specific task. It can be written in any simple human-readable format like English, Hindi, Pseudocode, or flowcharts. In this series of blogs, I will mostly use pseudocode.
Here is a general guideline on writing an algorithm:
Step 1: Define the problem
Clearly understand the problem you are trying to solve.
Identify the inputs and outputs of the algorithm.
Step 2: Understand the Constraints
- Consider any constraints or limitations that might affect your algorithm.
Step 3: Break Down the Problem
- Divide the problem into smaller, more manageable sub-problems or tasks.
Step 4: Outline and define the steps.
Start with a high-level overview of the steps needed to solve the problem.
Use pseudocode or natural language to describe the steps initially.
Step 5: Analyze and refine
Example: Finding the Largest Number
Let's illustrate the steps of writing an algorithm using a simple example: finding the largest number in a list of numbers.
Step 1: Define the problem
Problem: Find the largest number in a given list of numbers.
Inputs: A list of numbers (e.g., [5, 3, 8, 1, 9]).
Output: The largest number in the list (e.g., 9).
Step 2: Understand the constraints
- Assume the list contains only numerical values.
Step 3: Break down the problem
We can iterate through the list and compare each element with a variable initially set to the first element (assuming it's the largest).
If any element is found to be larger, we update the variable to hold that value.
Step 4: Outline and define the steps
Here's the algorithm in pseudocode:
function findLargest(numbers):
largest = numbers[0]
for i in range(1, len(numbers)):
if numbers[i] > largest:
largest = numbers[i]
return largest
Step 5: Analyze and refine
This is a basic and efficient algorithm for finding the largest number. We can further analyze its time complexity, which in this case is O(n), where n is the number of elements in the list. This means the execution time increases linearly with the input size.
This example demonstrates the process of breaking down a problem into steps, outlining them in an easy-to-understand format (pseudocode), and briefly analyzing its efficiency.
Different types of analysis
Here's the clear distinction between the different types of analysis used in computer science we will be doing on algorithms in our future blog posts of this series:
Analysis/Testing Type | Applies to | Analyzes | Focus |
Time Complexity Analysis | Algorithm | Theoretical time required | Efficiency in terms of input size |
Space Complexity Analysis | Algorithm | Theoretical memory required | Efficiency in terms of input size |
Testing | Program | Actual execution time and memory usage | Real-world performance under specific conditions |
That's a Wrap!
This blog post has been an introduction to algorithms in computer science. Remember, this journey is an ongoing exploration, and learning is an iterative process. As I continue my quest to understand data structures and algorithms, I'll share more insights and practical examples in future posts. In the next blog post in this series, I will write about how simple algorithms have loops and conditional statements.
Feel free to leave comments below with any questions or suggestions. Happy learning!
I will love to express my gratitude to Abdul Bari Sir from whose tutorials I studied this topic. ❤️
Cover Image Background Credit: Photo by MagicPatternon Unsplash
About the author
I am Ashmit JaiSarita Gupta, an engineering physics undergraduate at the National Institute of Technology Hamirpur. I am passionate about Web Development and Quantum Computing. I am currently exploring Data Structures and algorithms and spend my free time contributing to open-source (mostly at AsyncAPI and Uptane). Visit my portfolio website to learn more about me, my previous projects, and the places I have worked. Feel free to connect with me on Twitter, LinkedIn, or GitHub.
Subscribe to my newsletter
Read articles from Ashmit JaiSarita Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
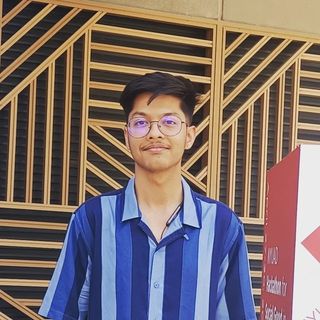
Ashmit JaiSarita Gupta
Ashmit JaiSarita Gupta
I am an engineering physics undergraduate passionate about Quantum Computing, Machine Learning, UI/UX, and Web Development. About two years ago, when I first discovered the field of Web Development and Quantum Computing, it totally amazed me and I have been dedicating my education to them ever since. Over the past two years, I have dedicated a considerable amount of time and effort to learning and developing skills in these fields by taking various online courses, reading different articles, making several projects, and being involved in various research internships and mentorship programs. Fast forward to today, I am currently working on a modern streaming platform and researching QUBO Relaxation Parameter Optimisation using Learning Surrogate Solver (QROSS).