React Guide - Hooks...
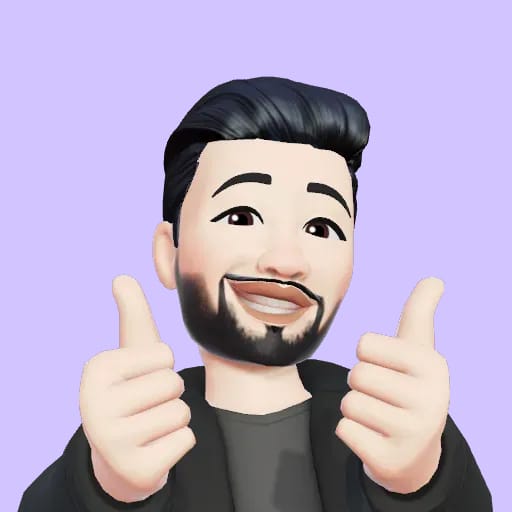
Understanding Hooks
Hooks are functions that provide access to React features from functional components. They cannot be called inside conditional statements or loops, and they should always be called at the top level of a component. With Hooks, you can manage state and access context within functional components, previously only possible with class components.
Meet the Essential Hooks:
While several Hooks exist, let's explore the two most fundamental ones:
useState Hook: The useState
Hook is the cornerstone of state management in functional components. It allows you to create state variables within a function and also provides a function to update them.
import React, { useState } from 'react';
function Counter() { const [count, setCount] = useState(0); //Initial state is 0
const handleClick = () => { setCount(count + 1); // Update state using setCount };
return (
You clicked {count} times
Click me
); }
Here, useState
returns an array with the current state (count
) and a function to update it (setCount
). The button click triggers handleClick
, which increments the count and updates the state using setCount
.
Advantages of Using Hooks
Improved Code Readability: Hooks promote a more readable and concise code structure compared to class components.
Reusability: Many Hooks, like
useState
, can be easily reused across components, fostering code sharing and maintainability.Easier Testing: Hooks often make functional components easier to test and isolate compared to class components with complex lifecycles.
Conclusion:
Hooks have become an inseparable part of React development. Their ability to manage state, perform side effects, and access context from functional components empowers developers to build performant and maintainable UIs. Remember, the journey of mastering Hooks is enriching and rewarding, allowing you to create innovative and interactive React applications.
Stay tuned for more insights, tips, and hands-on examples as we continue our React journey together. Happy coding!
Subscribe to my newsletter
Read articles from Aman Raj Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
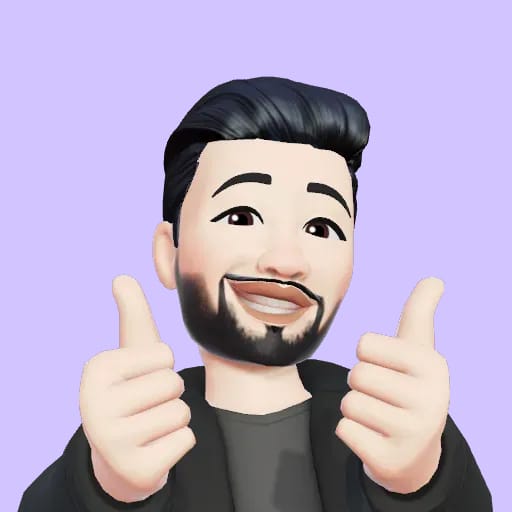