โ Simplify Your Node.js Backend Workflow: TypeScript, Express, ESLint

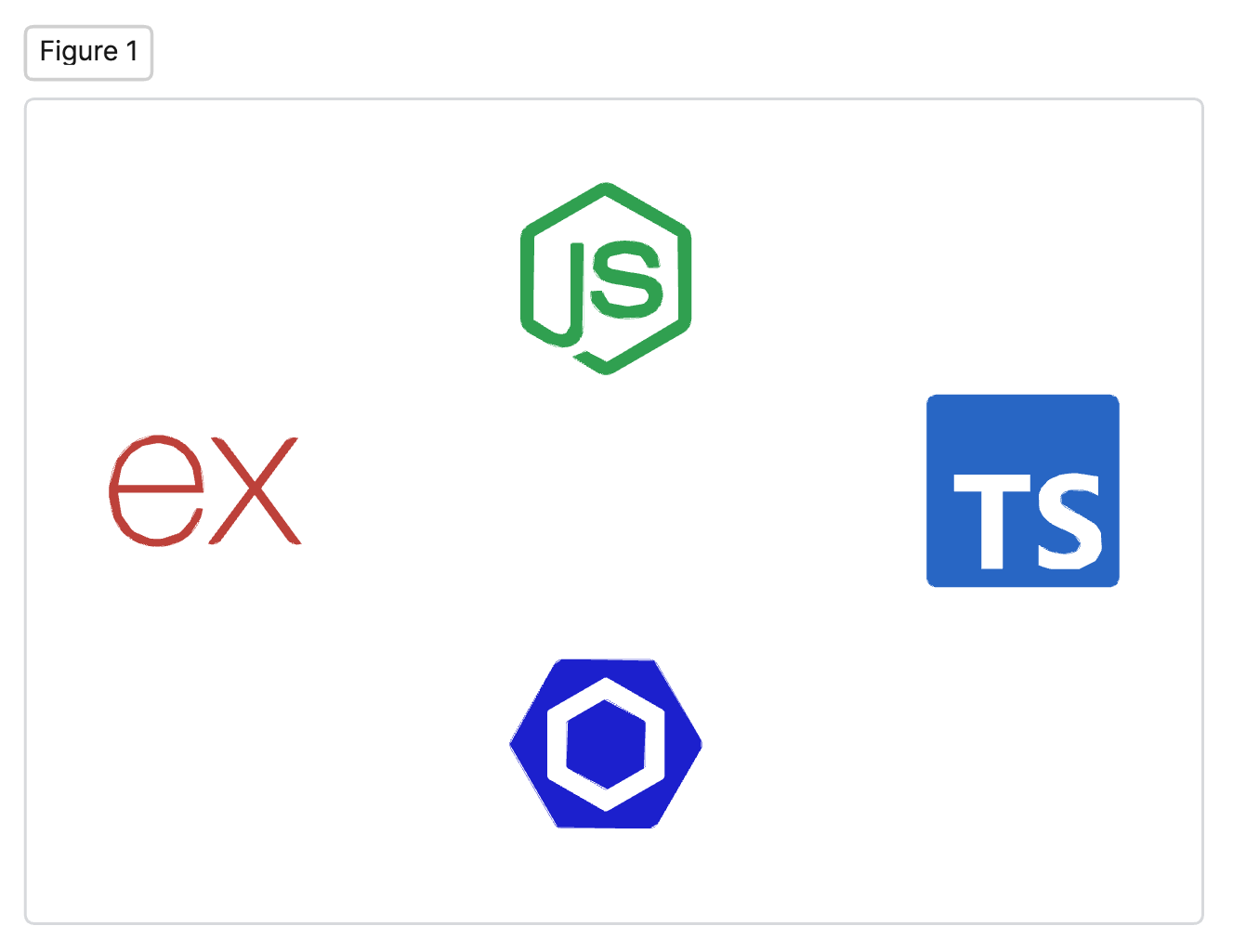
This blog describes briefly about how to setup environment for backend project.
Before you setup the backend environment I assume node
is already installed on your system. If not click here and download LTS (long term support) version. After that follow the below steps.
Create package.json
file
Initialise a new Node.js project by creating a
package.json
file with the commandnpm init -y
.npm init -y
Here
-y
flag automates the process, accepting default values for all prompts. Without-y
flagnpm init
would ask for a series of questions to provide information forpackage.json
file.package.json
file is essential for managingNode.js
project as it stores project metadata (name, author, version, etc), dependencies and scripts. You can always edit yourpackage.json
after it is created.
