Understanding the Web Storage API:

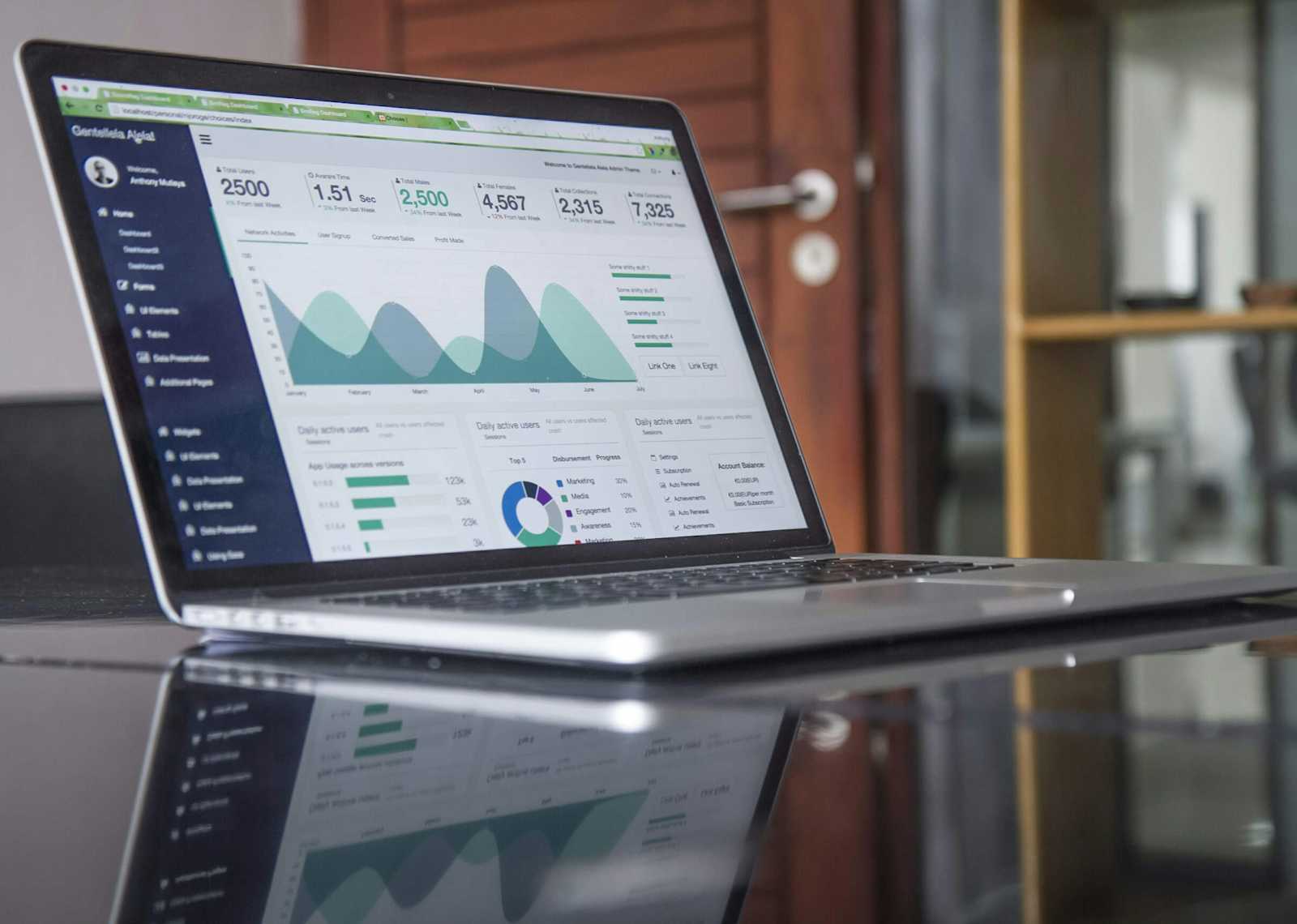
In the realm of web development, efficiently managing data storage on the client side is crucial for creating dynamic, user-friendly interfaces. The Web Storage API offers a powerful and intuitive solution, allowing web applications to store data locally within the user's browser. Here we will delve into the core concepts of the Web Storage API, its mechanisms (sessionStorage and localStorage), and practical examples to illuminate its usage.
Core Concepts and Mechanisms
The Web Storage API provides two primary storage mechanisms:
sessionStorage
Scope: It is limited to the duration of the page session, persisting across page reloads and restores until the browser or tab is closed.
Storage Lifecycle: Data stored with sessionStorage does not persist beyond the current browser session.
Server Interaction: This data is never transferred to the server.
Storage Limit: Typically allows for up to 5MB of data, significantly more than cookies.
localStorage
Scope: Unlike sessionStorage, localStorage data persists even after the browser is closed and reopened.
Storage Lifecycle: Data has no expiration date and can only be cleared programmatically via JavaScript or by the user clearing the browser's cache.
Storage Limit: Provides the maximum storage capacity of the two, often more than 5MB.
