React Router Dom For Beginners
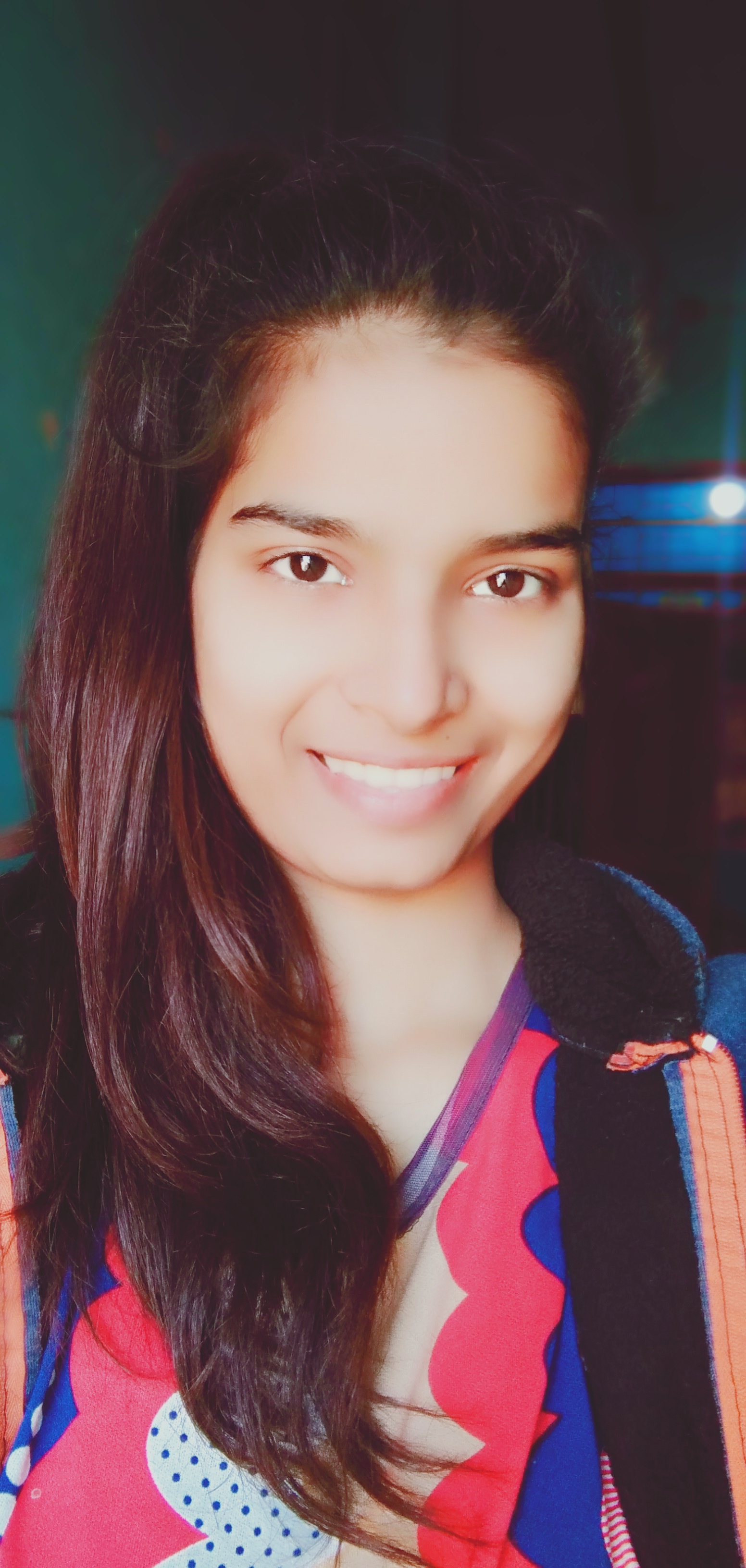
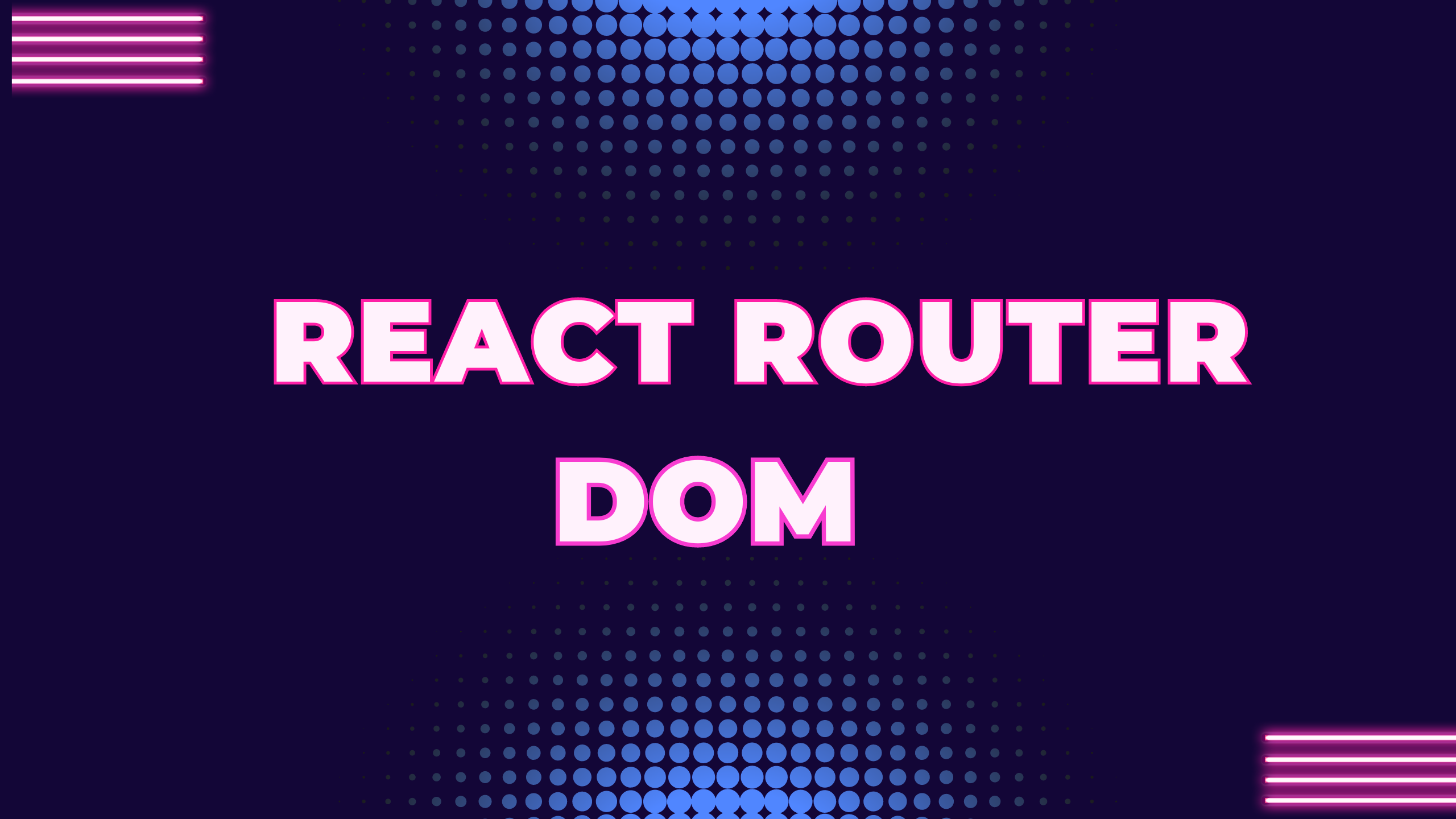
React Router is a popular library for handling routing in React applications. The react-router-dom
package is specifically designed for web applications. It allows you to create single-page applications with navigation and multiple views without a full page reload.
Here's a basic guide to get you started:
Step 1: Installreact-router-dom
Make sure you have React installed, and then install react-router-dom
:
npm install react-router-dom
Step 2: Set Up Routes
In your main application file (often App.js
), import BrowserRouter
and Route
from react-router-dom
:
// App.js
import React from 'react';
import { BrowserRouter as Router, Route } from 'react-router-dom';
Wrap your entire application with the Router
component:
// App.js
function App() {
return (
<Router>
{/* Routes will go here */}
</Router>
);
}
export default App;
Step 3: Define Routes:
Inside the Router
, you can define different routes using the Route
component. Each Route
component takes a path
prop and a component
prop.
// App.js
import React from 'react';
import { BrowserRouter as Router, Route } from 'react-router-dom';
import Home from './components/Home';
import About from './components/About';
import Contact from './components/Contact';
function App() {
return (
<Router>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
</Router>
);
}
export default App;
Step 4: Create Components :
Create the components for each route. For example:
// Home.js
import React from 'react';
const Home = () => {
return <div>Home Page</div>;
}
export default Home;
Step 5: Add Navigation:
You can use the Link
component to create navigation links:
// App.js
import { BrowserRouter as Router, Route, Link } from 'react-router-dom';
function App() {
return (
<Router>
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
<li>
<Link to="/contact">Contact</Link>
</li>
</ul>
</nav>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
</Router>
);
}
Step 6: Run Your App :
npm start
Visit http://localhost:3000
(or another port if specified) in your browser, you should see your basic React application with navigation.
