Leveraging useInterval in React and Flask: Keeping Frontend Data Fresh
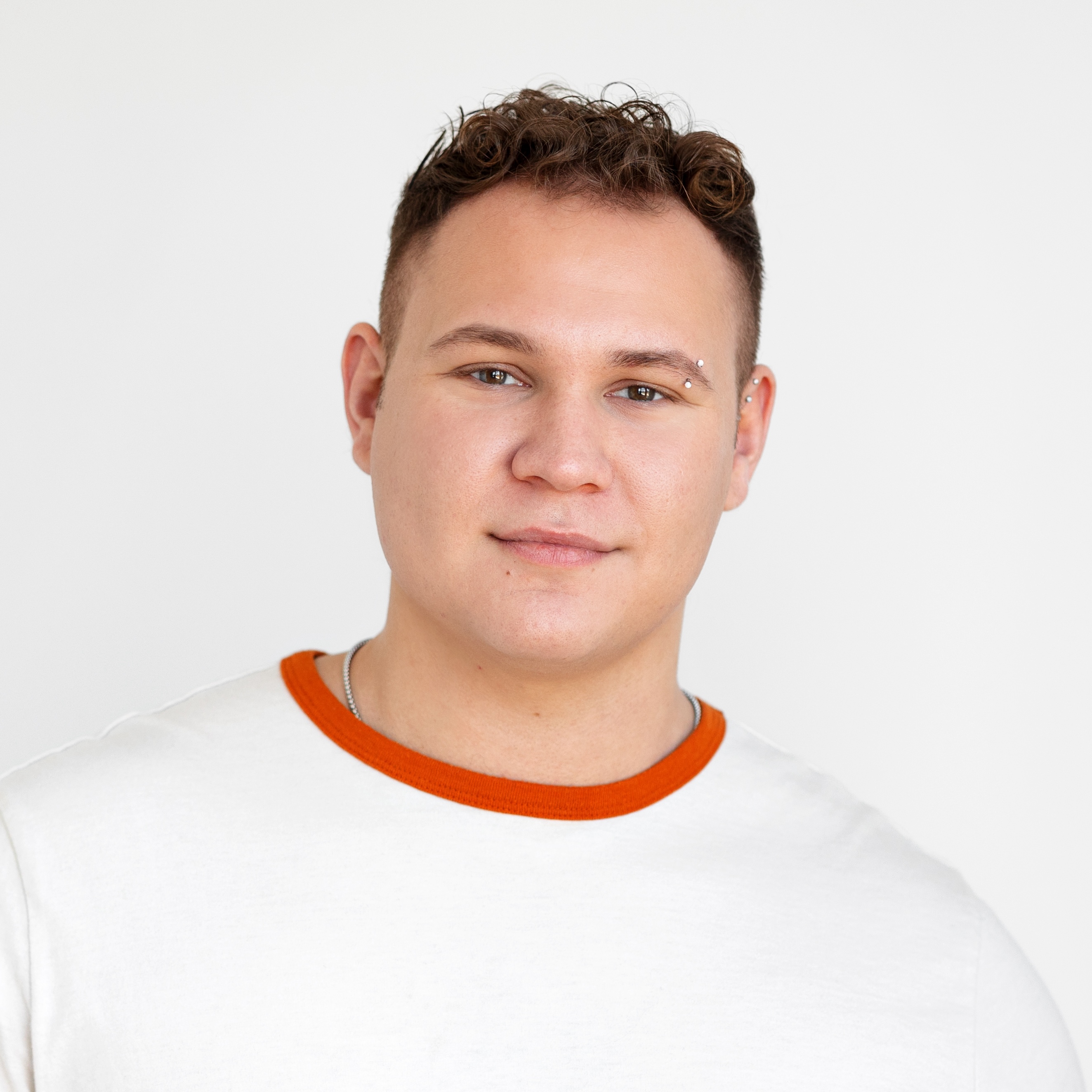
In the realm of modern web development, real-time data updates are not just a luxury but often a necessity. Whether it's social media feeds, stock prices, or chat applications, users expect information to be up-to-date without constant manual refreshing. In the context of a full-stack application built with React on the frontend and Flask on the backend, achieving this real-time data synchronization can be efficiently accomplished with the use of useInterval
.
What is useInterval
?
useInterval
is a custom React Hook that enables developers to execute a specified function at a regular interval. Unlike setInterval
, which is a built-in JavaScript function, useInterval
is designed to work seamlessly with React's component lifecycle, ensuring proper handling of effects and cleanup.
The Need for Real-Time Updates
Consider a scenario where you're building a social media dashboard using React for the frontend and Flask, a Python micro-framework, for the backend. Users expect to see the latest posts and notifications without having to manually refresh the page. This is where real-time updates come into play.
Intermittent Fetch Requests
To achieve real-time updates, we can utilize useInterval
to periodically fetch new data from the backend. In our Flask application, we would expose an endpoint that serves the latest data, such as new posts or notifications.
@app.route('/api/data') def get_data():
# Fetch and return the latest data from the database
# This could be posts, notifications, or any other relevant information
Implementing useInterval
in React
In our React frontend, we can use useInterval
to periodically make requests to the Flask backend and update the UI with the latest data. Here's how we can implement it:
import React, { useState, useEffect } from 'react';
const App = () => {
const [data, setData] = useState([]);
useEffect(() => {
fetchData(); // Fetch data initially when the component mounts
}, []);
useInterval(() => {
fetchData(); // Fetch data at regular intervals
}, 5000); // Fetch data every 5 seconds
const fetchData = async () => {
try {
const response = await fetch('/api/data');
const newData = await response.json();
setData(newData);
} catch (error) {
console.error('Error fetching data:', error);
}
};
return (
<div>
{/* Render the data in the UI */}
</div>
);
};
export default App;
Benefits of useInterval
Efficiency: Unlike continuous polling,
useInterval
allows us to control the frequency of data updates, preventing unnecessary server load.Consistency: By fetching data at regular intervals, we ensure that users always have access to the latest information without manual intervention.
