Python bytes Data Type with Example
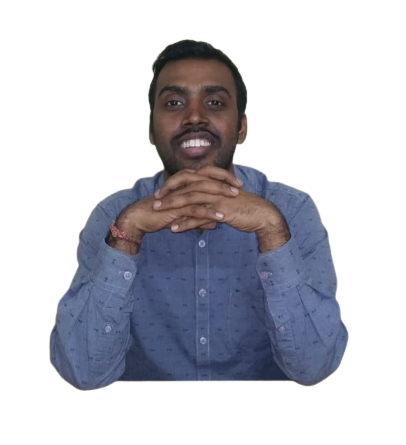
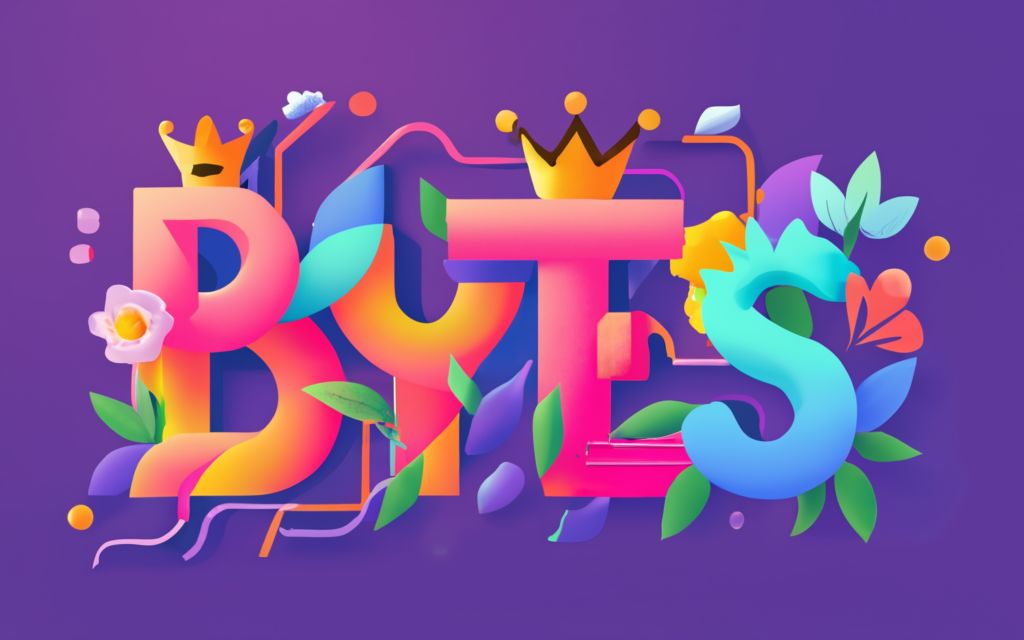
The bytes
data type in Python is a series of bytes. It's unchangeable, like a tuple or a string. Bytes are usually for storing binary data, like file contents or network information. Here's a sample of using the bytes
data type in Python:
# Creating a bytes object from a sequence of integers (0 to 255)
byte_data = bytes([65, 66, 67, 68, 69])
print(byte_data) # Output: b'ABCDE'
# Creating a bytes object from a string using the encode() method
str_data = "Hello, bytes!"
byte_data_from_str = str_data.encode('utf-8')
print(byte_data_from_str) # Output: b'Hello, bytes!'
# Accessing individual bytes in a bytes object
print(byte_data[0]) # Output: 65
# Iterating through the bytes
for byte in byte_data:
print(byte)
# Converting a bytes object back to a string using the decode() method
decoded_str = byte_data_from_str.decode('utf-8')
print(decoded_str) # Output: Hello, bytes!
In this example:
We create a
bytes
object from a sequence of integers representing ASCII values.Another
bytes
object is created by encoding a string using theencode()
method with the UTF-8 encoding.Individual bytes in a
bytes
object can be accessed using indexing.We iterate through the bytes using a loop.
Finally, we decode a
bytes
object back to a string using thedecode()
method.
Subscribe to my newsletter
Read articles from LingarajTechhub All About Programming directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
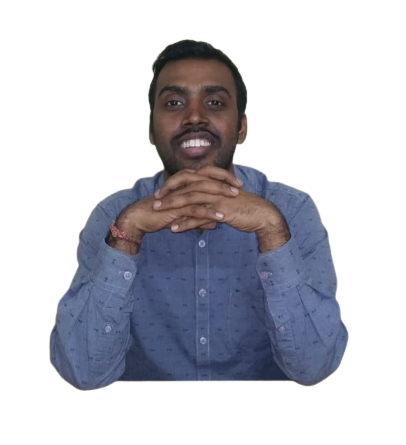
LingarajTechhub All About Programming
LingarajTechhub All About Programming
Corporate Software Development Trainer with a demonstrated track record of success in the IT and Ed-tech industries for product development. I started my career as a software developer and have since taught C, C++, Java, and Python to hundreds of IT enthusiasts, including corporate professionals, throughout the years. I have been developing software for over 12 years.