Understanding Exception Handling with Try-Catch Blocks in Programming
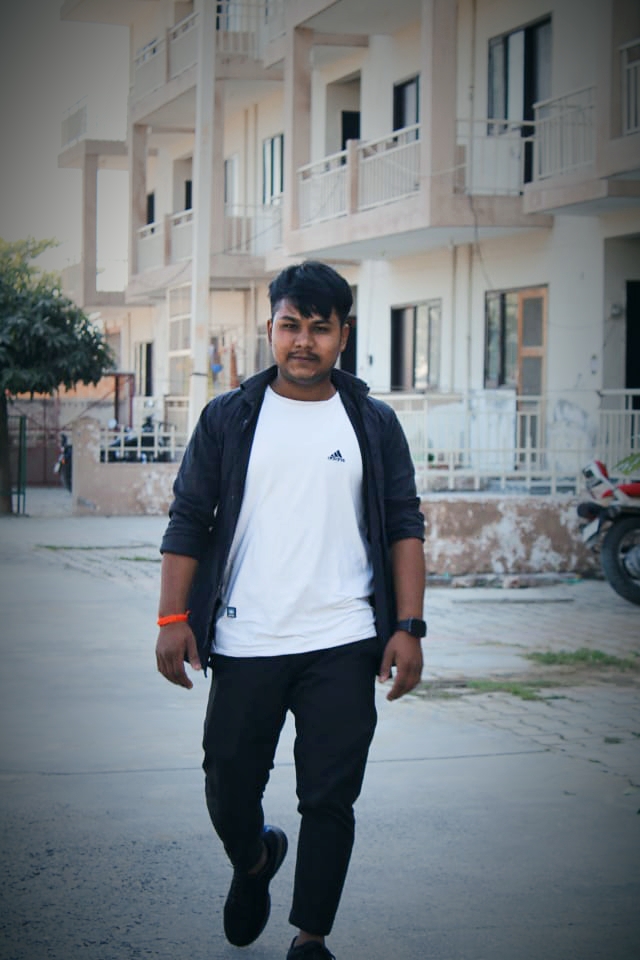
Exception handling is a crucial aspect of programming that allows developers to gracefully manage unexpected errors and failures in their code. Whether you're working with a simple script or a complex software application, incorporating robust error-handling mechanisms can greatly enhance the reliability and stability of your code. One commonly used method for managing exceptions is the "try-catch" block.
What is Exception Handling?
In programming, an exception is an event that disrupts the normal flow of a program's execution. Exceptions can occur for various reasons, such as invalid input, resource unavailability, or unexpected system behavior. Without proper handling, these exceptions can lead to crashes or undesired behavior, making it essential for developers to anticipate and manage them effectively.
Exception handling involves detecting and responding to exceptions in a controlled manner, ensuring that the program can recover gracefully from errors and continue executing as expected.
The Try-Catch Block
The try-catch block is a fundamental construct in many programming languages for implementing exception handling. It allows developers to isolate code that might throw an exception within a "try" block and specify how to handle any resulting exceptions in one or more "catch" blocks.
Here's the basic syntax of a try-catch block:
try {
// Code that might throw an exception
} catch (ExceptionType1 e1) {
// Handle ExceptionType1
} catch (ExceptionType2 e2) {
// Handle ExceptionType2
} finally {
//Optional: finally block for cleanup
}
The '
try'
block contains the code that is susceptible to throwing an exception.The
catch
block(s) specify how to handle exceptions of specific types. Each 'catch'
block is associated with a particular type of exception.Optionally, a '
finally'
block can be used to execute cleanup code that should always run, regardless of whether an exception occurred.
How Try-Catch Works
When a program encounters a try-catch block, it executes the code within the try block. If an exception occurs during the execution of this code, the runtime system looks for an appropriate catch block to handle the exception. It searches through the catch blocks in the order they are written until it finds one that matches the type of the thrown exception.
If a matching catch block is found, the exception is caught, and the corresponding catch block's code is executed. After executing the catch block, control usually transfers to the code following the try-catch block.
If no matching catch block is found within the current try-catch construct, the exception propagates up the call stack to the next enclosing try-catch block or the runtime system. If the exception remains uncaught, the program typically terminates, and an error message is displayed.
Best Practices
When using try-catch blocks, consider the following best practices:
Catch Specific Exceptions: Catch specific types of exceptions whenever possible to handle different error scenarios appropriately.
Keep Catch Blocks Concise: Keep the code inside catch blocks concise and focused on handling the exception. Avoid performing unrelated tasks within catch blocks.
Use Finally for Cleanup: Use the
finally
block for cleanup tasks such as releasing resources or closing connections, ensuring that critical cleanup code always executes, regardless of whether an exception occurs.Avoid Swallowing Exceptions: Avoid catching exceptions without handling them appropriately. Swallowing exceptions (i.e., catching exceptions without taking any action) can mask underlying issues and make debugging challenging.
Logging Exceptions: Consider logging exceptions to help diagnose issues and track the program's behavior during runtime.
Conclusion
Exception handling using try-catch blocks is an essential technique for writing robust and reliable code. By anticipating potential errors and implementing appropriate error-handling strategies, developers can improve the resilience and maintainability of their applications. Understanding how try-catch blocks work and following best practices for exception handling can greatly enhance the quality of software systems, leading to better user experiences and increased confidence in the reliability of the code.
Subscribe to my newsletter
Read articles from Arun kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
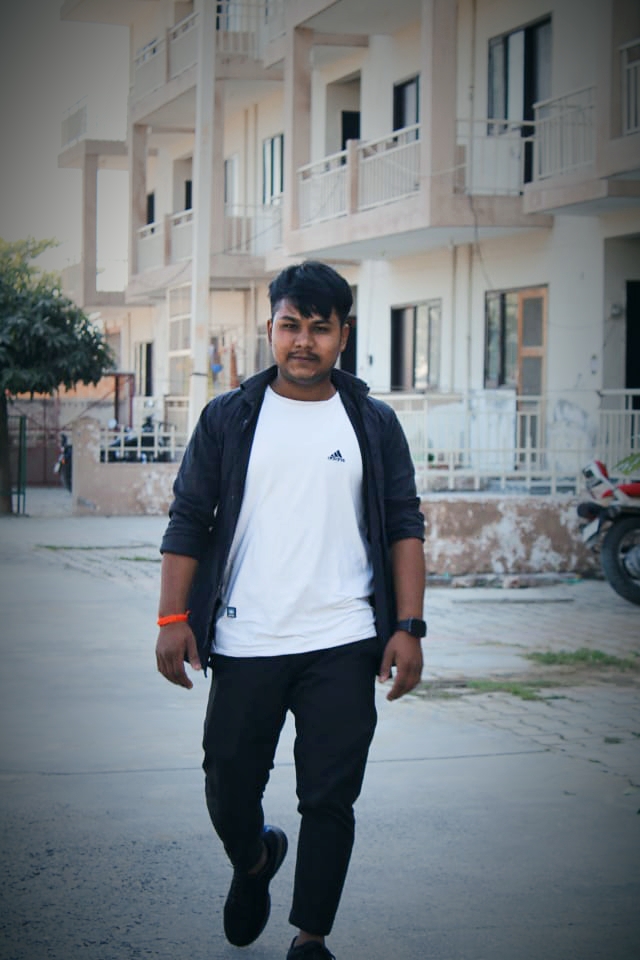