Setup Typescript
Install your first dependency
typescript
.npm install --save-dev typescript
After you run this command
typescript
will be installed and listed underdevDependencies
inpackage.json
file.There are two types of dependencies:
- dependencies: which are needed in both
development
andproduction
.- devDependencies: which are needed only during
development
.You can see a
node_modules
folder which contains packages which are installed, sotypescript
will be present inside this folder.After
typescript
is installed we need atsconfig
file which is a typescript configuration file which contains some information on howtypescript
should behave. It is same as like aconductor
inorchestra
who directs the performance with the movement of hands and arms, here it acts aconductor
and directstypescript
how to behave based on the configuration settings given.%[https://giphy.com/gifs/pudgypenguins-conductor-conducting-music-NMI3ndWANUmXBkC7tQ]
Run this command
npx tsc --init
npm
installs packagesnpx
executes packagestsc
is a typescript compiler
This command creates tsconfig.json
file. It contains lot of options
, most of them are optional so they are commented. There are some tsconfig bases
which provide set of default configuration settings tailored for specific environment. If you want to dig deep into this, check this repo. I will write a in-depth blog on bases
and tsconfig
options soon.
Tip: ๐ Shortcut for npm install
is npm i.
Sample tsconfig.json
file:
{
"compilerOptions":{
"module":"NodeNext",
"moduleResolution":"node",
"basUrl":"src",
"outDir":"dist",
"sourceMap":true,
"noImplicitAny":true,
},
"include":["src/**/*"] // recursively includes all files folders
// inside src folder
}
Now install
express
npm i express
In earlier versions we need to explictly install types for express
@types/express
, from theversion:4.17.0
it is not needed asexpress
includes its owntypescriptdefinition
files directly within itspackage
.
Setup nodemon and ts-node
Next: We need these two only in development
Install
nodemon
- This is a package which is used to automatically restart the server whenever we make changes to the code.npm i --save-dev nodemon
Install
ts-node
- This package transpiles the typescript code to javascript code.npm i --save-dev ts-node
Tip: ๐ Shortcut for
--save-dev
is-D
.Create a config file for nodemon
nodemon.json
which specifies settings to tailor the nodemon.Sample
nodemon.json
file:{ "watch": ["src"],//watch the directory should be same as in //tsconfig.json file "ext": ".ts,.js",//extensions "exec": "ts-node ./src/server.ts" }
Now add a command under
scripts
inpackage.json
."scripts":{ "start":"nodemon" }
Create a new file for example
server.ts
which will be the main entry point for the server and runnpm start
which starts theserver
, make changes to the file the server will automatically adopts to it because ofnodemon
andts-node
.
Setup ESLint
Next: Install
eslint
and create a configuration file for it.npm i -D eslint npx eslint --init
When you run this command it asks for some series of questions
Here we select
none of these
because we are using it in backendnode
environment.Select
package manager
of your choiceSample
eslintrc.js
config filemodule.exports = { "env": { "es2021": true, "node": true }, "extends": [ "eslint:recommended", "plugin:@typescript-eslint/recommended" ], "overrides": [ { "env": { "node": true }, "files": [ ".eslintrc.{js,cjs}" ], "parserOptions": { "sourceType": "script" } } ], "parser": "@typescript-eslint/parser", }
Now add
lint
script inpackage.json
"scripts":{ "start": "nodemon", "lint": "eslint . --ext .ts", }
Now run
npm run lint
command to run eslint to check for any problems in the code. There is additionally vscode extension eslint which shows up warnings directly in our code without need to run from command line. But we need to run this from command line before building the project for production to catch any potential problems.
Setup Prettier
Bonus ๐ : Setup
prettier
Firstly install it as a devDependency
npm i -D prettier
Create
.prettierrc
fileExample: { "singleQuote": false, "bracketSpacing": true, "tabWidth": 2, "trailingComma": "es5", "semi": true }
Mainly
prettier
used to format code with a given config settings in entire project. In large code bases when several developers are working on same projectformatting
rules are required so that entire code base isconsistent
. Change settings from above example file explore various options to configure. There is also a vscode extension called prettier, which can also be used for formatting. Check below flow for better understanding of howprettier
works.The command line comes into use when it is needed to format entire codebase at once, in other cases prettier extension is fine.
This is a sample pipeline for production grade typescript applications
Every tool requires its own config file to specify configuration settings to tailor its behaviour. These are not the only tools available, there are many tools to select from for each step. Explore online for more options. I will write in-depth blog about
production grade build pipelines
soon.
-----------------------------------------------------------------------------------
Hope this makes you feel comfortable with the setup for production grade node.js typescript backend project. Google or ask chatGPT the different options present to configure each tool mentioned. Feel free to reach out to me for any questions!
Happy learning :)
Subscribe to my newsletter
Read articles from Yashwanth K directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
