Day 6 : what is an Math Methods And Number Methods in javascript ?

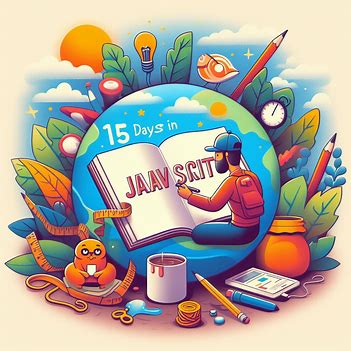
The syntax for Math any methods is : Math.method(number)
Math.round():-
Math.round(x) returns the nearest integer.
Example:-
Math.round(4.6); // 5
Math.round(4.3); // 4
Math.ceil():-
Math.ceil(x) returns the value of x rounded up to its nearest integer. Example:-
Math.ceil(4.6); // 5
Math.ceil(4.2); // 5
Math.ceil(-4.3); // -4
Math.floor():-
Math.floor(x) returns the value of x rounded down to its nearest integer. Example:-
Math.floor(4.6); // 4
Math.floor(4.2); // 4
Math.floor(-4.3); // -5
Math.trunc():-
Math.trunc(x) returns the integer part of x.
Example:-
Math.trunc(4.6); // 4
Math.trunc(4.2); // 4
Math.sign():-
Math.sign(x) returns if x is negative, null or positive.
Example:-
Math.sign(-4); // -1
Math.sign(0); // 0
Math.sign(4); // 1
Math.pow():-
Math.pow(x, y) returns the value of x to the power of y.
Example:-
Math.pow(2, 3); // 8
Math.pow(8, 2); // 64
Math.sqrt():-
Math.sqrt(x) returns the square root of x.
Example:-
Math.sqrt(16); // 4
Math.sqrt(121); // 11
Math.abs():-
Math.abs(x) returns the absolute (positive) value of x.
Example:-
Math.abs(-6); // 6
Math.abs(1.2); // 1.2
Math.min() and Math.max():-
Math.min() and Math.max() can be used to find the lowest or highest value in a list of arguments.
Example:-
Math.min(0, 150, 30, 20, -8, -200); // -200
Math.max(0, 150, 30, 20, -8, -200); // 150
Math.random():-
Math.random() returns a random number between 0 (inclusive), and 1 (exclusive). Example:-
Math.random(); // 0.3860669187533996
Math.sin():-
Math.sin(x) returns the sine (a value between -1 and 1) of the angle x (given in radians).
If you want to use degrees instead of radians, you have to convert degrees to radians:
Angle in radians = Angle in degrees x PI / 180.
Example:-
Math.sin(90 Math.PI / 180); // 1 (the sine of 90 degrees) Math.sin(30 Math.PI / 180); // 0.5 (the sine of 30 degrees)
Math.cos():-
Math.cos(x) returns the cosine (a value between -1 and 1) of the angle x (given in radians).
If you want to use degrees instead of radians, you have to convert degrees to radians:
Angle in radians = Angle in degrees x PI / 180.
Example:-
Math.cos(0 Math.PI / 180); // 1 (the cos of 0 degrees) Math.cos(60 Math.PI / 180); // 0.5 (the cos of 60 degrees)
Math.log():-
Math.log(x) returns the natural logarithm of x.
The natural logarithm returns the time needed to reach a certain level of growth. Example:-
Math.log(1); // 0
Math.log(2); // 0.6931471805599453
Math.log(10); // 2.302585092994046
Math.log2():-
Math.log2(x) returns the base 2 logarithm of x.
Example:-
Math.log2(8); // 3
Math.log10():-
Math.log10(x) returns the base 10 logarithm of x. Example:-
Math.log10(1000);// 3
Number Method
These number methods can be used on all JavaScript numbers:
Method | Description |
toString() | Returns a number as a string |
toExponential() | Returns a number written in exponential notation |
toFixed() | Returns a number written with a number of decimals |
toPrecision() | Returns a number written with a specified length |
Valueof() | Returns a number as a number |
Example:-
let x = 123;
x.toString(); // 123
(123).toString();// 123
(100 + 23).toString(); // 123
let x = 9.656;
x.toExponential(); // 9.656e+0
x.toExponential(2); // 9.66e+0
x.toExponential(4); // 9.6560e+0
x.toExponential(6); // 9.656000e+0
let x = 9.656;
x.toFixed(); // 10
x.toFixed(2); // 9.66
x.toFixed(4); // 9.6560
x.toFixed(6); // 9.656000
let x = 9.656;
x.toPrecision(); // 9.656
x.toPrecision(2);// 9.7
x.toPrecision(4);// 9.656
x.toPrecision(6);// 9.65600
let x = 123;
x.ValueOf(); // 123
(123).ValueOf(); // 123
(100 + 23).ValueOf(); // 123
In JavaScript, a number can be a primitive value (typeof = number) or an object (typeof = object).
The valueOf() method is used internally in JavaScript to convert Number objects to primitive values.
Subscribe to my newsletter
Read articles from Jemin Kikani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