Both mechanisms operate on a per-origin (domain) basis, ensuring that data stored by one origin cannot be accessed by another. They are accessible via Window.sessionStorage
and Window.localStorage
properties, each returning an instance of the Storage object. This object facilitates setting, retrieving, and removing key/value pairs.
Special Considerations
Browser Compatibility: Notably, in browsers like Firefox, the amount of data that can be saved per origin is capped at 10MB to prevent memory issues.
Third-Party Access: Web Storage access from third-party iframes is blocked if the user has disabled third-party cookies, impacting how embedded content can interact with Web Storage.
Determining Third-Party Storage Access
The access third-party code has to storage depends significantly on its browsing context. For instance:
When third-party code is injected via a
<script>
tag, it shares the storage access of the host page.Conversely, when third-party code runs within an
<iframe>
, it operates under the origin of the<iframe>
, affecting how it can access and manipulate storage.
Web Storage Interfaces
Storage: This interface allows manipulation (set, retrieve, remove) of data for a specific domain and storage type.
Window: The API extends the Window object with
sessionStorage
andlocalStorage
properties for accessing storage objects.StorageEvent: This event triggers on the Window object when changes occur within the storage area, facilitating reactive programming patterns.
Real-Life Example:
Imagine an e-commerce website, "ShopSmart," which allows users to customize their browsing experience by setting preferences such as theme color, language, and product categories of interest. These preferences enhance user experience by tailoring the site's appearance and the products displayed to match individual tastes and needs. To make these preferences persistent and immediately available upon the user's return without requiring server storage or processing, ShopSmart utilizes the Web Storage API, specifically localStorage
.
Implementation Example
When a user selects their preferred theme color, the website executes the following JavaScript code:
// Set the user's theme preference in localStorage
localStorage.setItem('themeColor', 'dark');
// Apply the theme
document.documentElement.style.setProperty('--theme-color', 'dark');
Similarly, when the user chooses their preferred language and categories, ShopSmart stores these preferences:
localStorage.setItem('language', 'English');
localStorage.setItem('categories', JSON.stringify(['electronics', 'books']));
Upon returning to the site or refreshing the page, ShopSmart reads these preferences and applies them immediately:
// Retrieve and apply the theme from localStorage
const themeColor = localStorage.getItem('themeColor');
if (themeColor) {
document.documentElement.style.setProperty('--theme-color', themeColor);
}
// Other preferences can be applied in a similar manner
Importance in Security
While the Web Storage API offers significant convenience and enhances user experience, it also introduces potential security concerns that developers must address:
Cross-Site Scripting (XSS): Since Web Storage can store sensitive information, an XSS attack could allow an attacker to retrieve this data using malicious scripts. To mitigate this, ShopSmart sanitizes all user input to prevent the insertion and execution of malicious scripts. Furthermore, implementing Content Security Policy (CSP) headers can help reduce the risk of XSS attacks.
Secure Transmission: Although Web Storage data is never transmitted to the server as part of HTTP requests, ensuring that all communication between the client and server is encrypted using HTTPS is crucial. This prevents man-in-the-middle attacks that could intercept or modify data in transit.
Access by Third-Party Scripts: ShopSmart carefully evaluates and restricts third-party scripts to prevent unauthorized access to Web Storage data. Using subresource integrity (SRI) tags ensures that only the intended, unaltered scripts are executed.
Data Integrity and Confidentiality: For more sensitive preferences or data, ShopSmart could implement additional layers of security, such as encrypting data before storing it in localStorage. This way, even if the data is accessed, it remains unreadable without the proper decryption key, which is not stored in Web Storage.
The Web Storage API significantly empowers web developers to create more engaging, efficient, and personalized web applications. By leveraging sessionStorage
for transient data and localStorage
for persistent information, developers can offer users enriched interactions. Nonetheless, the implementation of Web Storage must be accompanied by diligent security practices, including input sanitization, secure data transmission, cautious third-party script integration, and, when necessary, data encryption. By adhering to these security measures, developers can fully harness the potential of the Web Storage API, delivering sophisticated web applications while ensuring user data is protected and secure.
Summary:
The Web Storage API allows web applications to store data locally in the user's browser, enhancing user experience through sessionStorage
and localStorage
. It ensures data persistence and security, tailored for dynamic, user-friendly interfaces without server interaction. Key considerations include security practices against XSS, secure transmission, and careful third-party script management.
Subscribe to my newsletter
Read articles from SANKALP HARITASH directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

SANKALP HARITASH
SANKALP HARITASH
Hey ๐๐ป, I am , a Software Engineer from India. I am interested in, write about, and develop (open source) software solutions for and with JavaScript, ReactJs. ๐ฌ Get in touch Twitter: https://x.com/SankalpHaritash Blog: https://sankalp-haritash.hashnode.dev/ LinkedIn: https://www.linkedin.com/in/sankalp-haritash/ GitHub: https://github.com/SankalpHaritash21 ๐ง Sign up for my newsletter: https://sankalp-haritash.hashnode.dev/newsletter