Scalability: This approach scales well with larger applications and ensures smooth real-time updates across the board.
Conclusion
In summary, useInterval
is a powerful tool in the React developer's arsenal for implementing real-time updates in full-stack applications. By combining it with Flask on the backend, we can create dynamic, responsive web applications that meet the expectations of modern users. Whether it's social media feeds, live notifications, or data dashboards, the use of useInterval
enables us to keep our frontend interfaces fresh and engaging, providing a seamless user experience.
Subscribe to my newsletter
Read articles from Steven Mentzer directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
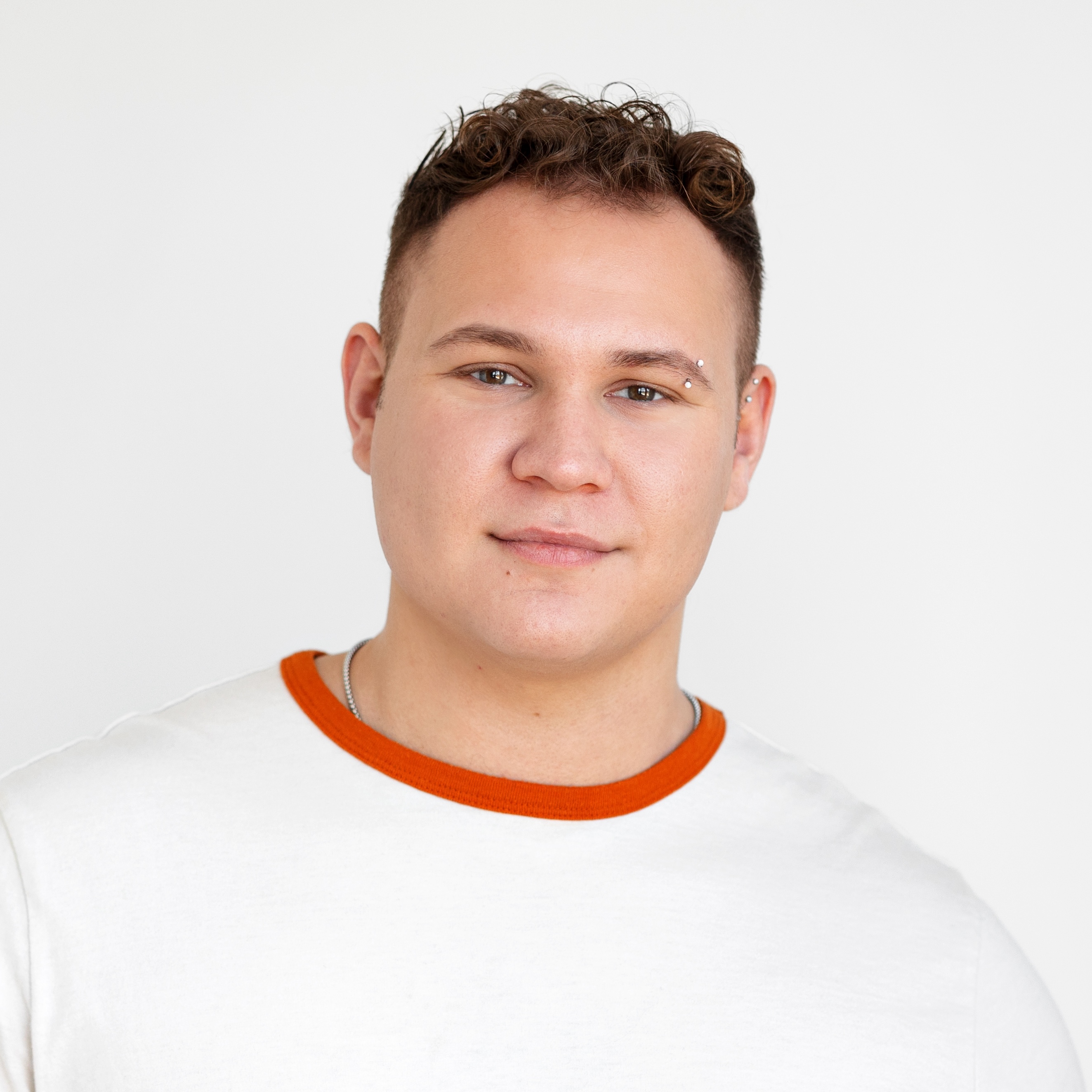
Steven Mentzer
Steven Mentzer
Hey there! ๐ Full-stack software engineer passionate about art and tech, crafting innovative solutions for today's dynamic landscape. BA in Studio Art ๐จ & cert. in Software Engineering ๐ป. Love blending creativity with precision! Currently honing skills in Swift ๐ฑ & mobile design. Let's collaborate on cool projects! ๐ก Also into weightlifting ๐๏ธโโ๏ธ, queer history ๐ณ๏ธโ๐, and board games ๐งฉ. Writing this blog to chronicle my journey into software engineering, aiming to both track my own growth and provide insights for fellow learners. Let's embark on this coding adventure together, learning and growing every step of the way!