✨This is a simple setup, and React Router provides many more features for handling complex routing scenarios, such as nested routes, route parameters, and more.
I'll explain all the stuff in the next post.
Thank you for reading this beautiful content.
Subscribe to my newsletter
Read articles from Kumari Ranjana Yadav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
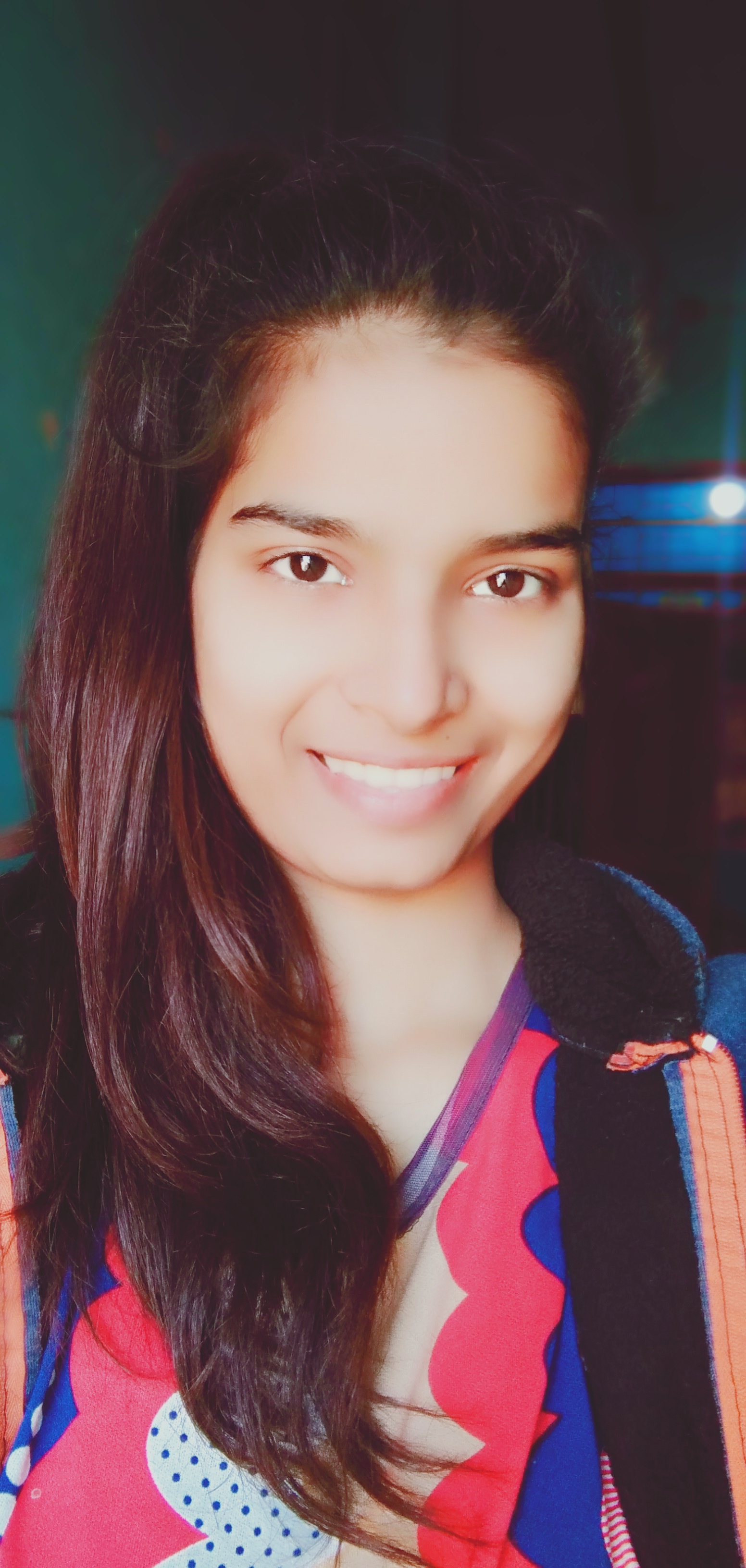
Kumari Ranjana Yadav
Kumari Ranjana Yadav
Passionate full-stack developer @MERN stack